目录
一般的new关键字 使用案例:
构造函数、构造器、实例、原型对象:
new关键字到底做了什么?
实现一个假的new关键字
new关键字实现方法解读
一般的new关键字 使用案例:
function A(a,b,c){
this.a=a;
this.b=b;
this.c=c;
}
let a = new A(1,2,3)
console.log("---------------------------")
console.log(A) //方法本体
console.log(typeof A) //function
console.log(A.prototype)
console.log("---------------------------")
console.log(a) //实例本体
console.log(typeof a) //object
console.log(a.__proto__)
console.log("---------------------------")
console.log(A.prototype == a.__proto__)
console.log(A.prototype.constructor==A)
console.log(a.__proto__.constructor==A)
console.log(a.__proto__.constructor==A.prototype.constructor)
输出结果:
?
构造函数、构造器、实例、原型对象:
可以看到,a是一个以A为构造函数构造出来的实例,使得a的__proto__指向了A的prototype。
对于这个我们如何理解?
- 在JavaScript中,我们定义一个函数时,这个函数一定会自动存在一个属性prototype(原型),也就是我们看到的A.prototype。
- 函数的prototype又一定会自动存在一个属性constructor(构造器),也就是我们看到的A.prototype.constructor。
- A.prototype.constructor等于A。这实际上是一个环。
- 我们使用new关键字创建出实例a时,a一定会自动存在一个属性__proto__,也就是我们看到的a.__proto__。
- a.__proto__等于A.prototype。
- 我们一般称A为构造函数,a为实例,A.protoytpe==a.__proto__为原型对象。
- 为什么称A.protoytpe为原型对象?实际上,typeof A == "function",typeof a == "object",typeof?A.protoytpe == "object",它就是一个对象!
new关键字到底做了什么?
? ? 基于上述信息,我们可以去理解new关键字到底做了什么:
- 创造出一个空对象a;
- a.__proto__=A.prototype?
- 在A的this指针指向a的情况下,运行构造函数function?A。
- 返回实例a;
实现一个假的new关键字
我们将假的new关键字命名为New:
function A(a,b,c){
this.a=a;
this.b=b;
this.c=c;
}
function New(constructor,...params){
let obj = {
__proto__:constructor.prototype
};
constructor.call(obj,...params)
return obj
}
let a = New(A,1,2,3)
console.log("---------------------------")
console.log(A) //方法本体
console.log(typeof A) //function
console.log(A.prototype)
console.log("---------------------------")
console.log(a) //实例本体
console.log(typeof a) //object
console.log(a.__proto__)
console.log("---------------------------")
console.log(A.prototype == a.__proto__)
console.log(A.prototype.constructor==A)
console.log(a.__proto__.constructor==A)
console.log(a.__proto__.constructor==A.prototype.constructor)
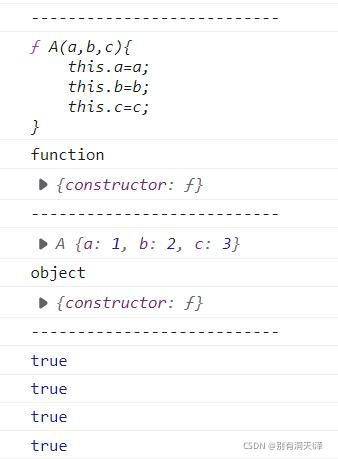
输出内容完全一致,功能实现成功!
new关键字实现方法解读
核心代码自然就是这一部分:
function New(constructor,...params){ ? ? let obj = { ? ? ? ? __proto__:constructor.prototype ? ? } ? ? constructor.call(obj,...params) ? ? return obj }
- 首先我们向New方法传入第一个参数constructor(构造函数),以及后续的构造函数所需参数。(使用了es6的新特性)
- 我们新建一个空对象obj,并且将obj的__proto__属性指向constructor.prototype。
- 使用call方法调用constructor函数,把this设置为obj,并传入参数数组。
- 返回obj。
|