vue学习记录(2)
页面
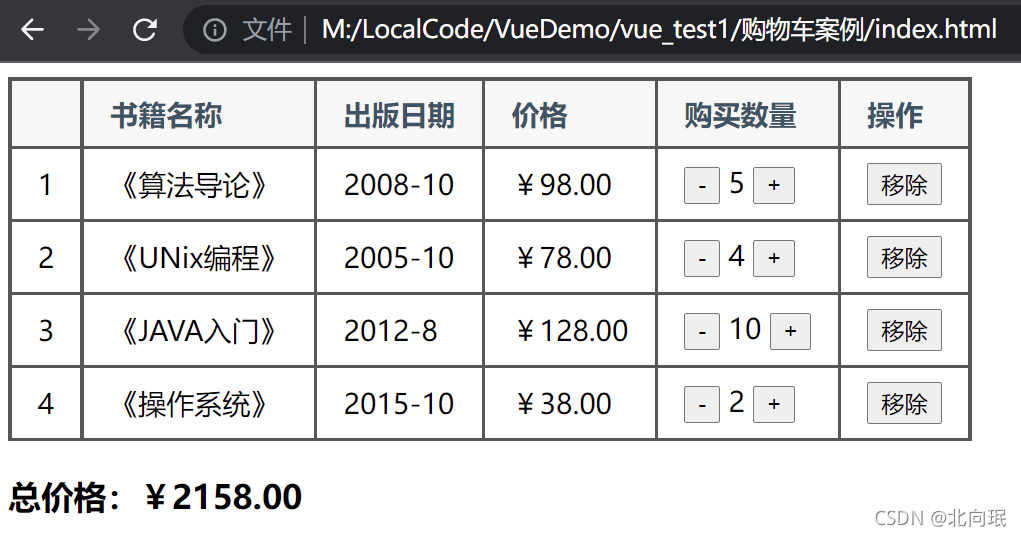 功能:实现数量自由增减,减到1不可再减,全部移除后显示”购物车为空“,价格根据数量和书本单价进行计算。
html
<div id="table">
<div v-if="books.length">
<table>
<thead>
<tr>
<th></th>
<th>书籍名称</th>
<th>出版日期</th>
<th>价格</th>
<th>购买数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item,index) in books">
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{{item.date}}</td>
<td>{{item.price | showPrice}}</td>
<td><button @click="decrement(index)" v-bind:disabled="item.count<=1">-</button>
{{item.count}}
<button @click="increment(index)">+</button>
</td>
<td><button @click="removeClick(index)">移除</button></td>
</tr>
</tbody>
</table>
<h3>总价格:{{totalPrice | showPrice}}</h3>
</div>
<h2 v-else>购物车为空</h2>
</div>
css
table{
border:2px solid #e9e9e9;
border-collapse:collapse;
border-spacing:0;
}
th,td{
padding:8px 15px;
border:2px solid #575656;
text-align:left;
}
th{
background-color: #f7f7f7;
color:#3c5366;
font-weight:600;
}
1.价格保留两位小数(使用过滤器实现) html
<td>{{item.price | showPrice}}</td>
js
filters: {
showPrice (price) {
return '¥' + price.toFixed(2)
}
}
toFixed():把数字转换为字符串,结果的小数点后有指定位数的数字 var num = 5.56789; var n=num.toFixed(2); //n=5.57(四舍五入)
2.价格保留两位小数(使用方法实现) html
<td>{{getFinalPrice(item.price)}}</td>
js
getFinalPrice (price) {
return '¥' + price.toFixed(2)
3.实现数量增减,减到1时不能再减 html
<td><button @click="decrement(index)" v-bind:disabled="item.count<=1">-</button>
{{item.count}}
<button @click="increment(index)">+</button>
</td>
js
increment (index) {
console.log('increment!', index)
this.books[index].count++
},
decrement (index) {
console.log('decrement!', index)
this.books[index].count--
},
4.当全部移除时,显示“购物车为空” html
<div v-if="books.length">
<table>...</table>
<td><button @click="removeClick(index)">移除</button></td>
</div>
<h2 v-else>购物车为空</h2>
js
removeClick (index) {
this.books.splice(index, 1)
}
5.根据增减自动计算总价格 html
<h3>总价格:{{totalPrice | showPrice}}</h3>
js
computed: {
totalPrice () {
let totalPrice = 0
for (let i = 0; i < this.books.length; i++) {
totalPrice += this.books[i].price * this.books[i].count
}
return totalPrice
导入其他文件:
//写在head
<link rel="stylesheet" href="style.css">
//写在body尾部
<script src="vue.js"></script>
<script src="main.js"></script>
js里的data:
books: [
{
id: 1,
name: '《算法导论》',
date: '2008-10',
price: 98.00,
count: 5
},
{
id: 2,
name: '《UNix编程》',
date: '2005-10',
price: 78.00,
count: 4
},
{
id: 3,
name: '《JAVA入门》',
date: '2012-8',
price: 128.00,
count: 10
},
{
id: 4,
name: '《操作系统》',
date: '2015-10',
price: 38.00,
count: 2
}
]
|