目录
一:什么使Redux?
二:Redux三大原则?
三:Redux组件有哪些?
四:使用
todolit案例:
一:什么使Redux?
Redux 是 JavaScript 状态容器,提供可预测化的状态管理。
二:Redux三大原则?
1:***单一事实来源:***整个应用的状态存储在单个 store 中的对象/状态树里。单一状态树可以更容易地跟踪随时间的变化,并调试或检查应用程序。 2:***状态是只读的:***改变状态的唯一方法是去触发一个动作。动作是描述变化的普通 JS 对象。就像 state 是数据的最小表示一样,该操作是对数据更改的最小表示。 3:***使用纯函数进行更改:***为了指定状态树如何通过操作进行转换,你需要纯函数。纯函数是那些返回值仅取决于其参数值的函数。
三:Redux组件有哪些?
- Action?– 这是一个用来描述发生了什么事情的对象。
- Reducer?– 这是一个确定状态将如何变化的地方。
- Store?– 整个程序的状态/对象树保存在Store中。
- View?– 只显示 Store 提供的数据。
四:使用
1:创建store
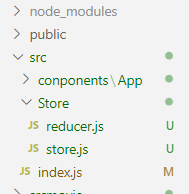
store.js
//引入createStore 专门用于创建redux中最为核心得store对象
import { createStore } from 'redux'
//引入reducer
import reducer from './reducer'
const store = createStore(reducer)
export default store
?reducer.js
//初始化状态
var initState = {
list: []
}
function reducer(state = initState, action) {
//根据type加工数据
switch (action.type) {
case "add":
var list = state.list
// console.log(action.data, 'shujui');
var obj = {
title: action.data,
edit: false,
done: false
}
list.push(obj)
return state
case "del":
var list = state.list
list.splice(action.data, 1)
return state
case "fx":
var list = state.list
list[action.data].done = !list[action.data].done
return state
case "allyidel":
var list = state.list
// console.log(action.data.list.length);
// console.log(action.data.list);
for (var i = action.data.list.length - 1; i >= 0; i--) {
// console.log(action.data.list[i].done);
if (action.data.list[i].done == true) {
list.splice(i, 1)
}
}
return state
case "allweidel":
var list = state.list
for (var i = action.data.list.length - 1; i >= 0; i--) {
if (action.data.list[i].done == false) {
list.splice(i, 1)
}
}
return state
case "kuang":
var list = state.list
list[action.data].edit = !list[action.data].edit
return state
case "gai":
var list = state.list
// console.log(action.data, '232222222222222523');
list.title = action.data
return state
case "okk":
var list = state.list
// console.log(action.data);
list[action.data].edit = !list[action.data].edit
return state
default:
return state
}
}
export default reducer
todolit案例:
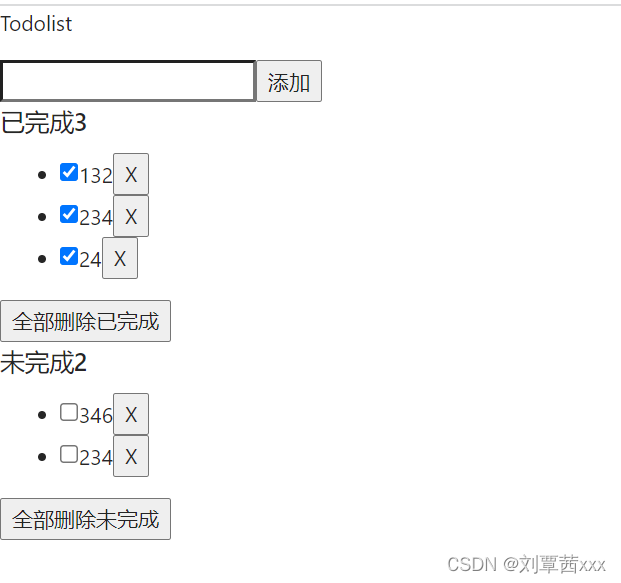
?
todo.js:给仓库传输数据
import React, { Component } from 'react'
import PropTypes from 'prop-types'
//引入store
import Store from '../../Store/store'
export default class Todo extends Component {
constructor(props) {
super(props)
this.state = {
todo: ''
}
}
static propTypes = {
}
//监听输入框变化
ch(e) {
// console.log(e.target.value);
this.setState({ todo: e.target.value })
}
//键盘事件
enter(e) {
// console.log(e.keyCode);
if (e.keyCode == 13) {
this.add()
}
}
//添加
add() {
//将数据传入reducer
Store.dispatch({ type: 'add', data: this.state.todo })
this.setState({ todo: "" })
}
render() {
return (
<div>
<p>Todolist</p>
<input type="text" value={this.state.todo} onChange={this.ch.bind(this)} onKeyUp={this.enter.bind(this)} />
<input type="button" value='添加' onClick={this.add.bind(this)} />
</div>
)
}
}
List.js 从仓库取数据
import React, { Component } from 'react'
import PropTypes from 'prop-types'
//引入store
import Store from '../../Store/store'
export default class List extends Component {
constructor(props) {
super()
//从仓库取数据
var list = Store.getState()
this.state = {
list
}
//把方法注册监听只要变化就调用
Store.subscribe(this.update.bind(this))
}
update() {
var list = Store.getState()
this.setState({ list: list })
}
//删除
del(i) {
//将数据传入reducer
Store.dispatch({ type: 'del', data: i })
}
//复选框
fx(i) {
Store.dispatch({ type: 'fx', data: i })
}
//全部删除已完成
allyidel() {
Store.dispatch({ type: 'allyidel', data: this.state.list })
}
//全部删除未完成
allweidel() {
Store.dispatch({ type: 'allweidel', data: this.state.list })
}
//已完成数量
yisum() {
let a = 0
this.state.list.list.map((item) => {
if (item.done) {
a++
}
})
return a
}
//未完成数量
weisum() {
let a = 0
this.state.list.list.map((item) => {
if (!item.done) {
a++
}
})
return a
}
//修改
kuang(i) {
Store.dispatch({ type: "kuang", data: i })
}
//修改输入框内容
gai(i, e) {
// console.log(i);
// console.log(e.target.value);
var list1 = this.state.list.list
list1[i].title = e.target.value
// console.log(list1[i].title);
Store.dispatch({ type: 'gai', data: list1[i].title })
}
//enter键盘事件
okk(i, e) {
// console.log(i);
// console.log(e.keyCode);
if (e.keyCode == 13) {
// console.log(1423);
Store.dispatch({ type: "okk", data: i })
}
}
static propTypes = {
}
render() {
return (
<div>
<h3>
已完成
<span>{this.yisum()}</span>
</h3>
<ul>
{
this.state.list.list.map((item, index) => {
if (item.done) {
return <li key={index}>
//复选框
<input type="checkbox" checked={item.done} onChange={this.fx.bind(this, index)} />
{/* 修改 */}
{
item.edit ?
<input type="text" value={item.title}
onChange={this.gai.bind(this, index)}
onKeyUp={this.okk.bind(this, index)}
/> :
<span onClick={this.kuang.bind(this, index)}>{item.title}</span>
}
<input type="button" value='X' onClick={this.del.bind(this, index)} />
</li>
}
})
}
</ul>
<input type="button" value='全部删除已完成' onClick={this.allyidel.bind(this)} />
<h3>
未完成
<span>{this.weisum()}</span>
</h3>
<ul>
{
this.state.list.list.map((item, index) => {
if (!item.done) {
return <li key={index}>
//复选框
<input type="checkbox" checked={item.done} onChange={this.fx.bind(this, index)} />
{/* 修改 */}
{
item.edit ?
<input type="text" value={item.title}
onChange={this.gai.bind(this, index)}
onKeyUp={this.okk.bind(this, index)}
/> :
<span onClick={this.kuang.bind(this, index)}>{item.title}</span>
}
<input type="button" value='X' onClick={this.del.bind(this, index)} />
</li>
}
})
}
</ul>
<input type="button" value='全部删除未完成' onClick={this.allweidel.bind(this)} />
</div>
)
}
}
|