题目
1 .制作一个复利计算器。创建一个页面,允许输入银行存款总额以及存款利率,然后计算出在给定时间段后,钱会变成多少。
效果如下: 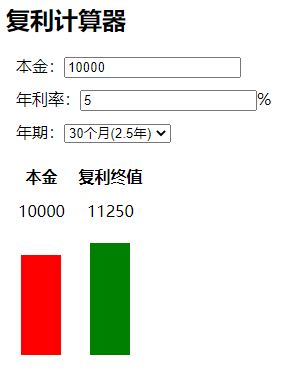
1.1 解题思路:
通过document.forms 获取到表单集合,将获取到的集合用一个变量来接收, 例如:
const form = document.forms.form; const baseField = form.elements[‘base’];//获取到表单中name="base"的集合
分别获取到值后,给整个表单添加input事件,进行判断,具体的方式,写在了代码中。 代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
form div {
margin: 10px;
}
thead {
text-align: center;
font-weight: 700;
}
#one {
width: 40px;
height: 100px;
background-color: red;
}
#two {
width: 40px;
height: 100px;
background-color: green;
}
table td {
text-align: center;
padding: 10px;
vertical-align: bottom;
}
</style>
</head>
<body>
<h1>复利计算器</h1>
<form action="" name="form">
<div>
本金:
<input type="text" name="base" id="" />
</div>
<div>
年利率:
<input type="number" name="expone" id="" />
</div>
<div>
年期:
<select name="time" id="">
<option value="0.25">3个月(0.25年)</option>
<option value="0.5">6个月(0.5年)</option>
<option value="1">12个月(1年)</option>
<option value="1.5">18个月(1.5年)</option>
<option value="2">24个月(2年)</option>
<option value="2.5">30个月(2.5年)</option>
<option value="3">36个月(3年)</option>
<option value="5">60个月(5年)</option>
</select>
</div>
</form>
<table>
<thead>
<th>本金</th>
<th>复利终值</th>
</thead>
<tbody>
<tr>
<td id="capital"></td>
<td id="compound"></td>
</tr>
<tr>
<td>
<div id="one"></div>
</td>
<td>
<div id="two"></div>
</td>
</tr>
</tbody>
</table>
<script>
const form = document.forms.form;
console.log(form);
const baseField = form.elements['base'];
console.log(baseField);
const exponeField = form.elements['expone'];
console.log(exponeField);
const timeField = form.elements['time'];
console.log(timeField);
form.addEventListener('input', () => {
const capital = document.getElementById('capital');
const compound = document.getElementById('compound');
const two = document.getElementById('two');
if (baseField.value != '') {
const getCompound = function getCompound(base, expone, time) {
if (expone != '' && base != '') {
capital.innerHTML = baseField.value;
const result = Math.round(base * (1 + expone * time));
let height = result / base * 100 + 'px';
two.style.height = height;
return result;
} else {
return '';
}
};
compound.innerHTML = getCompound(baseField.value, exponeField.value, timeField.value * 0.01);
}
}
)
</script>
</body>
</html>
|