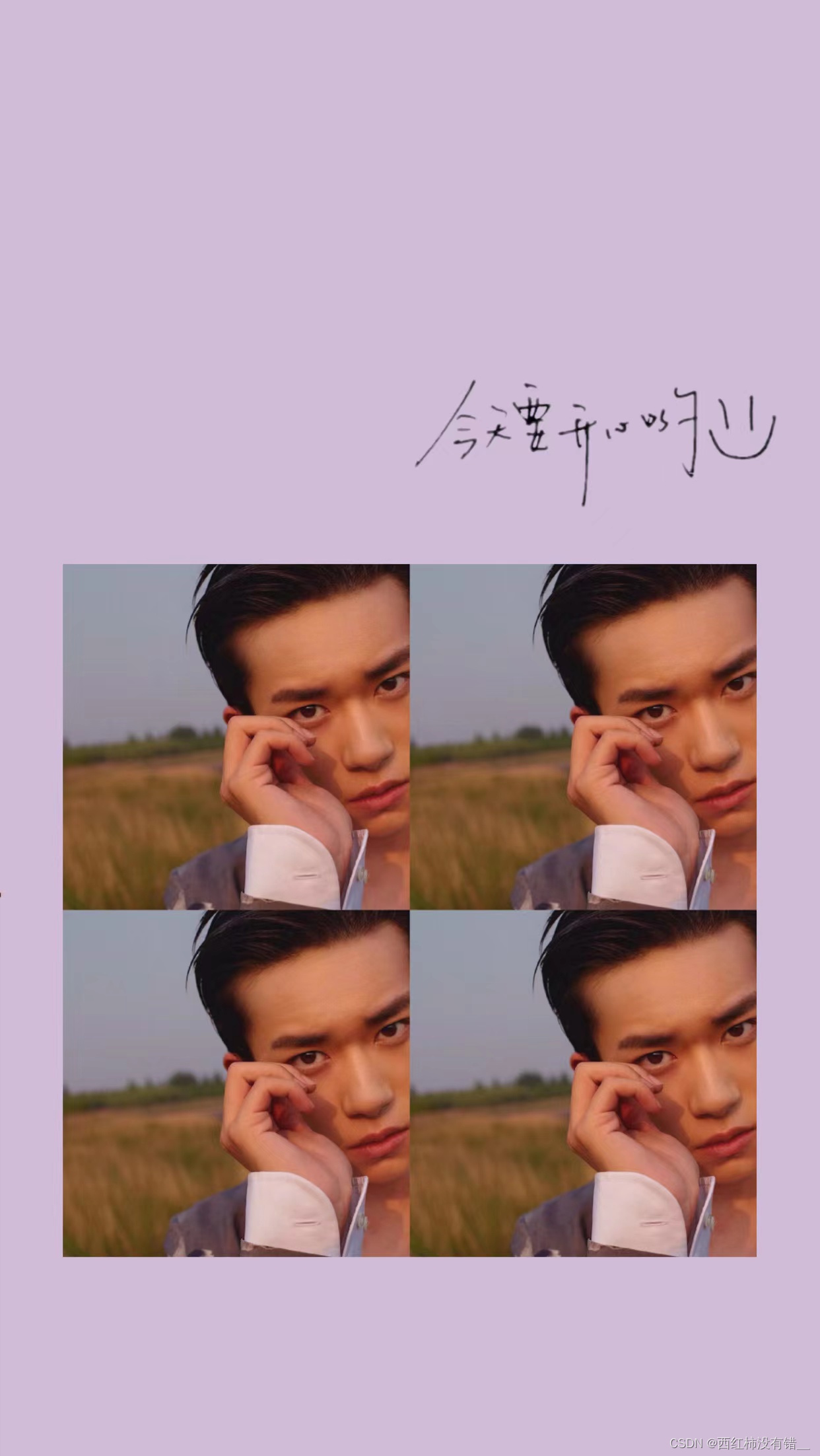
每天多学一分钟 时代峻峰挑老公
安装vue-router@04版本的
cnpm i vue-router@4
使用
1.创建与pages同级的目录router,新建index.js文件
import {createRouter,createWebHashHistory,createWebHistory} from 'vue-router'
// 导入创建的组件
import Home from '@/pages/Home.vue'
import About from '@/pages/About.vue'
// 配置路由的映射关系
const routes = [
{
path:"/home",
component:Home
},
{
path:"/about",
component:About
}
]
// 创建router对象
concst router = createRouter({
routes,
history:createWebHashHistory(), // 带#号
// 或者 createWebHistory() 不带#号,生产环境下不能直接访问项目,需要nginx转发)
})
// 抛出创建好的router对象
export default router
-
在vue项目的入口文件main.js里面引入 import {createApp} from 'vue'
import router from './router'
import App from './App.vue'
const app = createApp(App)
app.use(router) // 安装路由对象
app.mount(#app)
设置页面路由默认路径
- path 配置的是根路径: /
- redirect 是将路径重定向到某一个路由
router/index.js
const routes = [
{
path:"/",
redirect:"/home"
},
{
path:"/home",
component: Home
}
]
router-link 标签
<router-link to='/home' replace active-class='tomato-link-active' exact-active-class='tomato-link-exact-class'>我是router-link</router-link>
to : 是一个字符串或者一个对象 replace: 设置上,一旦点击会调用router.replace方法,回退就回不来了 active-class : 设置a元素active后应用的class,默认是router-link-active exact-active-class:路由精准匹配,应用于a元素的class , 默认是 router-link-exact-class
路由懒加载(路由分包)
(开发还是建议分包的,不分包的话所有组件代码都会打包到app.js里面,加载时间越长,首次进入白屏时间越长)
component: 路由懒加载要求返回的是一个Promise
router/index.js
const routes = [
{
path:"/home",
component:()=>import("../pages/Home.vue")
}
]
webpack的魔法注释
给分包的文件指定名字 home-chunk
const routes = [
{
path:"/home",
component:()=>import(/*webpackChunkName:"home-chunk"*/"../pages/Home.vue")
}
]
补充知识(属性)
cosnt routes = [
{
path:"/home",
name:"home",
component:() => import("../pages/Home.vue")
}
]
const routes = [
{
path:"/home",
name:"home",
component:() => import("../pages/Home.vue"),
meta:{
name:"tomato",
age:18,
height:1.5
}
}
]
动态路由的基本匹配
携带路径参数
// router> index.js
const routes = [
// 匹配一个
{
path:"/user:usename",
component:() => import("../pages/User.vue")
},
// 匹配多个
{
path:"/user/:id/info/:name",
component:() => import ("../pages/User.vue")
}
]
//跳转到User的router-link
<router-link to='/user/tomato'>查看用户tomato的相关信息</router-link>
匹配模式 | 匹配路径 | $route.params |
---|
/users/:id | /users/123 | {id:“123”} | /users/:id/info/:name | /users/123/info/tomato | {id:“123”,name:“tomato”} |
获取动态路由的值
// template 标签里
<template>
// 与路由定义那的名称保持一致
<h2>{{$route.params.username}}<h2>
</template>
// created生命周期里(vue2)
created(){
this.$route.params.username
}
// setup函数里面(vue3里面setup执行在created之前的)
import {useRoute} from 'vue-router'
setup(){
const route = useRoute(); // 返回一个route对象,里面是当前路由相关的值
route.params.username
}
错误页面or NotFound页面
当路由匹配到一个不存在的路径时,显示某一个组件
const routes = [
{
path:"/:pathMath(.*)",
component:() => import("../pages/NotFound.vue")
}
]
// NotFound.vue中获取传入的参数
<h2>{{$route.params.pathMatch}}</h2>
使用 “/:pathMath(.*)” 得到的$route.params对象 
使用 “/:path(.pathMath*)*” 得到的$route.params是一个数组 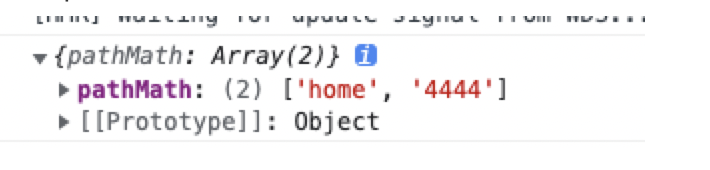
年底啦!真的好容易犯懒!但是我要执行我的flag,2022年每周发三篇笔记或者总结! (开端真的好好看!!
|