类的私有属性
class Person {
name
#age
#weight
constructor(name, age, weight) {
this.name = name
this.#age = age
this.#weight = weight
}
intro() {
console.log(this.name)
console.log(this.#age)
console.log(this.#weight)
}
}
const girl = new Person('晓红', 18, '45kg')
console.log(girl.name)
girl.intro()
Promise.allSettled()方法
- 每个异步任务都想得到结果就使用
Promise.allSettled() 方法 - 要求每个异步任务都成功才能往下执行则使用
Promise.all() 方法
const p1 = new Promise((resolve, reject) => {
setTimeout(() => {
reject('出错了1')
}, 1000)
})
const p2 = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('商品数据2')
}, 1000)
})
const result = Promise.allSettled([p1, p2])
console.log(result)
const res = Promise.all([p1, p2])
console.log(res)
结果: 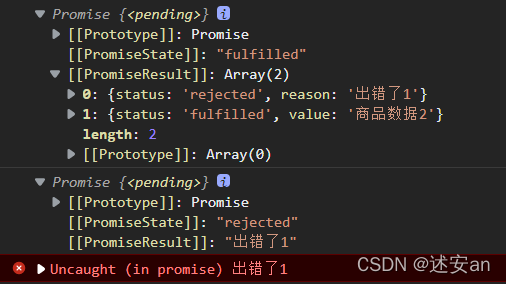
字符串新增方法:matchAll()
- 对数据的批量提取十分方便(可用于爬虫)
- 配合正则使用
let str =
`<ul>
<li>
<a>肖生克的救赎</a>
<p>上映日期: 1994-09-10</p>
</li>
<li>
<a>阿甘正传</a>
<p>上映日期: 1994-07-06</p>
</li>
</ul>`
const reg = /<li>.*?<a>(.*?)<\/a>.*?<p>(.*?)<\/p>/sg
const result = str.matchAll(reg)
console.log(result)
const arr = [...result]
console.log(arr)
结果: 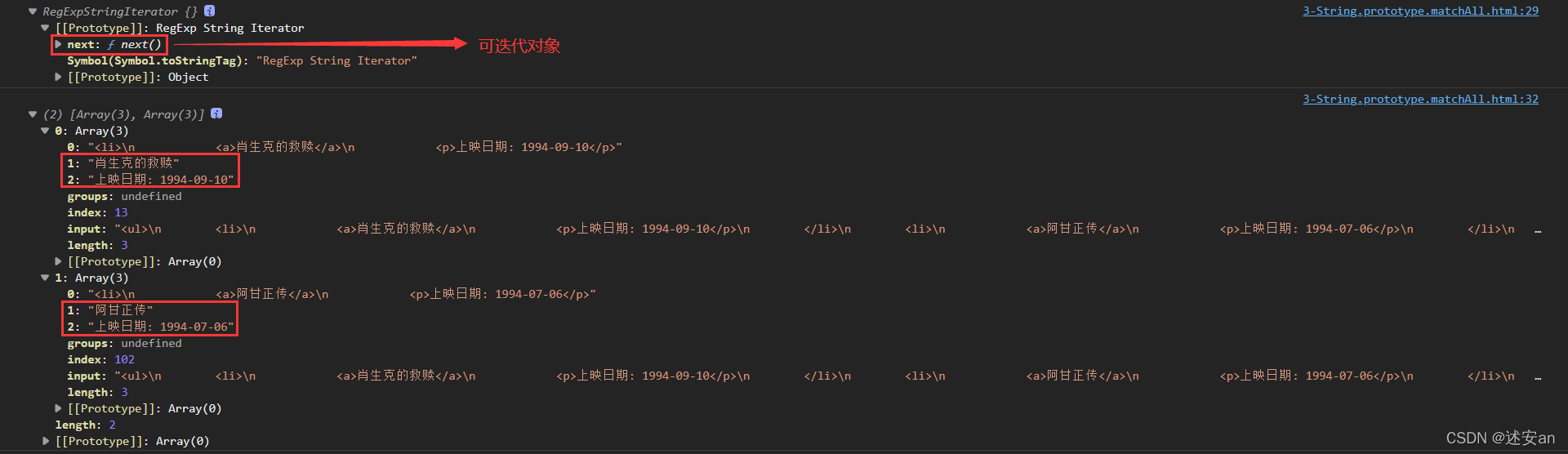
可选链操作符〔?.〕
function main(config) {
const dbHost = config?.db?.host
console.log(dbHost)
}
main({
db: {
host: '192.168.1.100',
username: 'root'
},
cache: {
host: '192.168.1.200',
username: 'admin'
}
})
动态 import
index.html:
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button id="btn">点击</button>
<script src="./js/app.js" type="module"></script>
</body>
</html>
app.js:
const btn = document.getElementById('btn')
btn.onclick = function () {
import('./hello.js').then(module => {
module.hello()
})
}
hello.js:
export function hello() {
alert('Hello')
}
新增的数据类型:BigInt(大整型)
let n = 521n
console.log(n, typeof (n))
console.log(BigInt(123))
let max = Number.MAX_SAFE_INTEGER
console.log(max)
console.log(max + 1)
console.log(max + 2)
console.log(BigInt(max))
console.log(BigInt(max) + BigInt(1))
console.log(BigInt(max) + 2n)
globalThis:全局的this
console.log(globalThis)
- 在nodeJS环境是
Object [global] 对象
|