单项数据流
React的特性中有一个概念叫做 “单项数据流” ,可能刚刚接触React的小伙伴不太明白这个概念,还是拿出《服务菜单》的 Demo ,来给大家讲解。比如我们在父组件中可以直接把this.state.list 传递过来。
例如下面代码:
<ul>
{
this.state.list.map((item,index)=>{
return (
<XiaojiejieItem
key={index+item}
content={item}
index={index}
list={this.state.list}
deleteItem={this.deleteItem.bind(this)}
/>
)
})
}
</ul>
其实这样传是没有问题的,问题是你只能使用这个值,而不能修改这个值,如果你修改了,比如我们把代码写成这样:
handleClick(){
//关键代码——---------start
this.props.list=[]
//关键代码-----------end
this.props.deleteItem(this.props.index)
}
就会报下面的错误;
TypeError: Cannot assign to read only property 'list' of object '#<Object>'
意思就是 list 是只读的,单项数据流。
那如果要改变这里边的值怎么办? 其实上篇《父子组件传值》已经讲过了,就是通过传递父组件的方法。
涉及的所有文件
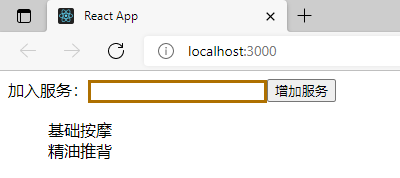 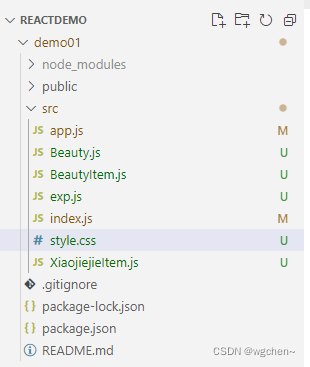
style.css
.input {border:3px solid #ae7000}
index.js
import React from 'react'
import ReactDOM from 'react-dom'
import App from './Beauty'
ReactDOM.render(<App />,document.getElementById('root'))
Beauty.js
import React,{Component,Fragment } from 'react'
import XiaojiejieItem from './XiaojiejieItem'
class Beauty extends Component{
//js的构造函数,由于其他任何函数执行
constructor(props){
super(props) //调用父类的构造函数,固定写法
this.state={
inputValue:'' , // input中的值
list:['基础按摩','精油推背'] //服务列表
}
}
render(){
return (
<Fragment>
{/* 正确注释的写法 */}
<div>
<label htmlFor="willem">加入服务:</label>
<input id="willem" className="input" value={this.state.inputValue} onChange={this.inputChange.bind(this)} />
<button onClick={this.addList.bind(this)}> 增加服务 </button>
</div>
<ul>
{
this.state.list.map((item,index)=>{
return (
<XiaojiejieItem
key={index+item}
content={item}
index={index}
list={this.state.list}
deleteItem={this.deleteItem.bind(this)}
/>
)
})
}
</ul>
</Fragment>
)
}
inputChange(e){
// console.log(e.target.value);
// this.state.inputValue=e.target.value;
this.setState({
inputValue:e.target.value
})
}
//增加服务的按钮响应方法
addList(){
this.setState({
list:[...this.state.list,this.state.inputValue],
inputValue:''
})
}
//删除单项服务
deleteItem(index){
let list = this.state.list
list.splice(index,1)
this.setState({
list:list
})
}
}
export default Beauty
XiaojiejieItem.js
import './style.css'
import React, { Component } from 'react'; //imrc
class XiaojiejieItem extends Component { //cc
//--------------主要代码--------start
constructor(props){
super(props)
this.handleClick=this.handleClick.bind(this)
}
//--------------主要代码--------end
render() {
return (
<div onClick={this.handleClick} dangerouslySetInnerHTML={{__html:this.props.content}}>
</div>
);
}
handleClick(){
console.log(this.props.index)
this.props.deleteItem(this.props.index)
}
}
export default XiaojiejieItem;
和其他框架配合使用
有小伙伴问我,React 和 jquery 能一起使用吗?
答案: 是可以的,React其实可以模块化和组件化开发。 看 /public/index.html 文件,代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="theme-color" content="#000000" />
<link rel="manifest" href="%PUBLIC_URL%/manifest.json" />
<title>React App</title>
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
</body>
</html>
其实React只对这一个 <div> ,外边的其他DOM并不受任何影响,比如我们在它的下方再写一个<div> ,然后查看效果。
<div id="root"></div>
<div style="color:red">今天过的好开心,服务很满意!</div>
你可以在其他的 div 里加入任何内容,但是这种情况很少,我也不建议这么使用。希望小伙伴们还是统一技术栈。
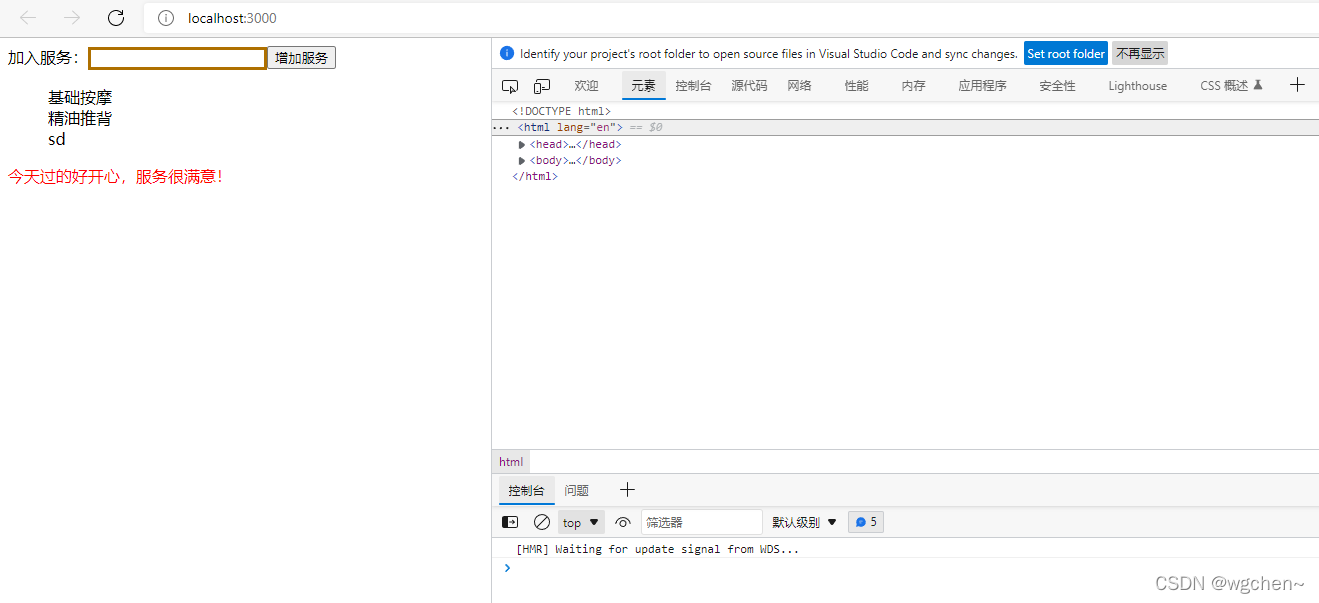
函数式编程
在面试React时,经常会问道的一个问题是: 函数式编程的好处是什么?
1、函数式编程让我们的代码更清晰,每个功能都是一个函数。 2、函数式编程为我们的代码测试代理了极大的方便,更容易实现前端自动化测试。
React框架也是函数式编程,所以说优势在大型多人开发的项目中会更加明显,让配合和交流都得心应手。
|