1. 如下代码测试对象的浅拷贝、深拷贝,请绘制出内存示意图。
import copy
class MobilePhone:
def __init__(self,cpu,screen):
self.cpu = cpu
self.screen = screen
class Cpu:
def calculate(self):
print('cpu对象',self)
class Screen:
def show(self):
print('显示一个好看的画面',self)
c1 = Cpu()
c2 = c1 # 单纯地引用,没有任何辅助,因为开始的地址一样
print(c1)
print(c2)
s1 = Screen()
m1 = MobilePhone(c1,s1)
m2 = copy.copy(m1) # 浅复制,复制本身,后面都是引用,最开始的地址不同,但内部的地址全部相同
print(m1,m1.cpu,m1.screen)
print(m2,m2.cpu,m2.screen)
m3 = copy.deepcopy(m1) # 深复制,复制一套体系,所有地址都不相同
print(m1,m1.cpu,m1.screen)
print(m3,m3.cpu,m3.screen)
运行结果如下
<__main__.Cpu object at 0x0000023408ABA5C8>
<__main__.Cpu object at 0x0000023408ABA5C8>
<__main__.MobilePhone object at 0x0000023408B1AB08> <__main__.Cpu object at 0x0000023408ABA5C8> <__main__.Screen object at 0x0000023408B1AA88>
<__main__.MobilePhone object at 0x0000023408B1AB48> <__main__.Cpu object at 0x0000023408ABA5C8> <__main__.Screen object at 0x0000023408B1AA88>
<__main__.MobilePhone object at 0x0000023408B1AB08> <__main__.Cpu object at 0x0000023408ABA5C8> <__main__.Screen object at 0x0000023408B1AA88>
<__main__.MobilePhone object at 0x0000023408B1ABC8> <__main__.Cpu object at 0x0000023408B1AC48> <__main__.Screen object at 0x0000023408B1ACC8>
原理如下
引用原理
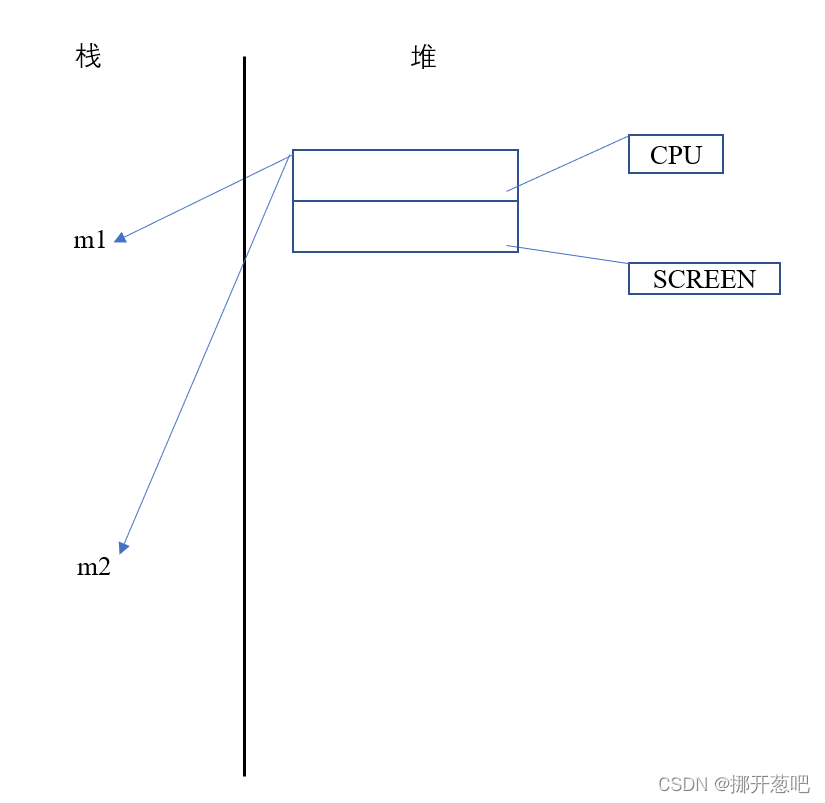
?
浅拷贝原理
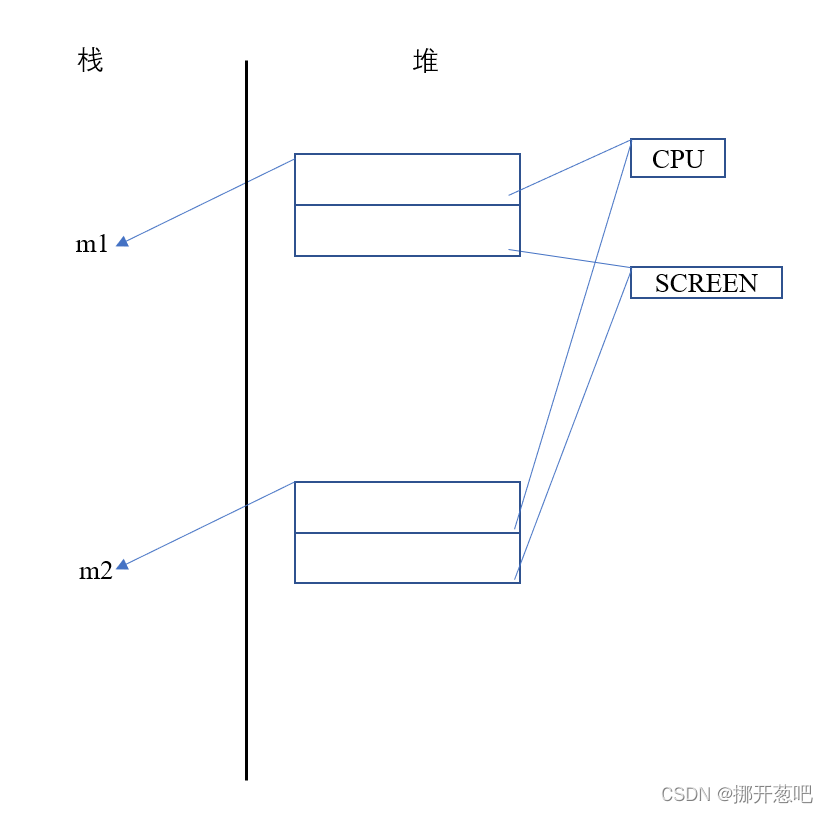
?
深拷贝原理
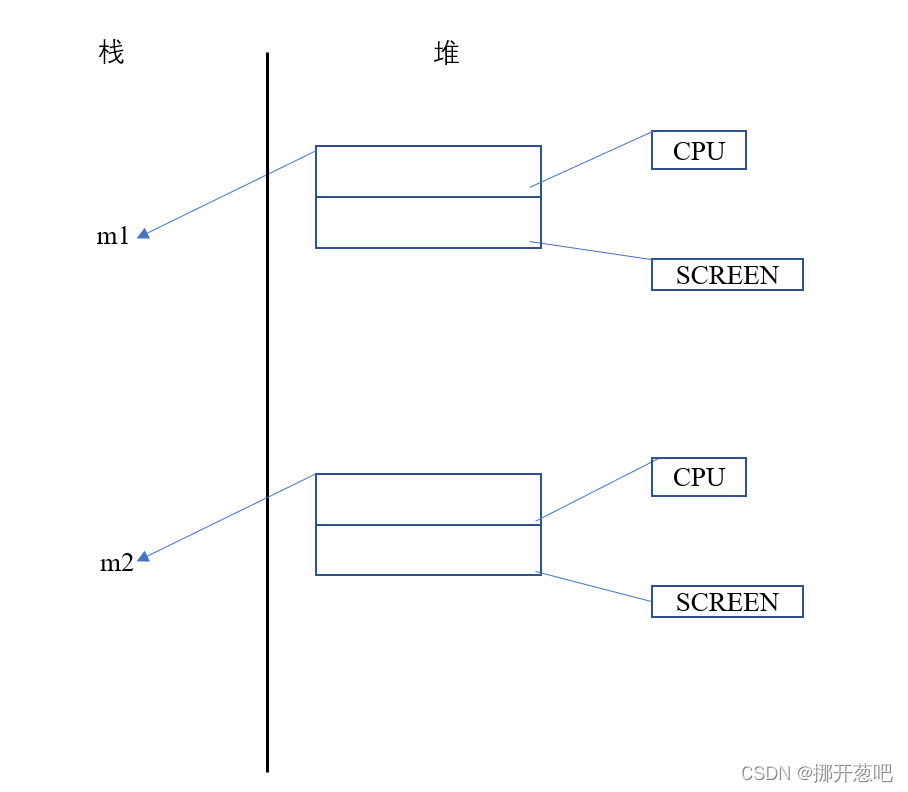
?
2. 定义发动机类 Motor、底盘类 Chassis、座椅类 Seat,车辆外壳类 Shell,并使用组合 关系定义汽车类。其他要求如下:
????????定义汽车的 run()方法,里面需要调用 Motor 类的 work()方法,也需要调用座椅 类 Seat 的 work()方法,也需要调用底盘类 Chassis 的 work()方法。
class Car():
def __init__(self,Motor,Chassis,Seat,Shell):
self.Motor = Motor
self.Chassis = Chassis
self.Seat = Seat
self.Shell = Shell
class Motor():
def work(self):
print('Motor is running')
class Chassis():
def work(self):
print('Chassis is running')
class Seat():
def work(self):
print('Seat is running')
class Shell():
def work(self):
print('Shell is running')
def CarRun():
m = Motor();c = Chassis();s1 = Seat();s2 =Shell()
c = Car(m,c,s1,s2)
c.Motor.work()
c.Chassis.work()
c.Seat.work()
c.Shell.work()
CarRun()
3. 使用工厂模式、单例模式实现如下需求:
(1) 电脑工厂类 ComputerFactory 用于生产电脑 Computer。工厂类使用单例模式, 也就是说只能有一个工厂对象。
(2) 工厂类中可以生产各种品牌的电脑:联想、华硕、神舟
(3) 各种品牌的电脑使用继承实现:
(4) 父类是 Computer 类,定义了 calculate 方法
(5) 各品牌电脑类需要重写父类的 calculate 方法
class ComputeFactory():
__obj = None # 私有类属性
__init_flag = True
def __new__(cls, *args, **kwargs):
if cls.__obj == None:
cls.__obj =object.__new__(cls)
return cls.__obj
def __init__(self):
if ComputeFactory.__init_flag:
print('单例初始化')
ComputeFactory.__init_flag = False
def ComputerProduce(self,kind):
self.kind = kind
if self.kind == '联想':
return LianXiang()
elif self.kind == '华硕':
return HuaShuo()
elif self.kind == '神舟':
return ShenZhou()
class Computer():
def calculate(self):
print('生产联想、华硕、神舟电脑的工厂')
class LianXiang(Computer):
def calculate(self):
print('生产联想电脑的工厂')
class HuaShuo(Computer):
def calculate(self):
print('生产华硕电脑的工厂')
class ShenZhou(Computer):
def calculate(self):
print('生产神舟电脑的工厂')
cf = ComputeFactory()
print(id(cf))
c1 = cf.ComputerProduce('联想')
c1.calculate()
cf = ComputeFactory()
print(id(cf))
c2 = cf.ComputerProduce('华硕')
c2.calculate()
cf = ComputeFactory()
print(id(cf))
c3 = cf.ComputerProduce('神舟')
c3.calculate()
运行结果如下:
单例初始化
1907473297160
生产联想电脑的工厂
1907473297160
生产华硕电脑的工厂
1907473297160
生产神舟电脑的工厂
4. 定义一个 Employee 雇员类,要求如下:
(1) 属性有:id、name、salary
(2) 运算符重载+:实现两个对象相加时,默认返回他们的薪水和
(3) 构造方法要求:输入 name、salary,不输入 id。id 采用自增的方式,从 1000 开 始自增,第一个新增对象是 1001,第二个新增对象是 1002。
(4) 根据 salary 属性,使用@property 设置属性的 get 和 set 方法。set 方法要求输入:1000-50000 范围的数字。
class Employee():
id = 999
def __init__(self,name,salary):
Employee.id += 1
self.name = name
self.__salary = salary
def __add__(self, other):
return self.salary + other.salary
@property
def salary(self):
return self.__salary
@salary.setter
def salary(self,salary):
if 1000 < salary < 50000:
self.__salary = salary#相当于只有正确的数据才会被传入到私有属性中,有一个缓存和隔离的中间变量
else:
print('录入错误')
a = [['张三',10000],['李四',20000],['王五',30000]]
e1 = Employee(a[0][0],a[0][1])
print('ID为{0}的姓名为:{1},他的薪资为:{2}'.format(e1.id,e1.name,e1.salary))
e2 = Employee(a[1][0],a[1][1])
print('ID为{0}的姓名为:{1},他的薪资为:{2}'.format(e2.id,e2.name,e2.salary))
e3 = Employee(a[2][0],a[2][1])
print('ID为{0}的姓名为:{1},他的薪资为:{2}'.format(e3.id,e3.name,e3.salary))
print('e1+e2={0}'.format(e1 + e2))
print('e1+e3={0}'.format(e1 + e3))
print('e2+e3={0}'.format(e2 + e3))
?运行结果为:
ID为1000的姓名为:张三,他的薪资为:10000
ID为1001的姓名为:李四,他的薪资为:20000
ID为1002的姓名为:王五,他的薪资为:30000
e1+e2=30000
e1+e3=40000
e2+e3=50000
|