String是对应的引用类型。创建一个String对象,要通过String构造函数进行创建
例:
var str = 'world';
var strobj = new String("hello");
一、基本的使用
1.获取String对象的原始值
Object对象通过valueOf()、toString()、toLocaleString()获取字符串对象的返回一个原始值
strobj = new String("hello");
console.log(typeof strobj); // object
console.log(strobj.valueOf()); // hello
console.log(typeof strobj.valueOf()) //string
console.log(strobj.toString());// hello
console.log(typeof strobj.toString()) //string
console.log(strobj.toLocaleString()); // hello
console.log(typeof strobj.toLocaleString()) //string
2.获取字符串的长度
var a = "hello world"
console.log(a.length)? //11
通过下标访问单个字符
console.log(a.[2] )? // 'l'? == a.charAt(2)
二、字符串中常用的方法
2.1?通过字符串查询索引
indexOf 和 lastIndexOf?
参数:
第一个参数是需要查询的字符串
第二个参数是开始查询的索引
返回值:
查询到了:返回第一个查询的字符下标
查询不到:返回-1
search() 和 indexOf 一样,但是没有第二个参数
例子👇
var str = "hello world"
console.log(str.indexOf('l')) //2
console.log(str.lastIndexOf('l')) //9
console.log(str.indexOf('z')) //-1
2.2 字符串截取的方法
2.2.1 substr()??截取字符串
用法:
str.substr(start[,num]);
参数:
第一个参数 : 开始截取的下标
第二个参数 :截取的个数
返回值:
返回截取的部分
不改表原字符串
代码例👇
var str = 'helloworldwotoplay';
console.log(str.substr(2,5));// cdefg 从下标为2的地方开始,截取5个字符
console.log(str.substr(2));// cdefgh 从下标为2的地方开始,截取剩余所有字
2.2.2substring() 截取字符串
用法:
str.substring(start[,end]);
参数:
第一个参数 : 开始截取的下标
第二个参数 : 结束截取的下标
返回值:
返回截取的部分
不改表原字符串
var str = 'helloworldwotoplay';
console.log(str.substring(2,5)); // llo 从下标为2的地方开始,截取5个字符
2.2.3 slice() 截取
用法:
str.slice(start[,end]);
参数:
第一个参数 : 开始截取的下标(如果为负数,截取全部字符)
第二个参数 : 截取的字符串不包含end索引对应的字符,可以理解截取了(end- start)个字符,
返回值:
返回截取的部分
不改表原字符串
substring和slice的区别
slice支持负数索引 str.slice(-2,-1) ==> 从索引-2开始,截取-1-(-2)个字符
2.3concat() 拼接字符串
用法:
str.concat(str1,str2....,strn);
参数:
需要拼接的字符串
返回值:
返回拼接后的新字符串
不改表原字符串
代码例👇
str1 = 'str';
str2 = 'ing';
str3 = '无敌';
let newstr = str1.concat(str2,str3);
console.log(newstr) ; //string无敌
2.4替换字符串
replace()和replaceAll()
用法:
str.concat(str1,str2....,strn);
参数:
第一个参数 : 需要替换的字符串。
第二个参数 : 替换后的字符串。
返回值:
返回替换后的新字符串
不改表原字符串
区别:
replace(只替换第一个满足要求字符串)
replaceAll(只替全部满足要求字符串)
代码例👇
var str = "hello world"
console.log(str.replace('l','o')) //heolo world
console.log(str.replaceAll('l','o')) //heooo worod
2.5 split() 字符串切割为数组
用法:
str.split('分隔符');
参数:
str通过分隔符进行分割
返回值:
返回分割后的新数组
不改表原字符串
代码例👇
var str = "hello world"
console.log(str.split('o'))
图例👇
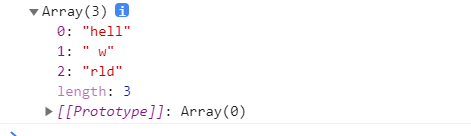
?2.6英文大小写
toLowerCase(),将英文字符转成小写
toUpperCase(),将引文字符转成大写
var str = 'Hello Wrold'
console.log(str.toLocaleLowerCase()); //hello wrold
console.log(str.toLocaleUpperCase()); //HELLO WROLD
2.7去除空格trim()
str.trimLeft() == 去除字符串开始的空格 str.trimRight() ==?去除字符串结束的空格 str.trim() == 去除字符串开始和结束的空格
无奈源于不够强大
|