前言:
之前我们说用redux可以方便的管理我们的数据,但是发布订阅的模式还是会有所不便,你还是需要在你们UI组件中编写订阅的函数。 React-Redux是Redux的官方React绑定库,它更加的简化了我们的redux内部操作,不需要我们再手动绑定订阅操作。
一、React-Redux 和 Redux在使用方式上的区别
(参考上一章例子大概写的,有不明白的地方还请往下看后面的完整代码。)
1.获取数据的对比
redux的获取数据,要使用getState( )并且还需要subscribe( )监听才能获取,后续更新也需要绑定事件然后setState()更新
const state = {
const state=store.getState()
}
updateState = ()=>{
this.setState(store.getState())
}
react-redux的获取数据只需要绑定UI组件上的state和store内部的state即可
const mapStateToProps = (state) => ({
state: state.xxxReducer
})
2.dispatch派发action对比
redux通过函数事件用dispatch派发action
increment = ()=>{
store.dispatch(incrementAction())
}
react-redux的dispatch派发action同理,也是直接绑定
const mapDispatchToProps = (dispatch) => ({
increment: ()=>dispatch(incrementAction())
})
const mapDispatchToProps = {
increment: ()=>incrementAction()
}
3.监听state的对比
redux通过subscribe函数监听store内的数据,之后才能有获取数据,不监听就啥也拿不到,还是比较麻烦的
store.subscribe(this.asyncState)
react-redux则是通过connect函数,把 state 和 dispatch 以及 ui 组件连接起来,不需要写订阅函数,十分方便
const Container = connect(mapStateToProps,mapDispatchToProps)(Counter)
function connect(mapStateToProps,mapDispatchToProps){
return (Component)=>{
return class Container extends React.Component{
constructor(props){
this.store = props.store
}
{
store => getState => state
=> dispatch
mapStateToProps
mapDispatchToProps
}
render(){
return (
<Component {...mapStateToProps} {...mapDispatchToProps}></Component>
)
}
}
}
}
4.react-redux的完整绑定数据流程
import React from 'react'
import store from './store'
import {incrementAction,decrementAction} from './store/action_creator'
import {connect, Provider} from 'react-redux';
function Counter(props){
const {value,increment,decrement} = props
return (
<div>
Clicked : { value } times
{' '}
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
)
}
const mapStateToProps = state => ({
value: state.count
})
const mapDispatchToProps = dispatch => ({
increment: ()=>dispatch(incrementAction()),
decrement: ()=>dispatch(decrementAction())
})
const Container = connect(mapStateToProps,mapDispatchToProps)(Counter)
class App extends React.Component {
render() {
return (
<div>
<Provider store={store}>
<Container />
</Provider>
</div>
)
}
}
二、React-Redux 的工作原理
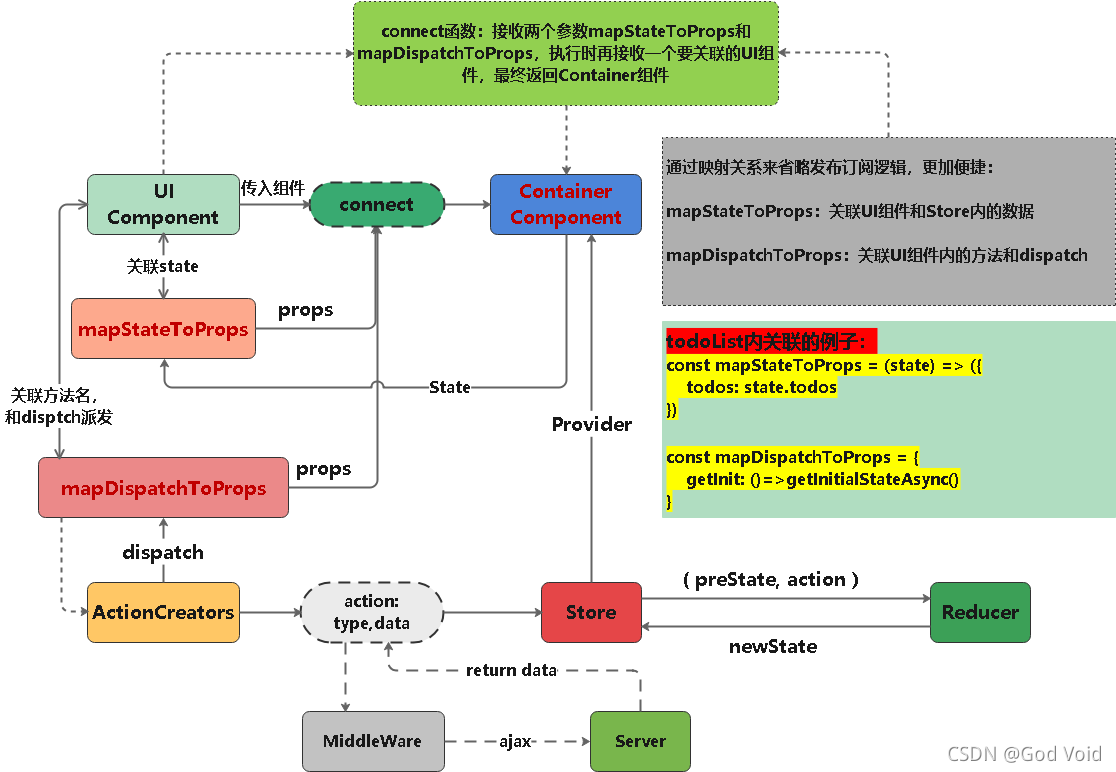 React-Redux把所有的组件都拆分成了两大类:UI组件 和 容器组件
1.UI组件:
只负责UI的呈现,不带有任何业务逻辑
没有状态(即不适用this.state,或定义 state)
所有数据都由 props 提供
不使用任何 Redux 的API
2.容器组件
负责管理数据和业务逻辑,不负责UI的呈现
带有内部状态
使用 Redux 的API
3.如何定义容器组件?
通过connect方法自动生成容器组件 对 UI组件的增强
connect接收两个参数: (1)mapStateToProps:负责输入逻辑,即 把 state 映射到 UI组件的参数(props) (2)mapDispatchToProps:负责输出逻辑,即 把用户对UI组件的操作 映射成 action
const Container = connect(mapStateToProps,mapDispatchToProps)(Counter)
4.当我们有多个reducer时,如何映射 state?
当我们使用combineReducers集成了多个reducer时
const reducer = combineReducers({
reducerCounter,
reducerStudentForm,
reducerTask
})
这时,我们只需要计数器Counter的reducer,我们需要怎么映射:
const mapStateToProps = (state) => ({
value: state.reducerCounter.count
})
const mapStateToProps = {reducerCounter} => ({
value: reducerCounter.count
})
三、总结
react-redux 和 redux 的差别其实并不大,除了在数据绑定方面 react-redux 十分便捷外,剩下的内部的reducer,action_creator那些其实都是一样的,react-redux只是方便了我们在数据的绑定上,而我们还是需要理解好 redux 的 action 派发流程,这样才能学的更好。
|