School代码
<template>
<div class="school">
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="sendSchoolName">把学校名给App</button>
</div>
</template>
<script>
export default {
name:'School',
props:['getSchoolName'],
data() {
return {
name:'UTM',
address:'柔佛',
}
},
methods:{
sendSchoolName(){
this.getSchoolName(this.name)
}
},
}
</script>
<style>
.school{
background-color: yellow;
}
</style>
Student代码:
<template>
<div class="student">
<h2>学生名称:{{name}}</h2>
<h2>学生性别:{{sex}}</h2>
<h2>当前求和为:{{number}}</h2>
<button @click="add">点我number++</button>
<button @click="sendStudentlName">把学生名给App</button>
<button @click="unbind">解绑atguigu事件</button>
<button @click="death">销毁了当前的Student组件的实例(vc)</button>
</div>
</template>
<script>
export default {
name:'Student',
data() {
return {
name:'张三',
sex:'男',
number:0
}
},
methods:{
add(){
console.log('add回调被调用了')
this.number++
},
sendStudentlName(){
//触发Student组件实例身上的atguigu事件
this.$emit('atguigu',this.name)
// this.$emit('demo')
},
unbind(){
this.$off('atguigu') //解绑一个自定义事件
// this.$off(['atguigu','demo']) //解绑多个自定义事件
this.$off() //解绑所有的自定义事件
},
death(){
this.$destroy() //销毁了当前的Student组件的实例,销毁后所有Student实例的自定义事件全都不奏效。
}
},
}
</script>
<style>
.student{
background-color: orangered;
}
</style>
App.vue代码:
<template>
<div class="app">
<h1>{{msg}}</h1>
<!-- 通过父组件给子组件传递函数类型的props实现:子给父传递数据 -->
<School :getSchoolName="getSchoolName"/>
<!-- 通过父组件给子组件绑定一个自定义事件实现:子给父传递数据(第一种写法,使用@或v-on) -->
<!-- <Student @atguigu.once="getStudentName"/> -->
<Student @atguigu="getStudentName" @demo="m1"/>
<!-- 通过父组件给子组件绑定一个自定义事件实现:子给父传递数据(第二种写法,使用ref) -->
<!-- <Student ref="student"/> -->
</div>
</template>
<script>
import Student from './components/Student.vue'
import School from './components/School.vue'
export default {
name: 'App',
components: {
School,Student
},
data(){
return {
msg:'你好'
}
},
methods:{
getSchoolName(name){
console.log('App收到了学校名称:',name)
},
getStudentName(name){
console.log('App收到了学生名字:',name)
},
m1(){
console.log('demo事件被触发了')
}
},
// demo(){
// console.log('demo被调用了')
// }
mounted(){
// this.$refs.student.$on('atguigu',this.getStudentName) //解绑自定义事件
},
}
</script>
<style>
.app{
background-color: gray;
}
</style>
main.js代码:
//引入Vue
import Vue from 'vue'
//引入App
import App from './App.vue'
//关闭Vue的生产提示
Vue.config.productionTip = false
//创建vm
new Vue({
el:'#app',
render: h => h(App)
})
效果: 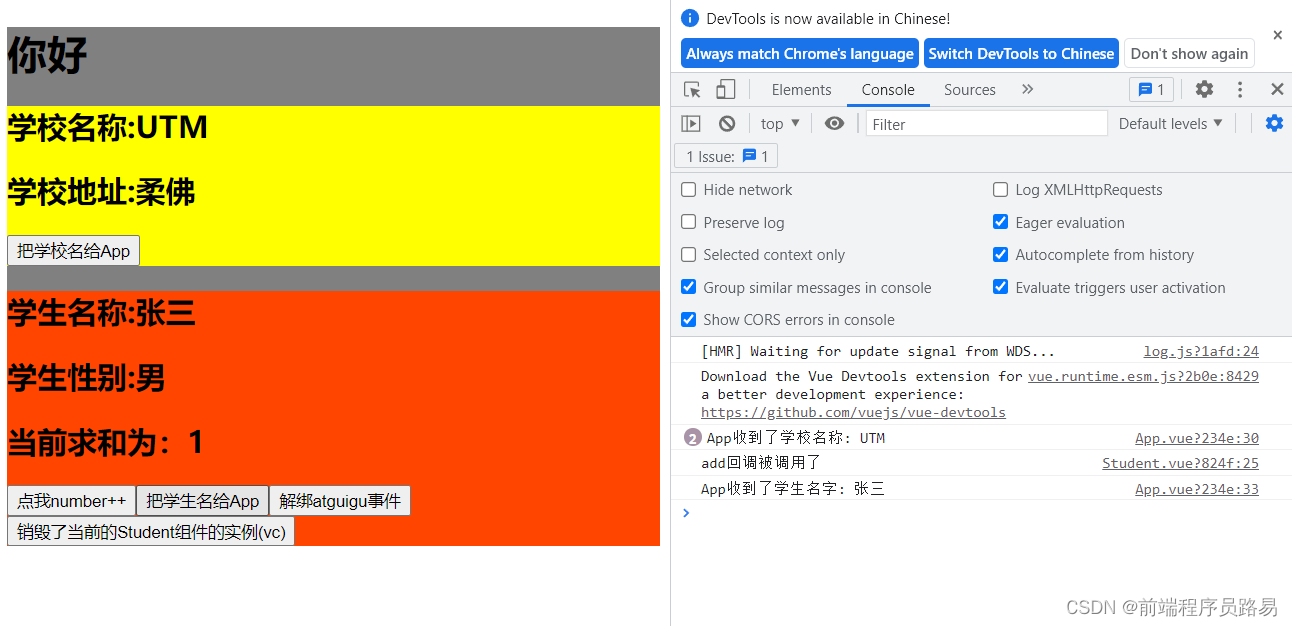 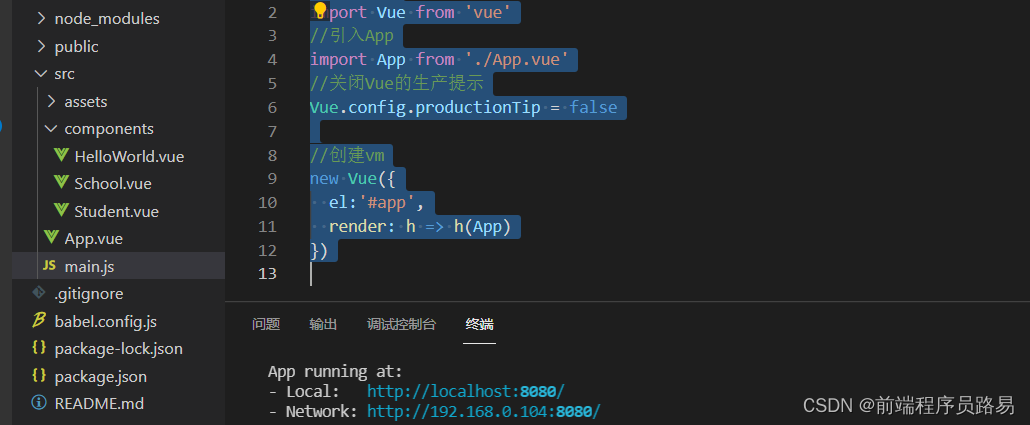
|