1.类
const int MAX_SIZE = 100;
template <class DataType>
class stack
{
private:
DataType* data;
int size;
int top;
public:
stack();
stack(int s);
~stack();
void push(DataType ch);
DataType pop();
DataType getTop();
bool isEmpty();
bool isFull();
void setNull();
class Full {};
class Empty {};
};
2.类外实现函数接口
1.无参构造函数
stack<DataType>::stack()
{
size = MAX_SIZE;
top = -1;
data = new DataType[MAX_SIZE];
}
2.有参构造函数
template <class DataType>
stack<DataType>::stack(int s) {
size = s;
top = -1;
data = new DataType[size];
}
3.//析构函数,释放堆区数据
template <class DataType>
stack<DataType>::~stack()
{
delete []data;
cout << "析构函数finished delete\n";
}
4.//入栈操作
template <class DataType>
void stack<DataType>::push(DataType ch) {
if (isFull())
throw stack<DataType>::Full();
else
data[++top] = ch;
}
5.//出栈操作
template <class DataType>
DataType stack<DataType>::pop() {
if (isEmpty())
throw stack<DataType>::Empty();
else
return data[top--];
}
6.//得到栈顶元素
template <class DataType>
DataType stack<DataType>::getTop()
{
if (!isEmpty())
return data[top];
}
7.//判断栈是否为空
template <class DataType>
bool stack<DataType>::isEmpty()
{
if (top == -1) {
return true;
}
else {
return false;
}
}
8.//判断栈是否满了
template <class DataType>
bool stack<DataType>::isFull() {
if (top == size - 1) {
return true;
}
else {
return false;
}
}
9.//设置栈为空!!!!
template <class DataType>
void stack<DataType>::setNull() {
top = -1;
cout << "top=-1!\n";
}
3.stack.cpp
#include "stack.hpp"
#include <iostream>
using namespace std;
int main() {
stack<char> st(2);
char ch;
try {
st.push('a');
st.push('b');
st.push('c');
}
catch (stack<char>::Full) {
cout << "stack full!\n";
}
try {
ch = st.pop();
cout << ch << endl;
ch = st.pop();
cout << ch << endl;
ch = st.pop();
cout << ch << endl;
}
catch (stack<char>::Empty)
{
cout << "stack empty!\n";
}
double c;
stack<double> sl(2);
try {
sl.push(1998.3);
sl.push(1999.9);
sl.push(2000.1);
}
catch (stack<double>::Full) {
cout << "stack full!\n";
}
try {
c = sl.pop();
cout << c << endl;
c = sl.pop();
cout << c << endl;
c = sl.pop();
cout << c << endl;
}
catch (stack<double>::Empty) {
cout << "stack empty!\n";
}
return 0;
}
4.总源码(分文件)
1.stack.hpp
#pragma once
const int MAX_SIZE = 100;
template <class DataType>
class stack
{
private:
DataType* data;
int size;
int top;
public:
stack();
stack(int s);
~stack();
void push(DataType ch);
DataType pop();
DataType getTop();
bool isEmpty();
bool isFull();
void setNull();
class Full {};
class Empty {};
};
#include <iostream>
using namespace std;
template <class DataType>
stack<DataType>::stack()
{
size = MAX_SIZE;
top = -1;
data = new DataType[MAX_SIZE];
}
template <class DataType>
stack<DataType>::stack(int s) {
size = s;
top = -1;
data = new DataType[size];
}
template <class DataType>
stack<DataType>::~stack()
{
delete []data;
cout << "析构函数finished delete\n";
}
template <class DataType>
void stack<DataType>::push(DataType ch) {
if (isFull())
throw stack<DataType>::Full();
else
data[++top] = ch;
}
template <class DataType>
DataType stack<DataType>::pop() {
if (isEmpty())
throw stack<DataType>::Empty();
else
return data[top--];
}
template <class DataType>
DataType stack<DataType>::getTop()
{
if (!isEmpty())
return data[top];
}
template <class DataType>
bool stack<DataType>::isEmpty()
{
if (top == -1) {
return true;
}
else {
return false;
}
}
template <class DataType>
bool stack<DataType>::isFull() {
if (top == size - 1) {
return true;
}
else {
return false;
}
}
template <class DataType>
void stack<DataType>::setNull() {
top = -1;
cout << "top=-1!\n";
}
2.stack.cpp
#include "stack.hpp"
#include <iostream>
using namespace std;
int main() {
stack<char> st(2);
char ch;
try {
st.push('m');
st.push('k');
st.push('v');
}
catch (stack<char>::Full) {
cout << "stack full!\n";
}
try {
ch = st.pop();
cout << ch << endl;
ch = st.pop();
cout << ch << endl;
ch = st.pop();
cout << ch << endl;
}
catch (stack<char>::Empty)
{
cout << "stack empty!\n";
}
double c;
stack<double> sl(2);
try {
sl.push(6165.2);
sl.push(65.2);
sl.push(12.888);
}
catch (stack<double>::Full) {
cout << "stack full!\n";
}
try {
c = sl.pop();
cout << c << endl;
c = sl.pop();
cout << c << endl;
c = sl.pop();
cout << c << endl;
}
catch (stack<double>::Empty) {
cout << "stack empty!\n";
}
return 0;
}
5,总源码(单文件)
#include <iostream>
using namespace std;
const int MAX_SIZE = 100;
template <class DataType>
class stack
{
private:
DataType* data;
int size;
int top;
public:
stack();
stack(int s);
~stack();
void push(DataType ch);
DataType pop();
DataType getTop();
bool isEmpty();
bool isFull();
void setNull();
class Full {};
class Empty {};
};
template <class DataType>
stack<DataType>::stack()
{
size = MAX_SIZE;
top = -1;
data = new DataType[MAX_SIZE];
}
template <class DataType>
stack<DataType>::stack(int s) {
size = s;
top = -1;
data = new DataType[size];
}
template <class DataType>
stack<DataType>::~stack()
{
delete[]data;
cout << "析构函数finished delete\n";
}
template <class DataType>
void stack<DataType>::push(DataType ch) {
if (isFull())
throw stack<DataType>::Full();
else
data[++top] = ch;
}
template <class DataType>
DataType stack<DataType>::pop() {
if (isEmpty())
throw stack<DataType>::Empty();
else
return data[top--];
}
template <class DataType>
DataType stack<DataType>::getTop()
{
if (!isEmpty())
return data[top];
}
template <class DataType>
bool stack<DataType>::isEmpty()
{
if (top == -1) {
return true;
}
else {
return false;
}
}
template <class DataType>
bool stack<DataType>::isFull() {
if (top == size - 1) {
return true;
}
else {
return false;
}
}
template <class DataType>
void stack<DataType>::setNull() {
top = -1;
cout << "top=-1!\n";
}
int main() {
stack<char> st(2);
char ch;
try {
st.push('m');
st.push('k');
st.push('v');
}
catch (stack<char>::Full) {
cout << "stack full!\n";
}
try {
ch = st.pop();
cout << ch << endl;
ch = st.pop();
cout << ch << endl;
ch = st.pop();
cout << ch << endl;
}
catch (stack<char>::Empty)
{
cout << "stack empty!\n";
}
double c;
stack<double> sl(2);
try {
sl.push(6165.2);
sl.push(65.2);
sl.push(12.888);
}
catch (stack<double>::Full) {
cout << "stack full!\n";
}
try {
c = sl.pop();
cout << c << endl;
c = sl.pop();
cout << c << endl;
c = sl.pop();
cout << c << endl;
}
catch (stack<double>::Empty) {
cout << "stack empty!\n";
}
return 0;
}
测试结果 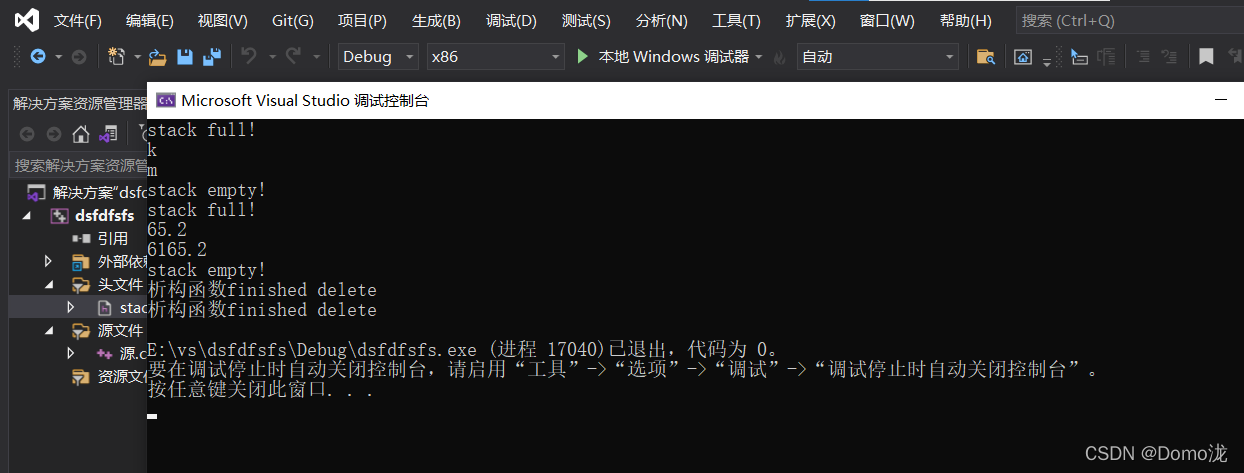 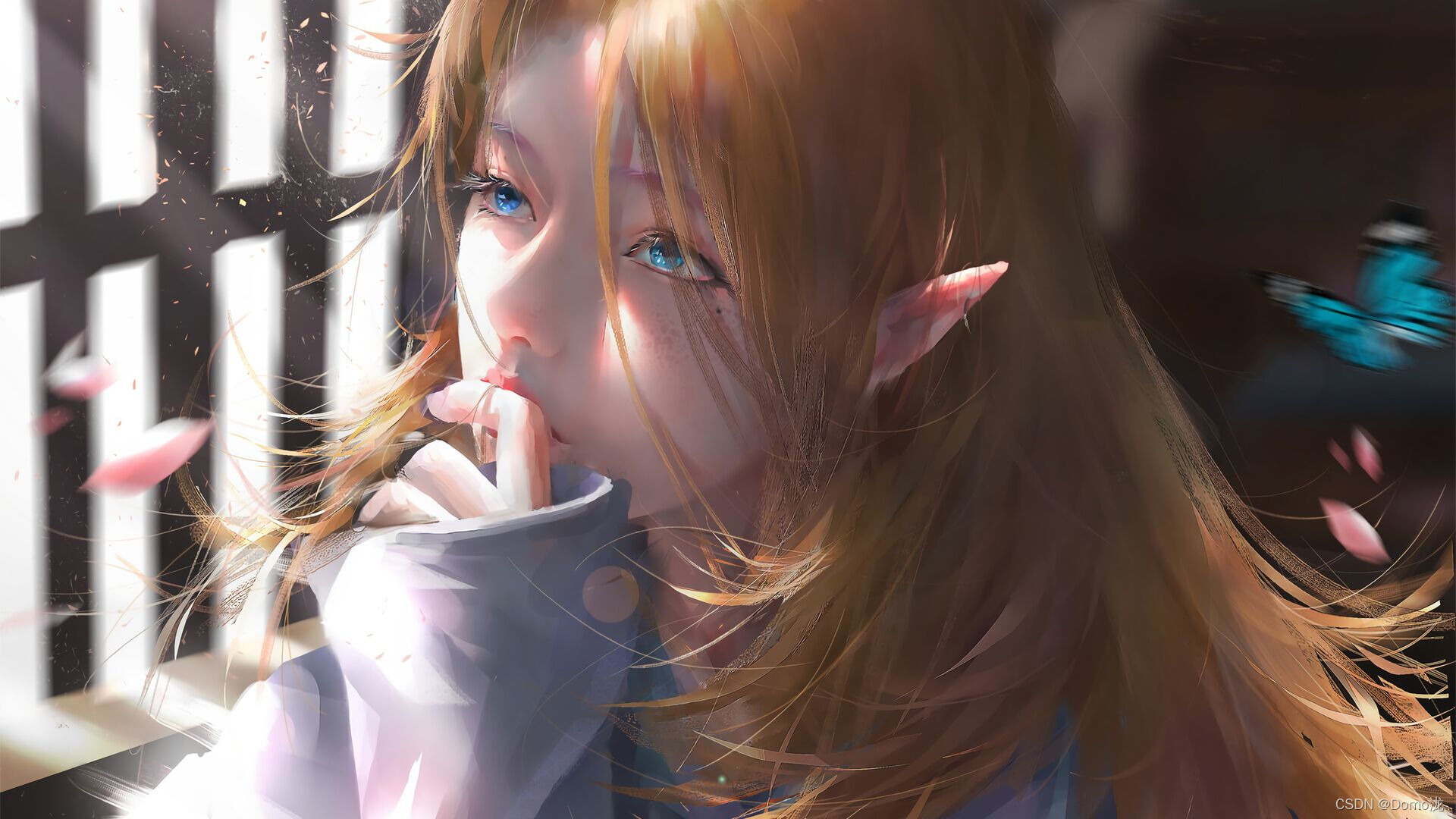
|