JavaScript深入之继承
1.借助原型链实现继承
function Parent(){
this.name = "zhu"
};
Parent.prototype.getName = function(){
console.log(this.name);
};
function Child() {
};
Child.prototype = new Parent();
var child1 = new Child();
child1.getName();
不过原型链继承有两个缺点: 1.引用实例会被所有实例共享
function Parent() {
this.name = ["猪","狗"];
}
Parent.prototype.getName = function () {
console.log(this.name);
};
function Child() {}
Child.prototype = new Parent();
var child1 = new Child();
var child2 = new Child();
child1.name.push("鸟")
child1.getName();
child2.getName();
我们向child1中push"鸟",但是child2中也会共享这个属性。 2.在创建 Child 的实例时,不能向Parent传参
2.构造函数继承
function Parent() {
this.name = ["猪","狗"];
}
function Child() {
Parent.call(this)
}
var child1 = new Child();
child1.name.push("鸟")
var child2 = new Child();
console.log(child1.name);
console.log(child2.name);
这个避免了引用类型的属性被所有实例共享,而且child可以向Parent传参。
function Parent(name) {
this.name = name;
}
function Child(name) {
Parent.call(this, name);
}
var child1 = new Child("猪");
console.log(child1.name);
var child2 = new Child("鸟")
console.log(child2.name);
4.组合继承
Object.create ()是es5的方法,将传入的对象作为创建的对象的原型,下面是他的模拟实现。
function createObj(obj) {
function F(){}
F.prototype = obj;
return new F();
}
我们将前两种组合并使用
function Parent() {
this.name = "parent";
this.number = [1, 2, 3];
}
function Child() {
Parent.call(this);
this.type = "child";
}
Child.prototype = Object.create(Parent.prototype);
Child.prototype.constructor = Child;
var child1 = new Child();
var child2 = new Child();
child1.number.push(4)
console.log(child1);
console.log(child2);
控制台输出一下: 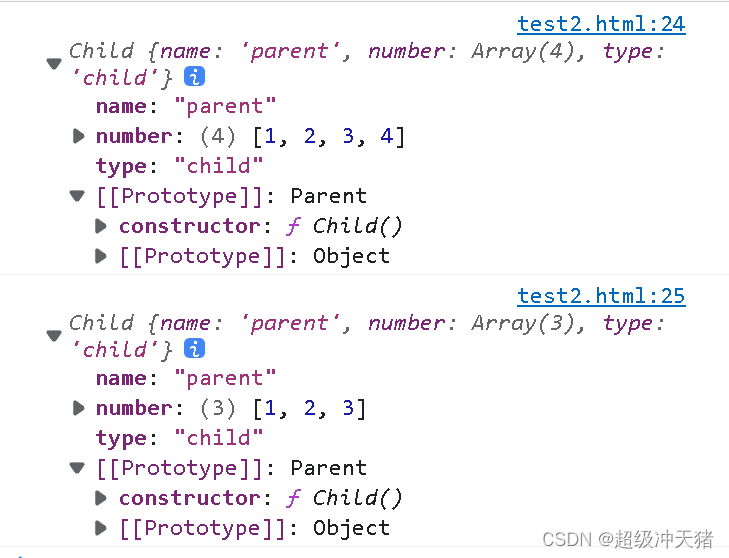
|