接下来,我们需要将文章数据变成动态的,也就是通过发ajax请求,从后端请求数据,并进行展示。
后端-是码神之路博客系统,已经实现好的
发起http请求我们使用axios: 易用、简洁且高效的http库
加入依赖:
?npm install --save axios vue-axios
//将下面代码 加入 main.js import Vue from 'vue' import axios from 'axios' import VueAxios from 'vue-axios'
Vue.use(VueAxios, axios)
建立统一请求处理
在src下创建request文件夹,在其下创建index.js:
import axios from "axios"
const service = axios.create({
baseURL:"http://localhost:8888",
timeout:10000
})
export default service
在src下创建api文件夹,在其下创建article.js:
import request from "@/request"
/**
* 文章列表
* @param PageParams
*/
export function getArticles(PageParams)
{
return request({
url:"/articles",
method:'post',
data:PageParams
})
}
ArticleScrollPage.vue就可以直接引入,然后使用该接口
注意:
引入文件时,../以该目录为基准,往上跳一个目录,ArticleScrollPage.vue目录为common,往上跳一个目录为components,需要再往上跳到src才能引入api
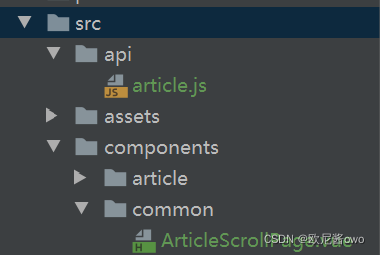
ArticleScrollPage.vue:
<template>
<scroll-page :loading="loading"
:offset="offset"
:no-data="noData"
@load="load">
<article-item v-for="article in articles"
:key="article.id"
v-bind="article">
</article-item>
</scroll-page>
</template>
<script>
import ArticleItem from '@/components/article/ArticleItem'
import ScrollPage from '@/components/scrollpage'
/*
引入文件时,../以该目录为基准,往上跳一个目录,ArticleScrollPage.vue目录为common,往上跳一个目录为components,需要再往上跳到src才能引入api
*/
import {getArticles} from '@/api/article'
export default
{
name: "ArticleScrollPage",
props: {
offset: {
type: Number,
default: 0
}
},
created()
{
this.getArticles();
},
data() {
return {
loading: false,
noData: true,
//分页参数
PageParams: {
page: 1,
pageSize: 5,
},
//文章列表
articles: []
}
},
methods: {
load() {
//下拉触发分页的时候,调用接口加载
alert("触发分页")
this.getArticles();
},
getArticles() {
let that = this;
that.loading = true;
getArticles(this.PageParams).then((res)=>
{
if(res.data.success)
{
if(res.data.data.length <= 0)
{
this.noData = true;
}
this.articles = this.articles.concat(res.data.data)
this.PageParams.page += 1;
}else
{
this.$message.error(res.data.msg)
}
}).catch((err)=>
{
console.log()
}).finally(()=>
{
that.loading = false
});
this.noData = false;
}
},
components: {
'article-item': ArticleItem,
'scroll-page': ScrollPage
}
}
</script>
<style scoped>
.el-card
{
border-radius: 0;
}
.el-card:not(:first-child)
{
margin-top: 10px;
}
</style>
?
|