1、根据图片,完成页面布局,100%还原,圆角使用border-radius:3px;
2、实现文本框添加姓名、年龄,要求不能添加空值,表格中ID需要按照序号依次自动生成。
3、点击移入变色按钮开启变色,再点击一次,关闭变色
4、搜索具体的姓名,实现搜索功能,高亮显示结果
5、点击删除,删除整行数据,删除之后序号重新排序
6、附加题:点击排序按钮实现年龄从小到大排序
tips:参考效果:http://js.7vnet.com/javascript/js005/js009.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>周测,表格练习</title>
<style type="text/css">
.container{
width: 1000px;
margin: 0 auto;
font-size: 12px;
}
.content{
padding: 22px 22px 0px 22px;
border: 1px solid #ececec;
text-align: left;
border-bottom: 10px solid #e8f3ec;
margin-bottom: 78px;
}
input{
height: 32px;
width: 182px;
border-radius: 3px;
border: 1px solid #cccccc;
padding-left: 12px;
outline: none;
display: inline-block;
}
button{
padding: 9px 12px;
border: 1px solid #4ab9db;
background-color: #5bc0de;
color: #FFFFFF;
border-radius: 3px;
}
button:hover{
color: #fff;
background-color: #31b0d5;
border-color: #269abc;
}
.top{
margin-bottom: 22px;
}
table{
border: 1px solid #cccccc;
color: #4f6f7d;
border-left: none;
}
thead th{
border-bottom: 1px solid #cccccc;
}
th{
border-left: 1px solid #cccccc;
height: 33px;
padding-left: 10px;
}
.main td{
border-top: 1px solid #cccccc;
border-left: 1px solid #cccccc;
height: 33px;
padding-left: 10px;
}
tbody tr{
background: none;
}
.bottom{
margin-top: 22px;
margin-bottom: 27px;
}
.text{
margin-bottom: 25px;
color: #4f6f7d;
}
</style>
</head>
<body>
<pre>
1、根据图片,完成页面布局,100%还原,圆角使用border-radius:3px;
2、实现文本框添加姓名、年龄,要求不能添加空值,表格中ID需要按照序号依次自动生成。
3、点击移入变色按钮开启变色,再点击一次,关闭变色
4、搜索具体的姓名,实现搜索功能,高亮显示结果
5、点击删除,删除整行数据,删除之后序号不需要考虑重新排序
6、附加题:点击排序按钮实现年龄从小到大排序
tips:参考效果:http://js.7vnet.com/javascript/js005/js009.html
</pre>
<!-- 1.根据图片,完成页面布局,100%还原,圆角使用border-radius:3px -->
<div class="container">
<div class="content">
<!-- 顶部添加 -->
<div class="top">
<label>添加:</label>
<input id="name1" type="text" placeholder="姓名" value="" />
<input id="age" type="text" placeholder="年龄" value="" />
<button id="add" type="button">添加</button>
<label>变色:</label>
<button id="changecolor" type="button">开始移入变色</button>
</div>
<!-- 主要表格内容 -->
<div id="main" class="main">
<table cellspacing="0" >
<thead>
<tr>
<th width="175px" >ID</th>
<th width="335px">姓名</th>
<th width="257px">年龄</th>
<th width="258px">操作</th>
</tr>
</thead>
<tbody id="tbody">
<tr>
<td>1</td>
<td>张三</td>
<td>33</td>
<td><span>删除</span></td>
</tr>
<tr>
<td>2</td>
<td>李四</td>
<td>34</td>
<td><span>删除</span></td>
</tr>
<tr>
<td>3</td>
<td>王五</td>
<td>25</td>
<td><span>删除</span></td>
</tr>
<tr>
<td>4</td>
<td>赵六</td>
<td>16</td>
<td><span>删除</span></td>
</tr>
<tr>
<td>5</td>
<td>王四</td>
<td>44</td>
<td><span>删除</span></td>
</tr>
<tr>
<td>6</td>
<td>黄小米</td>
<td>23</td>
<td><span>删除</span></td>
</tr>
</tbody>
</table>
</div>
<!-- 底部搜索 -->
<div class="bottom">
<label>搜索:</label>
<input id="name2" type="text" placeholder="姓名" value="" />
<button id="search" type="button">搜索</button>
<label>排序:</label>
<button id="sort" type="button">年龄从小排序</button>
</div>
<div class="text">
数组里存的是所有tr,排序的时候按照第三个单元格的innerHTML大小排序。最后按照新的顺序重新添加所有tr。
</div>
</div>
</div>
<script type="text/javascript">
//查找元素
var oName1=document.getElementById('name1');
var oAge=document.getElementById('age');
var oAdd=document.getElementById('add');
var oChangecolor=document.getElementById('changecolor');
var oAge=document.getElementById('age');
var oName2=document.getElementById('name2');
var oSearch=document.getElementById('search');
var oSort=document.getElementById('sort');
var oTbody=document.getElementById('tbody');
// 2、实现文本框添加姓名、年龄,要求不能添加空值,表格中ID需要按照序号依次自动生成。
//绑定事件
oAdd.οnclick=function(){
//去除两头空格,判断输入框是否为空
var name1 = oName1.value.trim();
var age = oAge.value.trim();
var pwd=parseInt(age);
var isValid=isNaN(pwd);
if(!name1&&!age){
alert('请输入姓名、年龄来进行添加');
return;
}else if(!name1){
alert('请输入姓名');
return;
}else if(!age){
alert('年龄为空,请输入年龄');
return;
}else if(isValid){
alert('请输入正确的年龄');
oAge.value='';
return;
}
//利用tr行数进行ID排序——有几行就有几个ID
var oTr=document.querySelectorAll('tbody tr');
for (var i = 0; i <oTr.length; i++){
var num=oTbody.children[i].children[0].innerText;
num=i+1;
}
//判断完成,进行新元素创建
var oTr=document.createElement('tr');
num++;
//修饰内容
oTr.innerHTML='<td>'+num+'</td><td>'+name1+'</td><td>'+age+'</td><td><span>删除</span></td>';
//在tbody里插入tr
oTbody.appendChild(oTr);
}
// 3、点击移入变色按钮开启变色,再点击一次,关闭变色
oChangecolor.οnclick=function(){
var oTr=document.querySelectorAll('tbody tr');
if(oChangecolor.innerText=='开始移入变色'){
oChangecolor.innerText='关闭移入变色';
oChangecolor.style.background="red";
oChangecolor.style.borderColor="black";
for(var i = 0; i< oTr.length; i ++){
oTr[i].οnmοuseοver=function(){
this.style.background = "rgb(238,238,238)";
}
oTr[i].onmouseout = function() {
this.style.background = "none";
}
}
}else if(oChangecolor.innerText=='关闭移入变色'){
oChangecolor.innerText='开始移入变色'
oChangecolor.style.backgroundColor ="#31b0d5";
oChangecolor.style.borderColor="#269abc";
oChangecolor.οnmοuseοver=function(){
this.style.backgroundColor="#269abc" ;
this.style.borderColor="#31b0d5" ;
}
oChangecolor.οnmοuseοut=function(){
this.style.backgroundColor="#31b0d5" ;
this.style.borderColor="#269abc" ;
}
for(var i=0;i< oTr.length;i++){
oTr[i].οnmοuseοver=function(){
this.style.background ="none";
}
oTr[i].onmouseout = function() {
this.style.background = "none";
}
}
}
}
// 4、搜索具体的姓名,实现搜索功能,高亮显示结果
//绑定搜索事件
oSearch.οnclick=function(){
//去除两头空格,判断输入框是否为空
var name2 = oName2.value.trim();
if(!name2){
alert('姓名为空,请输入姓名来进行搜索操作!');
return;
}
console.log(name2);
var oTr=document.querySelectorAll('tbody tr');
var value=0;
for(var i=0 ; i<oTr.length ; i++){
console.log(oTr[i].innerText)
if(name2 == oTr[i].children[1].innerText){
value=1;
oTr[i].style.backgroundColor = "yellow";
oTr[i].οnmοuseοver=function(){
this.style.background = "none";
}
}
}
if(value==0){
alert('查无此人!');
}
}
//5、按年龄排序
oSort.οnclick=function(){
var oTr=document.querySelectorAll('tbody tr');
var math=[];
//强制转换为数字后赋值给math数组
for (var i = 0; i < oTr.length; i++) {
math[i]=parseInt(oTr[i].children[2].innerText);
console.log(math[i]);
}
//按小到大排序
for (var i = 0; i < math.length-1; i++) {
for (var j = 0; j < math.length - 1 - i; j++) {
// if ( math[j] > math[j + 1]) {
// var flag = math[j];
// math[j] = math[j + 1];
// math[j + 1] = flag;
// var temp=oTr[j].innerText;
// oTr[j].innerText = oTr[j+1].innerText;
// oTr[j+1].innerText=temp;
// }
var temp1=oTbody.children[j].children[2].innerText;
var temp2=oTbody.children[j+1].children[2].innerText;
if(temp1>temp2){
oTbody.children[j+1].children[2].innerText=temp1;
oTbody.children[j].children[2].innerText=temp2;
var temp=oTbody.children[j+1].children[1].innerText;
oTbody.children[j+1].children[1].innerText=oTbody.children[j].children[1].innerText
oTbody.children[j].children[1].innerText=temp;
}
}
}
}
//6、事件委托,进行删除操作
oTbody.οnclick=function(event){
//判断是否点击删除按钮
console.log(event.target.tagName);
if(event.target.tagName=="SPAN"){
event.target.parentElement.parentElement.remove();
}
//删除后重新排序
var oTr=document.querySelectorAll('tbody tr');
for (var i = 0; i <oTr.length; i++){
oTbody.children[i].children[0].innerText=i+1;
}
}
</script>
</body>
</html>
运行效果如图: 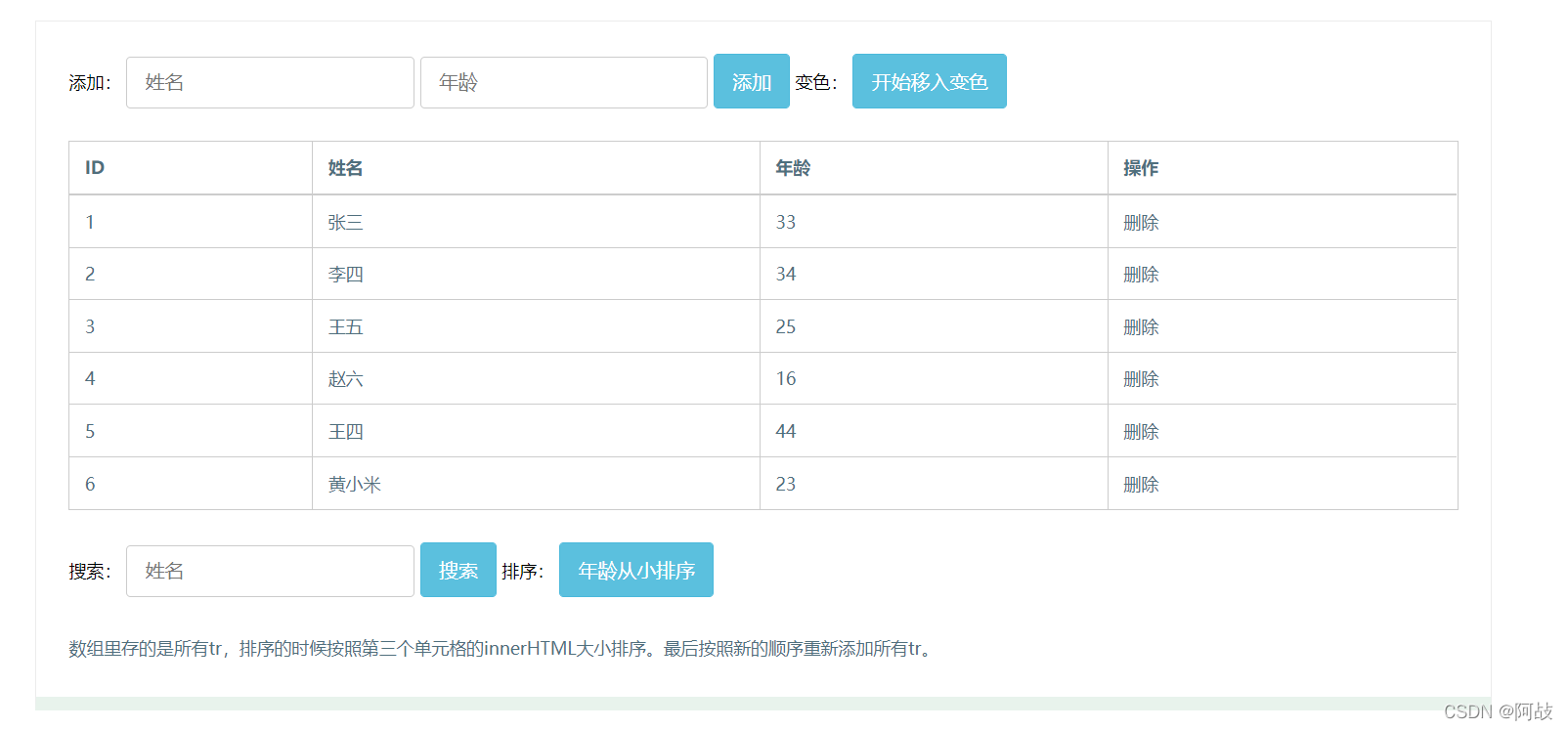
|