1.介绍
Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式。 它采用集中式存储管理应用的所有组件的状态,并以相应的规则保证状态以一种可预测的方式发生变化。 应用场景: 同级别组件之间通讯
let comA = {}
let comB = {}
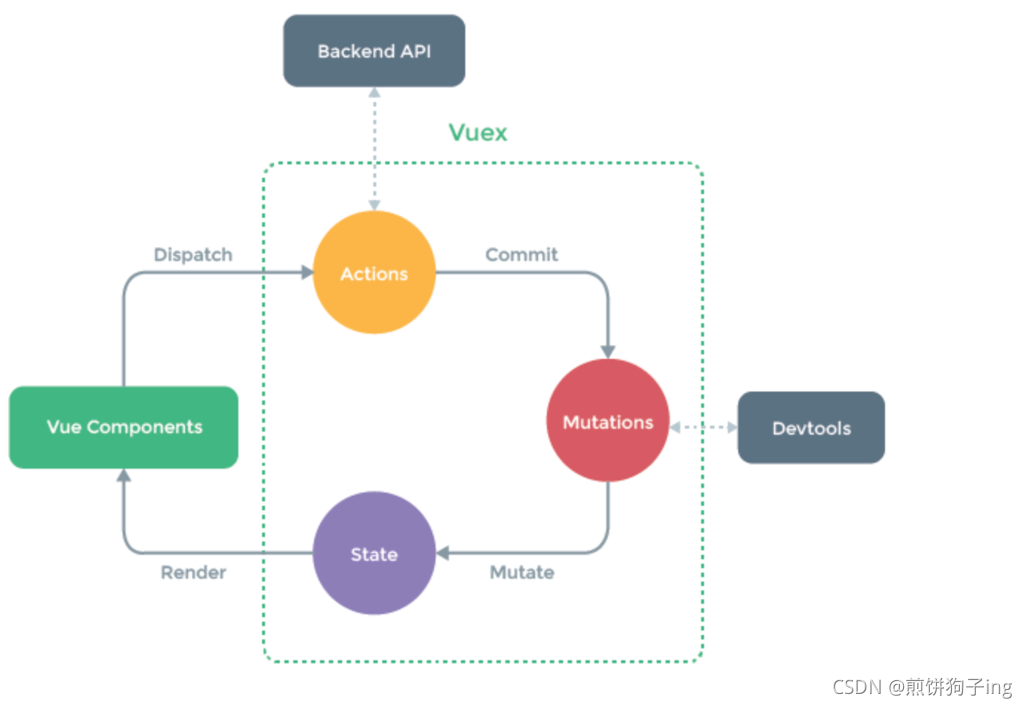
2.基本使用:
Vuex 使用单一状态树,用一个对象就包含了全部的应用层级状态,作为一个“唯一数据源”而存在,这也意味着,每个应用将仅仅包含一个 store 实例。Vuex 通过 store 选项,提供了一种机制将状态从根组件“注入”到每一个子组件中(需调用Vue.use(Vuex)) ,通过在根实例中注册 store 选项,该 store 实例会注入到根组件下的所有子组件中,且子组件能通过 this.$store 访问到。 1)State(类似于vue实例中data) 存储数据的地方,将每个vue实例中的公共变量抽取出来进行统一管理
state: {
msg: 'hello vuex'
}
在vue中,通过计算属性获取存储在vuex的状态,可以直接获取,也可以通过辅助函数获取
computed: {
count() {
return this.$store.state.count
}
...Vuex.mapState(["count"])
}
2)Getter(类似于vue实例中的computed) 用于将state中静态的数据经过计算再返回 getter也可以返回一个函数,来实现给 getter 传参。在你对store里的数组进行查询时非常有用。
getter: {
reMsg(state) {
return state.msg.toUpperCase()
}
}
在vue中,可以通过计算属性访问
computed: {
reMsg() {
return this.$store.getters.reMsg
}
...Vuex.mapGetters(["reMsg"])
}
3)Mutation(突变) 更改Vuex的store中的状态的唯一方法是提交 mutation,必须是同步操作。 mutation函数的第一个参数为state ,第二个参数是来接受在调用mutation的时候用户传递的实参
mutations: {
SET_MSG(state, payload) {
state.msg = payload
}
}
可以在vue生命周期钩子中提交突变或者在methods中映射突变方法
created() {
this.$store.commit('SET_MSG', 'hello mutation')
}
methods:{
...Vuex.mapMutations(['SET_MSG'])
}
4)actions(动作) 用来存放异步请求方法,action提交的是 mutation,从而间接的变更state中的状态 actions中的异步请求接受与store实例具有相同方法和属性的一个形参-context (上下文对象) 可使用context.state获取state 可使用context.getters获取getters 可使用context.commit()提交突变 可使用context.dispatch()分发动作
actions: {
async findAll(context) {
let res = await axios.get('http://...');
context.commit('SET_MSG', res.data.data)
}
}
可以在vue生命周期钩子中分发动作或者在methods中映射actions中的方法
created() {
this.$store.dispatch('findAll')
}
methods:{
...Vuex.mapActions(['findAll'])
}
3.辅助函数
Vuex构造函数内部提供了快捷映射数据、方法的方法 使用对象的解构,将内部的辅助函数解构出来
let { mapState, mapGetters, mapMutations, mapActions } = Vuex
使用
computed: {
...mapState(['msg']),
...mapGetters(['reMsg'])
},
methods: {
...mapMutations(['SET_MSG']),
...mapActions(['findAll'])
}
4.模块化开发
1)目的 由于使用单一状态树,应用的所有状态会集中到一个比较大的对象。 当应用变得非常复杂时,store 对象就有可能变得相当臃肿。 为了解决以上问题,Vuex 允许我们将 store 分割成模块(module)。 每个模块拥有自己的 state、mutation、action、getter、甚至是嵌套子模块。 namespaced表示设置命名空间 使用过程:
<div id="app">
<h3>{{data1}}</h3>
<h4>vuex-state-{{msg}}</h4>
<ul>
<li v-for="(item, index) in categories" :key="index">
栏目编号: {{item.id}}
栏目名称: {{item.name}}
</li>
</ul>
</div>
<script>
let store = new Vuex.Store({
state: {
msg: 'this is vuex data',
categories: []
},
mutations: {
SET_MSG(state, payload) {
state.msg = payload
},
SET_CATEGORIES(state, payload) {
state.categories = payload
}
},
actions: {
async findAllCategories(context) {
let res = {
status: 200,
message: '查询成功',
data: [
{ id: 1, name: '校园新闻' },
{ id: 2, name: '热点新闻' },
{ id: 3, name: '娱乐新闻' },
{ id: 4, name: '资讯快报' },
],
timestamp: 1634194101227
}
console.log(context);
context.commit('SET_CATEGORIES', res.data)
}
}
})
let vm = new Vue({
el: '#app',
data: {
data1: 'this is vm data'
},
computed: {
msg() {
return this.$store.state.msg
},
categories() {
return this.$store.state.categories
},
},
methods: {},
created() {
this.$store.dispatch('findAllCategories')
},
store: store
})
</script>
|