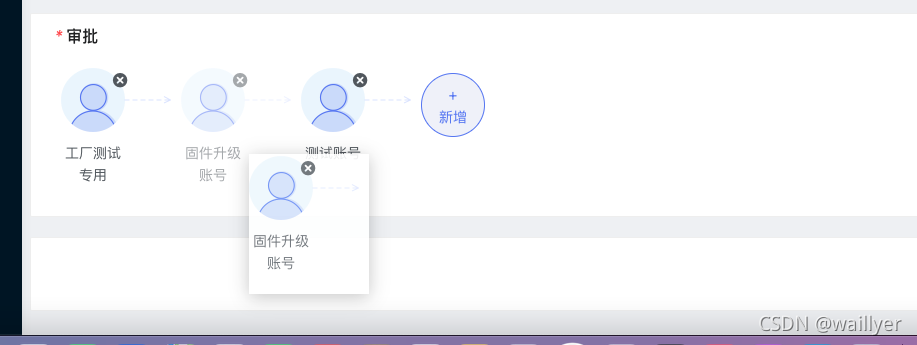
import React, { useEffect, useRef, useState } from 'react';
import { DndProvider } from 'react-dnd';
import { Form, Card } from 'antd';
import { useSelector } from 'umi';
import { cloneDeep } from 'lodash';
import HTML5Backend from 'react-dnd-html5-backend';
import styles from './index.less';
import ApprovePersonModel from './ApprovePersonModel';
import DragDropBox from './DragDropBox';
export const INPUT_LENGTH_LIMIT = 50;
export const TEXT_AREA_LENGTH_LIMIT = 500;
export default ({ currentModel, baseInfo, dispatch, type, change_reason }) => {
const { adminList = [] } = useSelector(state => state[currentModel]);
const [form] = Form.useForm();
const [isModal, setIsModal] = useState(false);
const { setFieldsValue } = form;
const formRef = useRef(null);
useEffect(() => {
formRef.current = form;
}, [form]);
useEffect(() => {
setFieldsValue({
change_reason,
});
}, [change_reason, setFieldsValue]);
useEffect(() => {
dispatch({
type: `${currentModel}/overrideStateProps`,
payload: {
otherForm: formRef,
},
});
}, [dispatch, formRef, currentModel]);
const approvePersonModelprops = {
title: '新增审批人',
currentModel,
modalVisible: isModal,
dispatch,
dataList: adminList,
limit: 5,
handleOk: res => {
dispatch({
type: `${currentModel}/overrideStateProps`,
payload: {
adminList: res?.adminList,
},
});
setIsModal(false);
},
handleCancel: () => {
setIsModal(false);
},
afterClose: () => {
setIsModal(false);
},
};
const changePosition = (dragIndex, hoverIndex) => {
const data = cloneDeep(adminList);
const temp = data[dragIndex];
data[dragIndex] = data[hoverIndex];
data[hoverIndex] = temp;
dispatch({
type: `${currentModel}/overrideStateProps`,
payload: {
adminList: data,
},
});
};
return (
<>
<Card
title={
<span className={styles.title}>
<i>*</i>审批
</span>
}
className={styles.card}
bordered
style={{ marginTop: 20 }}
>
<div className={styles.reviewerContainer}>
{adminList?.length && 0
? adminList.map((ele, index) => {
return (
<span key={ele?.id} className={styles.reviewer}>
<div className={styles.reviewerImg}>
<span
className="saas saas-failure1"
onClick={() => {
const listFilter = adminList.filter(
(_, itemIndex) => itemIndex !== index,
);
dispatch({
type: `${currentModel}/overrideStateProps`,
payload: {
adminList: listFilter,
},
});
}}
/>
</div>
<div className={styles.reviewerTxt}>{ele.name}</div>
</span>
);
})
: null}
<DndProvider backend={HTML5Backend}>
{adminList?.length ? (
<div style={{ display: 'flex' }}>
{adminList.map((item, index) => {
return (
<DragDropBox
key={item?.id}
index={index}
id={item?.id}
text={item?.text}
itemInfo={item}
changePosition={changePosition}
handleClick={val => {
const listFilter = adminList.filter(
(_, itemIndex) => itemIndex !== val,
);
dispatch({
type: `${currentModel}/overrideStateProps`,
payload: {
adminList: listFilter,
},
});
}}
/>
);
})}
</div>
) : null}
</DndProvider>
{adminList?.length < 5 ? (
<span
className={styles.createModal}
onClick={() => {
setIsModal(true);
}}
>
+ <br />
新增
</span>
) : null}
</div>
</Card>
<ApprovePersonModel {...approvePersonModelprops} />
</>
);
};
import React, { useRef } from 'react';
import { useDrop, useDrag } from 'react-dnd';
import styles from './index.less';
export default ({
id = '',
text = '',
index = '',
changePosition = () => {},
handleClick = () => {},
className = {},
itemInfo = {},
}) => {
const ref = useRef(null);
const [, drop] = useDrop({
accept: 'DragDropBox',
hover: (item, monitor) => {
if (!ref.current) return;
const dragIndex = item.index;
const hoverIndex = index;
if (dragIndex === hoverIndex) return;
changePosition(dragIndex, hoverIndex);
item.index = hoverIndex;
},
});
const [{ isDragging }, drag] = useDrag({
item: {
type: 'DragDropBox',
id,
index,
text,
},
collect: monitor => ({
isDragging: monitor.isDragging(),
}),
});
return (
<div ref={drag(drop(ref))} style={{ opacity: isDragging ? 0.5 : 1 }} className={className.dragBox}>
<span key={itemInfo?.id} className={styles.reviewer}>
<div className={styles.reviewerImg}>
<span className="saas saas-failure1" onClick={() => handleClick(index)} />
</div>
<div className={styles.reviewerTxt}>{itemInfo?.name}</div>
</span>
</div>
);
};
|