先上演示效果: 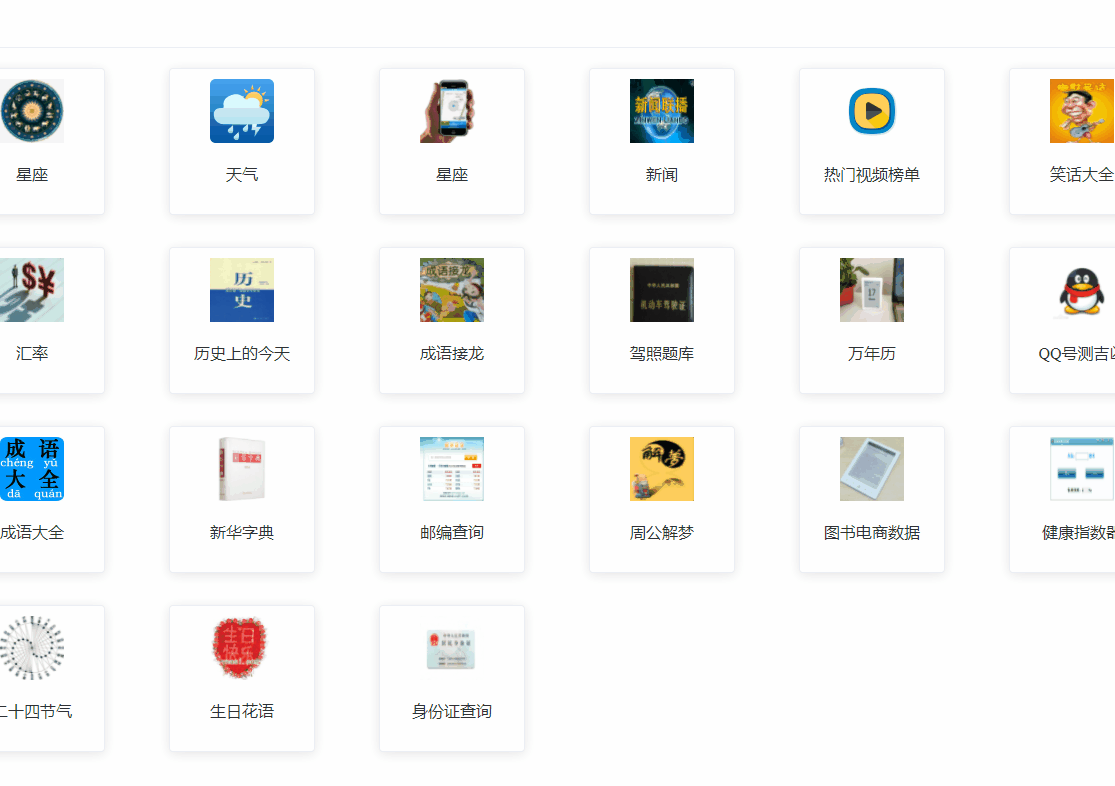
1: VUE 文件
<template>
<div class="videos-ele" vv-show="normal">
<el-card class="items">
<el-row>
<el-col :span="4" v-for="(v, index) in v_data" :key="index">
<el-card class="item" @click.native="newWindow(v.share_url)" :title="v.title">
<img class="item_cover" :src="v.item_cover">
<div>
<div class="item_title">{{ v.title }}</div>
<div class="item_bottom"><span class="play-count">{{ v.play_count }}</span> 次播放</div>
</div>
</el-card>
</el-col>
</el-row>
</el-card>
<el-card v-show="errEle">
<h2>something error</h2>
</el-card>
</div>
</template>
<script>
export default {
name: 'videos',
mounted: function () {
this.getData()
},
data () {
return {
normal: true,
errEle: false,
pagesize: 50,
apiUrl: '/api_5',
v_data: [
]
}
},
methods: {
getData: function (nmb) {
nmb = arguments[0] ? arguments[0] : this.pagesize
this.$axios({
url: this.apiUrl,
params: {
pagesize: nmb
}
}).then(res => {
if (res.data.error_code === 0) {
this.v_data = res.data.result
}
}).catch(err => {
this.normal = false
this.errEle = true
console.log(err)
})
},
newWindow: function (url) {
window.open(url, '_blank')
}
}
}
</script>
<style scoped>
.videos-ele {
margin-left: 15%;
}
.item {
margin: 0 3rem 2rem 0;
text-align: center;
cursor: pointer;
}
.item .item_cover{
width: 10rem;
}
.item .item_title{
line-height: 1.3rem;
height: 3rem;
overflow: hidden;
text-overflow: ellipsis;
}
.item .play-count {
color: red;
}
</style>
2: Vue 路由配置: config/index.js
proxyTable: {
'/api_1': {
target:'http://www.chaxun.com/joke',
changeOrigin:true,
pathRewrite:{
'^/api_1': '/api_1'
}
},
'/api_2': {
target: 'http://www.chaxun.com/constellation',
changeOrigin: true,
pathRewrite: {
'^/api_2': '/api_2'
}
},
'/api_3': {
target: 'http://www.chaxun.com/weather',
changeOrigin: true,
pathRewrite: {
'^/api_3': '/api_3'
}
},
'/api_4': {
target: 'http://www.chaxun.com/news',
changeOrigin: true,
pathRewrite: {
'^/api_4': '/api_4'
}
},
'/api_5': {
target: 'http://www.chaxun.com/videos',
changeOrigin: true,
pathRewrite: {
'^/api_5': '/api_5'
}
}
},
3: TP5 页面:
<?php
namespace app\index\controller;
use app\index\controller\HttpRequest;
use think\Route;
use think\Request;
class Api5 {
const CONST_KEY = "放入自己聚合申请的API即可";
public function test()
{
return "This videos control is working.";
}
public function index()
{
$rsq = request() -> param();
if (isset($rsq['pagesize'])) {
$url = "http://apis.juhe.cn/fapig/douyin/billboard";
$pagesize = $rsq['pagesize'];
$param = [
"type" => "hot_video",
"size" => $pagesize
];
$paramString = http_build_query($param);
$httpRsq = new HttpRequest;
$data = $httpRsq -> httpRequest($url, $paramString."&key=".self::CONST_KEY);
return $data;
} else {
$errData = file_get_contents('./err.json', true);
$err = json_decode($errData);
return json($err);
}
}
}
4: TP5 封装请求:
<?php
namespace app\index\controller;
class HttpRequest {
public function index () {
return "constellation api";
}
public function httpRequest($url, $params = false, $isPost = 0){
$httpInfo = [];
$ch = curl_init();
curl_setopt($ch, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1);
curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/95.0.4638.54 Safari/537.36');
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 3);
curl_setopt($ch, CURLOPT_TIMEOUT, 12);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
if ($isPost) {
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $params);
curl_setopt($ch, CURLOPT_URL, $url);
} else {
if ($params) {
curl_setopt($ch, CURLOPT_URL, $url . '?' . $params);
} else {
curl_setopt($ch, CURLOPT_URL, $url);
}
}
$reponse = curl_exec($ch);
if ($reponse === FALSE) {
return false;
}
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
$httpInfo = array_merge($httpInfo, curl_getinfo($ch));
curl_close($ch);
return $reponse;
}
}
?>
TP5后端,VUE前端请求聚合数据新闻接口
TP5后端,VUE前端请求聚合数据笑话大全接口
TP5后端,VUE前端请求聚合数据天气接口
|