toga文档:https://toga.readthedocs.io/en/latest/
创建项目
运行命令:
briefcase new
填写参照下几张图: 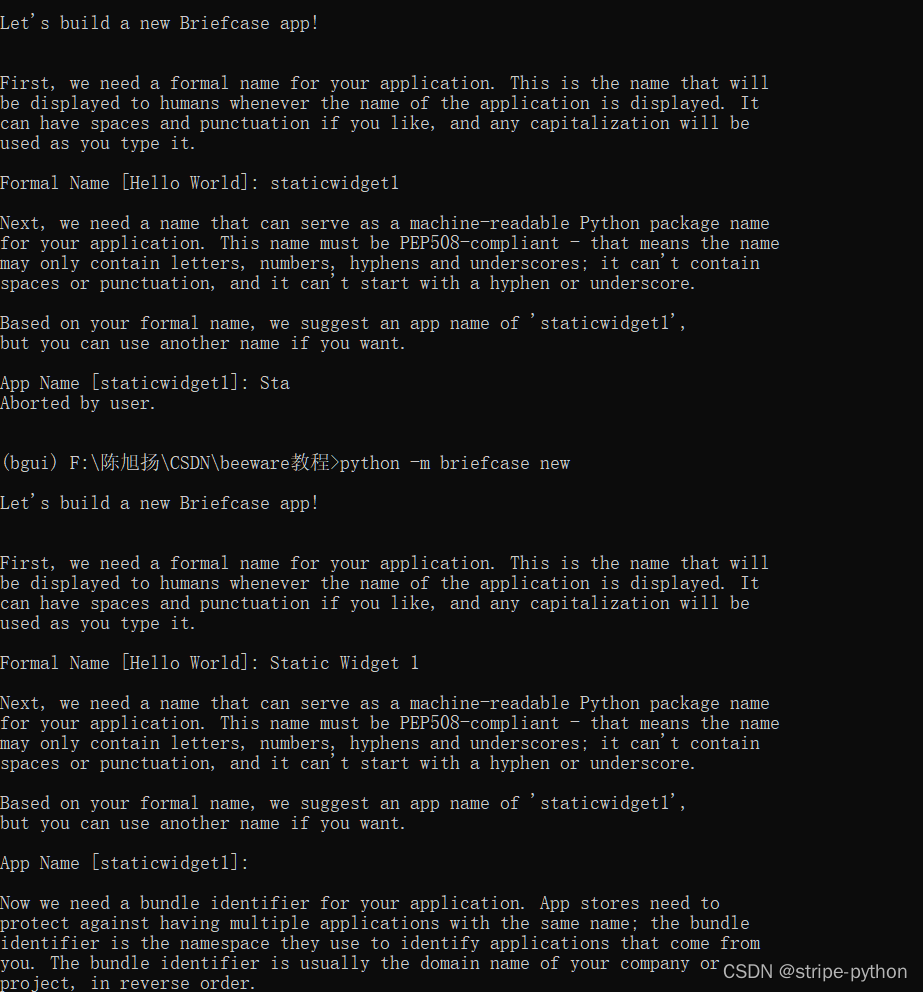 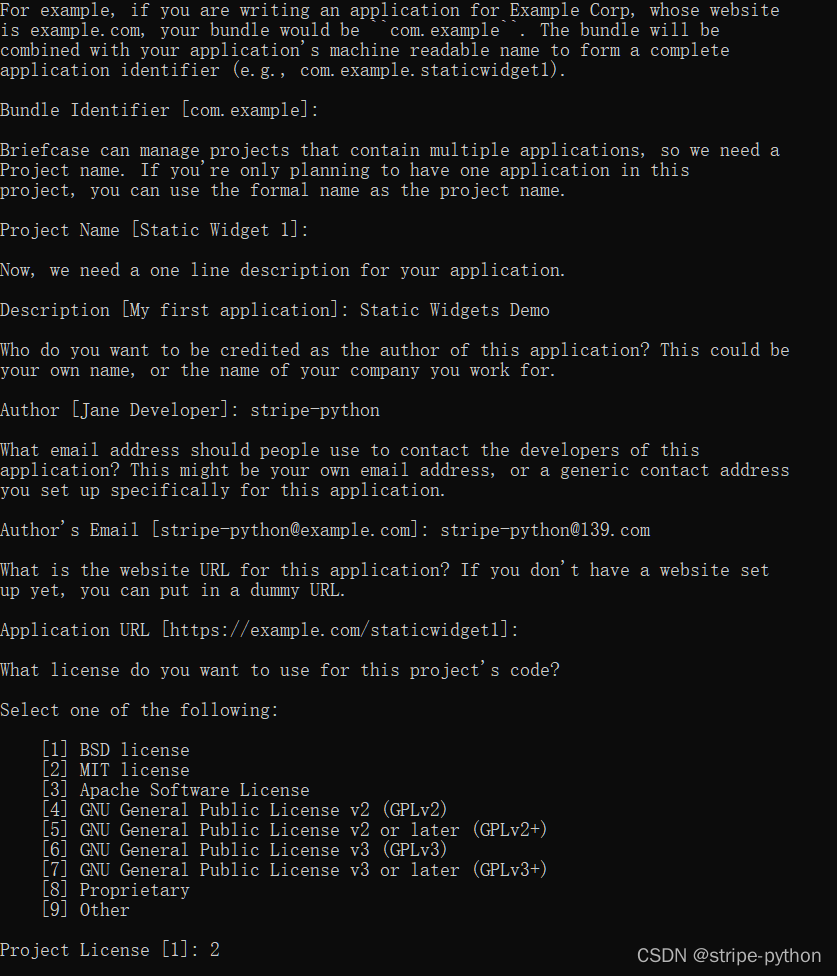 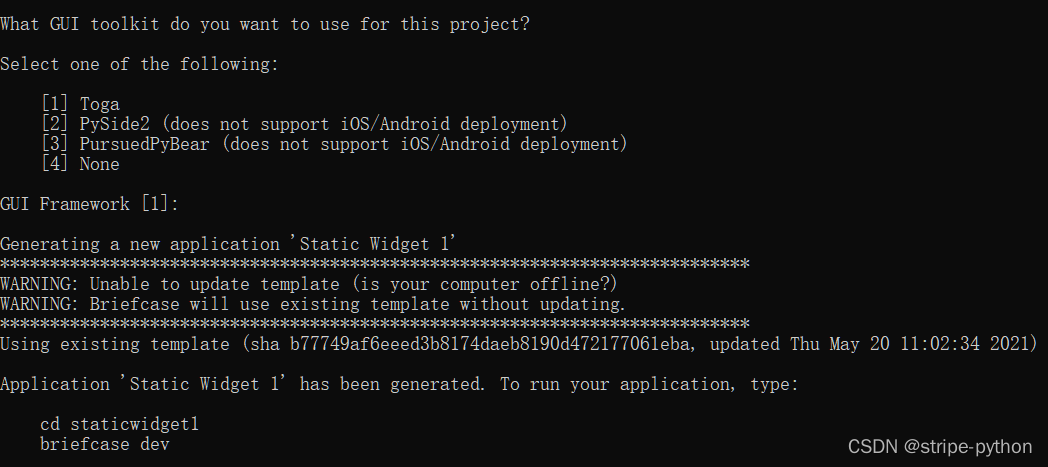 现在运行命令:
cd staticwidget1
open src/staticwidget1/resources/app.py
项目结构: 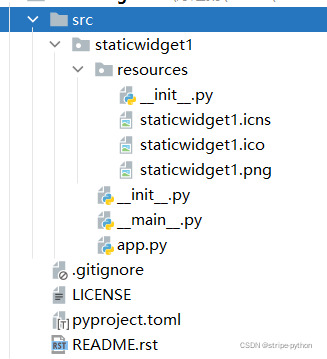
静态部件
沙箱
是toga 中的Box 对于平台的支持: 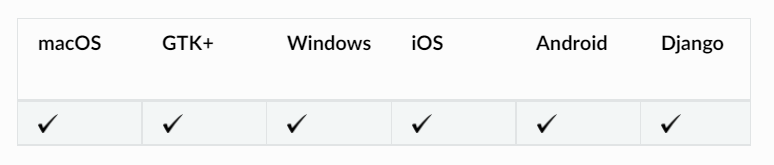 box类似于tkinter 中的Frame 和html 的div ,是一个承载部件的容器组件。 toga 中的例子:
import toga
box = toga.Box('box1')
button = toga.Button('Hello world', on_press=button_handler)
box.add(button)
Box类初始化方法:
Box(id=None, style=None, children=None, factory=None)
id: Box 的唯一标识,str类型。 style: Box 的风格。 children: Box 中的组件。 factory: 通常不用。
Box.add 支持添加多个组件。
按钮
toga.Button ,对于平台的支持:  Button 需要一个回调函数,该函数接收一个实参button 。 如:
import toga
def my_callback(button):
pass
button = toga.Button('Click me', on_press=my_callback)
Button初始化方法:
Button(label, id=None, style=None, on_press=None, enabled=True, factory=None)
label: 按钮上的文字 id: 按钮ID标识 style: 按钮风格 on_press: 按钮回调 enabled: 是否激活 factory: 通常不用。
Button可以被视作一个沙箱,支持add 方法。
输入框
toga.TextInput ,对于平台支持: 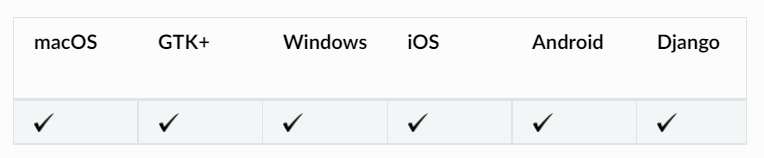 初始化:
TextInput(id=None, style=None, factory=None, initial=None, placeholder=None, readonly=False, on_change=None, on_gain_focus=None, on_lose_focus=None, validators=None)
id: 输入框ID标识 style: 输入框风格 factory: 通常不用 initial: 默认文字 placeholder: 提示文字 readonly: 是否采用只读 on_change: 回调,输入框中的文本更改时调用的方法 on_gain_focus: 回调,获得焦点时执行的函数 on_lose_focus: 回调,失去焦点时执行的函数 validators: 文本验证器,通常不用
文本
toga.Label ,平台支持: 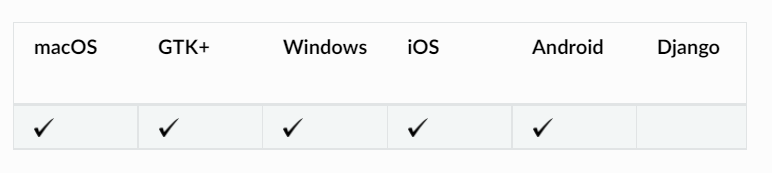 初始化:
Label(text, id=None, style=None, factory=None)
text: 文本 id: 文本ID标识 style: 文本风格 factory: 通常不用
密码输入框
toga.PasswordInput ,平台支持: 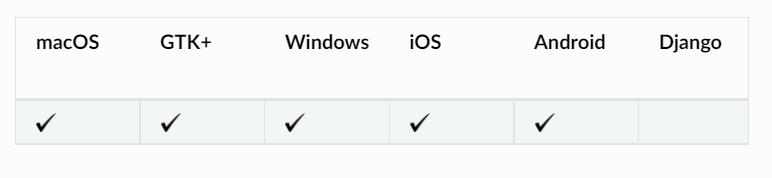 参数同toga.TextInput
实例:写一个登录页面
进入app.py ,默认模板:
"""
Static Widgets Demo
"""
import toga
from toga.style import Pack
from toga.style.pack import COLUMN, ROW
class StaticWidget1(toga.App):
def startup(self):
"""
Construct and show the Toga application.
Usually, you would add your application to a main content box.
We then create a main window (with a name matching the app), and
show the main window.
"""
main_box = toga.Box()
self.main_window = toga.MainWindow(title=self.formal_name)
self.main_window.content = main_box
self.main_window.show()
def main():
return StaticWidget1()
在startup 中定义UI:
main_box = toga.Box()
self.main_window = toga.MainWindow(title='Login')
self.main_window.content = main_box
self.main_window.show()
定义基本登录组件:
self.username_label = toga.Label('User Name:', 'username-label')
self.username_input = toga.TextInput('username-input', placeholder='input your username here')
self.password_label = toga.Label('Password:', 'password-label')
self.password_input = toga.PasswordInput('password-input', placeholder='input your password here')
定义登录按钮及其回调:
def login(button):
pass
self.login_button = toga.Button('Login', 'login-button', on_press=login)
添加至主Box :
main_box.add(self.username_label, self.username_input, self.password_label, self.password_input, self.login_button)
全部代码:
"""
Static Widgets Demo
"""
import toga
def login(button):
pass
class StaticWidget1(toga.App):
def startup(self):
"""
Construct and show the Toga application.
Usually, you would add your application to a main content box.
We then create a main window (with a name matching the app), and
show the main window.
"""
main_box = toga.Box()
self.username_label = toga.Label('User Name:', 'username-label')
self.username_input = toga.TextInput('username-input', placeholder='input your username here')
self.password_label = toga.Label('Password:', 'password-label')
self.password_input = toga.PasswordInput('password-input', placeholder='input your password here')
self.login_button = toga.Button('Login', 'login-button', on_press=login)
main_box.add(self.username_label, self.username_input, self.password_label, self.password_input, self.login_button)
self.main_window = toga.MainWindow(title='Login')
self.main_window.content = main_box
self.main_window.show()
def main():
return StaticWidget1()
进入项目根目录下,运行命令:
briefcase dev
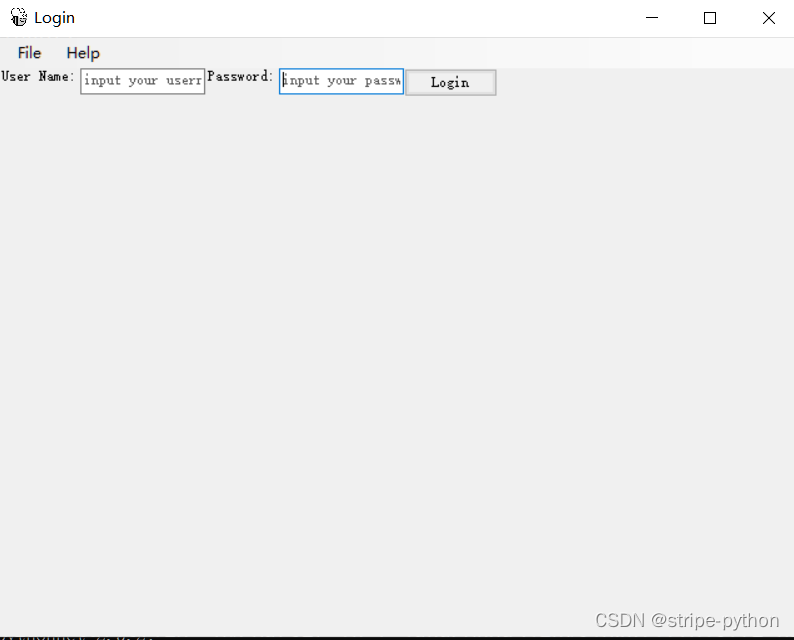 完善回调和布局后:
"""
Static Widgets Demo
"""
import toga
from toga.style import Pack
from toga.style.pack import COLUMN, ROW
class StaticWidget1(toga.App):
def startup(self):
main_box = toga.Box()
username_box = toga.Box(style=Pack(direction=ROW))
password_box = toga.Box(style=Pack(direction=ROW))
self.username_label = toga.Label('User Name:', 'username-label')
self.username_input = toga.TextInput('username-input', placeholder='input your username here', style=Pack(flex=1))
username_box.add(self.username_label, self.username_input)
self.password_label = toga.Label('Password:', 'password-label')
self.password_input = toga.PasswordInput('password-input', placeholder='input your password here')
password_box.add(self.password_label, self.password_input)
self.login_button = toga.Button('Login', 'login-button', on_press=self.login)
main_box.add(username_box, password_box, self.login_button)
self.main_window = toga.MainWindow(title='Login')
self.main_window.content = main_box
self.main_window.show()
def login(self, button):
username = self.username_input.value
password = self.password_input.value
print(f'Username: {username} Password: {password}')
def main():
return StaticWidget1()
|