01-jquery动画
名称 | 用法 | 描述 |
---|
show() | $(‘div’).show(动画时间,动画完成回调) | 展示动画,主要修改元素 宽高 + display为block | hide() | $(‘div’).hide(动画时间,动画完成回调) | 隐藏动画,主要修改元素 宽高 + display为none | slideDown() | $(‘div’).slideDown(动画时间,动画完成回调) | 滑入动画(卷帘门效果),主要修改元素 高度+ display为block | slideUp() | $(‘div’).slideUp(动画时间,动画完成回调) | 滑出动画(卷帘门效果),主要修改元素 高度+ display为none | fadeIn() | $(‘div’).fadeIn(动画时间,,动画完成回调) | 淡入动画(渐变效果),主要修改元素 透明度1+ display为block | fadeOut() | $(‘div’).fadeOut(动画时间,,动画完成回调) | 淡出动画(渐变效果),主要修改元素 透明度0+ display为block | fadeTo() | $(‘div’).fadeTo(动画时间,目标透明度 ,动画完成回调) | 淡入动画(渐变效果),主要修改元素 透明度+ display为block | animate() | $(‘div’).animate(属性对象,动画时间,动画速度,回调函数) | 相当于webApi中封装的缓动动画animationSlow(),只是参数不同而已 |
1.1-显示show()与隐藏hide()
[效果预览](file:///C:/Users/张晓坤/Desktop/张晓坤前端备课资料/AB模式/05-jQuery/课程资料/备课代码/day03/02-jquery动画/01-显示与隐藏.html)
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
div {
width: 200px;
height: 200px;
background-color: red;
display: none;
}
</style>
</head>
<body>
<input type="button" value="显示" id="show" />
<input type="button" value="隐藏" id="hide" />
<input type="button" value="切换" id="toggle" /> <br /><br />
<div id="div1"></div>
<br /><br />
<div id="div2"></div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('#show').click(function () {
$('div').show('');
});
$('#hide').on('click', function () {
$('div').hide('');
});
$('#toggle').on('click', function () {
$('div').toggle(1000);
});
});
</script>
</body>
</html>
1.2-滑入slideDown()与滑出slideUp()
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
div {
width: 200px;
height: 200px;
background-color: red;
display: none;
}
</style>
</head>
<body>
<input type="button" value="滑入" id="slideDown" />
<input type="button" value="滑出" id="slideUp" />
<input type="button" value="切换" id="slideToggle" /><br /><br />
<div id="div1"></div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('#slideDown').on('click', function () {
$('div').slideDown();
$('div').slideDown('',function(){
alert('666');
});
});
$('#slideUp').on('click', function () {
$('#div1').slideUp(1000);
});
$('#slideToggle').on('click', function () {
$('#div1').slideToggle(1000);
});
});
</script>
</body>
</html>
1.3-淡入fadeIn()与淡出fadeOut()
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
div {
width: 200px;
height: 200px;
background-color: red;
display: none;
}
</style>
</head>
<body>
<input type="button" value="淡入" id="fadeIn" />
<input type="button" value="淡出" id="fadeOut" />
<input type="button" value="切换" id="fadeToggle" />
<input type="button" value="淡入到指定透明度" id="fadeTo" /><br /><br />
<div id="div1"></div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('#fadeIn').on('click', function () {
$('div').fadeIn(2000,function(){
alert('淡淡的我来了');
});
});
$('#fadeOut').on('click', function () {
$('#div1').fadeOut(function(){
alert('正如我淡淡的走');
});
});
$('#fadeToggle').on('click', function () {
$('#div1').fadeToggle(1000);
});
$('#fadeTo').on('click', function () {
$('#div1').fadeTo('',0.5, function () {
alert('我完事了');
});
});
});
</script>
</body>
</html>
1.4-自定义动画animate
[效果预览](file:///C:/Users/张晓坤/Desktop/张晓坤前端备课资料/AB模式/05-jQuery/课程资料/备课代码/day03/02-jquery动画/04-自定义动画animate.html)
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
div {
width: 100px;
height: 100px;
background-color: red;
position: absolute;
left: 0px;
}
#div1 {
top: 50px;
}
#div2 {
left: 300px;
top: 200px;
background-color: green;
}
</style>
</head>
<body>
<input type="button" value="从左到右800" id="lr800" />
<div id="div1"></div>
<div id="div2"></div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$("#lr800").on('click', function () {
$('#div1').animate({
left: 300,
top: 200,
width: 500,
height: 300,
zIndex: 1,
opacity: 0.5,
}, function () {
$('#div1').animate(
{
left: 500,
top: 50,
width: 50,
height: 50,
opacity: 0.2
});
});
});
});
</script>
</body>
</html>
1.5-动画队列
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
div {
width: 200px;
height: 300px;
background-color: red;
display: none;
}
</style>
</head>
<body>
<input type="button" value="开始动画" id="start" />
<input type="button" value="停止动画" id="stop" /> <br /><br />
<div></div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('#start').on('click', function () {
$('div').slideDown(2000).slideUp(2000);
});
$('#stop').on('click', function () {
$('div').stop(true,false);
});
});
</script>
</body>
</html>
02-动画案例
1.1-360开机动画
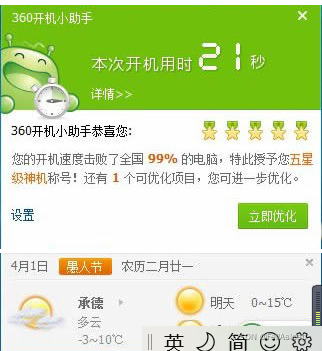
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
.box {
width: 322px;
position: fixed;
bottom: 0;
right: 0;
overflow: hidden;
}
span {
position: absolute;
top: 0;
right: 0;
width: 30px;
height: 20px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="box" id="box">
<span id="closeButton"></span>
<div class="hd" id="headPart">
<img src="images/t.jpg" alt="" />
</div>
<div class="bd" id="bottomPart">
<img src="images/b.jpg" alt="" />
</div>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('#closeButton').click(function () {
$('#bottomPart').animate({
height: 0
}, function () {
$('#box').animate({
width: 0
});
});
});
});
</script>
</body>
</html>
1.2-京东呼吸轮播图(渐变轮播图)
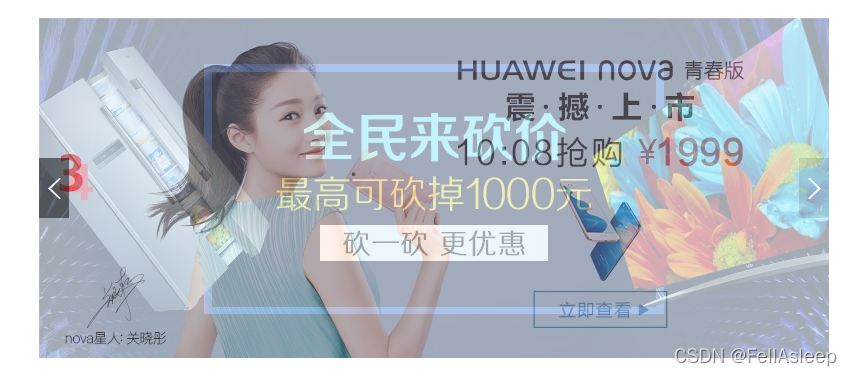
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<title>动画-案例《轮播图》</title>
<style>
* {
margin: 0;
padding: 0;
list-style: none;
}
.slider {
height: 340px;
width: 790px;
margin: 100px auto;
position: relative;
}
.slider li {
position: absolute;
display: none;
}
.slider li:first-child {
display: block;
}
.arrow {
display: none;
}
.slider:hover .arrow {
display: block;
}
.arrow-left,
.arrow-right {
font-family: "SimSun", "宋体";
width: 30px;
height: 60px;
background-color: rgba(0, 0, 0, 0.1);
position: absolute;
top: 50%;
margin-top: -30px;
cursor: pointer;
text-align: center;
line-height: 60px;
color: #fff;
font-weight: 700;
font-size: 30px;
}
.arrow-left:hover,
.arrow-right:hover {
background-color: rgba(0, 0, 0, .5);
}
.arrow-left {
left: 0;
}
.arrow-right {
right: 0;
}
</style>
</head>
<body>
<div class="slider">
<ul>
<li><a href="#"><img src="images/jd/1.jpg" alt=""></a></li>
<li><a href="#"><img src="images/jd/2.jpg" alt=""></a></li>
<li><a href="#"><img src="images/jd/3.jpg" alt=""></a></li>
<li><a href="#"><img src="images/jd/4.jpg" alt=""></a></li>
<li><a href="#"><img src="images/jd/5.jpg" alt=""></a></li>
<li><a href="#"><img src="images/jd/6.jpg" alt=""></a></li>
</ul>
<div class="arrow">
<span class="arrow-left"><</span>
<span class="arrow-right">></span>
</div>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
let index = 0;
$('.arrow-right').click(function () {
index == $('ul').children().length - 1 ? index = 0 : index++;
$('.slider li').eq(index).fadeIn(500).siblings('li').fadeOut(500);
})
$('.arrow-left').click(function () {
index == 0 ? index = $('ul').children().length - 1 : index--;
$('.slider li').eq(index).fadeIn(500).siblings('li').fadeOut(500);
});
});
</script>
</body>
</html>
1.3-手风琴
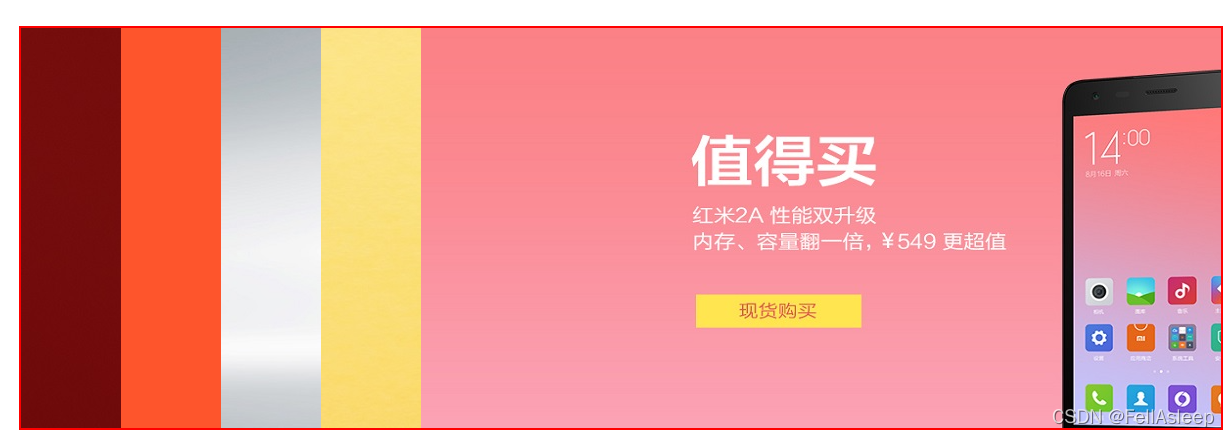
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>动画-案例《手风琴》</title>
<style>
* {
margin: 0;
padding: 0;
}
ul {
list-style: none;
width: 2400px;
}
#box {
width: 1200px;
height: 400px;
border: 2px solid red;
margin: 100px auto;
overflow: hidden;
}
#box li {
width: 240px;
height: 400px;
float: left;
}
</style>
</head>
<body>
<div id="box">
<ul>
<li><img src="./images/collapse/1.jpg" alt=""></li>
<li><img src="./images/collapse/2.jpg" alt=""></li>
<li><img src="./images/collapse/3.jpg" alt=""></li>
<li><img src="./images/collapse/4.jpg" alt=""></li>
<li><img src="./images/collapse/5.jpg" alt=""></li>
</ul>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('#box li').mouseenter(function () {
$(this).stop(true, false).animate({ width: 800 }, 500).siblings('li').stop(true, false).animate({ width: 100 }, 500);
});
$('#box').mouseleave(function () {
$(this).find('li').stop(true, false).animate({ width: 240 }, 200);
});
});
</script>
</body>
</html>
1.4-下拉菜单
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style type="text/css">
* {
margin: 0;
padding: 0;
}
ul {
list-style: none;
}
.wrap {
width: 330px;
height: 30px;
margin: 100px auto 0;
padding-left: 10px;
background-image: url(images/bg.jpg);
}
.wrap li {
background-image: url(images/libg.jpg);
}
.wrap>ul>li {
float: left;
margin-right: 10px;
position: relative;
}
.wrap a {
display: block;
height: 30px;
width: 100px;
text-decoration: none;
color: #000;
line-height: 30px;
text-align: center;
}
.wrap li ul {
position: absolute;
top: 30px;
display: none;
}
</style>
</head>
<body>
<div class="wrap">
<ul>
<li>
<a href="javascript:void(0);">一级菜单1</a>
<ul>
<li><a href="javascript:void(0);">二级菜单1</a></li>
<li><a href="javascript:void(0);">二级菜单2</a></li>
<li><a href="javascript:void(0);">二级菜单3</a></li>
</ul>
</li>
<li>
<a href="javascript:void(0);">一级菜单1</a>
<ul>
<li><a href="javascript:void(0);">二级菜单1</a></li>
<li><a href="javascript:void(0);">二级菜单2</a></li>
<li><a href="javascript:void(0);">二级菜单3</a></li>
</ul>
</li>
<li>
<a href="javascript:void(0);">一级菜单1</a>
<ul>
<li><a href="javascript:void(0);">二级菜单1</a></li>
<li><a href="javascript:void(0);">二级菜单2</a></li>
<li><a href="javascript:void(0);">二级菜单3</a></li>
</ul>
</li>
</ul>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('.wrap>ul>li').mouseenter(function () {
$(this).children('ul').stop(true,false).slideDown();
});
$('.wrap>ul>li').mouseleave(function () {
$(this).children('ul').stop(true,false).slideUp();
});
});
</script>
</body>
</html>
1.5-旋转木马
1.3-手风琴
[效果预览](file:///C:/Users/张晓坤/Desktop/张晓坤前端备课资料/AB模式/05-jQuery/课程资料/备课代码/day03/03-动画案例/03-手风琴.html)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>动画-案例《手风琴》</title>
<style>
* {
margin: 0;
padding: 0;
}
ul {
list-style: none;
width: 2400px;
}
#box {
width: 1200px;
height: 400px;
border: 2px solid red;
margin: 100px auto;
overflow: hidden;
}
#box li {
width: 240px;
height: 400px;
float: left;
}
</style>
</head>
<body>
<div id="box">
<ul>
<li><img src="./images/collapse/1.jpg" alt=""></li>
<li><img src="./images/collapse/2.jpg" alt=""></li>
<li><img src="./images/collapse/3.jpg" alt=""></li>
<li><img src="./images/collapse/4.jpg" alt=""></li>
<li><img src="./images/collapse/5.jpg" alt=""></li>
</ul>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('#box li').mouseenter(function () {
$(this).stop(true, false).animate({ width: 800 }, 500).siblings('li').stop(true, false).animate({ width: 100 }, 500);
});
$('#box').mouseleave(function () {
$(this).find('li').stop(true, false).animate({ width: 240 }, 200);
});
});
</script>
</body>
</html>
1.4-下拉菜单
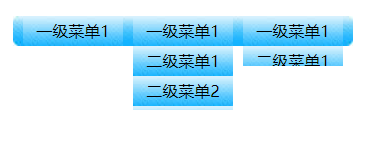
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style type="text/css">
* {
margin: 0;
padding: 0;
}
ul {
list-style: none;
}
.wrap {
width: 330px;
height: 30px;
margin: 100px auto 0;
padding-left: 10px;
background-image: url(images/bg.jpg);
}
.wrap li {
background-image: url(images/libg.jpg);
}
.wrap>ul>li {
float: left;
margin-right: 10px;
position: relative;
}
.wrap a {
display: block;
height: 30px;
width: 100px;
text-decoration: none;
color: #000;
line-height: 30px;
text-align: center;
}
.wrap li ul {
position: absolute;
top: 30px;
display: none;
}
</style>
</head>
<body>
<div class="wrap">
<ul>
<li>
<a href="javascript:void(0);">一级菜单1</a>
<ul>
<li><a href="javascript:void(0);">二级菜单1</a></li>
<li><a href="javascript:void(0);">二级菜单2</a></li>
<li><a href="javascript:void(0);">二级菜单3</a></li>
</ul>
</li>
<li>
<a href="javascript:void(0);">一级菜单1</a>
<ul>
<li><a href="javascript:void(0);">二级菜单1</a></li>
<li><a href="javascript:void(0);">二级菜单2</a></li>
<li><a href="javascript:void(0);">二级菜单3</a></li>
</ul>
</li>
<li>
<a href="javascript:void(0);">一级菜单1</a>
<ul>
<li><a href="javascript:void(0);">二级菜单1</a></li>
<li><a href="javascript:void(0);">二级菜单2</a></li>
<li><a href="javascript:void(0);">二级菜单3</a></li>
</ul>
</li>
</ul>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$('.wrap>ul>li').mouseenter(function () {
$(this).children('ul').stop(true,false).slideDown();
});
$('.wrap>ul>li').mouseleave(function () {
$(this).children('ul').stop(true,false).slideUp();
});
});
</script>
</body>
</html>
1.5-旋转木马
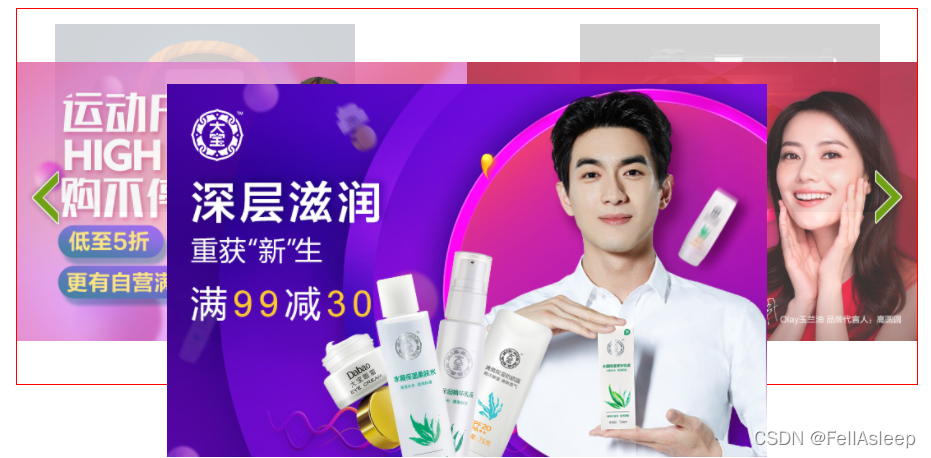
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>旋转木马轮播图</title>
<style>
blockquote,
body,
button,
dd,
dl,
dt,
fieldset,
form,
h1,
h2,
h3,
h4,
h5,
h6,
hr,
input,
legend,
li,
ol,
p,
pre,
td,
textarea,
th,
ul {
margin: 0;
padding: 0
}
body,
button,
input,
select,
textarea {
font: 12px/1.5 "Microsoft YaHei", "微软雅黑", SimSun, "宋体", sans-serif;
color: #666;
}
ol,
ul {
list-style: none
}
a {
text-decoration: none
}
fieldset,
img {
border: 0;
vertical-align: top;
}
a,
input,
button,
select,
textarea {
outline: none;
}
a,
button {
cursor: pointer;
}
.wrap {
width: 1200px;
margin: 100px auto;
border: 1px solid red;
}
.wrap:hover .arrow{
display: block;
}
.slide {
height: 500px;
position: relative;
}
.slide li {
position: absolute;
left: 200px;
top: 0;
}
.slide li img {
width: 100%;
}
.arrow {
display: none;
}
.prev,
.next {
width: 76px;
height: 112px;
position: absolute;
top: 50%;
margin-top: -56px;
background: url(images/rotate/prev.png) no-repeat;
z-index: 99;
}
.next {
right: 0;
background-image: url(images/rotate/next.png);
}
.slide .one {
width: 400px;
top: 20px;
left: 50px;
opacity: 0.2;
z-index: 2
}
.slide .two {
width: 600px;
top: 70px;
left: 0px;
opacity: 0.8;
z-index: 3
}
.slide .three {
width: 800px;
top: 100px;
left: 200px;
opacity: 1;
z-index: 4
}
.slide .four {
width: 600px;
top: 70px;
left: 600px;
opacity: 0.8;
z-index: 3
}
.slide .five {
width: 400px;
top: 20px;
left: 750px;
opacity: 0.2;
z-index: 2
}
</style>
</head>
<body>
<div class="wrap" id="wrap">
<div class="slide" id="slide">
<ul>
<li class="one"><a href="#"><img src="images/rotate/bingbing.jpg" alt="" /></a></li>
<li class="two"><a href="#"><img src="images/rotate/lingengxin.jpg" alt="" /></a></li>
<li class="three"><a href="#"><img src="images/rotate/yuanyuan.png" alt="" /></a></li>
<li class="four"><a href="#"><img src="images/rotate/slidepic4.jpg" alt="" /></a></li>
<li class="five"><a href="#"><img src="images/rotate/slidepic5.jpg" alt="" /></a></li>
</ul>
<div class="arrow" id="arrow">
<a href="javascript:;" class="prev" id="arrLeft"></a>
<a href="javascript:;" class="next" id="arrRight"></a>
</div>
</div>
</div>
<script src="./jquery-1.12.4.js"></script>
<script>
let config = [
{
"zIndex": 2,
"width": 400,
"top": 20,
"left": 50,
"opacity": 0.2,
},
{
"zIndex": 3,
"width": 600,
"top": 70,
"left": 0,
"opacity": 0.8,
},
{
"zIndex": 4,
"width": 800,
"top": 100,
"left": 200,
"opacity": 1,
},
{
"zIndex": 3,
"width": 600,
"top": 70,
"left": 600,
"opacity": 0.8,
},
{
"zIndex": 2,
"width": 400,
"top": 20,
"left": 750,
"opacity": 0.2,
}
];
console.log(config);
$('#arrRight').click(function () {
config.unshift(config.pop());
for (let i = 0; i < $('#slide li').length; i++) {
$('#slide li').eq(i).animate(config[i],1000);
};
});
$('#arrLeft').click(function () {
config.push(config.shift());
for (let i = 0; i < $('#slide li').length; i++) {
$('#slide li').eq(i).animate(config[i],1000);
};
});
</script>
</body>
</html>
1.6-手风琴动画
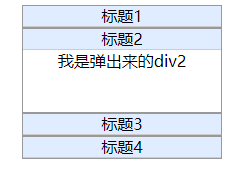
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style type="text/css">
* {
padding: 0;
margin: 0;
}
ul {
list-style-type: none;
}
.parentWrap {
width: 200px;
text-align: center;
margin: 50px auto auto;
}
.menuGroup {
border: 1px solid #999;
background-color: #e0ecff;
}
.groupTitle {
display: block;
height: 20px;
line-height: 20px;
font-size: 16px;
border-bottom: 1px solid #ccc;
cursor: pointer;
}
.menuGroup>div {
height: 200px;
background-color: #fff;
display: none;
}
</style>
</head>
<body>
<ul class="parentWrap">
<li class="menuGroup">
<span class="groupTitle">标题1</span>
<div>我是弹出来的div1</div>
</li>
<li class="menuGroup">
<span class="groupTitle">标题2</span>
<div>我是弹出来的div2</div>
</li>
<li class="menuGroup">
<span class="groupTitle">标题3</span>
<div>我是弹出来的div3</div>
</li>
<li class="menuGroup">
<span class="groupTitle">标题4</span>
<div>我是弹出来的div4</div>
</li>
</ul>
<script src="jquery-1.12.4.js"></script>
<script>
$('.menuGroup').on('click', function () {
if ($(this).children('div').css('display') == 'block') {
$(this).children('div').slideUp();
} else {
$(this).children('div').slideDown()
$(this).siblings().children('div').slideUp()
}
})
</script>
</body>
</html>
1.7-开窗动画
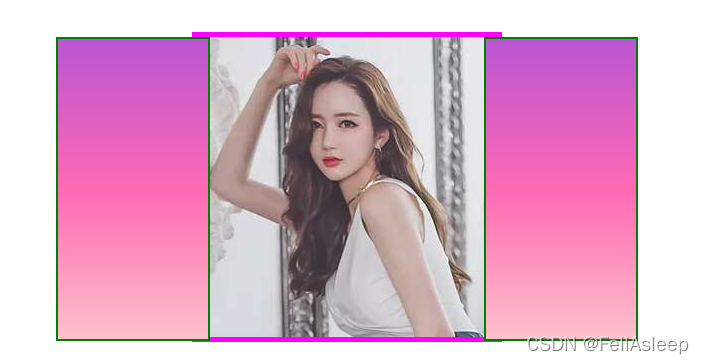
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
#box {
width: 300px;
height: 300px;
margin: 50px auto;
position: relative;
border: 5px solid magenta;
}
span {
position: absolute;
width: 50%;
height: 100%;
background: linear-gradient(0deg, pink, hotpink, mediumorchid);
top: 0;
border: 2px solid green;
}
.left {
left: 0;
}
.right {
right: 0;
}
</style>
</head>
<body>
<h2 style="text-align: center">来黑马,学编程,送女朋友</h2>
<div id="box">
<img src="images/mm1.jpg" alt="">
<span class="left"></span>
<span class="right"></span>
</div>
<script src="./jquery-1.12.4.js"></script>
<script>
let imgArr = ['images/mm1.jpg', 'images/mm2.jpg', 'images/mm3.jpg', 'images/mm4.jpg', 'images/mm5.jpg', 'images/mm6.jpg']
let index = 0;
$('#box').mouseenter(function () {
$('.left').stop(true,false).animate({ left: '-50%' }, 1000);
$('.right').stop(true,false).animate({ right: '-50%' }, 1000);
});
$('#box').mouseleave(function () {
$('.left').stop(true,false).animate({ left: '0' }, 1000);
$('.right').stop(true,false).animate({ right: '0' }, 1000, function () {
index++;
if (index > imgArr.length - 1) {
index = 0;
};
$('#box>img').attr('src', imgArr[index]);
});
});
</script>
</body>
</html>
1.8-九宫格抽奖
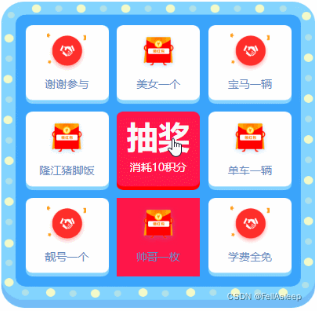
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
.lottery {
overflow: hidden;
padding: 20px;
width: 400px;
margin: 30px auto;
background-repeat: no-repeat;
background-size: 100% 100%;
background-image: url(images/bg1.png);
}
.lottery.active {
background-image: url(images/bg2.png);
}
.lottery .lottery-item {
height: 340px;
position: relative;
margin-top: 10px;
margin-left: 10px;
}
.lottery .lottery-item .lottery-start {
position: absolute;
left: 33.33333333%;
width: 33.33333333%;
top: 110px;
padding-right: 10px;
}
.lottery .lottery-item .lottery-start.active {
opacity: 0.7;
}
.lottery .lottery-item .lottery-start .box {
height: 100px;
font-size: 14px;
color: #fff;
cursor: pointer;
text-align: center;
overflow: hidden;
background: url(images/red.png) no-repeat center;
background-size: 100% 100%;
}
.lottery .lottery-item .lottery-start .box p b {
font-size: 40px;
margin-top: 16px;
margin-bottom: 15px;
line-height: 30px;
display: block;
}
.lottery .lottery-item ul li {
list-style: none;
width: 33.33333333%;
position: absolute;
padding-right: 10px;
}
.lottery .lottery-item ul li:nth-child(2) {
left: 33.33333333%;
}
.lottery .lottery-item ul li:nth-child(3) {
left: 66.66666666%;
}
.lottery .lottery-item ul li:nth-child(4) {
left: 66.66666666%;
top: 110px;
}
.lottery .lottery-item ul li:nth-child(5) {
left: 66.66666666%;
top: 220px;
}
.lottery .lottery-item ul li:nth-child(6) {
left: 33.33333333%;
top: 220px;
}
.lottery .lottery-item ul li:nth-child(7) {
left: 0;
top: 220px;
}
.lottery .lottery-item ul li:nth-child(8) {
left: 0;
top: 110px;
}
.lottery .lottery-item ul li .box {
height: 100px;
position: relative;
text-align: center;
overflow: hidden;
background: url(images/white.png) no-repeat center;
background-size: 100% 100%;
}
.lottery .lottery-item ul li.active .box {
background: url(images/red.png) no-repeat center;
}
.lottery .lottery-item ul li .box p {
color: #708ABF;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
font-size: 14px;
}
.lottery .lottery-item ul li .box img {
display: block;
height: 50px;
margin: 0 auto;
margin-top: 10px;
margin-bottom: 5px;
}
</style>
</head>
<body>
<div class="lottery">
<div class="lottery-item">
<div class="lottery-start">
<!---->
<div class="box">
<p><b>抽奖</b></p>
<p>消耗10积分</p>
</div>
<!---->
</div>
<ul>
<li class="">
<div class="box">
<p><img src="images/j1.png" alt=""></p>
<p>谢谢参与</p>
</div>
</li>
<li class="">
<div class="box">
<p><img src="images/j2.png" alt=""></p>
<p>美女一个</p>
</div>
</li>
<li class="">
<div class="box">
<p><img src="images/j1.png" alt=""></p>
<p>宝马一辆</p>
</div>
</li>
<li class="">
<div class="box">
<p><img src="images/j2.png" alt=""></p>
<p>单车一辆</p>
</div>
</li>
<li class="on">
<div class="box">
<p><img src="images/j1.png" alt=""></p>
<p>学费全免</p>
</div>
</li>
<li class="">
<div class="box">
<p><img src="images/j2.png" alt=""></p>
<p>帅哥一枚</p>
</div>
</li>
<li class="">
<div class="box">
<p><img src="images/j1.png" alt=""></p>
<p>靓号一个</p>
</div>
</li>
<li class="">
<div class="box">
<p><img src="images/j2.png" alt=""></p>
<p>隆江猪脚饭</p>
</div>
</li>
</ul>
</div>
</div>
<script src="./jquery-1.12.4.js"></script>
<script>
$('.lottery-start').on('click', function () {
setInterval(function () {
$('.lottery').toggleClass('active')
}, 500)
let timeID = setInterval(function () {
let randomIndex = parseInt(Math.random() * $('li').length)
$('li').eq(randomIndex).addClass('active')
$('li').eq(randomIndex).siblings().removeClass('active')
}, 300)
setTimeout(function () {
alert($('li.active p').text())
clearInterval(timeID)
}, 5000)
})
</script>
<!-- <script>
setInterval(function () {
$('.lottery').toggleClass('active');
}, 500);
$('.lottery-start').mousedown(function () {
$(this).addClass('active');
});
let index = 0;
let timeID = null;
$('.lottery-start').mouseup(function () {
$(this).removeClass('active');
clearInterval(timeID);
index = 0;
let timeout = Math.random() * 3 * 1000;
timeID = setInterval(function () {
lotteryAnimal();
}, 600);
setTimeout(function () {
clearInterval(timeID);
timeID = setInterval(function () {
lotteryAnimal();
}, 100);
}, timeout);
setTimeout(function () {
clearInterval(timeID);
timeID = setInterval(function () {
lotteryAnimal();
}, 50);
}, timeout * 2);
setTimeout(function () {
clearInterval(timeID);
timeID = setInterval(function () {
lotteryAnimal();
}, 400);
}, timeout * 3);
setTimeout(function () {
clearInterval(timeID);
timeID = setInterval(function () {
lotteryAnimal();
}, 800);
}, timeout * 4);
setTimeout(function () {
clearInterval(timeID);
alert($('ul>li').eq(index).text().trim());
}, timeout * 5);
});
function lotteryAnimal() {
index++;
if (index > $('ul>li').length - 1) {
index = 0;
};
$('ul>li').eq(index).addClass('active').siblings().removeClass('active');
}
</script> -->
</body>
</html>
03-jquery三大家族操作(尺寸操作)
名称 | 用法 | 描述 |
---|
outerWidth() / outerHieght() | $(‘div’).outerWidth() | 获取 width + padding· + border (相当于原生的offsetWidth/Height) | width() / height() | $(‘div’).width() | 获取 width | innerWidth()/innerHeight() | $(‘div’).innerWidth() | 获取 wdith + padding | outerWidth(true)/outerHeight(true) | $(‘div’).outerWidth(true) | 获取 width + padding· + border + margin | position() | $(‘div’).position() | 对象类型,自身左外边框 到 定位父级 左内边框距离(相当于原生的offsetLeft/Top) | offset() | $(‘div’).offset() | 对象类型, 自身左外边框 距离 页面 左内边框距离 | scrollLeft() / scrollTop() | $(‘div’).scrollLeft() | 与原生的scrollLeft/Top作用一样.支持修改 |
1.1-宽高尺寸
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
</body>
</html>
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
* {
margin: 0;
padding: 0;
}
.one {
width: 200px;
border: 10px solid red;
padding: 20px;
margin: 30px;
}
</style>
</head>
<body>
<div id="one" class="one" style="height: 200px;"></div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
console.log($('#one').css('width'));
console.log($('#one').css('padding'));
console.log($('#one').css('border'));
console.log($('#one').css('border-left-width'));
console.log($('#one').outerWidth());
console.log($('#one').outerHeight());
$('#one').outerWidth(300);
console.log($('#one').width());
console.log($('#one').height());
$('#one').height(100);
console.log($('#one').innerWidth());
console.log($('#one').innerHeight());
$('#one').innerWidth(100);
console.log($('#one').outerWidth(true));
console.log($('#one').outerHeight(true));
});
</script>
</body>
</html>
1.2-position与offset
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
* {
margin: 0;
padding: 0;
}
.father {
width: 400px;
height: 400px;
border: 10px solid red;
position: relative;
top: 10px;
left: 10px;
}
.son {
width: 100px;
height: 100px;
border: 10px solid green;
position: absolute;
top: 100px;
left: 100px;
}
</style>
</head>
<body>
<div id="father" class="father">
<div id="son" class="son"></div>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
console.log($('#son').position());
console.log($('#son').position().left);
console.log($('#son').position().top);
$('#son').position({
left:200,
top:200
});
console.log($('#son').offset());
console.log($('#son').offset().left);
console.log($('#son').offset().top);
$('#son').offset({
left:150,
top:150
});
});
</script>
</body>
</html>
1.3-获取可视区域宽高
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script src="./jquery-1.12.4.js"></script>
<script>
console.log( $(window).width());
console.log( $(window).height());
$(window).resize(function(){
if($(window).width() >= 1000){
$('body').css('backgroundColor','red');
}else if($(window).width() >= 600){
$('body').css('backgroundColor','yellow');
}else{
$('body').css('backgroundColor','green');
}
})
</script>
</body>
</html>
1.4-scrollLeft()与scrollTop()
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>标题</title>
<style>
* {
margin: 0;
padding: 0;
}
body {
width: 2000px;
height: 2000px;
}
div {
width: 200px;
height: 200px;
border: 1px solid red;
overflow: auto;
}
img {
vertical-align: top;
}
</style>
</head>
<body>
<div>
<img src="./images/1.jpg" alt="" />
</div>
<script src="./jquery-1.12.4.js"></script>
<script>
$(function () {
$('div').scroll(function(){
console.log($(this).scrollLeft());
console.log($(this).scrollTop());
});
$(window).scroll(function(){
console.log($(this).scrollLeft());
console.log($(this).scrollTop());
});
});
</script>
</body>
</html>
1.5-案例:固定导航
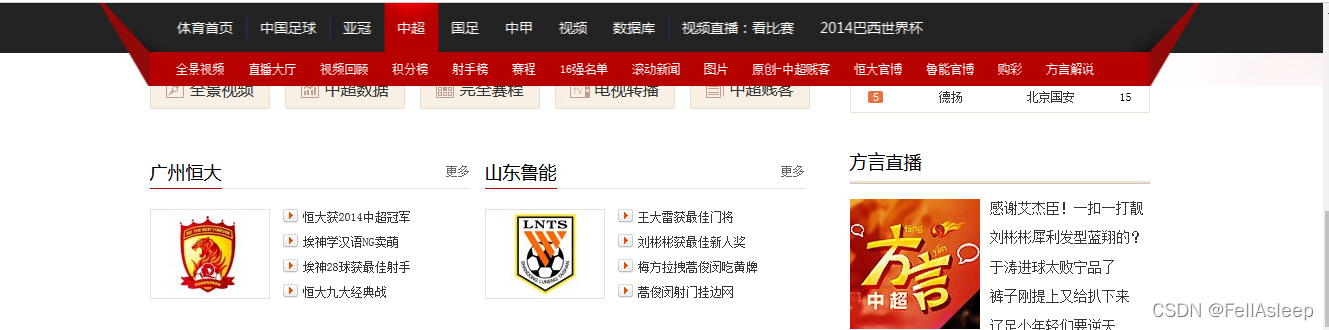
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>固定导航栏</title>
<style type="text/css">
* {
margin: 0;
padding: 0;
}
.top,
.nav {
width: 1423px;
margin-left: 50%;
transform: translate(-50%);
}
.main {
width: 1000px;
margin: 10px auto;
}
img {
display: block;
vertical-align: middle;
}
</style>
</head>
<body>
<div class="top">
<img src="images/top.png" />
</div>
<div class="nav">
<img src="images/nav.png" />
</div>
<div class="main">
<img src="images/main.png" />
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
$(window).on('scroll', function () {
if ($(this).scrollTop() > $('.top').height()) {
$('.nav').css({
position: 'fixed',
top: 0,
left: 0
});
$('.main').css({
marginTop: $('.nav').height() + 10 + 'px'
});
} else {
$('.nav').css({
position: 'static'
});
$('.main').css({
marginTop: '10px'
});
}
});
});
</script>
</body>
</html>
|