1. 什么是vuex?
Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式。它采用集中式存储 管理应用所有组件的状态,并以相应的规则保证状态以一种可预测的方式发生变化
2. vuex能做什么
存储组件公共数据
?3. vuex的核心
-
state 存储数据 -
mutations 存储方法 用来直接操作state中的数据 -
actions 存储方法 调用mutations中的方法 里面的方法返回的是promise对象可以实现异步操作 -
getters 对state中的数据做逻辑计算 类似于computed -
modules 模块存储 -
plugins 插件(持久化存储)
?4.?vuex的运行原理
?在组件中通过dispatch来调用actions中的方法,在actions中通过commit来调用mutations中的方法,在mutaions中的方法中可以直接操作state中的数据,只要state中的数据发生改变,就会立即响应到所有的组件上
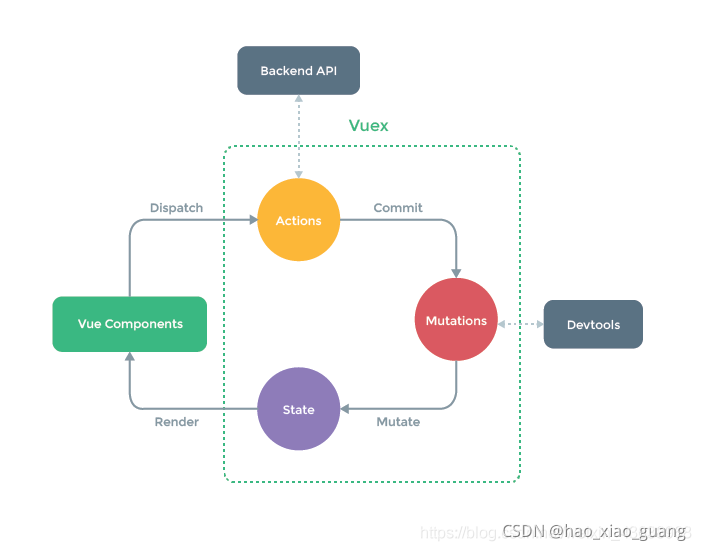
5.?如何使用
基本使用:
在vue的原型上有一个$store对象可以调用vuex的任何东西?
-
state this.$store.state -
mutations this.$store.commit("方法名",参数) 参数只能传一个 -
actions this.$store.dispatch("方法名",参数) -
getters this.$store.getters -
module this.$store.模块名.state
export default new Vuex.Store({
state: {
},
mutations: {
},
actions: {
},
modules: {
}
})
使用映射函数:
将vuex的中的成员映射到组件中然后使用
mapState mapMutations mapGetters mapActions
步骤:
import { mapActions, mapGetters, mapMutations, mapState } from 'vuex'
import {createNamespacedHelpers} from 'vuex'
const {mapState:mapStateUser,mapActions:mapActionUser,mapMutations:mapMutaionuser} = createNamespacedHelpers('user')
const {mapState:mapStateCart,mapActions:mapActionCart,mapMutations:mapMutaionCart} = createNamespacedHelpers('cart')
export default {
name: '',
data() {
return {}
},
computed: {
...mapState({
token: 'token'
}),
...mapGetters(['token-x']),
...mapSateUser(['userid']),
...mapStateCart({cartid:'userid'})
},
//生命周期 - 创建完成(访问当前this实例)
created() {
this.setToken('123456')
},
//生命周期 - 挂载完成(访问DOM元素)
mounted() {},
methods: {
...mapActions({
setToken: 'setToken'
}),
...mapMutations(['SET_TOKEN']),
...mapMutaionUser({
setId:"setToken"
})
}
}
6. vuex的持久化
-
就是将vuex的数据存储在本地(localStorage sessionStorage)
7. vuex的优缺点 ?
vuex的优点:
????????js 原生的数据对象写法, 比起 localStorage 不需要做转换, 使用方便 ? 属于 vue 生态一环, 能够触发响应式的渲染页面更新 (localStorage 就不会) ? 限定了一种可预测的方式改变数据, 避免大项目中, 数据不小心的污染 ?
vuex的缺点:
????????刷新浏览器,vuex中的state会重新变为初始状态 ? 解决方案-插件vuex-persistedstate
8.??模块化管理数据 (modules)
1.?什么时候需要用到模块 管理vuex数据。
项目庞大,数据信息量特别大的时候,我们可以考虑分模块形式管理数据,比如user模块管理用户信息数据,cart模块管理购物车数据,shop模块管理商品信息数据。
import vue from 'vue'
import Vuex from 'vuex'
Vue.use(vuex);
const state= ()=>{ token:''}
const actions = {
set_token({commit},val){
commit("to_token",val)
}
}
const mutations = {
to_token(state,val){
state.token=val;
}
}
const getters = {}
//user模块
let user = {
namespaced: true, //一定要开始命名空间。
state: { userid: 1234 },
actions: {
},
mutations: {
SET_USERID(state, val) {
state.userid = val;
}
},
getters: {
}
}
//购物车数据的模块
let cart = {
namespaced: true,
state: { userid: 567 },
actions: {
},
mutations: {
},
getters: {
}
}
const store = new Vuex.Store({
state,
mutations,
actions,
getters,
modules: {
user,
cart
},
plugins: [createPersistedState({
storage: sessionStorage,
key: "token"
})]//会自动保存创建的状态。刷新还在
})
export default store
?
|