小帽学堂
9. 添加课程信息完善(课程简介)
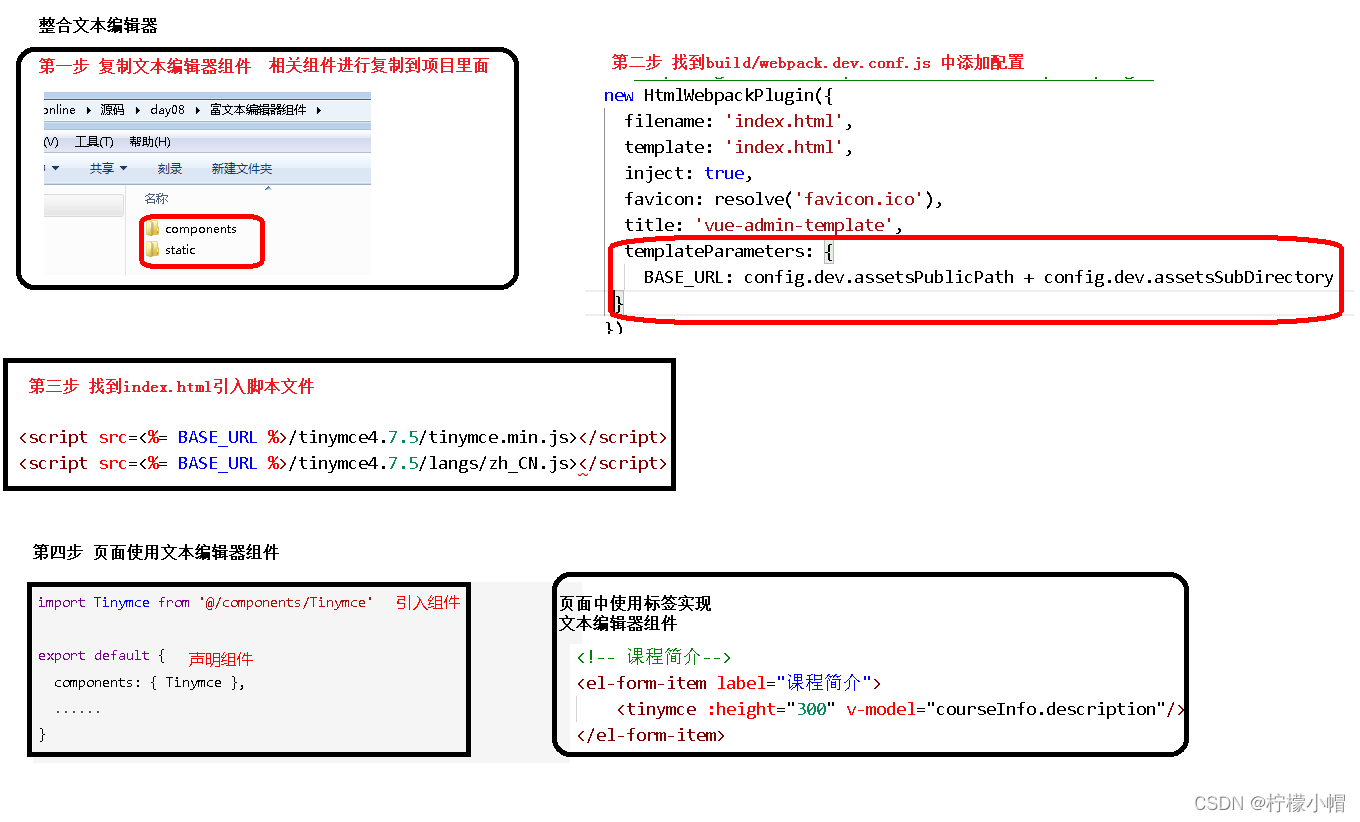
<el-form-item label="课程简介">
<tinymce :height="300" v-model="courseInfo.description"/>
</el-form-item>
<script>
import Tinymce from '@/components/Tinymce'
export default {
components: { Tinymce },
......
}
</script>
<style scoped>
.tinymce-container {
line-height: 29px;
}
</style>
- 图片的base64编码
- Tinymce中的图片上传功能直接存储的是图片的base64编码,因此无需图片服务器
10. 课程大纲列表(后端)
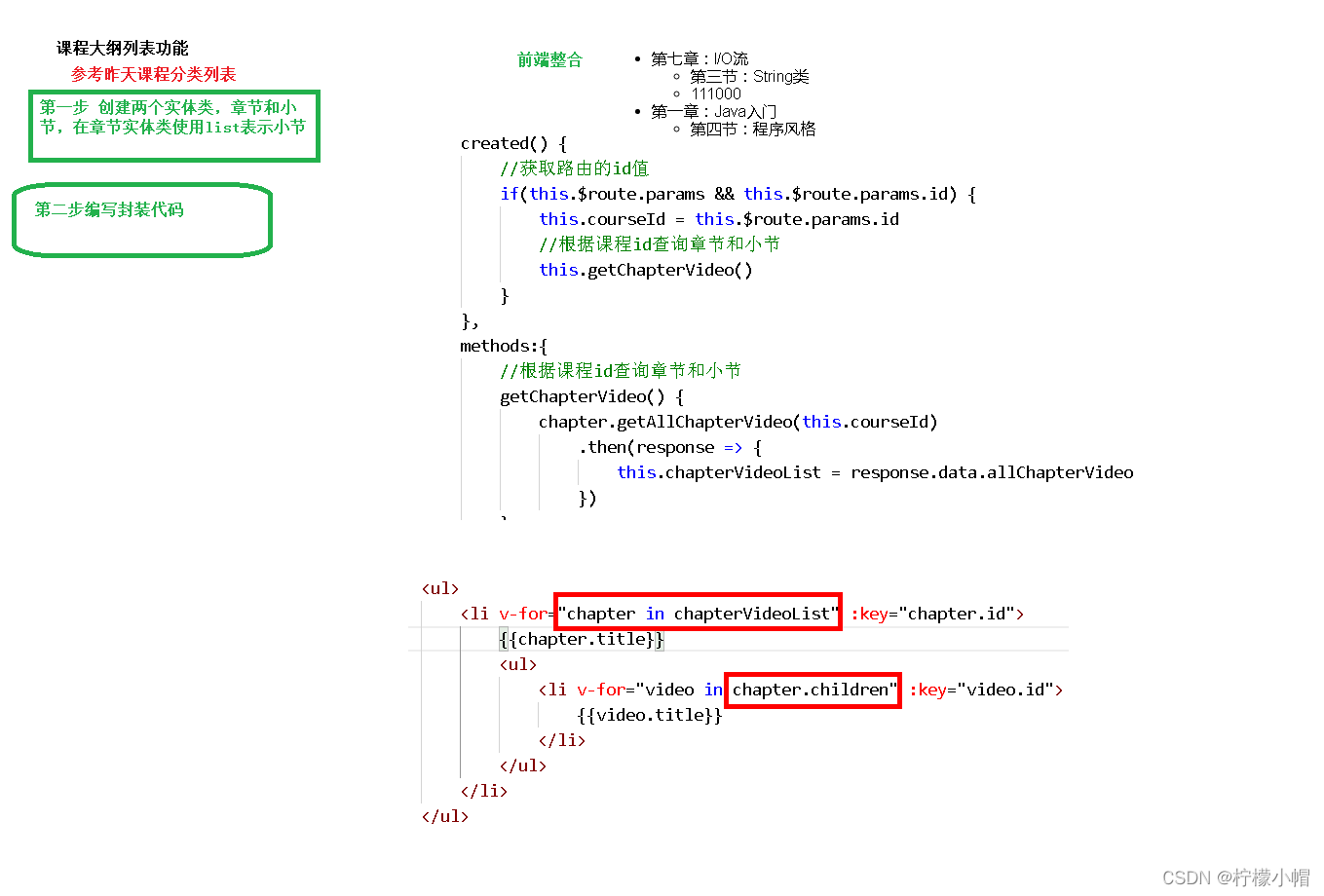
@Data
public class ChapterVo {
private String id;
private String title;
private List<VideoVo> children = new ArrayList<>();
}
@Data
public class VideoVo {
private String id;
private String title;
}
@RestController
@RequestMapping("/eduservice/chapter")
public class EduChapterController {
@Autowired
private EduChapterService chapterService;
@GetMapping("getChapterVideo/{courseId}")
public R getChapterVideo(@PathVariable String courseId) {
List<ChapterVo> list = chapterService.getChapterVideoByCourseId(courseId);
return R.ok().data("allChapterVideo", list);
}
}
public interface EduChapterService extends IService<EduChapter> {
List<ChapterVo> getChapterVideoByCourseId(String courseId);
}
@Service
public class EduChapterServiceImpl extends ServiceImpl<EduChapterMapper, EduChapter> implements EduChapterService {
@Autowired
private EduVideoService videoService;
@Override
public List<ChapterVo> getChapterVideoByCourseId(String courseId) {
QueryWrapper<EduChapter> wrapperChapter = new QueryWrapper<>();
wrapperChapter.eq("course_id", courseId);
List<EduChapter> eduChapterList = baseMapper.selectList(wrapperChapter);
QueryWrapper<EduVideo> wrapperVideo = new QueryWrapper<>();
wrapperVideo.eq("course_id", courseId);
List<EduVideo> eduVideoList = videoService.list(wrapperVideo);
List<ChapterVo> finalList = new ArrayList<>();
for (int i = 0; i < eduChapterList.size(); i++) {
EduChapter eduChapter = eduChapterList.get(i);
ChapterVo chapterVo = new ChapterVo();
BeanUtils.copyProperties(eduChapter, chapterVo);
finalList.add(chapterVo);
List<VideoVo> videoList = new ArrayList<>();
for (int m = 0; m < eduVideoList.size(); m++) {
EduVideo eduVideo = eduVideoList.get(m);
if(eduVideo.getChapterId().equals(eduChapter.getId())) {
VideoVo videoVo = new VideoVo();
BeanUtils.copyProperties(eduVideo,videoVo);
videoList.add(videoVo);
}
}
chapterVo.setChildren(videoList);
}
return finalList;
}
}
|