html:
<div class="info">
分数<span class="score"></span>
</div>
<div class="gameBox">
<div class="">
<button class="action">开始</button>
</div>
</div>
CSS:
* {
padding: 0;
margin: 0;
}
.info {
text-align: center;
font-size: 30px;
}
.gameBox {
position: relative;
margin: auto;
width: 500px;
height: 600px;
background: #55AAFF;
}
.hero {
position: absolute;
background: #008000;
width: 50px;
height: 50px;
transition: .1s;
}
.enemy {
position: absolute;
background: #FF0000;
width: 30px;
height: 30px;
}
.bullet{
position: absolute;
background: #000000;
width: 5px;
height: 10px;
top: 555px;
}
.action{
position: absolute;
top: 35%;
left: 40%;
width: 100px;
height: 60px;
background: pink;
z-index: 10;
}
JavaScript:
//创建己方飞机
function creathero() {
var hero = document.createElement('div');
hero.className = "hero";
var gameBox = document.querySelector('.gameBox');
gameBox.appendChild(hero);
var y1 = hero.offsetLeft;
hero.style.top = 550 + 'px';
}
function creat() {
//创建敌方飞机
var time1 = setInterval(function() {
var gameBox = document.querySelector('.gameBox');
var width = gameBox.offsetWidth;
var num = Math.floor(Math.random() * (width - 30));
var enemy = document.createElement('div');
enemy.className = "enemy";
var gameBox = document.querySelector('.gameBox');
gameBox.appendChild(enemy);
//var enemy = document.querySelector('.enemy');
enemy.style.left = num + 'px';
}, 800)
var time2 = setInterval(function() {
var enemy = document.querySelectorAll('.enemy')
var i = 0;
for (i; i < enemy.length; i++)
enemy[i].style.top = enemy[i].offsetTop + 2 + 'px';
}, 10);
//创建子弹
var time3 = setInterval(function() {
var hero = document.querySelector('.hero');
var bullet = document.createElement('div');
bullet.className = "bullet";
var gameBox = document.querySelector('.gameBox');
gameBox.appendChild(bullet);
bullet.style.left = hero.offsetLeft + 22.5 + 'px';
}, 300)
var time4 = setInterval(function() {
var bullet = document.querySelectorAll('.bullet')
var i = 0;
for (i; i < bullet.length; i++)
bullet[i].style.top = bullet[i].offsetTop - 2 + 'px';
}, 10);
//打飞机,添加计分器
var number = 0;
var score = document.querySelector('.score');
score.innerHTML = number;
var time5 = setInterval(function() {
var gameBox = document.querySelector('.gameBox');
var bullet = document.querySelectorAll('.bullet');
var enemy = document.querySelectorAll('.enemy');
var j = 0,
k = 0;
for (k; k < enemy.length; k++)
for (j; j < bullet.length; j++) {
if (bullet[j].offsetLeft >= enemy[k].offsetLeft && bullet[j].offsetLeft <= enemy[k].offsetLeft + enemy[k].offsetWidth &&
bullet[j].offsetTop <= enemy[k].offsetTop + enemy[k].offsetHeight) {
gameBox.removeChild(enemy[k]);
gameBox.removeChild(bullet[j]);
number += 10; //增加分数
score.innerHTML = number;
}
}
}, 1);
//己方飞机死亡
var time6 = setInterval(function() {
var gameBox = document.querySelector('.gameBox');
var enemy = document.querySelectorAll('.enemy');
var hero = document.querySelector('.hero');
var action = document.querySelector('.action');
var j = 0;
for (j; j < enemy.length; j++) {
if (enemy[j].offsetTop + enemy[j].offsetHeight >= hero.offsetTop && enemy[j].offsetLeft <= hero.offsetLeft + hero.offsetWidth &&
enemy[j].offsetLeft + enemy[j].offsetWidth >= hero.offsetLeft) {
clearInterval(time1);
clearInterval(time2);
clearInterval(time3);
clearInterval(time4);
action.style.display = 'block';
clearInterval(time5);
clearInterval(time6);
gameBox.removeChild(hero);
}
}
}, 1);
}
//己方飞机移动
document.onkeydown = function(hero) {
var hero = document.querySelector('.hero');
if (event.keyCode == 65 && hero.offsetLeft >= 20) {
hero.style.left = hero.offsetLeft - 20 + 'px';
}
if (event.keyCode == 68 && hero.offsetLeft <= 430) {
hero.style.left = hero.offsetLeft + 20 + 'px';
}
}
//清除越界的盒子
function clearbox() {
var time5 = setInterval(function() {
var gameBox = document.querySelector('.gameBox');
var bullet = document.querySelectorAll('.bullet');
var i = 0;
for (i; i < bullet.length; i++) {
if (bullet[i].offsetTop < 0)
gameBox.removeChild(bullet[i]);
}
var enemy = document.querySelectorAll('.enemy');
i = 0;
for (i; i < enemy.length; i++) {
if (enemy[i].offsetTop > gameBox.offsetHeight - enemy[i].offsetHeight)
gameBox.removeChild(enemy[i]);
}
}, 1);
}
//开始游戏
var action = document.querySelector('.action');
action.onclick = function init(){
var gameBox = document.querySelector('.gameBox');
var enemy = document.querySelectorAll('.enemy');
var bullet = document.querySelectorAll('.bullet');
var hero = document.querySelector('.hero');
for(var i=0;i<enemy.length;i++)
gameBox.removeChild(enemy[i]);
for(var i=0;i<bullet.length;i++)
gameBox.removeChild(bullet[i]);
creathero();
creat();
clearbox();
action.style.display = 'none';
}
效果图:
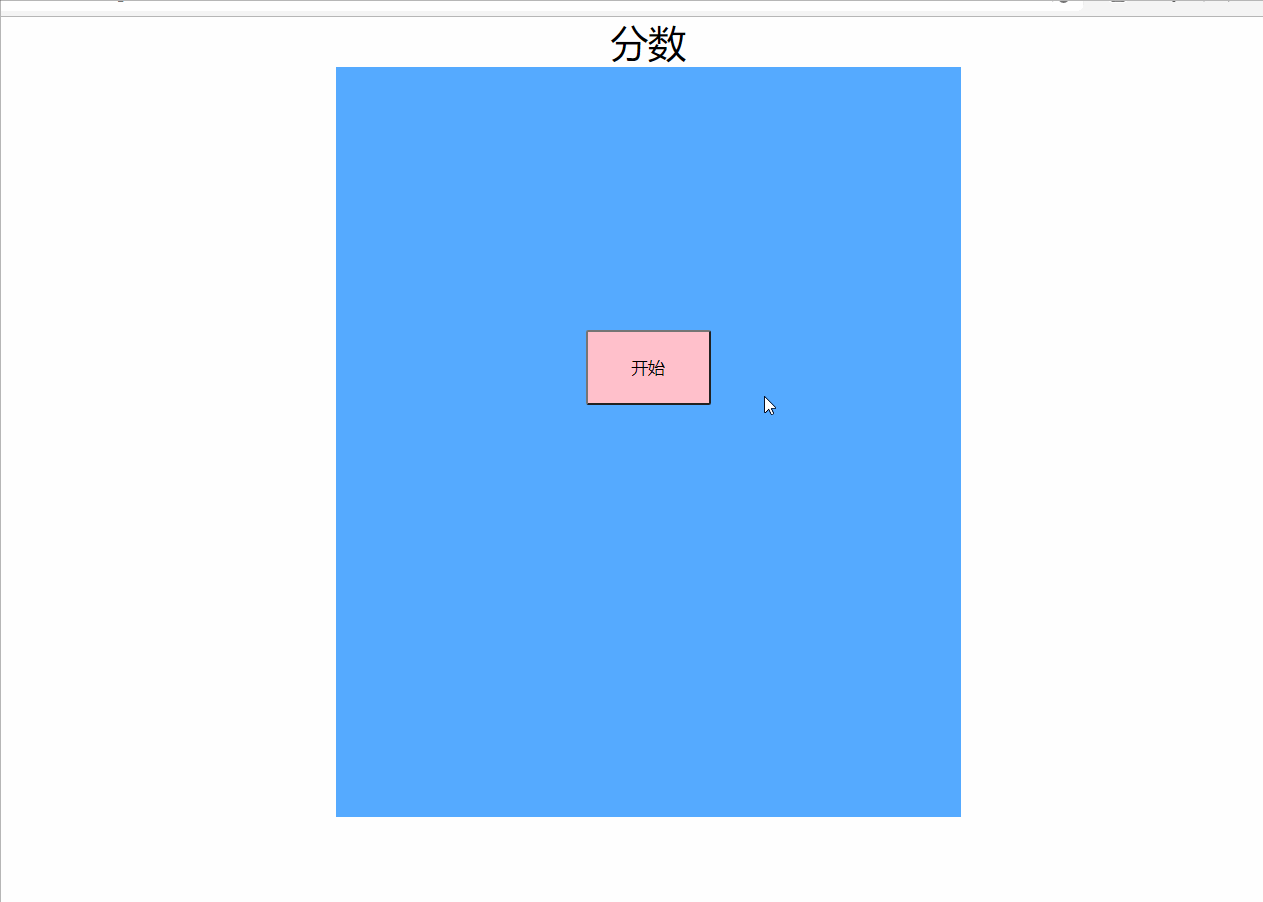
|