效果图:?
????????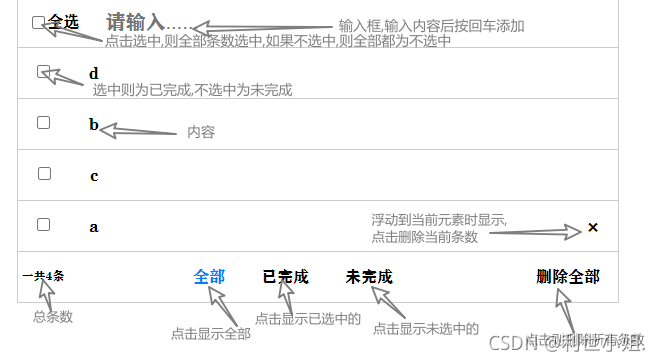
vuex:
import Vue from "vue";
import Vuex from "vuex";
Vue.use(Vuex);
export default new Vuex.Store({
state: {
list: [],
},
mutations: {
add(state, data) {
state.list.unshift({ name: data, com: false, id: new Date().getTime() });
},
qh(state, data) {
state.list.map((item) => {
if (item.id == data) {
// console.log(item.com);
item.com = !item.com;
}
});
},
qbxz(state, data) {
state.list.map((item) => {
item.com = data;
});
},
del(state, data) {
// console.log(data);
// console.log(state.list);
state.list.splice(data, 1);
},
ck(state) {
state.list = [];
},
},
actions: {},
modules: {},
});
HTML:
<template>
<div class="home">
<div class="zt">
<div class="header">
<div class="qx">
<input
type="checkbox"
:checked="qx"
@click="qxss()"
v-show="$store.state.list.length"
/><span v-show="$store.state.list.length">全选</span>
</div>
<div class="add">
<input
@keyup.enter="add()"
v-model="sr"
type="text"
placeholder="请输入......"
/>
</div>
</div>
<div class="content" v-for="(items, keys) in showlist" :key="keys">
<div class="nr">
<div class="xz">
<input type="checkbox" :checked="items.com" @click="xs(items.id)" />
</div>
<div class="context" v-if="!items.com">{{ items.name }}</div>
<div class="context" v-if="items.com">
<del style="color: #ccc">{{ items.name }}</del>
</div>
</div>
<div class="del" @click="delc(keys)"><span>×</span></div>
</div>
<div
style="
width: 100%;
height: 50px;
line-height: 50px;
text-align: center;
border-bottom: 1px solid #ccc;
background: #fff;
"
v-if="!$store.state.list.length"
>
暂无内容~~~~~
</div>
<div class="foot">
<div class="ts">一共{{ $store.state.list.length }}条</div>
<div class="sx">
<router-link
tag="div"
:class="{ active: mr == 'all' }"
:to="{ name: 'Home', query: { tab: 'all' } }"
>全部</router-link
>
<router-link
tag="div"
:class="{ active: mr == 'yes' }"
:to="{ name: 'Home', query: { tab: 'yes' } }"
>已完成</router-link
>
<router-link
tag="div"
:class="{ active: mr == 'no' }"
:to="{ name: 'Home', query: { tab: 'no' } }"
>未完成</router-link
>
</div>
<div
class="all"
style="cursor: pointer"
@click="$store.commit('ck'), (qx = false)"
>
删除全部
</div>
</div>
</div>
</div>
</template>
CSS:
.active {
color: #007fff;
}
.zt {
width: 600px;
height: auto;
background: #eee;
margin: 0 auto;
border: 1px solid #ccc;
}
.zt > .header {
width: 100%;
height: 50px;
background: #fff;
display: flex;
align-items: center;
justify-content: space-around;
/* overflow:hidden; */
border-bottom: 1px solid #ccc;
/* box-sizing: border-box;
-moz-box-sizing: border-box;
-webkit-box-sizing: border-box; */
}
.header > .qx {
width: 50px;
height: 100%;
/* background:steelblue; */
display: flex;
align-items: center;
justify-content: space-around;
}
.header > .add {
width: 500px;
height: 100%;
/* background:springgreen; */
}
.header > .add > input {
height: 100%;
width: 100%;
border: 0px;
outline: 0px;
font-size: 20px;
}
.content {
width: 100%;
height: 50px;
background: #fff;
border-bottom: 1px solid #ccc;
display: flex;
justify-content: space-between;
}
.content > .nr {
width: 100px;
height: 100%;
display: flex;
justify-content: space-around;
align-items: center;
/* background:red; */
}
.content > .del {
width: 50px;
height: 50px;
/* background:red; */
text-align: center;
line-height: 50px;
font-size: 20px;
}
.content > .del > span {
opacity: 0;
}
.content > .del:hover {
font-size: 25px;
font-weight: bold;
cursor: pointer;
}
.content:hover > .del > span {
opacity: 1;
}
.foot {
width: 100%;
background: #fff;
height: 50px;
display: flex;
justify-content: space-between;
}
.ts {
width: 50px;
height: 50px;
line-height: 50px;
text-align: center;
font-size: 12px;
}
.sx {
width: 200px;
height: 50px;
/* background:teal; */
display: flex;
justify-content: space-between;
align-items: center;
}
.sx > div {
cursor: pointer;
}
.all {
width: 100px;
height: 50px;
text-align: center;
line-height: 50px;
}
.context {
font-size: 17px;
}
JS:
export default {
data() {
return {
sr: "",
mr: "all",
qx: false,
};
},
methods: {
add() {
if (this.sr.trim()) {
this.$store.commit("add", this.sr);
let res = this.$store.state.list.every((item) => item.com == true);
this.qx = res;
}
this.sr = "";
},
xs(a) {
this.$store.commit("qh", a);
let res = this.$store.state.list.every((item) => item.com == true);
this.qx = res;
},
qxss() {
this.qx = !this.qx;
this.$store.commit("qbxz", this.qx);
},
delc(a) {
this.$store.commit("del", a);
if (!this.$store.state.list.length) {
this.qx = false;
}
let res = this.$store.state.list.every((item) => item.com == true);
this.qx = res;
},
},
computed: {
showlist() {
if (this.mr == "all") {
return this.$store.state.list;
}
if (this.mr == "yes") {
return this.$store.state.list.filter((item) => item.com);
}
if (this.mr == "no") {
return this.$store.state.list.filter((item) => !item.com);
}
},
},
watch: {
$route: {
handler(value) {
// console.log(value.query.tab);
if (!value.query.tab) {
this.mr = "all";
} else {
this.mr = value.query.tab;
}
},
immediate: true,
},
},
created() {
console.log(new Date().getTime());
},
};
|