<style>
* {
margin: 0;
padding: 0;
}
#wrap {
width: 1200px;
margin: 100px auto;
display: flex;
justify-content: space-between;
align-items: center;
}
#tip,
#score {
height: 200px;
padding: 10px;
border: 1px solid #000;
border-radius: 20px;
}
#tip {
width: 200px;
order: -1;
text-indent: 2em;
display: flex;
flex-direction: column;
justify-content: space-evenly;
}
#score {
width: 180px;
text-align: center;
display: flex;
align-items: center;
justify-content: center;
}
#scoreboard {
color: red;
}
#box {
width: 600px;
height: 650px;
border: 1px solid #000;
background-image: radial-gradient(879px at 10.4% 22.3%, rgb(255, 235, 238) 0%, rgb(186, 190, 245) 93.6%);
position: relative;
}
#ball {
width: 20px;
height: 20px;
border-radius: 50%;
background-color: #000;
/* 加上定位,让小球运动 */
position: absolute;
box-shadow: 0px 0px 3px 3px #7ed6df;
top: 540px;
}
#slider {
width: 120px;
height: 20px;
background-color: #686de0;
border-radius: 10px;
position: absolute;
top: 570px;
cursor: pointer;
}
#btn {
width: 150px;
height: 90px;
position: absolute;
border-radius: 10px;
top: 400px;
left: 50%;
margin-left: -60px;
font-size: 24px;
}
#bricks div {
width: 98px;
height: 20px;
border: 1px solid rgb(255, 255, 255);
float: left;
}
</style>
</head>
<body>
<!-- 大盒子 -->
<div id="wrap">
<!-- 游戏区域 -->
<div id="box">
<!-- 砖块区 -->
<div id="bricks">
</div>
<!-- 小球 -->
<div id="ball">
</div>
<!-- 滑块 -->
<div id="slider">
</div>
<!-- 按钮 -->
<button id="btn">
开始游戏
</button>
</div>
<!-- 游戏说明 -->
<div id="tip">
<h1>游戏说明</h1>
<p>点击开始按钮开始游戏</p>
<p>使用鼠标拖拽滑块,反弹小球得分</p>
<p>当小球触底时,游戏结束</p>
<p>所有方块消失,游戏胜利</p>
</div>
<!-- 计分板 -->
<div id="score">
<h2>得分:<span id="scoreboard">0</span></h2>
</div>
</div>
<script>
/* 获取游戏区域 */
var box = document.getElementById("box")
/* 砖块区 */
var bricks = document.getElementById("bricks")
/* 小球 */
var ball = document.getElementById("ball")
/* 滑块 */
var slider = document.getElementById("slider")
/* 按钮 */
var btn = document.getElementById("btn")
/* 获取计分板 */
var scoreboard = document.getElementById('scoreboard')
/* 记录总分 */
var score = 0;
/* ----------------------------------- */
/* -------------------------------------- */
/* 为小球和砖块添加left的值 */
var randomLeft = getRandom(100, 500)
ball.style.left = randomLeft + 'px'
slider.style.left = randomLeft + ball.offsetWidth / 2 - slider.offsetWidth / 2 + 'px'
/* 随机得到小球的运动速度 */
var speedX = getRandom(3, 7)
var speedY = getRandom(-4, -8)
/* 定义一个定时器的变量为空 */
var timer = null;
/* ------------------------------------------- */
btn.onclick = function() {
/* 点击开始后把按钮隐藏 */
btn.style.display = "none"
/* 每次开始之前先清除定时器,确保只有一个定时器在工作。 */
clearInterval(timer)
/* 让小球运动 */
timer = setInterval(function() {
/* 计算出left位置 */
var arrivedX = ball.offsetLeft + speedX;
/* 计算出top位置 */
var arrivedY = ball.offsetTop + speedY;
/* 求小球运动的边界 */
var maxLeft = box.offsetWidth - ball.offsetWidth;
var maxTop = box.offsetHeight - ball.offsetHeight;
/* 判断小球是否出界 */
/* 左右边界 */
if (arrivedX <= 0) {
speedX *= -1;
arrivedX = 0
} else if (arrivedX > maxLeft) {
speedX *= -1
arrivedX = maxLeft;
}
/* 上下边界 */
if (arrivedY <= 0) {
speedY *= -1
arrivedY = 0;
} else if (arrivedY >= maxTop) {
/* 如果小球到底部,则游戏结束 */
alert('游戏结束');
/* 并清除定时器 */
clearInterval(timer);
/* 刷新页面 */
location.reload()
}
/* 设置小球的left和top */
ball.style.left = arrivedX + 'px'
ball.style.top = arrivedY + 'px'
/* 获取所有的砖块 */
var items = bricks.querySelectorAll('div')
/* 遍历所有div砖块 */
for (var i = 0; i < items.length; i++) {
/* 检测碰撞 小球和砖块 */
if (konck(ball, items[i])) {
/* 结果为true时,证明发生了碰撞。 */
// 执行 小球反弹
speedY *= -1;
// 删除砖块
items[i].remove();
// 计分板加1
score++;
/* 将数值加入到span标签中 */
scoreboard.innerHTML = score;
setTimeout(function() {
if (items.length == 1) {
alert('恭喜你通关')
/* 刷新页面 */
location.reload();
}
}, 0)
/* 结束 */
break;
}
}
/* 判断是否和滑块发生了碰撞 */
if (konck(ball, slider)) {
/* 碰撞就原路返回 */
speedY *= -1;
}
}, 16)
}
/* ------------------------------ */
/* 滑块拖拽部分 */
/* 鼠标按下 */
slider.onmousedown = function(ev) {
document.onmousemove = function(e) {
/* 获取偏移值 */
var x = e.pageX - box.offsetLeft - ev.offsetX
/* 获取最大值 */
var maxX = box.offsetWidth - slider.offsetWidth
/* 判断是否出界,出界则停留在原地 */
if (x <= 0) {
x = 0;
} else if (x >= maxX) {
x = maxX
}
slider.style.left = x + 'px'
}
}
/* 鼠标抬起 将事件解绑*/
document.onmouseup = function() {
document.onmousemove = null;
}
/* ------------------------------ */
createBrick(72)
/* 生成砖块 */
function createBrick(n) {
for (var k = 0; k < n; k++) {
//创建一个div节点
var node = document.createElement('div')
/* 为新节点创建颜色 */
node.style.backgroundColor = getColor()
/* 将新建的节点加入到砖块区 */
bricks.appendChild(node)
/* 获取砖块的left和top */
var l = node.offsetLeft;
var t = node.offsetTop
/* 把获取的加入到元素中 */
node.style.left = l + 'px'
node.style.top = t + 'px'
}
}
/* 所有砖块都获坐标后 才能绝对定位 */
var items = bricks.querySelectorAll('div')
/* 为每一个块添加定位 */
for (var j = 0; j < items.length; j++) {
items[j].style.position = "absolute"
}
/* ---------------------------------------------- */
/* 生成随机数 */
function getRandom(n, m) {
return Math.floor(Math.random() * (m - n + 1) + n)
}
/* 生成随机颜色 */
function getColor() {
var arr = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, "A", "B", "C", "D", "E", "F"];
color = "#"
for (i = 0; i < 6; i++) {
color += arr[getRandom(0, 15)]
}
return color
}
/* 碰撞函数 */
function konck(el1, el2) {
// 以下4中情况,只要满足一个,就说明没有碰撞
// 1.盒子1的左边框大于盒子2的右边框
// 2.盒子1的右边框小于盒子2的左边框
// 3.盒子1的上边框大于盒子2的下边框
// 3.盒子1的下边框小于盒子2的上边框
var l1 = el1.offsetLeft; //盒子1的左边框
var r1 = el1.offsetLeft + el1.offsetWidth; //盒子1的右边框
var t1 = el1.offsetTop; //盒子1的上边框
var b1 = el1.offsetTop + el1.offsetHeight; //盒子1的下边框
var l2 = el2.offsetLeft; //盒子2的左边框
var r2 = el2.offsetLeft + el2.offsetWidth; //盒子2的右边框
var t2 = el2.offsetTop; //盒子2的上边框
var b2 = el2.offsetTop + el2.offsetHeight; //盒子2的下边框
/* 判断 */
if (l1 > r2 || r1 < l2 || t1 > b2 || b1 < t2) {
return false;
} else {
return true;
}
}
</script>
</body>
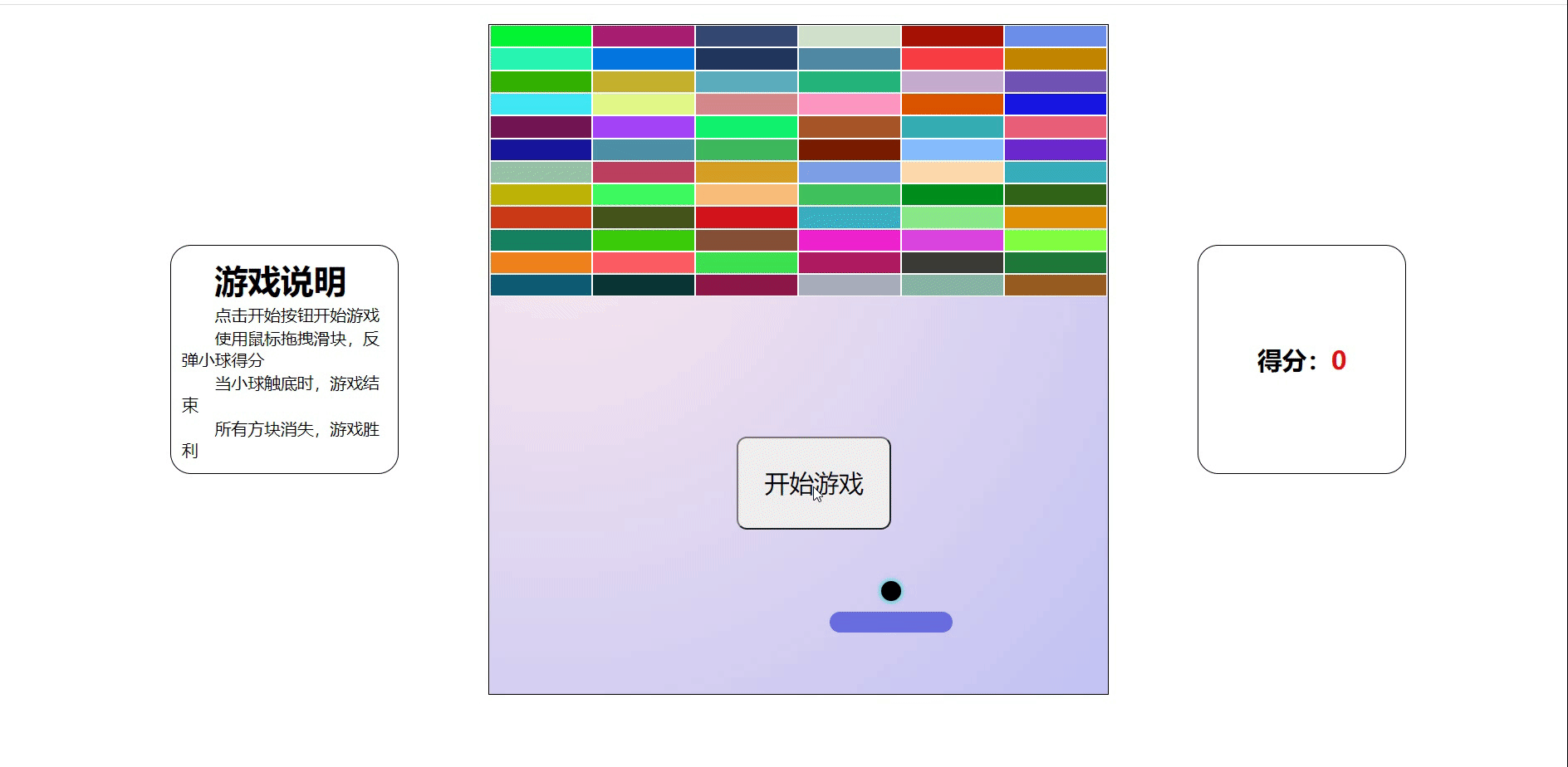
|