react事件绑定this的几种写法
正常我们不绑定this,this是null
import React, { Component } from 'react'
export default class App extends Component {
constructor() {
super()
this.state = { }
}
clickHandler() {
console.log("this",this);
}
render() {
return (
<div>
<h1>我是App页面</h1>
<button onClick={ this.clickHandler }>点击按钮</button>
</div>
)
}
}
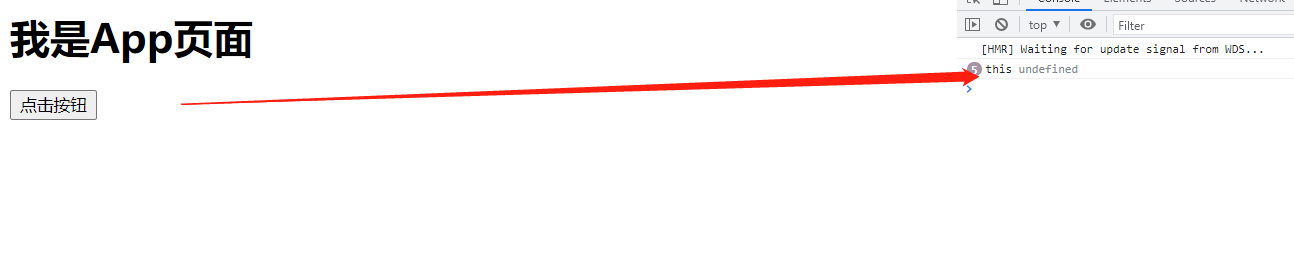
1: this.clickHandler.bind(this)
import React, { Component } from 'react'
export default class App extends Component {
constructor() {
super()
this.state = {}
}
clickHandler() {
console.log("this",this);
}
render() {
return (
<div>
<h1>我是App页面</h1>
<button onClick={ this.clickHandler.bind(this) }>点击按钮</button>
</div>
)
}
}
2: this.clickHandler = this.clickHandler.bind(this)
import React, { Component } from 'react'
export default class App extends Component {
constructor() {
super()
this.state = {}
this.clickHandler = this.clickHandler.bind(this)
}
clickHandler() {
console.log("this",this);
}
render() {
return (
<div>
<h1>我是App页面</h1>
<button onClick={ this.clickHandler }>点击按钮</button>
</div>
)
}
}
3: () => this.clickHandler()
import React, { Component } from 'react'
export default class App extends Component {
constructor() {
super()
this.state = {}
}
clickHandler() {
console.log("this",this);
}
render() {
return (
<div>
<h1>我是App页面</h1>
<button onClick={ () => this.clickHandler() }>点击按钮</button>
</div>
)
}
}
4: clickHandler = () => { console.log(“this”,this); } (工作中推荐这种写法)
import React, { Component } from 'react'
export default class App extends Component {
constructor() {
super()
this.state = {}
}
clickHandler = () => { console.log("this",this); } // 推荐写法
render() {
return (
<div>
<h1>我是App页面</h1>
<button onClick={ this.clickHandler }>点击按钮</button>
</div>
)
}
}
4中绑定this展示效果

|