使用jquery实现局部刷新
后端使用springMVC模式进行数据处理
前端测试代码:
<%@ page contentType="text/html; UTF-8" pageEncoding="UTF-8" %>
<html>
<body>
内容:
<p id="pp" onmouseover="change()" onmousedown="change2()">
click
</p>
<p id="ppp"></p>
</body>
<script src="/js/jquery-3.6.0.min.js"></script>
<script>
function change(){
document.getElementById("pp").style.color = "red";
}
function change2(){
document.getElementById("pp").style.color = "black";
$.ajax({
url:"/Test/test",
dataType:"text",
data:{
data:"this is a data"
},
success:function (data){
$("#ppp").text(data);
},
error:function (){
$("#ppp").text("error");
}
})
}
</script>
</html>
后端的处理代码:
@RequestMapping("/Test/")
@Controller
public class Test {
@RequestMapping("test")
@ResponseBody
public void test(@Parameters String data,HttpServletResponse response) throws IOException {
System.out.println("i got it");
System.out.println(data);
data = "this is a new data";
response.getWriter().write(data);
}
}
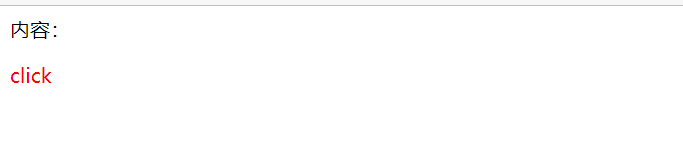 点击click后
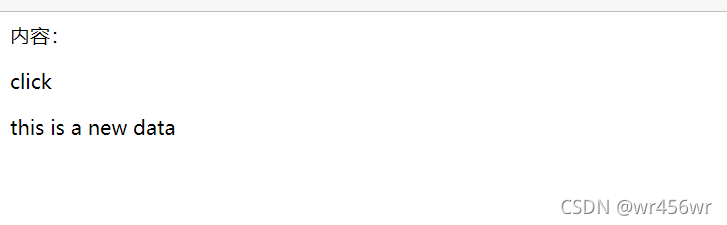
后端使用Servlet进行数据处理
前端的代码:
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP - Hello World</title>
</head>
<body>
<h1><%= "Hello World!"%>
</h1>
<br/>
<p id="pp" onmouseover="change()" onmousedown="fun()">click me</p>
<p id="ppp"></p>
</body>
<script src="jquery-3.6.0.min.js"></script>
<script>
function change(){
document.getElementById('pp').style.color = "red";
}
function fun(){
document.getElementById('pp').style.color = "black";
console.log("i will begin");
$.ajax({
url:"/Servlet2",
dataType:"text",
data:{
data:"this is a data"
},
success:function (data){
console.log(data);
$("#ppp").text(data);
},
error:function (){
$("#ppp").text("error");
}
})
console.log("i am over");
}
</script>
</html>
后端的java代码:
import javax.servlet.*;
import javax.servlet.http.*;
import javax.servlet.annotation.*;
import java.io.IOException;
@WebServlet(name = "Servlet2", value = "/Servlet2")
public class Servlet2 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String data = request.getParameter("data");
System.out.println(data);
data = "this is new data";
response.getWriter().write(data);
}
}
关于更具体的jquery的ajax方法和参数
关于更具体的jquery的ajax方法和参数可以参考:(w3school的教程)
https://www.w3school.com.cn/jquery/ajax_ajax.asp
关于跨域访问
跨域是指跨域名的访问,以下情况都属于跨域:
跨域原因说明 示例
域名不同 www.jd.com 与 www.taobao.com
域名相同,端口不同 www.jd.com:8080 与 www.jd.com:8081
二级域名不同 item.jd.com 与 miaosha.jd.com
如果域名和端口都相同,但是请求路径不同,不属于跨域,如:
www.jd.com/item
www.jd.com/goods
资料参考: https://www.w3school.com.cn/jquery/ajax_ajax.asp https://blog.csdn.net/Wen__Fei/article/details/101458322
|