using System;
using System.Data;
using MySql.Data.MySqlClient;
public static void debug_mysqladapter()
{
MySqlConnection conn = new MySqlConnection(GetConnectString());
try
{
conn.Open();
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
throw ex;
}
Console.WriteLine($"ConnectionState:{conn.State}");
MySqlCommand cmd = conn.CreateCommand();
cmd.CommandText = "show tables;";
//Create a SqlDataAdapter for the Suppliers table.
MySqlDataAdapter adapter = new MySqlDataAdapter();
// A table mapping names the DataTable.
adapter.TableMappings.Add("Table", "Suppliers");
// Set the SqlDataAdapter's SelectCommand.
adapter.SelectCommand = cmd;
// Fill the DataSet.
DataSet dataSet = new DataSet();
dataSet.Reset();
adapter.Fill(dataSet);
// Fill the DataSet.
adapter.Fill(dataSet);
DataTableReader reader = dataSet.CreateDataReader();
{
if (!reader.HasRows)
{
Console.WriteLine("Empty DataTableReader");
}
else
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write(reader[i] + " ");
}
Console.WriteLine();
}
}
Console.WriteLine("========================");
} while (reader.NextResult()) ;
reader.Close();
dataSet.Clear();
conn.Close();
Console.WriteLine($"ConnectionState:{conn.State}");
}
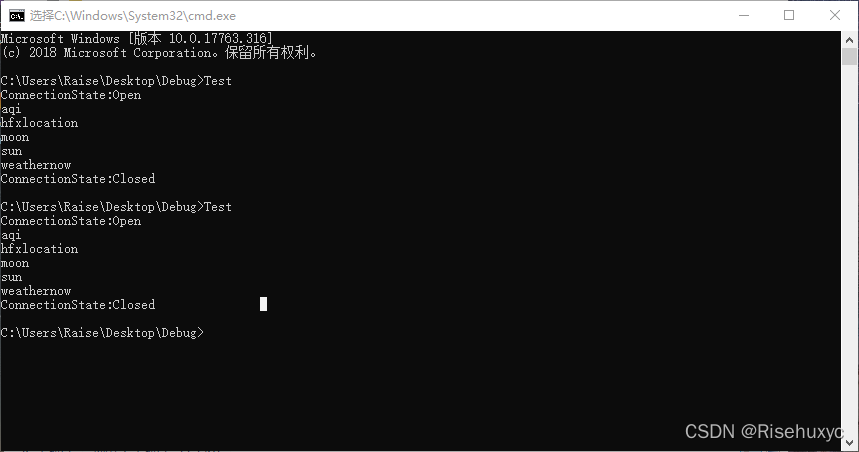
?
MySQL用C#Adapt模式连接。
|