?props的传递是异步的
案例如下:
父组件
<template>
<div class="approvals-container">
<Son :id="num" ref="refSon" />
<button @click="changeID">修改id</button>
</div>
</template>
<script>
import Son from './son'
export default {
components: { Son },
data() {
return {
num: 1
}
},
methods: {
changeID() {
this.num = 2
console.log(this.$refs.refSon.id) // 通过 ref 的引用方式拿到子组件的 id值看看是多少?
this.$refs.refSon.doSomething() // 假如发送ajax
}
}
}
</script>
子组件
<template>
<div>
props:{{ id }}
</div>
</template>
<script>
export default {
props: {
id: {
type: Number,
required: true
}
},
methods: {
doSomething() {
console.log('发ajax', this.id)
}
}
}
</script>
当我们点击父组件的修改 修改id 按钮 时,
1. 修改 num?的值,并传给子组件的 id 属性
2. 通过 ref 的引用方式拿到子组件的 id值看看是多少?
3. 假如我们发ajax
结果如下:
修改 id前:
可以看到子组件中的 props 的 id 值是 1
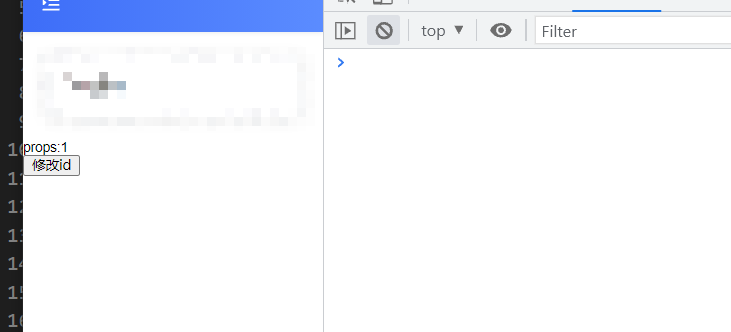
?修改 id 后
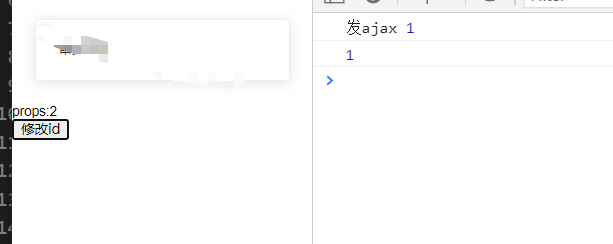
?点击修改 id 后可以看到
通过 ref 的引用方式拿到子组件的 id值和ajax 的值还是1?
但是插槽的 id 值却是2
解决方法
父组件的? ?this.$refs.refSon.doSomething()
用??this.$nextTick(() => { } 包裹起来,这样就解决了问题
?this.$nextTick(()=>{ })? 解释如下:?
?this.$nextTick(()=>{ }) 将回调函数中的操作放到下一次DOM更新之后执行,类似全局方法vue.nextTick,只不过 this.$nextTick 只作用于调用它的实例
<template>
<div class="approvals-container">
<Son :id="num" ref="refSon" />
<button @click="changeID">修改id</button>
</div>
</template>
<script>
import Son from './son'
export default {
components: { Son },
data() {
return {
num: 1
}
},
methods: {
changeID() {
this.num = 2
this.$nextTick(() => {
this.$refs.refSon.doSomething()
console.log(this.$refs.refSon.id)
})
}
}
}
</script>
结果如下:
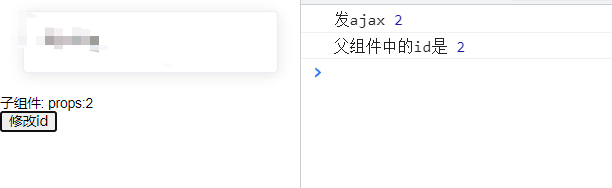
|