前言
写在前面的话:本篇文章主要讲到的知识点是如何通过原生JavaScript 实现 Ajax,首先就需要对Json、HTTP网络传输协议、网络请求方式、异步编程等有一点点的了解;
1、实现步骤
- 创建
XMLHttpRequest 对象; - 通过
onreadystatechange 事件判断请求是否成功; - 请求配置
open() - 发送请求
send()
1.1 创建 XMLHttpRequest 对象
XMLHttpRequest是一个构造函数,创建该对象的实例化,可以使用构造函数的方式:
let xhr;
xhr = new XMLHttpRequest();
现在很多的浏览器都已经有内置的 XMLHttpRequest 对象,但是为了兼容老版本的 浏览器,还有下面这个写法:
let xhr;
new ActiveXObject("Microsoft.XMLHTTP");
1.2 通过 onreadystatechange 事件判断请求是否成功
在 Ajax 异步通信中,需要客户端向后端发起请求,在这个过程中会涉及到 TCP 协议中的 三次握手四次挥手 规则; ? 使用 XMLHttpRequest 的 onreadystatechange 事件可以判断当前事务的请求进度以及请求状态
xhr.onreadystatechange = function(){
if(xhr.readyState != 4){ // 当 readyState 值等于 4 的时候 请求完成
return
}
if(xhr.status == 200){ // 当 status 的值等于 200 的时候,请求成功
console.log(xhr.resposeText); // 收到后端返回的数据
} else {
console.log("请求失败", xhr);
}
}
2.3 请求配置 open()
前端向后端发送请求需要使用到 XMLHttpRequest 对象中的 open() 方法进行请求配置:
xhr.open(method, url, async);
参数解释:
- method:请求方式(“POST”、“GET”、“HEAD”、“PUT”、“DELETE”);
- url:请求地址(通过与后端开发人员沟通后的接口文档确定);
- async:布尔值(是否异步请求,true代表 异步请求,false代表不使用异步请求,一般使用异步);
2.4 发送请求 send()
前端向后端发送请求则需要使用到 XMLHttpRequest 对象中的 send() 方法进行发送:
xhr.send(String);
参数解释:
注意:
- 当请求类型为 “POST” 的时候,该参数是 “POST” 请求所携带的数据信息(如果没有数据信息,也可以填写 “null”);
- 当请求类型为 “GET” 类型的时候,该参数填写为 “null” 因为,“GET” 请求类型的数据信息是必须写在 url 请求地址中的,并以 “?” 开头,以 “&” 连接,如:?key=value&key=value
2、综合演示
通过 Promise 对象处理 Ajax 的异步操作,关于XMLHttpRequest的 status值,网虫找到一篇精简版本的解释说明:
function myAjax({
method = "GET",
url = null,
data = null,
async = true,
timeout = 3000
}) {
return new Promise((resolve, reject) => {
let xhr;
if (window.XMLHttpRequest) {
xhr = new XMLHttpRequest()
} else if (window.ActiveXObject) {
xhr = new ActiveXObject("Microsoft.XMLHTTP")
}
xhr.onreadystatechange = function () {
if (xhr.readyState != 4) {
return
}
if (xhr.status == 200) {
console.log(JSON.parse(xhr.responseText))
resolve(JSON.parse(xhr.responseText))
} else {
reject(xhr)
}
}
xhr.timeout = timeout;
if (method === "GET") {
xhr.open(method, url + "?" + formatData(data), async)
xhr.send(null)
} else if (method === "POST") {
xhr.open(method, async)
xhr.setRequestHeader("Content-type", "application/x-www-form-urlencodeed")
xhr.send(formatData(data))
}
})
}
function formatData(data) {
if (data instanceof Object) {
let arr = []
for (let key in data) {
arr.push(key + "=" + data[key])
}
return arr.join("&")
}
return data
}
相关文章
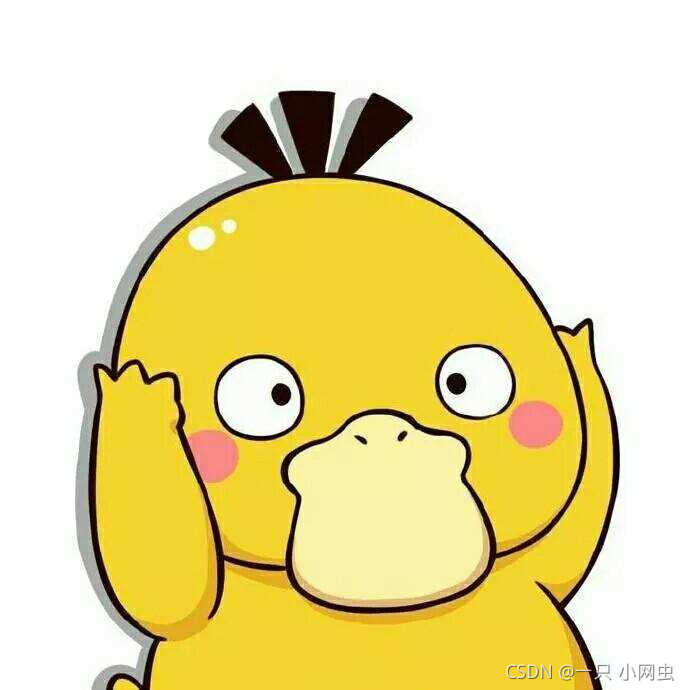
|