什么是防抖和节流
- 防抖: 触发高频事件后n秒后,函数只会执行依次,如果n秒内再次触发,则重新计时
- 节流: 高频事件触发,但是在n秒内只会执行一次,在这n秒内,无论触发多少次,只会执行一次,节流会稀释函数的执行频率
防抖的应用场景:
1 resize窗口缩放 2 输入框中不停输入
节流应用场景
1 scroll滚动事件,上拉加载 2 按钮不停点击
debounce.js
function debounce(fn,delay=500) {
let timer = null;
return function() {
if(timer) clearTimeout(timer);
timer = setTimeout(() => { // 箭头函数捕获上下文的this
fn.apply(this,arguments)
},delay)
// timer = setTimeout(function() { // this指向window
// console.log("_this",_this)
// console.log("this",this) // window
// fn.apply(_this,arguments)
// });
}
}
throttle.js(变量版本)
function throttle(fn,delay=500) {
let flag = true;
return function() {
let _this = this;
if(!flag) return
flag = false;
setTimeout(() => {
fn.apply(_this,arguments)
flag = true
},delay)
}
}
throttle.js(时间戳版本)
function throttle(fn,delay=500) {
let previous = 0;
return function() {
let now = Date.now()
if(now - previous > delay) {
fn.apply(this,arguments)
previous = now;
}
}
}
<body>
<div id="app">
</div>
<script>
// 防抖
function debounce(fn,delay=500) {
let timer = null;
return function() {
if(timer) clearTimeout(timer);
timer = setTimeout(() => { // 箭头函数捕获上下文的this
fn.apply(this,arguments)
},delay)
// timer = setTimeout(function() { // this指向window
// console.log("_this",_this)
// console.log("this",this) // window
// fn.apply(_this,arguments)
// });
}
}
// 节流throttle (变量标识符版本)
function throttle(fn,delay=500) {
let flag = true;
return function() {
let _this = this;
if(!flag) return
flag = false;
setTimeout(() => {
fn.apply(_this,arguments)
flag = true
},delay)
}
}
// // 节流throttle (时间戳版本)
function throttle(fn,delay=500) {
let previous = 0;
return function() {
let now = Date.now()
if(now - previous > delay) {
fn.apply(this,arguments)
previous = now;
}
}
}
var app = document.querySelector("#app");
var count = 0;
function doSomething() {
app.innerHTML= count++;
}
// window.addEventListener("mousemove",debounce(doSomething))
window.addEventListener("mousemove",throttle(doSomething))
// app.onmousemove = throttle(doSomething)
</script>
防抖节流在vue中使用
- 新建optimization(优化).js
- optimization.js
export function debounce(fn,delay=500) {
let timer = null;
return function() {
if(timer) clearTimeout(timer)
timer = setTimeout(()=>{
fn.apply(this,arguments)
},delay)
}
}
export function throttle(fn,wait=500) {
let previous = 0;
return function() {
let now = Date.now();
if(now - previous > wait) {
fn.apply(this,arguments)
}
}
}
<template>
<div id="app">
<div>
<button @click="clickHandler">不是节流点击</button>
</div>
<div>
<button @click="clickHandler1">是节流点击</button>
</div>
<div>
不是防抖: <input type="text" @input="inputHandler">
</div>
<div>
是防抖:<input type="text" @input="inputHandler1">
</div>
</div>
</template>
<script>
import { debounce,throttle} from './utils/optimization.js'
export default {
data() {
return {
num: 0
}
},
methods: {
clickHandler: throttle(function() {
console.log("普通点击")
}),
clickHandler1: throttle(function() {
console.log("节流点击")
}),
inputHandler() {
console.log("普通输入")
},
inputHandler1: debounce(function() {
console.log("防抖输入")
})
}
}
</script>
<style lang="less">
#app {
width: 400px;
height: 400px;
text-align: center;
font-size: 30px;
}
</style>
展示的效果如下,可以看出节流稀释了执行频率,防抖只有最后一次输入才有效
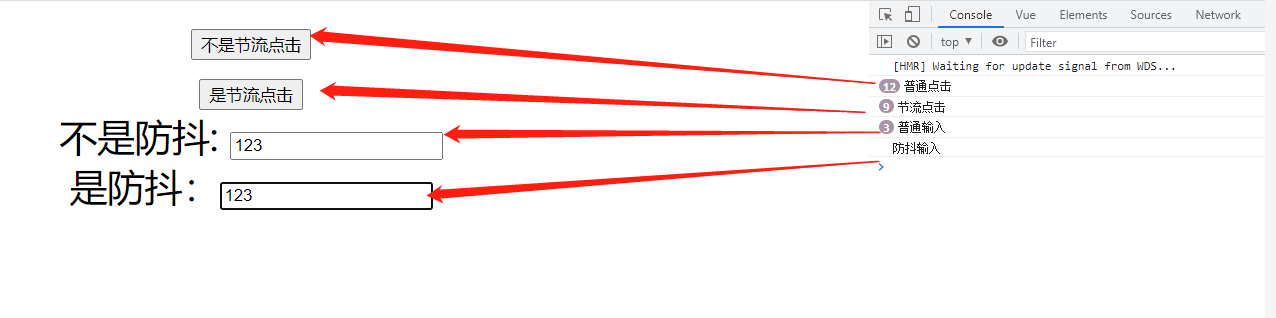
|