一、Vue3-初识
首先对于vue.js 3.0,它是vue.js 2.0的升级版本,总得来说是对响应式,模板等,更多的是对性能方面的优化,在底层代码和api方面都有更新,而且在vue3中,逐渐使用typeScript语言,更严格!

1、通过script引入的方式实现vue3应用
vue.global.js
<script src="../vuejs/vue.global.js"></script>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body>
<div id="counter">
<h1>counter:{{ num }}</h1>
</div>
<script src="../vuejs/vue.global.js"></script>
<script>
const Counter = {
data: function() {
return {
num: 0
};
}
};
let app = Vue.createApp(Counter).mount("#counter");
</script>
</body>
</html>
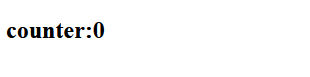
//方式一
data: function() {
return {
num: 0
};
}
//方式二
data1(){
return{
num:0
}
}
2.vite构建工具创建vue3项目
1.按照步骤输入命令,生成文件目录
npm init vite-app vue3demo02
cd vue3demo02
npm install
npm run dev
按住ctrl点击链接打开项目 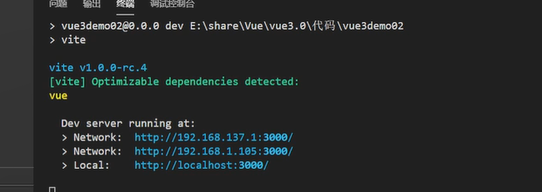
入口文件 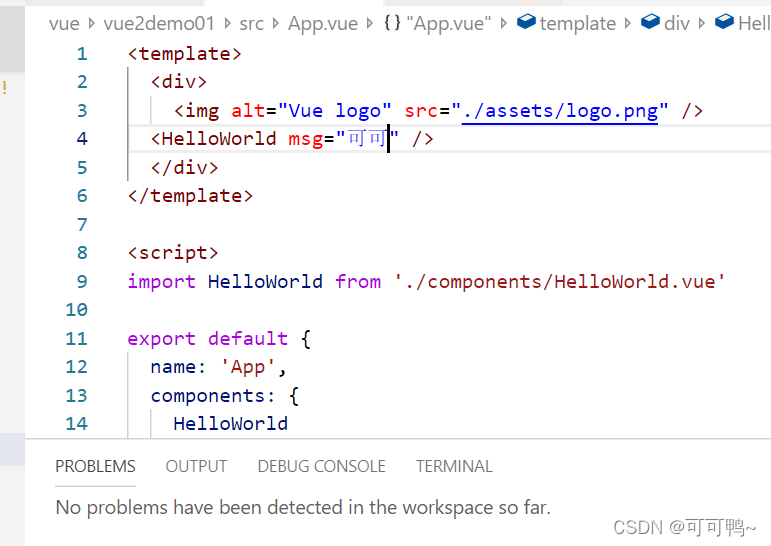 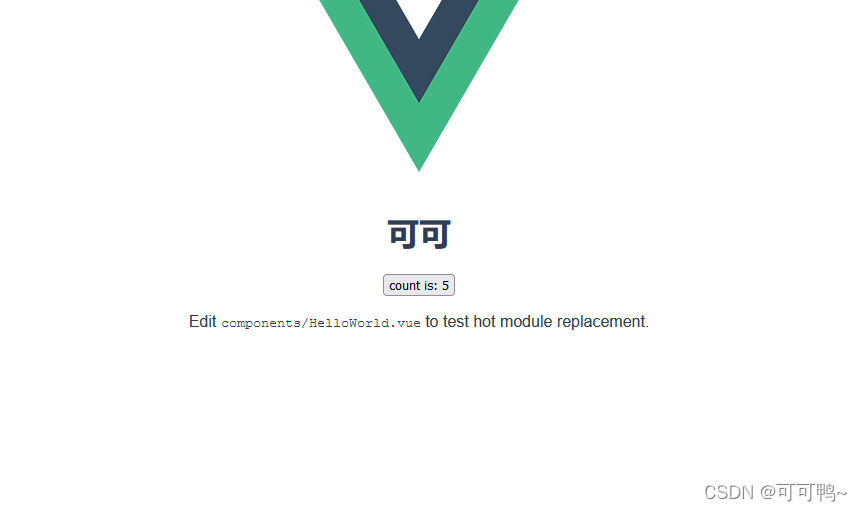
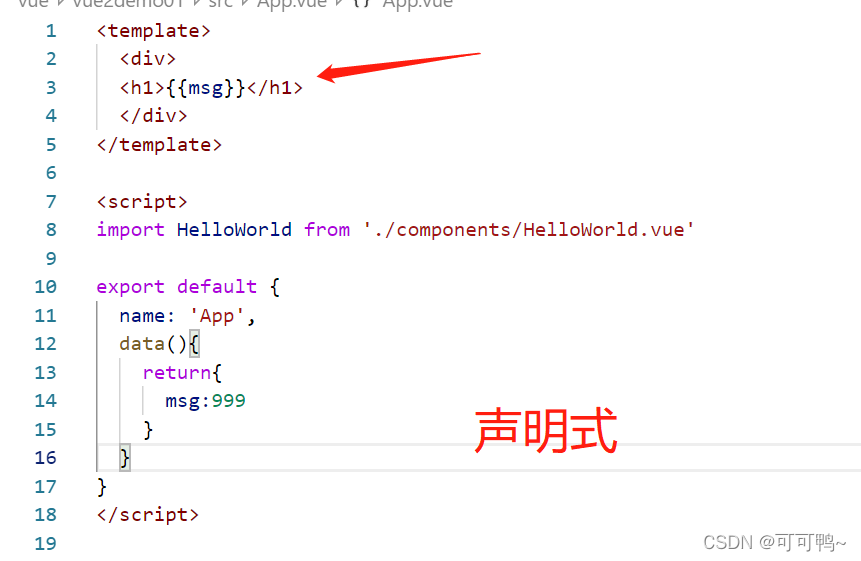 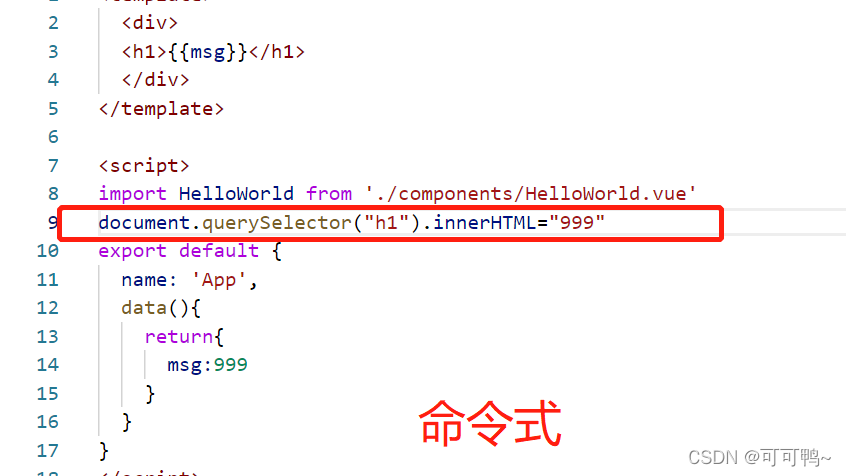 示例
<template>
<div>
<h1>{{msg}}</h1>
<button @click="changeMsg">显示内容</button>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
msg:999
}
},
methods:{
changeMsg(){
this.msg = "你今天有没有喝牛奶"
}
}
}
</script>
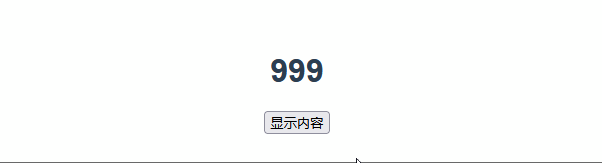
二、基本操作
1.Vue声明式语法与数据双向绑定
双向绑定 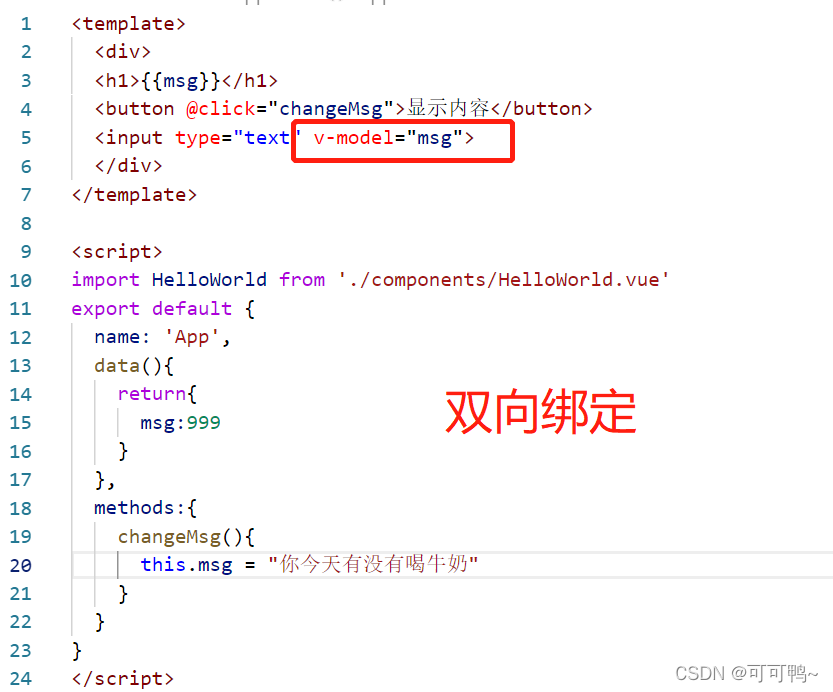
###### 4.模板语法常用指令 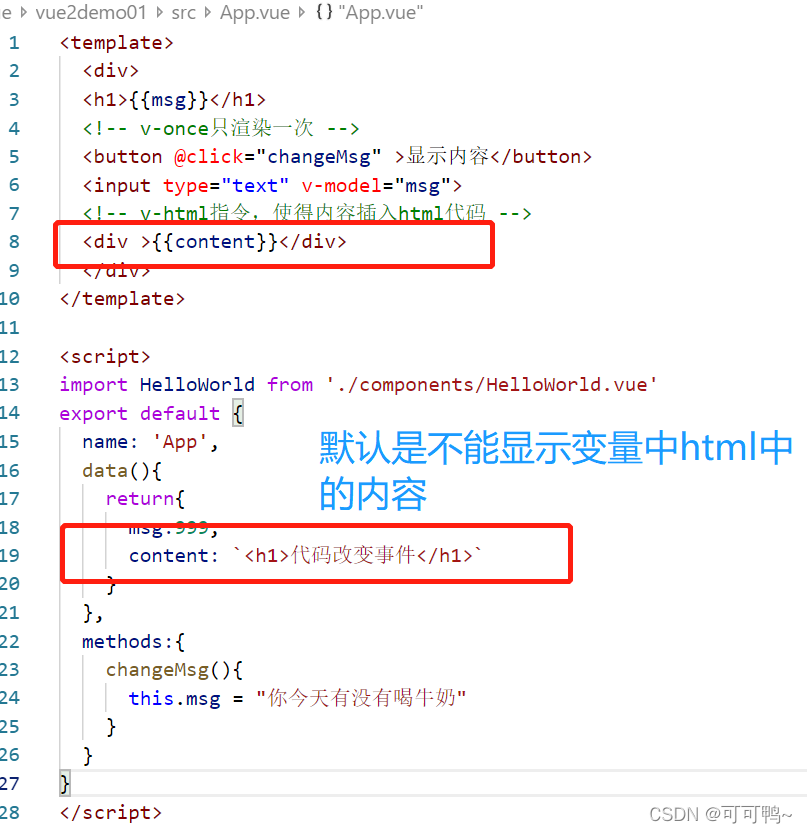
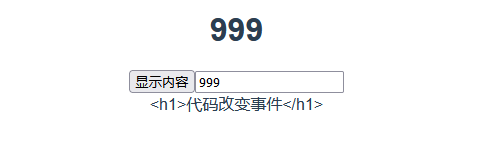 使用 v-html指令
<template>
<div>
<h1>{{msg}}</h1>
<button @click="changeMsg" >显示内容</button>
<input type="text" v-model="msg">
<div v-html="content"></div>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
msg:999,
content: `<h1>代码改变事件</h1>`
}
},
methods:{
changeMsg(){
this.msg = "你今天有没有喝牛奶"
}
}
}
</script>
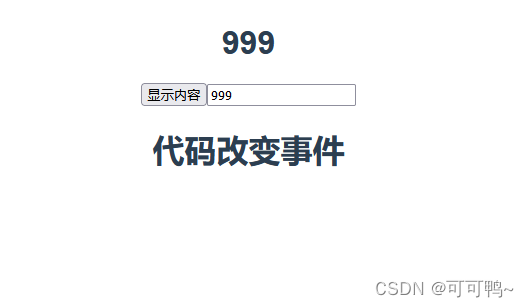
<template>
<div>
<h1>{{msg}}</h1>
<button @click="changeMsg" >显示内容</button>
<input type="text" v-model="msg">
<div v-html="content"></div>
<div :id="id" :class="id"></div>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
msg:999,
content: `<h1>代码改变事件</h1>`,
id:"d1"
}
},
methods:{
changeMsg(){
this.msg = "你今天有没有喝牛奶"
this.id = "d2"
}
}
}
</script>
<style>
.d1{
width: 100px;
height: 100px;
background-color: rgb(45, 155, 155);
}
.d2{
width: 100px;
height: 100px;
background-color: blueviolet;
}
</style>
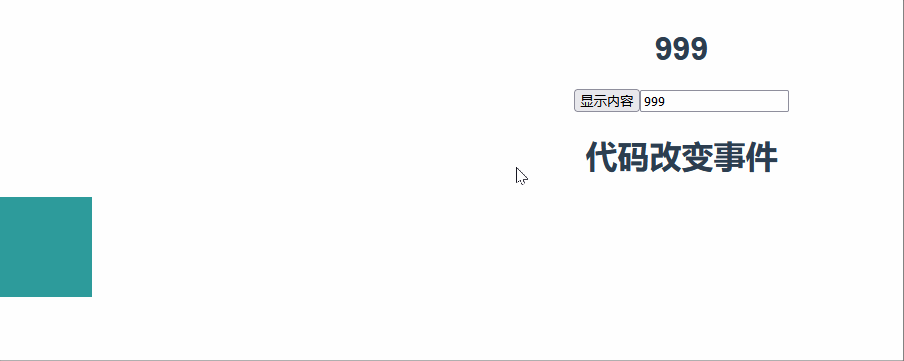
2.JavaScript表达式在模板中使用
<template>
<div>
<h1>{{msg.split('')}}</h1>
<h1>{{msg.split('').reverse()}}</h1>
<h1>{{msg.split('').reverse().join('')}}</h1>
<div :id="msg.split('').reverse().join('')"></div>
<h1>{{color=="red"?"开心":"难过"}}</h1>
<button @click="changeMsg">颜色切换</button>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
msg:"HelloWorld",
color:"red"
}
},
methods:{
changeMsg(){
this.color = "pink"
}
}
}
</script>
<style>
</style>
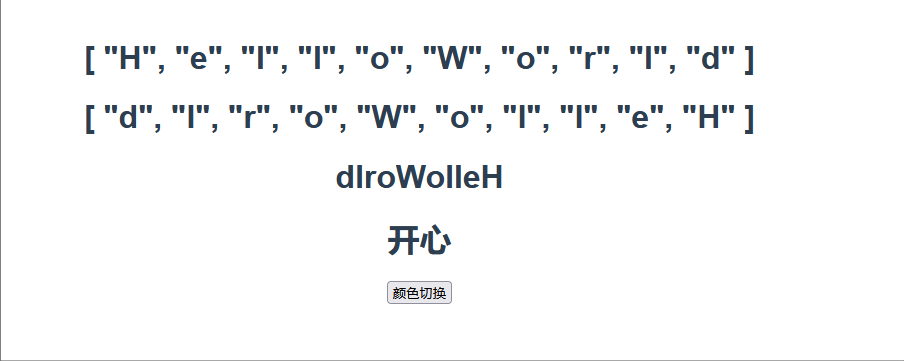
3.动态指令(比较少用)
<template>
<div>
<div :[attributeName]="id"></div>
<div></div>
<button @click="changeMsg">切换颜色</button>
<button @[eventName]="changeMsg">切换颜色</button>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
attributeName:"class",
id:"d1",
eventName:"click"
}
},
methods:{
changeMsg(){
this.id = "d2"
}
}
}
</script>
<style>
.d1{
width: 100px;
height: 100px;
background-color: rgb(45, 155, 155);
}
.d2{
width: 100px;
height: 100px;
background-color: blueviolet;
}
</style>
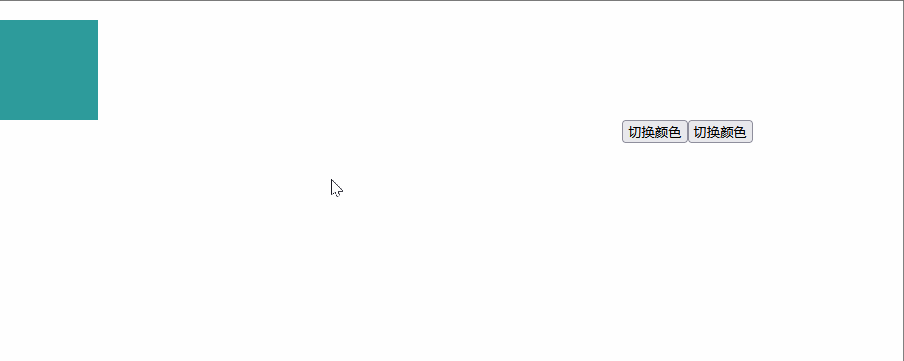
4.计算属性
因为在插值表达式中方太多的逻辑对(逻辑:split,join,reverse等方法),不方便
<template>
<div>
<div>{{reverseMsg}}</div>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
msg:"click"
}
},
computed:{
reverseMsg:function(){
return this.msg.split('').reverse().join('')
}
},
}
</script>
结果:kcilc
5.watch监听数据的变化
<template>
<div>
<input type="text" v-model="msg">
<h1>{{msg}}</h1>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
msg:"click"
}
},
computed:{
reverseMsg:function(){
return this.msg.split('').reverse().join('')
}
},
methods:{
},
watch:{
msg:function(newvalue,oldvalue){
console.log(newvalue)
console.log(oldvalue)
if(newvalue.length<3){
alert("输入内容太短!")
}
}
}
}
</script>
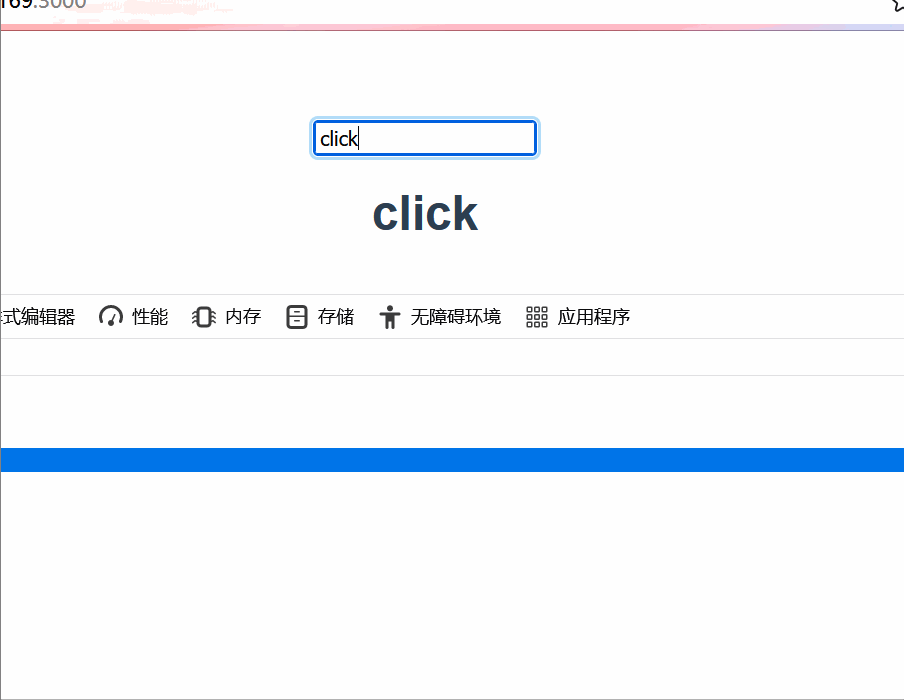
6.class类名的多种操作方式
<template>
<div>
<div :class="msg"></div>
<dir :class="{active:isShow}">hello</dir>
<div :class="arry">hello</div>
<div :class="[classname,{active:isShow}]"></div>
<button @click="taggleBtn">taggleBtn</button>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
msg:"d1",
isShow:true,
arry:['active','d2'],
classname:'d3'
}
},
methods:{
taggleBtn:function(){
this.isShow = ! this.isShow
this.arry.pop();
}
},
}
</script>
<style>
.d1{
background-color: rgb(255, 176, 19);
height: 20px;
}
.active{
background-color: aquamarine;
}
.d2{
width: 200px;
height: 20px;
}
.d3{
margin-top: 15px;
width:100%;
height: 20px;
}
</style>

<template>
<div>
<div v-if="user=='vip'">金主爸爸</div>
<div v-else>金主宝宝</div>
<h1 v-show="isShow">充值会让你更强大!</h1>
<button @click="taggleBtn">切换</button>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
user:"vip",
isShow:"true"
}
},
methods:{
taggleBtn:function(){
this.isShow = ! this.isShow
}
},
}
</script>
7.列表渲染
<template>
<div>
<ol>
<li v-for="(item,i) in news" :key="i">{{item}}</li>
</ol>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
user:"vip",
isShow:"true",
news:[
"国内",
"国外",
]
}
},
}
</script>
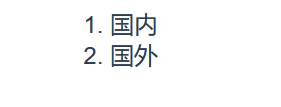
<template>
<div>
<ol>
<li v-for="(item,i) in news" :key="i">{{item.title}}</li>
</ol>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
user:"vip",
isShow:"true",
news:[
{
title:"直升机坠落",
tag:"热"
},
{
title:"中秋出现圆月",
tag:"新"
}
]
}
},
methods:{
taggleBtn:function(){
this.isShow = ! this.isShow
}
},
}
</script>
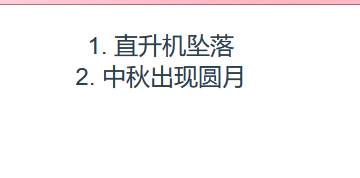
<template>
<div>
<ol>
<li v-for="(item,i) in table" :key="i">{{item+""+i}}</li>
</ol>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
user:"vip",
isShow:"true",
table:{
name:"coco",
age:18
}
}
},
methods:{
taggleBtn:function(){
this.isShow = ! this.isShow
}
},
}
</script>
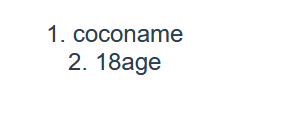
8.事件与参数和事件修饰符
<template>
<div>
<h1 @click="addEvent" class="d1">{{num}}</h1>
<h1 v-on:click="addEvent" class="d1">{{num}}</h1>
<h1 v-on:click="num++" class="d1">{{num}}</h1>
<h1 @click="addEvent(),changeColor()" :style="{background:color}">{{num}}</h1>
<h1 v-on:click.once="addEvent" class="d1">{{num}}</h1>
<input type="text" @keydown.enter="searchEvent">
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
num:0,
color:"red"
}
}
,
methods:{
addEvent:function(){
this.num++;
},
changeColor:function(){
this.color = "pink"
},
searchEvent:function(){
console.log("执行了回车搜索事件")
}
},
}
</script>
<style>
.d1{
background-color: aquamarine;
}
</style>
9.表单的数据双向绑定v-model与修饰符
复选框
香蕉<input type="checkbox" value="香蕉" v-model="fruits">
苹果<input type="checkbox" value="苹果" v-model="fruits">
葡萄<input type="checkbox" value="葡萄" v-model="fruits">
草莓<input type="checkbox" value="草莓" v-model="fruits">
<h2>{{fruits}}</h2>
多行文本输入
<textarea name="" id="" cols="30" rows="10" v-model="cheack"></textarea>
<h1>{{cheack}}</h1>
单选框
女<input type="radio" value="女" v-model="sex">
男<input type="radio" value="男" v-model="sex">
<h2>{{sex}}</h2>
选项框单选
<select name="" id="" v-model="city">
<option value="上海">上海</option>
<option value="北京">北京</option>
<option value="深圳">深圳</option>
<option value="青岛">青岛</option>
</select>
<h2>{{city}}</h2>
选项框多选
<select name="" id="" v-model="citys" multiple>
<option value="上海">上海</option>
<option value="北京">北京</option>
<option value="深圳">深圳</option>
<option value="青岛">青岛</option>
</select>
<h2>{{citys}}</h2>
表单获取是否选中
<input type="checkbox" value="" v-model="checked1" true-value="喜欢" false-value="不喜欢" name="like">
<h2>{{checked1}}</h2>
.lazy、input变为change事件改变值
<input type="text" v-model.lazy="lazy" >
<h2>{{lazy}}</h2>
number修饰符转化为数字
<input type="text" v-model.lazy.number="changenumber">
<h2>{{changenumber}}</h2>
trim修饰符去掉空格
<input type="text" v-model.trim="changenumber">
<template>
<div class="content">
<textarea name="" id="" cols="30" rows="10" v-model="cheack"></textarea>
<h1>{{cheack}}</h1>
香蕉<input type="checkbox" value="香蕉" v-model="fruits">
苹果<input type="checkbox" value="苹果" v-model="fruits">
葡萄<input type="checkbox" value="葡萄" v-model="fruits">
草莓<input type="checkbox" value="草莓" v-model="fruits">
<h2>{{fruits}}</h2>
女<input type="radio" value="女" v-model="sex">
男<input type="radio" value="男" v-model="sex">
<h2>{{sex}}</h2>
<select name="" id="" v-model="city">
<option value="上海">上海</option>
<option value="北京">北京</option>
<option value="深圳">深圳</option>
<option value="青岛">青岛</option>
</select>
<h2>{{city}}</h2>
<select name="" id="" v-model="citys" multiple>
<option value="上海">上海</option>
<option value="北京">北京</option>
<option value="深圳">深圳</option>
<option value="青岛">青岛</option>
</select>
<h2>{{citys}}</h2>
<input type="checkbox" value="" v-model="checked1" true-value="喜欢" false-value="不喜欢" name="like">
<h2>{{checked1}}</h2>
<input type="text" v-model.lazy="lazy" >
<h2>{{lazy}}</h2>
<input type="text" v-model.lazy.number="changenumber">
<h2>{{changenumber}}</h2>
<input type="text" v-model.trim="changenumber">
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
data(){
return{
cheack:"",
fruits:[],
sex:"",
city:"青岛",
citys:["青岛"],
checked1:"喜欢",
lazy:"",
changenumber:"1"
}
}
,
methods:{
searchEvent:function(){
console.log("执行了回车搜索事件")
},
},
}
</script>
<style>
textarea{
resize: none;
width: 200px;
height: 50px;
}
</style>
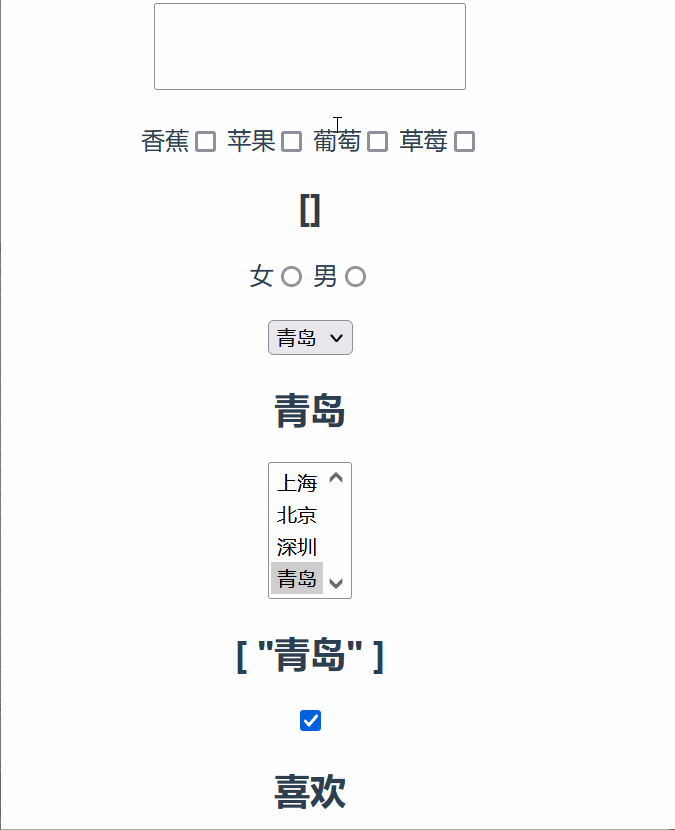
结束~~
|