网页获取地理位置信息,通过百度地图API,实现网页加载地图功能
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no, minimal-ui">
<meta content="yes" name="apple-mobile-web-app-capable">
<meta content="black" name="apple-mobile-web-app-status-bar-style">
<meta content="telephone=no" name="format-detection">
<meta content="email=no" name="format-detection">
<title>获取地理位置并显示</title>
<style>
* {
margin: 0;
padding: 0;
font-size: 17px;
}
.map {
width: 100%;
height: 400px;
}
.btn-box {
margin-top: 1em;
width: 100%;
height: 44px;
display: box;
display: -webkit-box;
display: flex;
display: -webkit-flex;
-webkit-box-pack: justify;
justify-content: space-between;
webkit-justify-content: space-between;
-webkit-box-align: center;
align-items: stretch;
webkit-align-items: stretch;
}
.btn {
color: #fff;
background-color: #007AFF;
border-radius: 10px;
font-size: 17px;
text-align: center;
padding: 10px
}
.tips {
margin-top: 10px;
text-align: center;
}
</style>
<script src="http://api.map.baidu.com/api?v=1.2&ak=Ggf8yroht24x4KszoWsGV2OwtgExn8YQ"></script>
</head>
<body>
<div id="showmap" class="map">
</div>
<div class="btn-box">
<div class="btn btn-get">
获取位置数据
</div>
<div class="btn btn-show">
展示地图标注
</div>
</div>
<div class="tips"></div>
<script>
var map = new BMap.Map("showmap");
var point = new BMap.Point(116.404, 39.915);
map.centerAndZoom(point, 14);
map.enableScrollWheelZoom(true);
map.disableDragging();
setTimeout(function() {
map.enableDragging();
}, 1000);
setTimeout(function() {
map.setZoom(8);
}, 2000);
var currentPoint = {
latAndLong: [116.404, 39.915],
name: "这是默认的位置"
};
document.querySelector(".btn-get").addEventListener('click', function() {
getLocation();
});
document.querySelector(".btn-show").addEventListener('click', function() {
addMarker(currentPoint.latAndLong, currentPoint.name);
});
function getLocation() {
if(navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition, showError);
} else {
alert("浏览器不支持地理定位。");
};
};
function showPosition(position) {
var lat = position.coords.latitude;
var lng = position.coords.longitude;
console.log('纬度:' + lat + ',经度:' + lng);
currentPoint.latAndLong = (lng + ',' + lat).split(',');
currentPoint.name = '我的位置';
console.log(currentPoint);
document.querySelector(".tips").innerText = currentPoint.name + '纬度:' + lat + ',经度:' + lng;
};
function addMarker(arr, name) {
var pt = new BMap.Point(arr[0], arr[1]);
var myIcon = new BMap.Icon("http://sandbox.runjs.cn/uploads/rs/269/px1iaa72/peoicon.png", new BMap.Size(30, 30));
var marker = new BMap.Marker(pt, {
icon: myIcon
});
map.addOverlay(marker);
var label = new BMap.Label(name, {
offset: new BMap.Size(20, -10)
});
marker.setLabel(label);
marker.disableDragging();
document.querySelector(".tips").innerText = "如所在位置为展示在当前窗口中,请拖动地图查看。";
};
function showError(error) {
switch(error.code) {
case error.PERMISSION_DENIED:
document.querySelector(".tips").innerText = "定位失败,您已拒绝请求地理定位";
break;
case error.POSITION_UNAVAILABLE:
document.querySelector(".tips").innerText = "定位失败,位置信息是不可用";
break;
case error.TIMEOUT:
document.querySelector(".tips").innerText = "定位失败,请求获取用户位置超时";
break;
case error.UNKNOWN_ERROR:
document.querySelector(".tips").innerText = "定位失败,定位系统失效";
break;
}
};
</script>
</body>
</html>
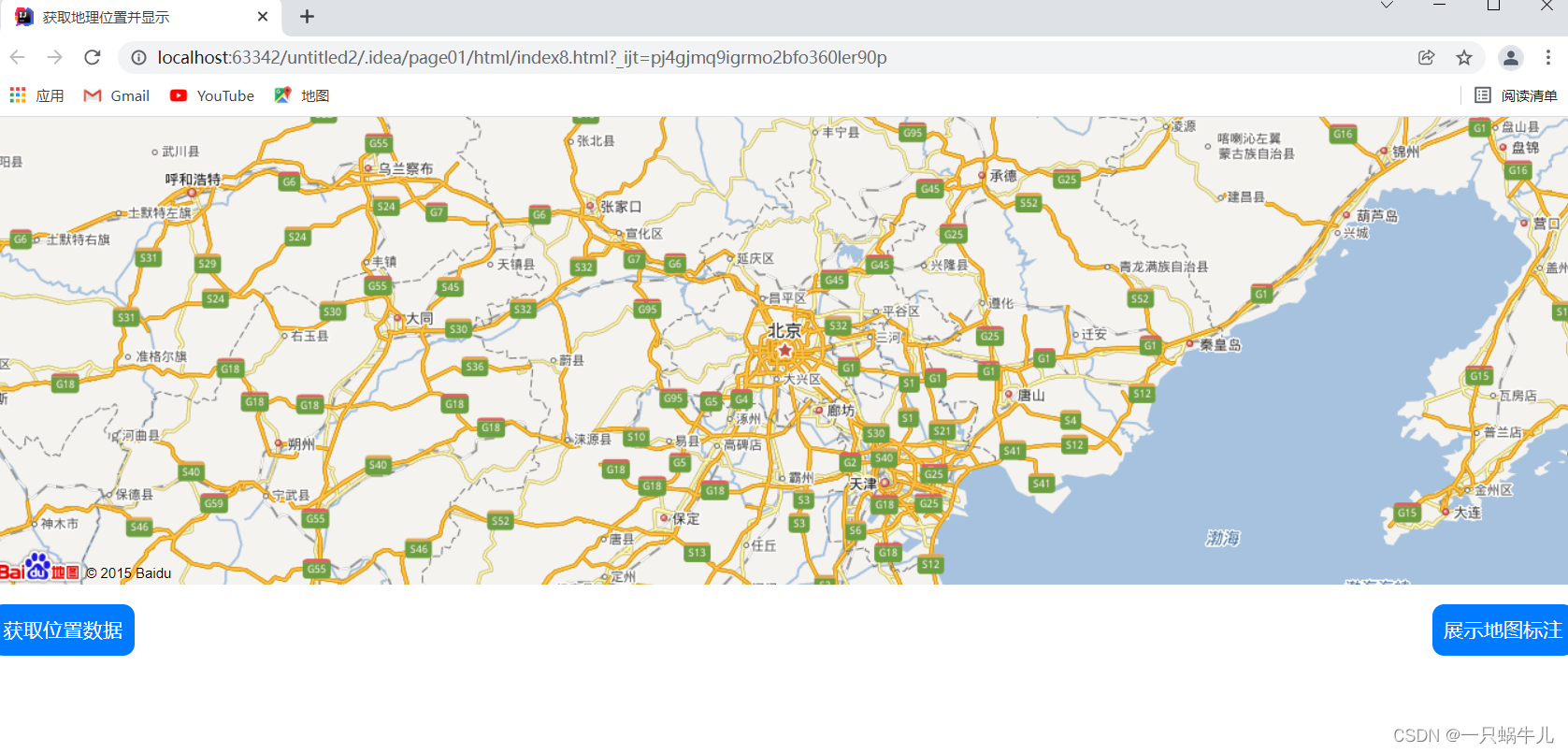
|