v-text
<div id="root">
<h2 v-text="msg">哈哈哈</h2>
<h2 v-text="a"></h2>
<h2>{{ msg }}</h2>
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: "#root",
data: {
msg: "hello dudu",
a: "<hr></hr>",
},
});
</script>
v-html
<div id="root">
<h2 v-html="a">哈哈哈</h2>
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: "#root",
data: {
a: "<hr></hr>",
},
});
</script>
v-cloak
<style>
[v-cloak] {
display: none;
}
</style>
<div id="root">
<h2 v-cloak>{{ msg }}</h2>
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: "#root",
data: {
msg: '大河之剑天上来!'
},
});
</script>
v-once
<div id="root">
<h2 v-once>初始化n的值为:{{ n }}</h2>
<h2>当前n的值为:{{ n }}</h2>
<button @click="n++">点我n+1</button>
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: "#root",
data: {
n: 1,
},
});
</script>
v-pre
<div id="root">
<h2 v-pre>hello dudu</h2>
<h2>当前n的值为:{{ n }}</h2>
<button @click="n++">点我n+1</button>
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: "#root",
data: {
n: 1
},
});
</script>
自定义指令
<div id="root">
<h2>当前的n值是:<span v-text="n"></span></h2>
<h2>放大后的n值是:<span v-big="n"></span></h2>
<button @click="n++">点我n+1</button>
<hr>
<input type="text" v-fbind:value="n">
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: "#root",
data: {
n: 1
},
directives: {
big(element, binding) {
element.innerText = binding.value * 10;
console.log("big");
},
fbind: {
bind(element, binding) {
console.log('bind');
element.value = binding.value;
},
inserted(element, binding) {
console.log('inserted');
element.focus();
},
update(element, binding) {
console.log('update');
element.value = binding.value;
}
}
}
});
</script>
1. 如果指令名比较复杂需要多个单词,单词之间使用 - 连接(v-big-number),在配置directives时,
需要使用单引号来声明对象的key('big-number':{})
2. 指令回调函数中的this都是window对象
3. 自定义指令只能在当前的Vue实例中使用,
如果要全局使用,可以使用 Vue.directive('fbind', {配置对象})
生命周期
生命周期又叫生命周期回调函数、生命周期函数、生命周期钩子
生命周期函数是Vue在关键时刻帮我们调用的一些特殊名称的函数
生命周期函数的名字不可更改,但函数的具体内容是程序员根据需求编写的
生命周期函数中的this指向的是vm或组件实例对象
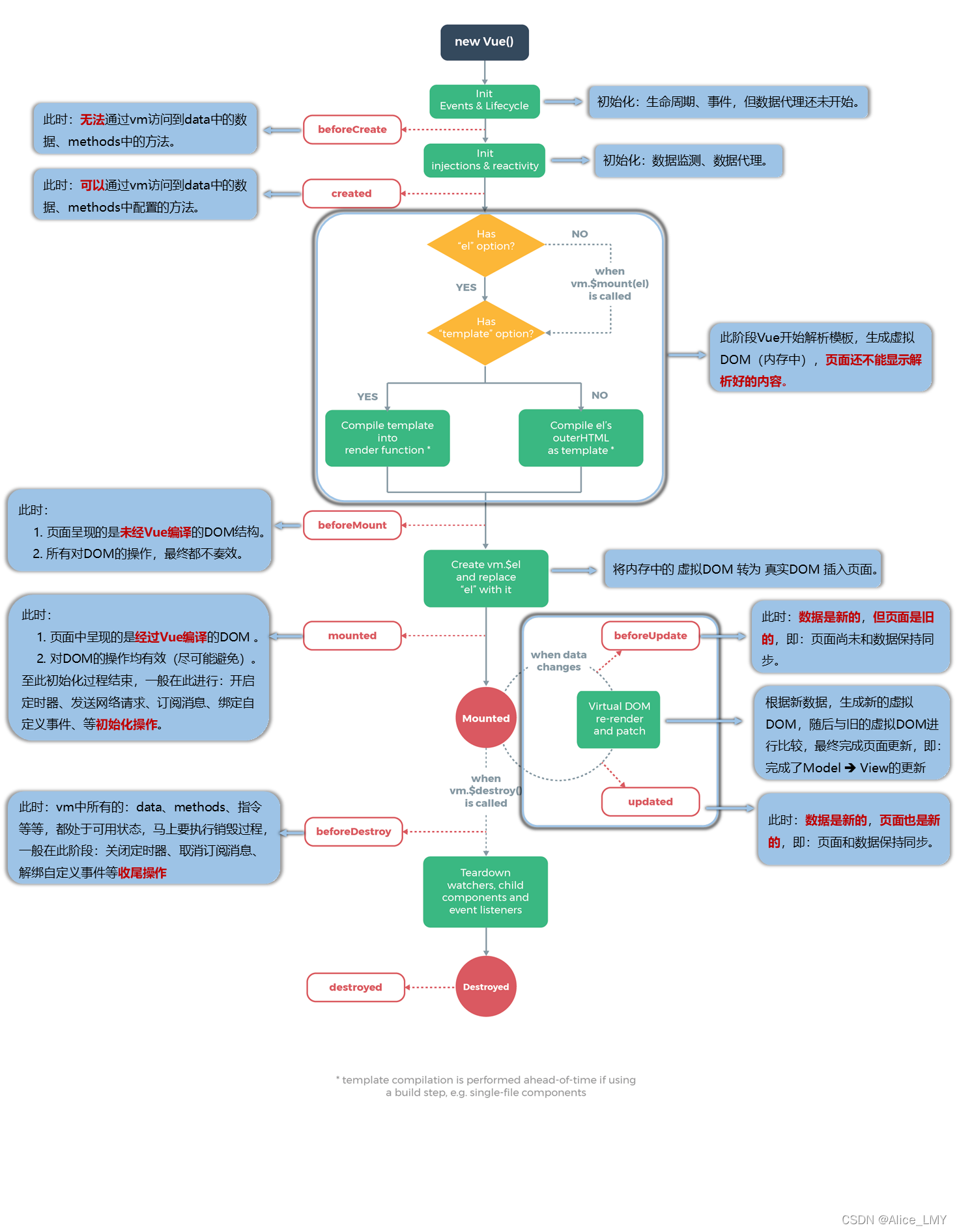
关于销毁Vue实例
1. 销毁后借助Vue开发者工具看不到任何信息
2. 销毁后自定义事件会失效,但原生DOM事件依然有效
3. 一般不会在beforeDestroy中操作数据,因为即便操作数据,也不会再出发更新流程了
非单文件组件
1. 组件的使用
<div id="root">
<h1>{{msg}}</h1>
<hr>
<school></school>
<hr>
<student></student>
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const school = Vue.extend({
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="show">点我提示学校名</button>
</div>
`,
data() {
return {
schoolName: 'sgg',
address: 'beijing'
}
},
methods: {
show() {
alert(this.schoolName)
}
},
});
const student = Vue.extend({
template: `
<div>
<h2>学生姓名:{{studentName}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
`,
data() {
return {
studentName: 'dudu',
age: 22
}
}
});
Vue.component('school', school);
const vm = new Vue({
el: "#root",
components: { student },
data: {
msg: "你好啊"
},
});
</script>
一些注意点
组件名写法:
* 一个单词:school 或者 School
* 多个单词:MySchool(需要脚手架支持) 或者 my-school
标签名写法:
* <school></school> 或者 <school/>(需要使用脚手架,否则后续组件不能渲染)
创建组件的简写方式:const school = {}
可以在对象中添加name属性指定组件在开发者工具中呈现的名字 const school = { name: 'SchoolNameTest', template... }
2. 组件的嵌套
标准写法
<div id="root"></div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const student = {};
const school = {
template: `<student></student>`,
components: { student }
};
const hello = {};
const app = {
template: `
<div>
<hello></hello>
<hr>
<school></school>
</div>
`,
components: { school, hello }
};
const vm = new Vue({
el: "#root",
template: `<app></app>`,
components: { app },
});
</script>
3. VueComponent 构造函数
<div id="root">
<hello></hello>
<school></school>
</div>
<script src="../tools/vue.js"></script>
<script>
Vue.config.productionTip = false;
const school = Vue.extend({
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="show">点我提示学校名</button>
</div>
`,
data() {
return {
schoolName: 'sgg',
address: 'beijing'
}
},
methods: {
show() {
console.log("show", this);
alert(this.schoolName);
}
},
});
const hello = Vue.extend({
template: `
<div>
<h1>{{msg}}</h1>
</div>
`,
data() {
return {
msg: '你好',
}
},
});
console.log("@", school);
console.log(hello === school);
const vm = new Vue({
el: "#root",
components: { school, hello }
});
console.log(vm);
</script>
一个重要的内置关系:VueComponent.prototype.__proto__ === Vue.prototype ,作用是让vc可以访问到Vue原型上的属性和方法
|