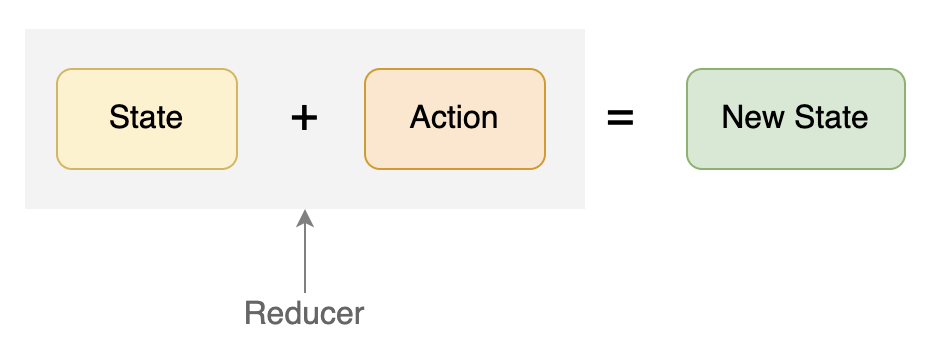
In Class Components
1. reducer
(state, action) => state 根据action.type判断,修改state
const initState = { count: 0 };
const counter = (state = initState, action) => {
switch (action.type) {
case "PLUS_ONE":
return { count: start.count + 1 };
case "CUSTOM_COUNT":
return { count: start.count + action.payload.count };
default:
break;
}
}
2. create store
createStore(reducer)
const store = createStore(counter);
3. action creator
function minusOne() {
return { type: "MINUS_ONE" };
}
4. map + connect
mapStateToProps(state) 之拿出来组件会用到的state
function mapStateToProps(state) {
return {
count: state.count
};
}
mapDispatchToProps(dispatch) 绑定action creator
function mapDispatchToProps(dispatch) {
bindActionCreators({ plusOne, minusOne }, dispatch);
}
connect(mapStateToProps, mapDispatchToProps)(Component) 用来创建高阶组件
const ConnectedCounter = connect(mapStateToProps, mapDispatchToProps)(Counter);
5. add provider in root
根结点有Provider即可,所有子节点均可访问store
import { Provider } from 'react-redux';
import store from './store';
export default class CounterSample extends React.Component {
render() {
<Provider store={store}>
<ConnectedCounter />
</Provider>
}
}
In Functional Components
1. add provider in root (similar to 5 above)
2. select + dispatch
import React from 'react'
import { useSelector, useDispatch } from 'react-redux'
export function Counter() {
const count = useSelector(state => state.value)
const dispatch = useDispatch()
return (
<div>
<button
onClick={() => dispatch({ type: 'counter/incremented' })}
>+</button>
<span>{count}</span>
<button
onClick={() => dispatch({ type: 'counter/decremented' })}
>-</button>
</div>
)
}
Reference: 极客时间《React实战进阶45讲》 & 极客时间《React Hooks核心原理与实战》
|