vuex配置
store的index.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
count: 0
},
mutations: {
},
actions: {
},
modules: {
}
})
main.js进行挂载store
import Vue from 'vue'
import App from './App.vue'
import store from './store'
Vue.config.productionTip = false
new Vue({
store,
render: h => h(App)
}).$mount('#app')
一、state
方式1
$store.state.count
{{$store.state.count}}
方式2
1.先导入mapState
import { mapState } from 'vuex'
2.在computed 全局使用count,... 是展开运算符
import { mapState } from 'vuex'
export default {
data () {
return {}
},
computed: {
...mapState(['count'])
}
}
</script>
使用这两种方法都可以访问到count
二、mutations
在mutations访问state
方法1
1.在mutations 修改state 里面的数据 (官方规定 ,只有mutations才能修改state里面的数据) 2.在mutations定义一函数 ,函数的第一个参数 必须是state ,第二个参数 从组件里面传来 的数据,这里是自增的count任意数,
export default new Vuex.Store({
state: {
count: 0
},
mutations: {
add (state, step) {
state.count += step
}
},
actions: {
},
modules: {
}
})
3.commit 的作用就是调用 某个mutations函数 ,这里是add(){}
methods: {
addition () {
console.log(this)
this.$store.commit('add', 3)
}
}
方法2
在mutations 函数定义jianfa(){} 函数,组件可以通过导入mapMutations包来用这个函数 不要在mutations函数中进行异步操作
export default new Vuex.Store({
state: {
count: 0
},
mutations: {
add (state, step) {
state.count += step
},
jianfa (state, step) {
state.count -= step
}
},
actions: {
},
modules: {
}
})
1.从vuex中导入mapMutations函数
import {mapMutations } from 'vuex'
2.通过刚才导入的mapMutations函数,将需要的mutations函数,映射为当前组件的methods方法
methods: {
...mapMutations(['jianfa']),
reMove () {
this.jianfa(2)
}
}
三、actions
actions通过mutations间接访问state
方法一 this.$store.dispatch()
触发action异步任务不带参数的时候
在actions里面要通过mutations函数 调用里面的add(){} 函数方法,这还是说明state 只能通过mutations修改,actions只是间接 通过mutations修改访问了state数据
<h3>当前的count值为:{{ $store.state.count }}</h3>
<button @click="asyncAdd">异步+1</button>
export default new Vuex.Store({
state: {
count: 0
},
mutations: {
add (state, step) {
state.count += step
},
addd (state) {
state.count += 1
},
jianfa (state, step) {
state.count -= step
}
},
actions: {
addAsync (context) {
setTimeout(() => {
context.commit('addd')
}, 1000)
}
},
modules: {
}
})
1.actions 处理异步 任务 2.如果通过异步操作变更数据,必须通过Action ,而不能使用Mutation,但是在Action中还是要通过触发Mutation 的方式间接 变更数据。 3.在actions 中,不能直接修改 state 中的数据; 4.必须通过context. commit() 触发某个mutation函数 才行
actions: {
addAsync (context) {
setTimeout(() => {
context.commit('addd')
}, 1000)
}
}
5.这里的addAsync 就是在actions 里面定义的addAsync函数
export default {
data() {
return {};
},
methods: {
asyncAdd() {
this.$store.dispatch("addAsync");
},
},
};
触发action异步任务带参数的时候
<h3>当前的count值为:{{ $store.state.count }}</h3>
<button @click="asyncAddN">异步+N</button>
1.携带的参数就是step
mutations: {
addN (state, step) {
state.count += step
}
}
actions: {
addNAsync (context, step) {
setTimeout(() => {
context.commit('addN', step)
}, 1000)
}
},
数字5通过asyncAddN 方法传递到actions的addNAsync 方法里,然后通过commit 去访问mutations 里面的方法addN(){} ,从而传递给参数给addN给step
asyncAddN () {
this.$store.dispatch('addNAsync', 5)
}
方法二
触发action异步任务没有携带参数的时候
从vuex中导入mapMutations函数
import {mapActions } from 'vuex'
通过刚才导入的mapActions函数,将需要的actions函数,映射为当前组件的methods方法
...mapActions(['jianfaAsync']),
asyncSub () {
this.jianfaAsync()
}
jianfaa (state) {
state.count--
}
actions: {
jianfaAsync (context) {
setTimeout(() => {
context.commit('jianfaa')
}, 1000)
}
},
触发action异步任务带参数的时候
jianfaN (state, step) {
state.count -= step
}
jianfaNAsync (context, step) {
setTimeout(() => {
context.commit('jianfaN', step)
}, 1000)
}
...mapActions(['jianfaAsync', 'jianfaNAsync']),
asyncNSub () {
this.jianfaNAsync(5)
}
<h3>当前的count值为: {{count}}</h3>
<button @click="asyncNSub">异步-N</button>
整体效果展示
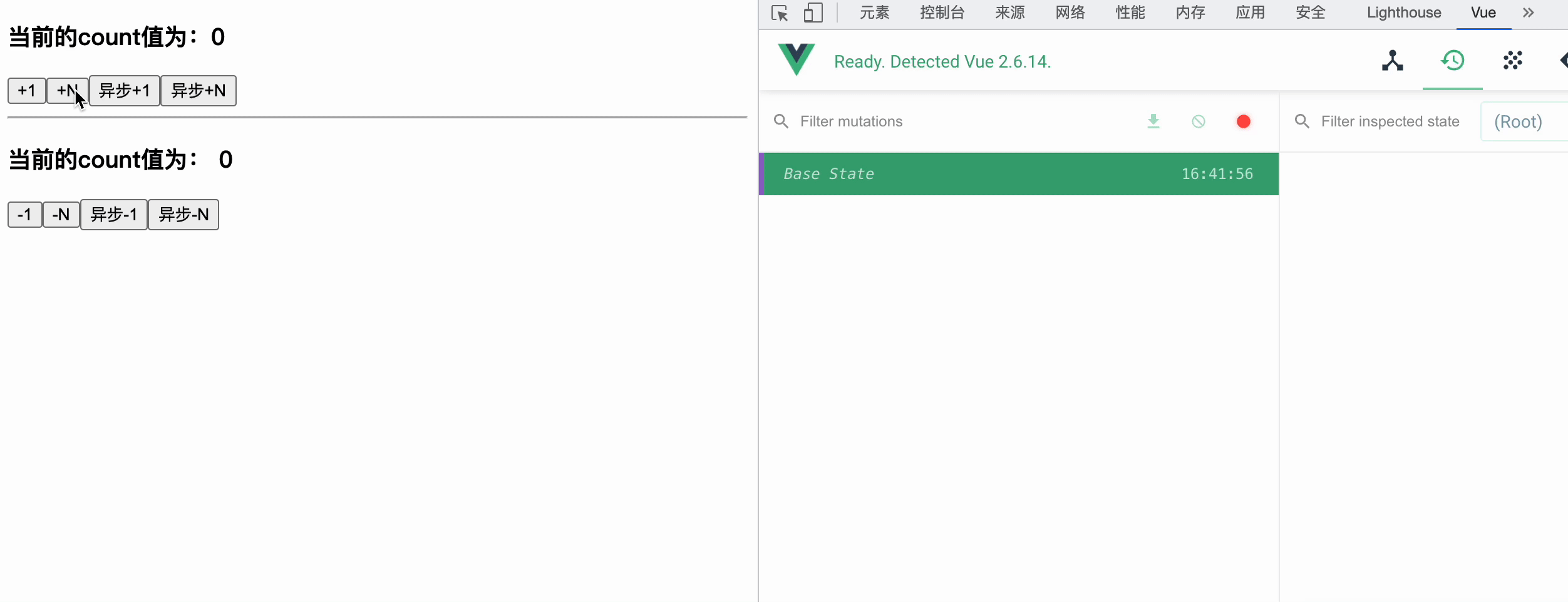
🌈🌈🌈🌈🌈🌈🌈🌈🌈完整代码
store/index.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
count: 0
},
mutations: {
addN (state, step) {
state.count += step
},
addd (state) {
state.count += 1
},
jianfaa (state) {
state.count--
},
jianfaN (state, step) {
state.count -= step
}
},
actions: {
addAsync (context) {
setTimeout(() => {
context.commit('addd')
}, 1000)
},
addNAsync (context, step) {
setTimeout(() => {
context.commit('addN', step)
}, 1000)
},
jianfaAsync (context) {
setTimeout(() => {
context.commit('jianfaa')
}, 1000)
},
jianfaNAsync (context, step) {
setTimeout(() => {
context.commit('jianfaN', step)
}, 1000)
}
},
modules: {
}
})
Add.vue
<template>
<div>
<h3>当前的count值为:{{ $store.state.count }}</h3>
<button @click="add1">+1</button>
<button @click="addition">+N</button>
<button @click="asyncAdd">异步+1</button>
<button @click="asyncAddN">异步+N</button>
</div>
</template>
<script>
export default {
data () {
return {}
},
methods: {
add1 () {
this.$store.commit('addd')
},
addition () {
console.log(this)
this.$store.commit('addN', 3)
},
asyncAdd () {
this.$store.dispatch('addAsync')
},
asyncAddN () {
this.$store.dispatch('addNAsync', 5)
}
}
}
</script>
<style>
</style>
Remove.vue
<template>
<div>
<h3>当前的count值为: {{count}}</h3>
<button @click="jianfa1">-1</button>
<button @click="reMoveN">-N</button>
<button @click="asyncSub">异步-1</button>
<button @click="asyncNSub">异步-N</button>
</div>
</template>
<script>
import { mapState, mapMutations, mapActions } from 'vuex'
export default {
data () {
return {}
},
computed: {
...mapState(['count'])
},
methods: {
...mapMutations(['jianfaa', 'jianfaN']),
...mapActions(['jianfaAsync', 'jianfaNAsync']),
jianfa1 () {
this.jianfaa()
},
reMoveN () {
this.jianfaN(2)
},
asyncSub () {
this.jianfaAsync()
},
asyncNSub () {
this.jianfaNAsync(5)
}
}
}
</script>
<style>
</style>
App.vue
<template>
<div>
<Add></Add>
<hr>
<Remove></Remove>
</div>
</template>
<script>
import Add from '@/components/Add.vue'
import Remove from '@/components/Remove.vue'
export default {
data () {
return {}
},
components: {
Add,
Remove
}
}
</script>
<style>
</style>
|