小伙伴们在开箱Vue3的过程中一点会踩到不少坑。
比如很多小伙伴想要通过ref来操纵DOM,可偏偏翻车。
这里分享两个常用的方法,使用以下两个例子为例。
父组件
<template>
<child ref="childRef"></child>
</template>
<script setup>
import { ref } from "vue";
// 引入子组件
import child from "./child.vue";
// 获取子组件
const childRef = ref(null);
const fun = () => {
childRef.value.childFun();// 调用子组件的方法
}
</script >
方法一:
defineComponent
如果你使用的是defineComponent定义组件的方法,
那么除了在父组件绑定ref="childRef"外,最关键在于你需要把你想要在父组件中控制的方法return出去。
//子组件一 child
<template>
<div>
<a-modal title="Title" v-model:visible="visible" :confirm-loading="confirmLoading" @ok="handleOk">
<p>{{ modalText }}</p>
</a-modal>
</div>
</template>
<script lang="ts">
import { ref, defineComponent } from 'vue';
export default defineComponent({
setup() {
const modalText = ref<string>('Content of the modal');
const visible = ref<boolean>(false);
const confirmLoading = ref<boolean>(false);
const childFun = () => {
visible.value = true;
};
const handleOk = () => {
modalText.value = 'The modal will be closed after two seconds';
confirmLoading.value = true;
setTimeout(() => {
visible.value = false;
confirmLoading.value = false;
}, 2000);
};
return {
modalText,
visible,
confirmLoading,
childFun,
handleOk,
};
},
});
</script>
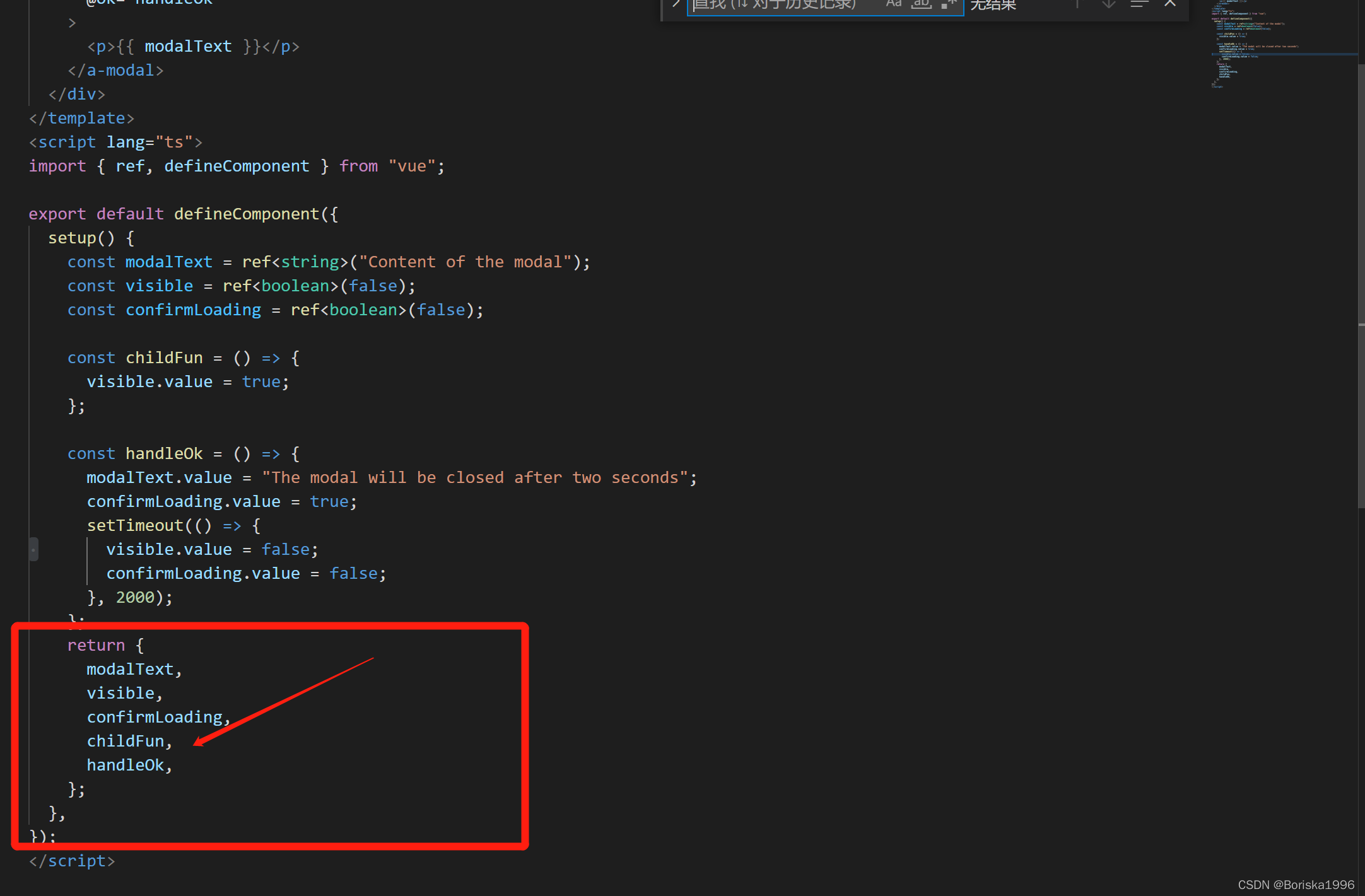
方法二:
setup语法糖
如果你使用的setup导出定义组件的方法,你就需要把将要在父组件中使用的数据和方法使用defineExpose暴露出去。
// 子组件
<script setup>
import { defineExpose } from 'vue'
const childFun = () => {
console.log('我是子组件方法')
}
defineExpose({
childFun
})
</script>
|