适配效果: 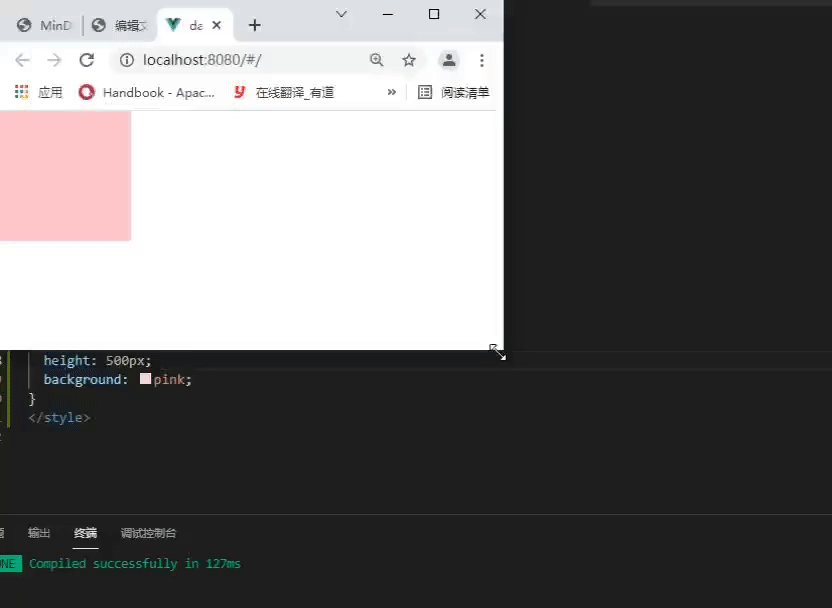
选择的适配方案
0. 新建 vue 项目,项目目录如下
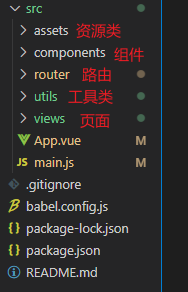
1. src / assets 文件下创建一个新的文件夹 css, css 文件夹下新建一个base.css文件用于定义全局样式
html,
body,
#app {
padding: 0;
margin: 0;
height: 100%;
min-width: 1024px;
}
body,
div,
span,
header,
footer,
nav,
section,
aside,
article,
ul,
ol,
dl,
dt,
dd,
li,
a,
p,
h1,
h2,
h3,
h4,
h5,
h6,
i,
b,
textarea,
button,
input,
select,
figure,
figcaption {
padding: 0;
margin: 0;
list-style: none;
font-style: normal;
text-decoration: none;
border: none;
font-weight: normal;
box-sizing: border-box;
-webkit-tap-highlight-color: transparent;
-webkit-font-smoothing: antialiased;
}
2. src 目录下新建一个 utils 文件夹,新建 flexible.js 文件,添加以下代码
(function(win, lib) {
var doc = win.document
var docEl = doc.documentElement
var metaEl = doc.querySelector('meta[name="viewport"]')
var flexibleEl = doc.querySelector('meta[name="flexible"]')
var dpr = 0
var scale = 0
var tid
var flexible = lib.flexible || (lib.flexible = {})
if (metaEl) {
var match = metaEl.getAttribute('content').match(/initial\-scale=([\d\.]+)/)
if (match) {
scale = parseFloat(match[1])
dpr = parseInt(1 / scale)
}
} else if (flexibleEl) {
var content = flexibleEl.getAttribute('content')
if (content) {
var initialDpr = content.match(/initial\-dpr=([\d\.]+)/)
var maximumDpr = content.match(/maximum\-dpr=([\d\.]+)/)
if (initialDpr) {
dpr = parseFloat(initialDpr[1])
scale = parseFloat((1 / dpr).toFixed(2))
}
if (maximumDpr) {
dpr = parseFloat(maximumDpr[1])
scale = parseFloat((1 / dpr).toFixed(2))
}
}
}
if (!dpr && !scale) {
var isAndroid = win.navigator.appVersion.match(/android/gi)
var isIPhone = win.navigator.appVersion.match(/iphone/gi)
var devicePixelRatio = win.devicePixelRatio
if (isIPhone) {
if (devicePixelRatio >= 3 && (!dpr || dpr >= 3)) {
dpr = 3
} else if (devicePixelRatio >= 2 && (!dpr || dpr >= 2)) {
dpr = 2
} else {
dpr = 1
}
} else {
dpr = 1
}
scale = 1 / dpr
}
docEl.setAttribute('data-dpr', dpr)
if (!metaEl) {
metaEl = doc.createElement('meta')
metaEl.setAttribute('name', 'viewport')
metaEl.setAttribute('content', 'initial-scale=' + scale + ', maximum-scale=' + scale + ', minimum-scale=' + scale + ', user-scalable=no')
if (docEl.firstElementChild) {
docEl.firstElementChild.appendChild(metaEl)
} else {
var wrap = doc.createElement('div')
wrap.appendChild(metaEl)
doc.write(wrap.innerHTML)
}
}
function refreshRem() {
var width = docEl.getBoundingClientRect().width
var rem = width / 10
docEl.style.fontSize = rem + 'px'
flexible.rem = win.rem = rem
}
win.addEventListener('resize', function() {
clearTimeout(tid)
tid = setTimeout(refreshRem, 300)
}, false)
win.addEventListener('pageshow', function(e) {
if (e.persisted) {
clearTimeout(tid)
tid = setTimeout(refreshRem, 300)
}
}, false)
if (doc.readyState === 'complete') {
doc.body.style.fontSize = 12 * dpr + 'px'
} else {
doc.addEventListener('DOMContentLoaded', function() {
doc.body.style.fontSize = 12 * dpr + 'px'
}, false)
}
refreshRem()
flexible.dpr = win.dpr = dpr
flexible.refreshRem = refreshRem
flexible.rem2px = function(d) {
var val = parseFloat(d) * this.rem
if (typeof d === 'string' && d.match(/rem$/)) {
val += 'px'
}
return val
}
flexible.px2rem = function(d) {
var val = parseFloat(d) / this.rem
if (typeof d === 'string' && d.match(/px$/)) {
val += 'rem'
}
return val
}
})(window, window.lib || (window.lib = {}))
3. 在 main.js 中引入该 flexible.js 文件 和 全局样式文件 base.css
import Vue from 'vue'
import App from './App.vue'
import router from './router'
import './utils/flexible'
import './assets/css/base.css'
Vue.config.productionTip = false
new Vue({
router,
render: h => h(App)
}).$mount('#app')
4. 安装等比适配插件,二选一即可
(4-1) postcss-px2rem 和 (4-2) cssrem 二选一即可
4-1(推荐). 安装 等比适配插件 postcss-px2rem 及 px2rem-loader
npm install postcss-px2rem px2rem-loader --save
在根目录下,新建一个 vue.config.js 文件,设置
const px2rem = require('postcss-px2rem')
const postcss = px2rem({
remUnit: 192
})
module.exports = {
lintOnSave: true,
css: {
loaderOptions: {
postcss: {
plugins: [
postcss
]
}
}
}
}
完成以上步骤后,整个项目目录如下
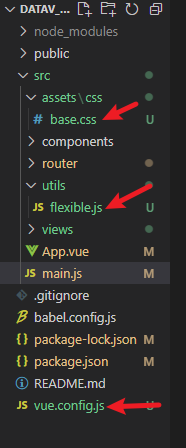
4-2.(不推荐) vscode 安装 cssrem 插件 (自动将px 换算为rem)
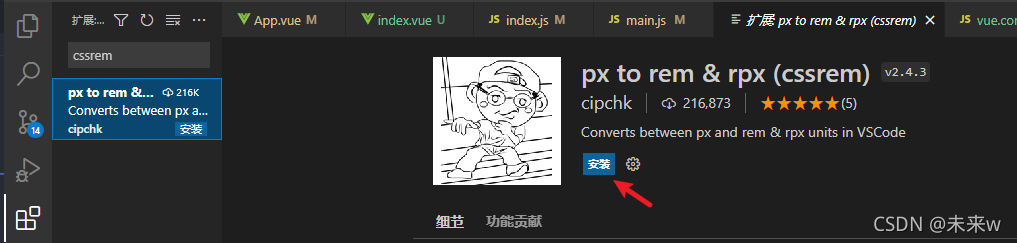
在扩展设置中,可以设置 cssrem 的基准值
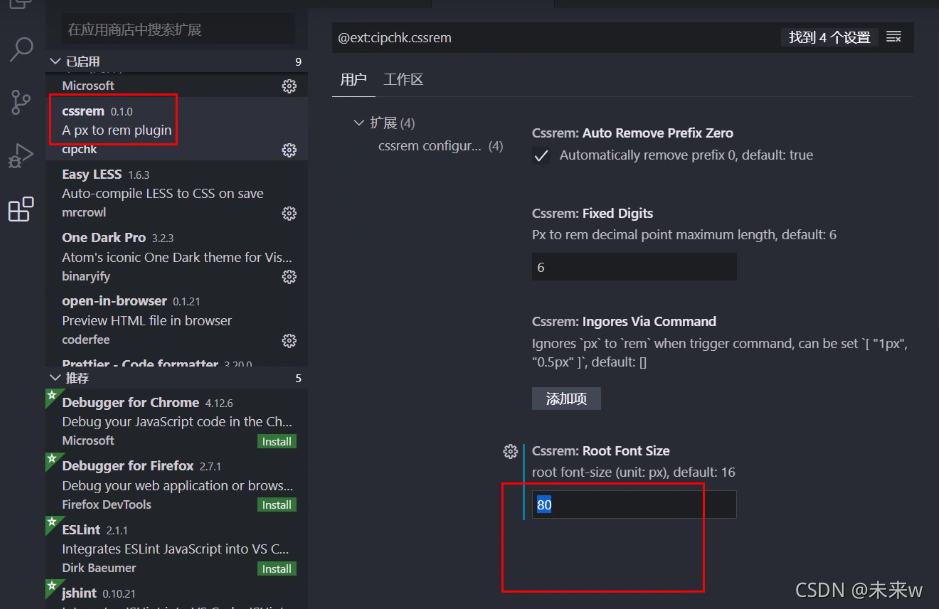
5. 测试是否适配成功
1. 安装 less less-loader
npm install less less-loader@6 --save
2. 测试 适配方案是否配置成功
在 src/views/index.vue 中添加一个div ,调整窗口大小,观察该div是否随窗口大小变化而变化
<template>
<div class="index">
index.js
<div class="box"></div>
</div>
</template>
<script>
export default {
name: 'index'
}
</script>
<style lang="less" scoped>
.box{
width: 150px;
height: 150px;
background: pink;
}
</style>
3. 运行后在浏览器查看,拉动浏览器,观察盒子是否随浏览器大小变化
如下所示,则适配成功。 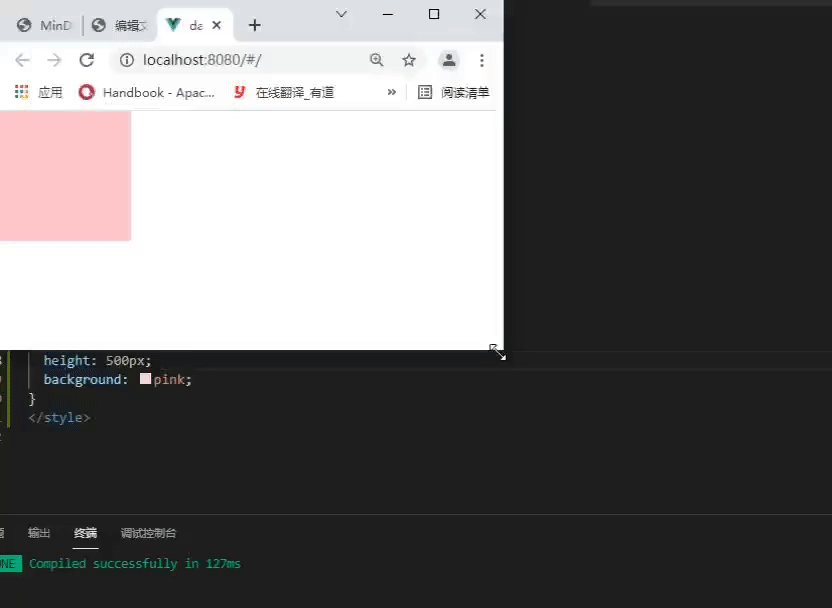
|