Vue 使用指南
Element - The world’s most popular Vue UI framework
父子组件通信
传值 props & 传结构 slot 插槽
父组件
<c-or-e
@success="handleSuccess"
@cancel="dialogFormVisible = false"
:is-edit="isEdit"
:role-id="roleId"
v-if="dialogFormVisible"
>
<!-- 给子组件传的结构 -->
<!-- 如果需要用到子组件的数据,那么这样接收的是一个子组件传来的数据对象 -->
<template v-slot:header="dataObj">
<h1>头部组件{{dataObj.num}}</h1>
</template>
<template v-slot:default>
<p>content</p>
</template>
<template #footer="{value, num}">
<p>foot{{value}}</p>
<p>foot{{num}}</p>
</template>
</c-or-e>
子组件
<templete>
<header>
<!-- 给父组件传来的插槽,绑定值 但父组件将接收到一个对象,需要使用dataObj.num才能取到里面的值 -->
<slot name="header" :num="num"></slot>
</header>
<main>
<slot></slot>
</main>
<el-button @click="onCancel">取消</el-button>
<el-button type="primary" @click="onSubmit">确认</el-button>
<footer>
<slot name="footer" :num="num" :value="value"></slot>
</footer>
</templete>
<script>
export default {
name: 'COrE',
props: {
isEdit: {
type: Boolean,
default: false
},
roleId: {
type: [Number, String]
}
},
data () {
value: '给父组件传来的结构里面设计值',
number: 100
},
methods: {
onCancel () {
this.$emit('cancel')
this.form = {}
},
async onSubmit () {
const { data } = await getRoleSaveOrUpdate(this.form)
if (data.code === '000000') {
this.$emit('success')
this.$message.success('提交成功!')
this.form = {}
}
}
}
}
</script>
父组件设置 v-model
- 由子组件的 value 接收
- 默认被子组件的 input 方法触发,且将值传递给 v-model
<course-image v-model="courseInfo.courseImgUrl"></course-image>
export default {
name:"CourseImage",
props:{
value:{
type: String
}
},
methods: {
async handleUpload (options) {
const fd = new FormData()
fd.append('file',options.file)
const {data} = await uploadImg(fd)
if(data.code==="000000"){
this.$emit('input',data.data.name)
this.$message.success('上传成功')
}
}
}
父组件与「路由和子组件」的通信
-
父组件传给路由
<!-- scope.row 为作?域插槽提供的当前?数据 -->
<el-button
type="text"
@click="$router.push({
name: 'alloc-menu',
params: {
roleId: scope.row.id
},
query: {
lessonId: scope.row.lessonId
}
})">分配菜单
</el-button>
-
路由传给子组件
- 详见 Vue Router 阶段 -> 路径传参处理
{
path: '/role/:roleId/alloc-menu',
name: 'alloc-menu',
component: () => import('@/views/role/alloc-menu'),
props: true
},
- 子组件接收
- 接收路由传来的动态参数 接收到了这个roleId值。可以使用了。
props: {
roleId: {
type: [String, Number],
required: true
}
}
this.$route.query.lessonId
定位到某组件
<el-tree ref="menu-tree"></el-tree>
this.$refs['menu-tree']
this.$refs.menu-tree
<el-button @click="$refs['menu-tree'].setCheckedKeys([])">清空</el-button>
判断数据类型
在JavaScript中,如何判断数组是数组?
往数组增加内容
this.defaultKeys.push(menu.id)
this.defaultKeys = [...this.defaultKeys, menu.id]
filters使用
<template slot-scope="scope">
<span>{{ scope.row.createTime | dateFormat }}</span>
</template>
<script>
filters: {
dateFormat(date){
date = new Date(date)
return `${date.getFullYear()}-${date.getMonth()}-${date.getDate()}-${date.getHours()}:${date.getMinutes()}`}}
</script>
request
import request from '@/utils/requests'
export const getQueryCourses = (data) => {
return request({
method: 'POST',
url: '/boss/course/getQueryCourses',
data
})
}
export const changeState = (params) => {
return request({
method: 'GET',
url: '/boss/course/changeState',
params
})
}
export const getCourseById = (courseId) => {
return request({
method: 'GET',
url: '/boss/course/getCourseById',
params:{courseId}
})
}
对封装的 get 方法传递 params,进行调用
import {getQueryCourses,changeState} from '@/services/courses'
const {data} = await changeState({
courseId: row.id,
status: row.status
})
const {data} = await getCourseById(this.courseId)
上传请求进度检测 onUploadProgress axios
export const uploadImg = (data,onUploadProgress) => {
return request({
method: 'POST',
url: '/boss/course/upload',
data,
onUploadProgress
})
}
const {data} = await uploadImg(fd,event =>{
this.precentage = Math.floor(event.loaded/event.total*100)
})
插件使用 — 以富文本编辑器为例
常?的富?本编辑器有:(各有优缺点,建议优先根据维护性选择,相同时看喜好)
wangEditor - 轻量级 web 富文本编辑器
这?以 wangEditor 使?为例进?功能处理
wangEditor
npm 安装 npm i wangeditor --save ,几行代码即可创建一个编辑器。
import E from 'wangeditor'
const editor = new E('#div1')
editor.create()
methods:{
loadEditor () {
const editor = new E(this.$refs.editor)
editor.config.onchange = value => {
this.$emit('input',value)
}
editor.create()
editor.txt.html(this.value)
this.editor = editor
},
},
watch: {
value () {
if (!this.isLoaded){
this.editor.txt.html(this.value)
this.isLoaded = true
}
}
}
this
修改为箭头函数,内部 this 才能访问 Vue 实例
过滤器
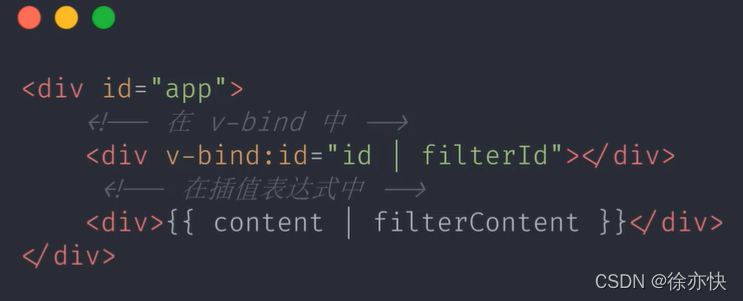
监听器
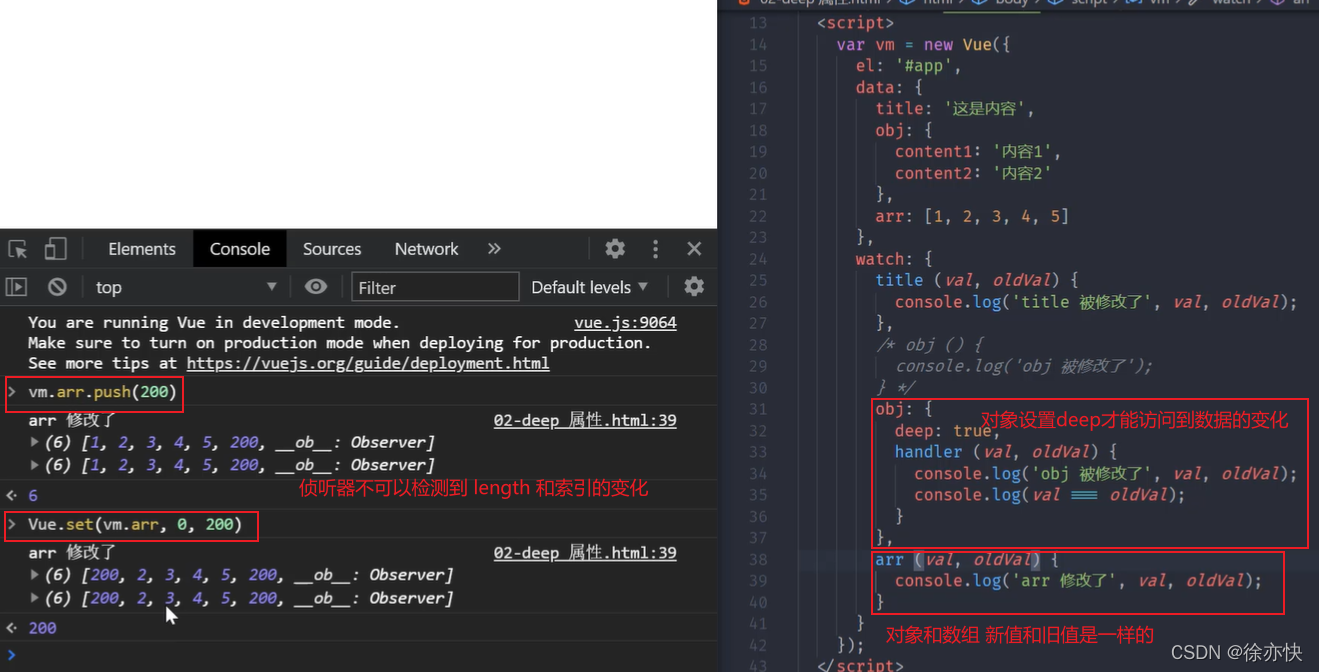
其他
my-components kebab-case
MyComponents PascalCase
用于设置组件的结构,最终通过自定义标签名的方式被引入根实例或其他组件中。
template标签是一个内容占位符,用来标记某一块内容用于统一处理。
子通过props属性接收使用驼峰命名,父传递使用kebab-case(html)。此为单向数据流,仅父传递子。(数组和对象除外)
若子组件没有通过props接受,这些属性会被自动绑定到子组件的根元素上,若已存在则会被替换,class和style会被合并。
props: {
myObject: {
type: Number,
default: 200,
required: true
}
}
props: {
myObject: {
type: [Array, Object],
default: function (){
return [1,2,3]
}
}
}
- 插槽
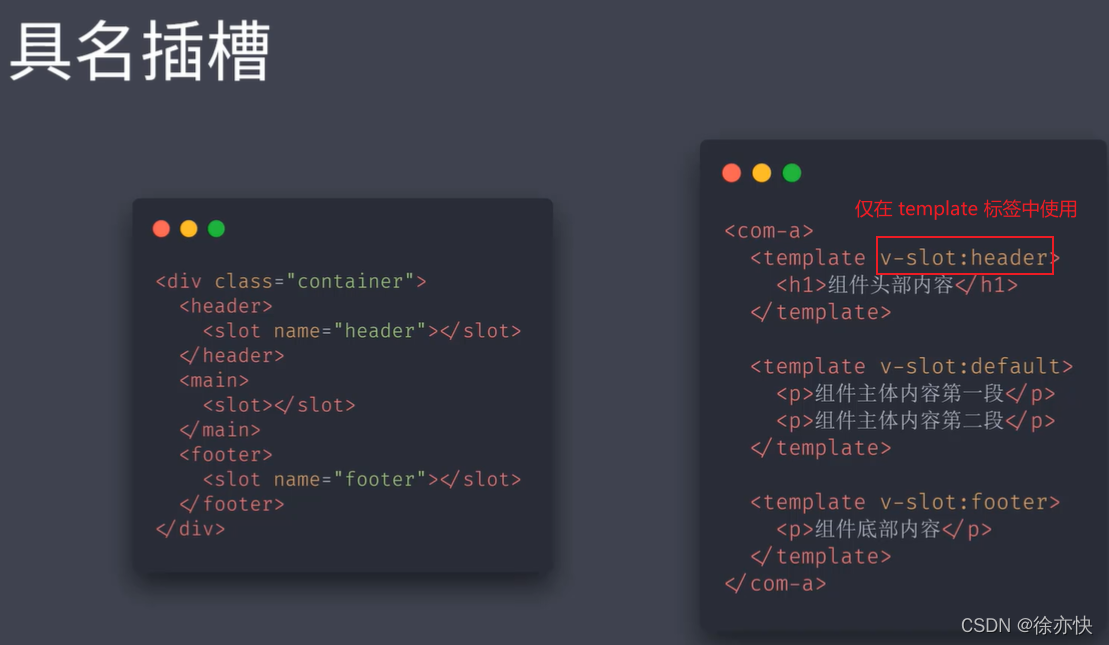
可以简写为: 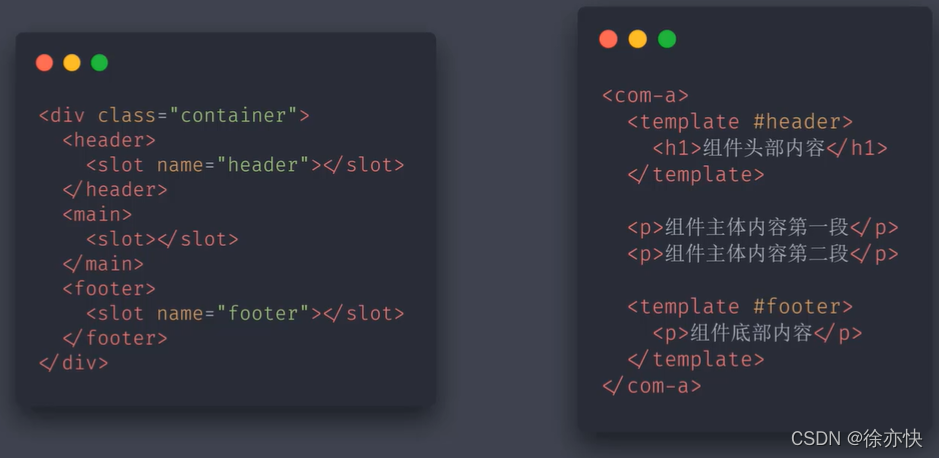
前端路由
style和class的绑定
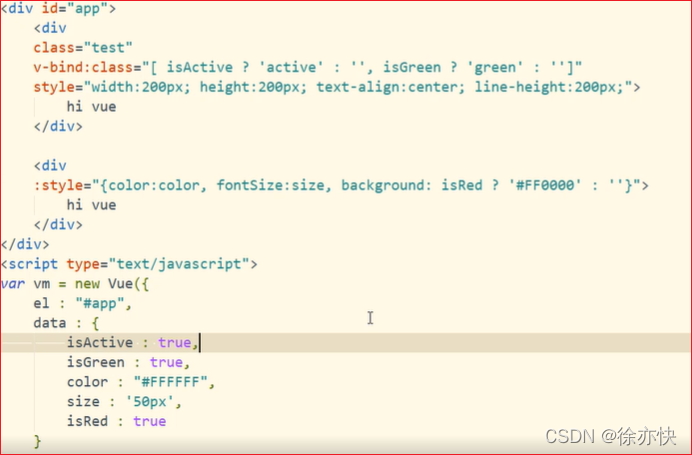
|