轮播图在前端开发中我认为是一个比较重要的点,因为包含了很多原生js知识点,以下是我学习制作轮播图的过程
难点:
- 如何让底下圆圈和图片所对应自动动态生成
- 如何让底下圆圈和图片所对应的起来
- 上一页和下一页所在盒子所移动的距离
- 图片切换时的渐出动画效果
- 节流阀的概念
效果:
?
?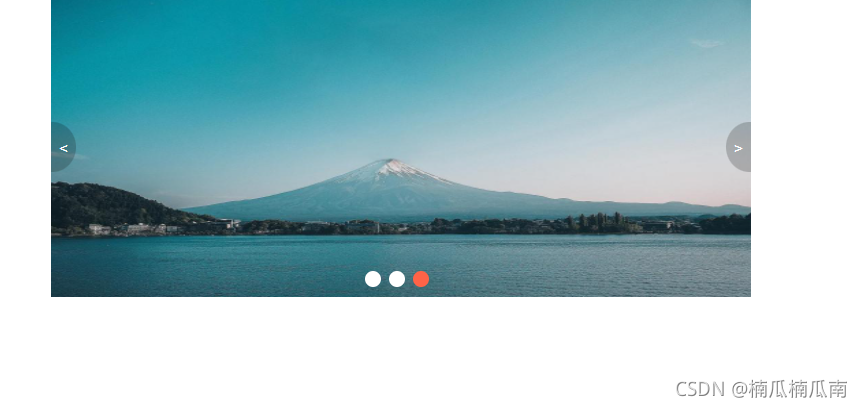
代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
padding: 0;
margin: 0;
}
a {
text-decoration: none;
color: white;
line-height: 50px;
text-align: center;
}
li {
list-style: none;
}
.tb-promo {
position: relative;
width: 700px;
height: 300px;
margin: auto;
overflow: hidden;
}
.tb-promo .imgg {
position: absolute;
top: 0;
left: 0;
width: 3000px;
}
.tb-promo .imgg li {
float: left;
}
.tb-promo .imgg li img {
width: 700px;
height: 300px;
}
.tb-promo .prev {
display: none;
position: absolute;
top: 125px;
left: 0;
width: 25px;
height: 50px;
background-color: rgba(0, 0, 0, 0.2);
border-top-right-radius: 25px;
border-bottom-right-radius: 25px;
z-index: 999;
}
.tb-promo .prev:hover {
background-color: rgba(0, 0, 0, 0.5);
}
.tb-promo .next {
display: none;
position: absolute;
top: 125px;
right: 0;
width: 25px;
height: 50px;
background-color: rgba(0, 0, 0, 0.2);
border-top-left-radius: 25px;
border-bottom-left-radius: 25px;
z-index: 999;
}
.tb-promo .next:hover {
background-color: rgba(0, 0, 0, 0.5);
}
.tb-promo .promo-nav {
position: absolute;
top: 270px;
left: 50%;
margin-left: -40px;
height: 25px;
}
.tb-promo .promo-nav li {
float: left;
width: 16px;
height: 16px;
background-color: white;
border-radius: 8px;
margin: 4px;
}
.tb-promo .promo-nav .one {
background-color: tomato;
}
</style>
</head>
<body>
<div class="tb-promo">
<a href="javascript:;" class="prev"><</a>
<a href="javascript:;" class="next">></a>
<ul class="imgg">
<li><img src="./61.jpeg" alt=""></li>
<li><img src="./62.jpeg" alt=""></li>
<li><img src="./63.jpeg" alt=""></li>
</ul>
<ol class="promo-nav">
</ol>
</div>
<script>
var prev = document.querySelector('.prev');
var next = document.querySelector('.next');
var tbpromo = document.querySelector('.tb-promo');
var ul = document.querySelector('ul');
var ol = document.querySelector('ol');
//动画函数
function animate(obj, target) {
clearInterval(obj.timer);//调用防止点击多次调用
obj.timer = setInterval(function () {
var step = (target - obj.offsetLeft) / 10;
step = step > 0 ? Math.ceil(step) : Math.floor(step); //正直和负值取整
if (obj.offsetLeft == target) {
clearInterval(obj.timer);
} else {
obj.style.left = obj.offsetLeft + step + 'px';
}
}, 10)
}
//生成动态导航圈圈
var tbpromWidth = tbpromo.offsetWidth;
for (var i = 0; i < ul.children.length; i++) {
var li = document.createElement('li');
ol.appendChild(li);
//记录索引号 通过自定义属性
li.setAttribute('index', i);
//排他思想 写圆圈变色
li.addEventListener('click', function () {
//清除所有圈圈颜色
for (var i = 0; i < ol.children.length; i++) {
ol.children[i].className = '';
}
this.className = 'one';
var index = this.getAttribute('index');
//点击li 把li 的索引号分别给控制变量
num = index;
circle=index;
animate(ul, -index * tbpromWidth);
})
ol.children[0].className = 'one';
}
//克隆第一张图片li放在最后面 无缝切换
var frist = ul.children[0].cloneNode(true);
ul.appendChild(frist);
//隐藏显示 下一页上一页
tbpromo.addEventListener('mouseenter', function () {
prev.style.display = 'block';
next.style.display = 'block';
clearInterval(timer);
timer=0;//清除定时器变量
})
tbpromo.addEventListener('mouseleave', function () {
prev.style.display = 'none';
next.style.display = 'none';
timer=setInterval(function () {
next.click();//手动调动点击事件
},1500)
})
//next prev动画
var num = 0;
//控制圆圈
var circle = 0;
//节流阀变量
var flag=true;
//下一页
next.addEventListener('click', function () {
//最后一张进行判断 ul复原 left为0
if (num == (ul.children.length - 1)) {
ul.style.left = 0;
num = 0;
}
num++;
animate(ul, -num * tbpromWidth);
circle++;
if (circle == 3) {
circle = 0;
}
for (var i = 0; i < ol.children.length; i++) {
ol.children[i].className = '';
}
ol.children[circle].className = 'one';
})
//上一页
prev.addEventListener('click', function () {
if (num == 0) {
num = ul.children.length-1;
ul.style.left = -num*tbpromWidth+'px';
}
else {
num--;
animate(ul, -num * tbpromWidth);
circle--;
if (circle <0) {
circle = 2;
}
for (var i = 0; i < ol.children.length; i++) {
ol.children[i].className = '';
}
ol.children[circle].className = 'one';
}
})
//自动播放
var timer=setInterval(function () {
next.click();//手动调动点击事件
},2000)
</script>
</body>
</html>
?
?
|