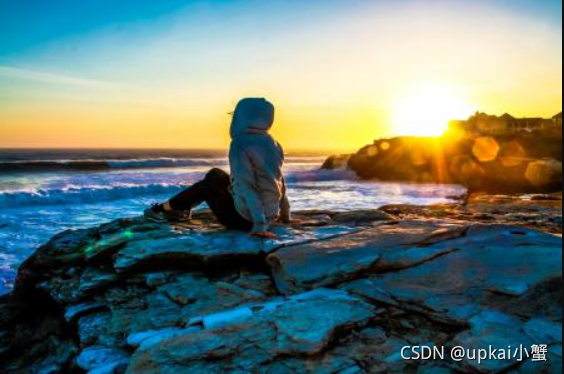
?1、什么是AJAX请求?
- AJAX是一种创建交互式网页应用的网页开发技术
- AJAX是一种浏览器通过JS异步发起请求,局部更新页面的技术
- AJAX请求的局部更新,浏览器地址栏不会发生变化
- 局部更新不会舍弃原来页面的内容
2、原生AJAX请求的示例
BaseServlet
package com.example.json_ajax_i18n.Servlet;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.lang.reflect.Method;
public abstract class BaseServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doPost(req, resp);
}
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// 解决post请求中文乱码问题
// 一定要在获取请求参数之前调用才有效
req.setCharacterEncoding("UTF-8");
// 解决响应中文乱码问题
resp.setContentType("text/html; charset=UTF-8");
String action = req.getParameter("action");
try {
// 获取action业务鉴别字符串,获取相应的业务 方法反射对象
Method method = this.getClass().getDeclaredMethod(action, HttpServletRequest.class, HttpServletResponse.class);
// System.out.println(method);
// 调用目标业务 方法
method.invoke(this, req, resp);
} catch (Exception e) {
e.printStackTrace();
}
}
}
AjaxServlet
package com.example.json_ajax_i18n.Servlet;
import com.example.json_ajax_i18n.pojo.Person;
import com.google.gson.Gson;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class AjaxServlet extends BaseServlet{
protected void JavaScriptAjax(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("Ajax请求发送过来了");
Person person = new Person(1,"Ajax yyds");
//json格式的字符串
Gson gson = new Gson();
String personJsonString = gson.toJson(person);
resp.getWriter().write(personJsonString);
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<servlet-name>AjaxServlet</servlet-name>
<servlet-class>com.example.json_ajax_i18n.Servlet.AjaxServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>AjaxServlet</servlet-name>
<url-pattern>/ajaxServlet</url-pattern>
</servlet-mapping>
</web-app>
ajax.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="pragma" content="no-cache" />
<meta http-equiv="cache-control" content="no-cache" />
<meta http-equiv="Expires" content="0" />
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
//在这里使用javaScript语言发起Ajax请求,访问服务器AjaxServlet中的javaScriptAjax
function ajaxRequest() {
// 1、我们首先要创建XMLHttpRequest
var xmlhttprequest = new XMLHttpRequest();
// 2、调用open方法设置请求参数
xmlhttprequest.open("GET","http://localhost:8080/Json_Ajax_i18n/ajaxServlet?action=JavaScriptAjax",true);
// 4、在send方法前绑定onreadystatechange事件,处理请求完成后的操作。
xmlhttprequest.onreadystatechange = function () {
if (xmlhttprequest.readyState == 4 && xmlhttprequest.status == 200) {
var jsonObj = JSON.parse(xmlhttprequest.responseText);
document.querySelector("#div01").innerHTML = "用户编号:"+jsonObj.id+"<br/>用户名:"+jsonObj.name;
}
}
// 3、调用send方法发送请求
xmlhttprequest.send();
}
</script>
</head>
<body>
<button onclick="ajaxRequest()">ajax request</button>
<div id="div01">
</div>
</body>
</html>
结果
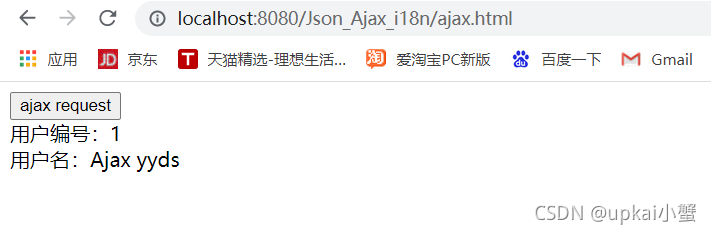
?3、jQuery 中的AJAX请求
$.ajax 方法
- url? ? ? ? 表示请求的地址
- type? ? ? ? 表示请求的类型get或post请求
- data? ? ? ? 表示发送给服务器的数据
格式有两种: 一:name=value&name=value 二:{key:value} - success? ? ? ? 表示请求成功后的回调函数
- dataType? ? ? ? 表示服务器返回的数据类型
常用的数据类型有:text(表示纯文本)、xml(表示xml数据)、json(表示json对象)
// ajax请求
$("#ajaxBtn").click(function(){
$.ajax({
url:"http://localhost:8080/Json_Ajax_i18n/ajaxServlet",
// data:"action=jQueryAjax",也可以如下写
data:{action:"jQueryAjax"},
type:"GET",
success:function (data){
// data服务器返回的数据
alert("服务器返回的数据是:"+data);
var data2 = JSON.parse(data);
$("#msg").html("编号:"+data2.id+"<br/>姓名:"+data2.name);
},
dataType:"text"//如果数据类型是json就不用自己转换为json对象了
});
});
protected void jQueryAjax(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("jQueryAjax请求发送过来了");
Person person = new Person(1,"Ajax yyds");
//json格式的字符串
Gson gson = new Gson();
String personJsonString = gson.toJson(person);
resp.getWriter().write(personJsonString);
}
$.get 方法和 $.post 方法
- url? ? ? ? 请求的url地址
- data? ? ? ? 发送的数据
- callback? ? ? ? 成功的回调函数
- type? ? ? ? 返回的数据类型
// ajax--get请求
$("#getBtn").click(function(){
$.get("http://localhost:8080/Json_Ajax_i18n/ajaxServlet","action=jQueryGet",function (data) {
$("#msg").html("编号:"+data.id+"<br/>姓名:"+data.name);
},"json")
});
// ajax--post请求
$("#postBtn").click(function(){
// post请求
$.post("http://localhost:8080/Json_Ajax_i18n/ajaxServlet","action=jQueryPost",function (data) {
$("#msg").html("编号:"+data.id+"<br/>姓名:"+data.name);
},"json")
});
protected void jQueryGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("jQueryGet请求发送过来了");
Person person = new Person(1,"Ajax yyds");
//json格式的字符串
Gson gson = new Gson();
String personJsonString = gson.toJson(person);
resp.getWriter().write(personJsonString);
}
protected void jQueryPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("jQueryPost请求发送过来了");
Person person = new Person(1,"Ajax yyds");
//json格式的字符串
Gson gson = new Gson();
String personJsonString = gson.toJson(person);
resp.getWriter().write(personJsonString);
}
$.getJSON 方法
- url? ? ? ? 请求的url地址
- data? ? ? ? 发送的数据
- callback? ? ? ? 成功的回调函数
?
// ajax--getJson请求
$("#getJSONBtn").click(function(){
// 调用
$.getJSON("http://localhost:8080/Json_Ajax_i18n/ajaxServlet","action=jQueryGetJSON",function (data) {
$("#msg").html("编号:"+data.id+"<br/>姓名:"+data.name);
})
});
protected void jQueryGetJSON(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("jQueryGetJSON请求发送过来了");
Person person = new Person(1,"Ajax yyds");
//json格式的字符串
Gson gson = new Gson();
String personJsonString = gson.toJson(person);
resp.getWriter().write(personJsonString);
}
表单序列化 serialize()
serialize()可以表单中所有的表单项的内容都获取到,并以name=value&name=value的形式拼接,这样服务器那边就可以接收到表单数据了通过getParameter方法。
// ajax请求
$("#submit").click(function(){
// 把参数序列化
alert($("#form01").serialize());
$.getJSON("http://localhost:8080/Json_Ajax_i18n/ajaxServlet","action=jQuerySerialize&"+$("#form01").serialize(),function (data) {
$("#msg").html("编号:"+data.id+"<br/>姓名:"+data.name);
})
});
protected void jQuerySerialize(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("jQuerySerialize请求发送过来了");
//接收发送过来的表单数据
System.out.println("用户名:"+req.getParameter("username"));
System.out.println("密 码:"+req.getParameter("password"));
Person person = new Person(1,"Ajax yyds");
//json格式的字符串
Gson gson = new Gson();
String personJsonString = gson.toJson(person);
resp.getWriter().write(personJsonString);
}
|