写在前面 :
- 原生JS实现自动播放+手动播放
- 展示图片的div块无需粘来粘去,通过拼接字符串 将 数组 中的元素上传到页面
每种方式实现的具体效果都不同哦!都可运行!!!
一、原生JS实现
效果展示
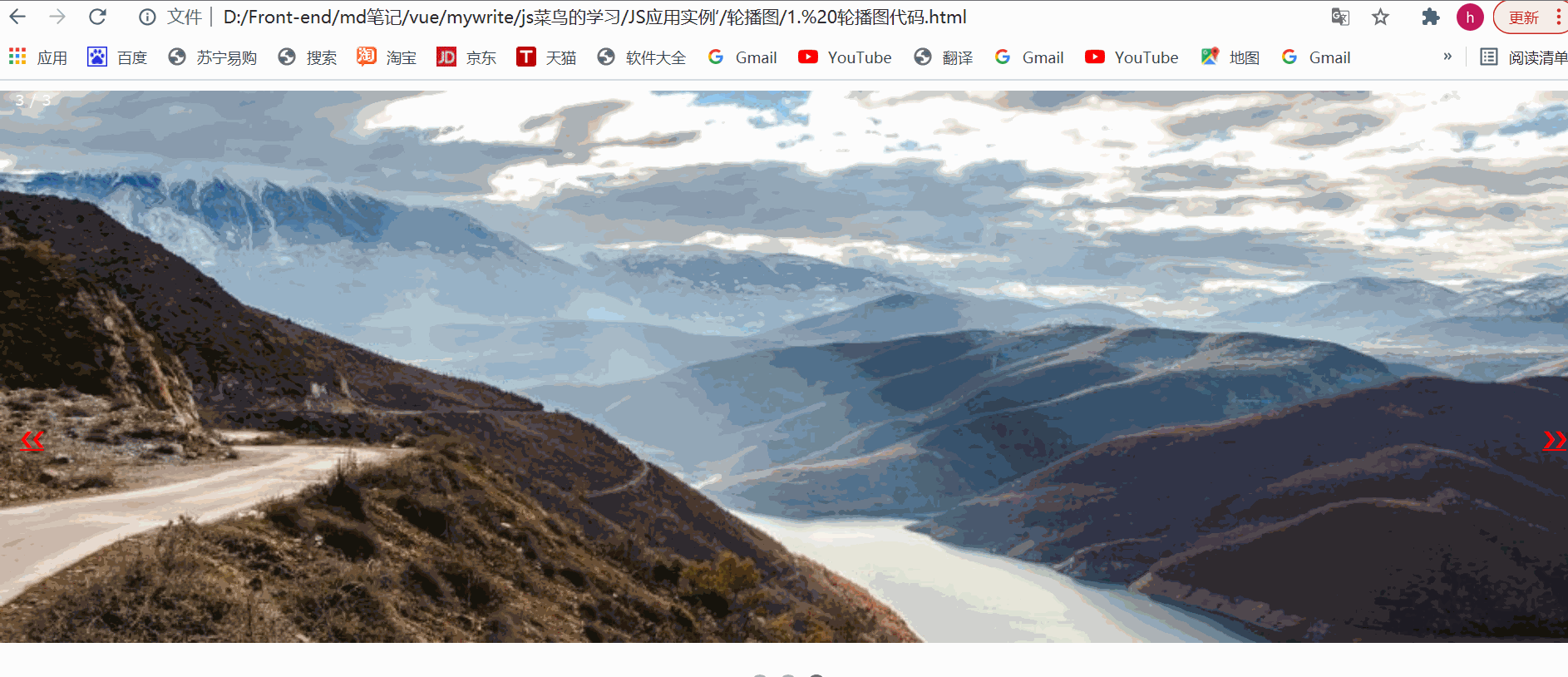
代码展示
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>javascript幻灯片代码</title>
<style>
* {
box-sizing: border-box;
}
body {
font-family: Verdana, sans-serif;
}
.mySlides {
display: none;
}
.slidedshow-container {
max-width: 1000px;
position: relative;
margin: auto;
}
.prev,
.next {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
margin-top: -22px;
padding: 16px;
color: red;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
border-radius: 0 3px 3px 0;
}
.next {
right: 0;
border-radius: 3px 0 0 3px;
}
.prev:hover,
.next:hover {
background-color: rgba(0, 0, 0, .8);
}
.text {
color: #f2f2f2;
font-size: 15px;
padding: 8px 12px;
position: absolute;
bottom: 8px;
width: 100%;
text-align: center;
}
.numbertext {
color: #f2f2f2;
font-size: 12px;
padding: 8px 12px;
position: absolute;
top: 0;
}
.dot {
cursor: pointer;
height: 13px;
width: 13px;
margin: 0 2px;
background-color: #bbb;
border-radius: 50%;
display: inline-block;
transition: background-color 0.6s ease;
}
.active,
.dot:hover {
background-color: #717171;
}
.fade {
-webkit-animation-name: fade;
-webkit-animation-duration: 1.5s;
animation-name: fade;
animation-duration: 1.5s;
}
@-webkit-keyframes fade {
from {
opacity: .4
}
to {
opacity: 1
}
}
@keyframes fade {
from {
opacity: .4
}
to {
opacity: 1
}
}
</style>
</head>
<body>
<div class="slideshow-container">
<div id="slideshow-container"></div>
<a href="javascript:;" class="prev" onclick="plusSlides(-1)">??</a>
<a href="javascript:;" class="next" onclick="plusSlides(1)">??</a>
</div>
<br>
<div style="text-align:center">
<span class="dot" onclick="currentSlide(1)"></span>
<span class="dot" onclick="currentSlide(2)"></span>
<span class="dot" onclick="currentSlide(3)"></span>
</div>
<script>
var mynumbertext = new Array("1 / 3", "2 / 3", "3 / 3");
var imgsrc = new Array("https://c.runoob.com/wp-content/uploads/2017/01/img_nature_wide.jpg", "https://c.runoob.com/wp-content/uploads/2017/01/img_fjords_wide.jpg", "https://c.runoob.com/wp-content/uploads/2017/01/img_mountains_wide.jpg");
var html = '';
for (var i = 0; i < mynumbertext.length; i++) {
html += "<div class=\"mySlides fade\">";
html += "<div class=\"numbertext\">";
html += mynumbertext[i];
html += "</div><img src=\"";
html += imgsrc[i];
html += "\" style=\"width:100%\"><div class=\"text\">文本";
html += i + 1;
html += "</div></div>";
}
document.getElementById("slideshow-container").innerHTML = html;
</script>
<script>
var slideIndex = 0;
showSlides();
function showSlides() {
var i;
var slides = document.getElementsByClassName("mySlides");
var dots = document.getElementsByClassName("dot");
for (i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
slideIndex++;
if (slideIndex > slides.length) {
slideIndex = 1;
}
for (i = 0; i < dots.length; i++) {
dots[i].className = dots[i].className.replace("active", "");
}
slides[slideIndex - 1].style.display = "block";
dots[slideIndex - 1].className += " active";
setTimeout(showSlides, 2000);
}
</script>
</body>
</html>
二、jQuery实现轮播图
上代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" name="viewport" content="width=device-width,initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no">
<title>JQuery轮播图</title>
<script src="https://cdn.bootcss.com/jquery/2.0.2/jquery.min.js" type="text/javascript"></script>
<style>
* {
padding: 0;
margin: 0;
}
.container {
width: 600px;
height: 400px;
overflow: hidden;
position: relative;
margin: 0 auto;
}
.list {
width: 3000px;
height: 400px;
position: absolute;
}
.list>img {
float: left;
width: 600px;
height: 400px;
}
.pointer {
position: absolute;
width: 100px;
bottom: 20px;
left: 250px;
}
.pointer>span {
cursor: pointer;
display: inline-block;
width: 10px;
height: 10px;
background: #7b7d80;
border-radius: 50%;
border: 1px solid #fff;
}
.pointer .on {
background: #28a4c9;
}
.arrow {
position: absolute;
text-decoration: none;
width: 40px;
height: 40px;
background: #727d8f;
color: #fff;
font-weight: bold;
line-height: 40px;
text-align: center;
top: 180px;
display: none;
}
.arrow:hover {
background: #0f0f0f;
}
.left {
left: 0;
}
.right {
right: 0;
}
.container:hover .arrow {
display: block;
}
</style>
</head>
<body>
<div class="container">
<div class="list" style="left:0px;">
<img src="https://c.runoob.com/wp-content/uploads/2017/01/img_nature_wide.jpg" alt="1" />
<img src="https://c.runoob.com/wp-content/uploads/2017/01/img_fjords_wide.jpg" alt="2" />
<img src="https://c.runoob.com/wp-content/uploads/2017/01/img_mountains_wide.jpg" alt="3" />
</div>
<div class="pointer">
<span index="1" class="on"></span>
<span index="2"></span>
<span index="3"></span>
</div>
<a href="#" rel="external nofollow" rel="external nofollow" class="arrow left"><</a>
<a href="#" rel="external nofollow" rel="external nofollow" class="arrow right">></a>
</div>
<script>
var imgCount = $("img").length;
var index = 1;
var intervalId;
var buttonSpan = $('.pointer')[0].children;
autoNextPage();
function autoNextPage() {
intervalId = setInterval(function() {
nextPage(true);
}, 3000);
}
$('.container').mouseover(function() {
console.log('hah');
clearInterval(intervalId);
});
$('.container').mouseout(function() {
autoNextPage();
});
$('.left').click(function() {
nextPage(false);
});
$('.right').click(function() {
nextPage(true);
});
clickButtons();
function clickButtons() {
var length = buttonSpan.length;
for (var i = 0; i < length; i++) {
buttonSpan[i].onclick = function() {
$(buttonSpan[index - 1]).removeClass('on');
if ($(this).attr('index') == 1) {
index = imgCount;
} else {
index = $(this).attr('index') - 1;
}
nextPage(true);
};
}
}
function nextPage(next) {
var targetLeft = 0;
$(buttonSpan[index - 1]).removeClass('on');
if (next) {
if (index == imgCount) {
targetLeft = 0;
index = 1;
} else {
index++;
targetLeft = -600 * (index - 1);
}
} else {
if (index == 1) {
index = 5;
targetLeft = -600 * (imgCount - 1);
} else {
index--;
targetLeft = -600 * (index - 1);
}
}
$('.list').animate({
left: targetLeft + 'px'
});
$(buttonSpan[index - 1]).addClass('on');
}
</script>
</body>
</html>
三、 VUE实现轮播图
上代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div class="center">
<div class="banner">
<a href=""><img v-for="(item,index) in dataList" :key="index" :src="item" v-show="n === index" alt="" @mouseenter="clearGo" @mouseleave="go"></a>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
var figure = new Vue({
el: '.banner ',
data: {
dataList: [
"https://c.runoob.com/wp-content/uploads/2017/01/img_mountains_wide.jpg",
"https://c.runoob.com/wp-content/uploads/2017/01/img_fjords_wide.jpg",
"https://c.runoob.com/wp-content/uploads/2017/01/img_nature_wide.jpg",
"https://c.runoob.com/wp-content/uploads/2017/01/img_mountains_wide.jpg"
],
n: 0,
interId: null
},
methods: {
go() {
this.interId = setInterval(() => {
this.n++;
if (this.n == this.dataList.length) {
this.n = 0;
}
}, 2000)
},
clearGo() {
clearInterval(this.interId)
},
clickPage(str) {
if (str === 'up ') {
if (this.n == 0) {
this.n = this.dataList.length;
}
this.n--;
} else {
this.n++;
if (this.n == this.dataList.length) {
this.n = 0;
}
}
}
},
mounted() {
this.go()
}
})
</script>
</body>
</html>
|