项目结构:
webpack-demo
|- package.json
|- package-lock.json
|- webpack.config.js
|- /dist
|- bundle.js
|- index.html
|- /src
|- index.js
|- /node_modules
修改webpack.config.js:
const path = require('path')
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist')
}
}
src/index.js:
import _ from 'lodash';
function component () {
const element = document.createElement('div');
element.innerHTML = _.join(['Hello', 'webpack'], ' ');
return element;
}
document.body.appendChild(component());
移除之前安装的依赖:
npm uninstall css-loader csv-loader json5 style-loader toml xml-loader yamljs
移除完成: 
准备工作
print.js
新建文件 src/print.js :
src/print.js:
export default function printFunx () {
console.log('This is print.js~');
}
项目结构:
webpack-demo
|- package.json
|- package-lock.json
|- webpack.config.js
|- /dist
|- /src
|- index.js
|- print.js
|- /node_modules
src/index.js
修改 src/index.js :
import _ from 'lodash';
import printFunx from './print.js'
function component () {
const element = document.createElement('div');
const btn = document.createElement('button')
element.innerHTML = _.join(['Hello', 'webpack'], ' ');
btn.innerHTML = 'Let click it!'
btn.onclick = printFunx
element.appendChild(btn)
return element;
}
document.body.appendChild(component());
dist/index.html
更新 dist/index.html 文件,为 webpack 分离入口做好准备:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>webpack demo - 管理输出</title>
<script src="./print.bundle.js"></script>
</head>
<body>
<script src="./index.bundle.js"></script>
</body>
</html>
调整了配置,将在 entry 添加 src/print.js 作为新的入口起点(print )。
webpack.config.js
修改 output,以便根据入口起点定义的名称,动态地产生 bundle 名称:
const path = require('path')
module.exports = {
entry: {
index: './src/index.js',
print: './src/print.js',
},
output: {
filename: '[name].bundle.js',
path: path.resolve(__dirname, 'dist')
}
}
执行 npm run build :
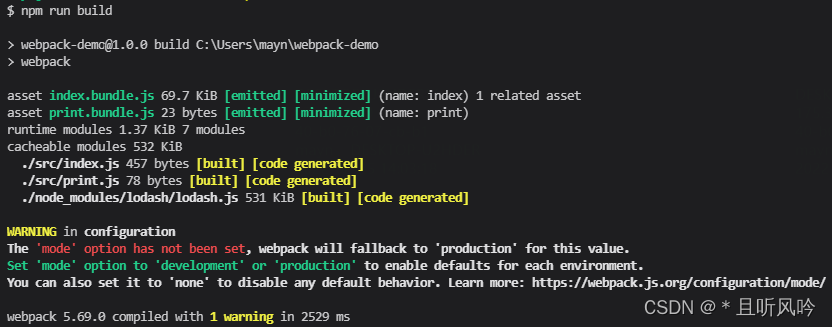
可以看到,webpack 生成 print.bundle.js 和 index.bundle.js 文件,这和在 index.html 文件中指定的文件名称相对应。
在浏览器中打开 index.html ,点击按钮,打印出了信息:
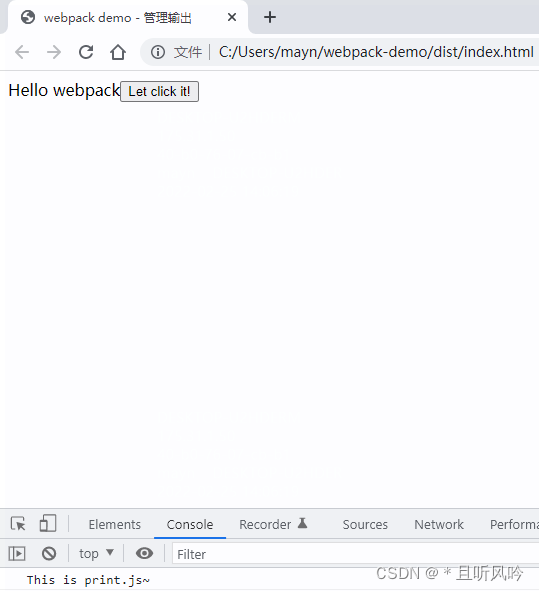
但是,如果更改了一个入口起点的名称,甚至添加了一个新的入口,会在构建时重新命名生成的 bundle,但是 index.html 文件仍然引用旧的名称。
接下来,用 HtmlWebpackPlugin 来解决这个问题。
设置 HtmlWebpackPlugin
安装插件
首先,安装插件:
npm install --save-dev html-webpack-plugin
安装完成: 
webpack.config.js
并且调整 webpack.config.js 文件:
const path = require('path')
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: {
index: './src/index.js',
print: './src/print.js',
},
plugins: [
new HtmlWebpackPlugin({
title: '管理输出',
}),
],
output: {
filename: '[name].bundle.js',
path: path.resolve(__dirname, 'dist')
}
}
执行 npm run build : 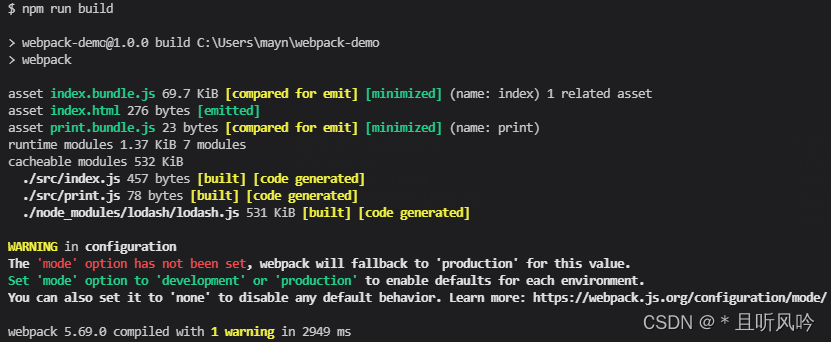
虽然在 dist/ 文件夹已经有了一个 index.html 这个文件,但是 HtmlWebpackPlugin 还是会默认生成它自己的 index.html 文件。
也就是说,用新生成的 index.html 文件,替换了原有的文件。
在代码编辑器中打开 index.html :
<!doctype html><html><head><meta charset="utf-8"><title>管理输出</title><meta name="viewport" content="width=device-width,initial-scale=1"><script defer="defer" src="index.bundle.js"></script><script defer="defer" src="print.bundle.js"></script></head><body></body></html>
可以看到, HtmlWebpackPlugin 创建了一个全新的文件,所有的 bundle 会自动添加到 html 中。
清理 /dist 文件夹
webpack 生成文件,并放置在 /dist 文件夹中,但是它不会追踪哪些文件是实际在项目中用到的。
由于遗留了之前的指南和代码示例, /dist 文件夹显得相当杂乱。
推荐做法:在每次构建前清理 /dist 文件夹,这样只会生成用到的文件。
现在,使用 output.clean 配置项实现这个需求。
webpack.config.js
修改webpack.config.js:
const path = require('path')
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: {
index: './src/index.js',
print: './src/print.js',
},
plugins: [
new HtmlWebpackPlugin({
title: '管理输出',
}),
],
output: {
filename: '[name].bundle.js',
path: path.resolve(__dirname, 'dist'),
clean: true
}
}
执行 npm run build : 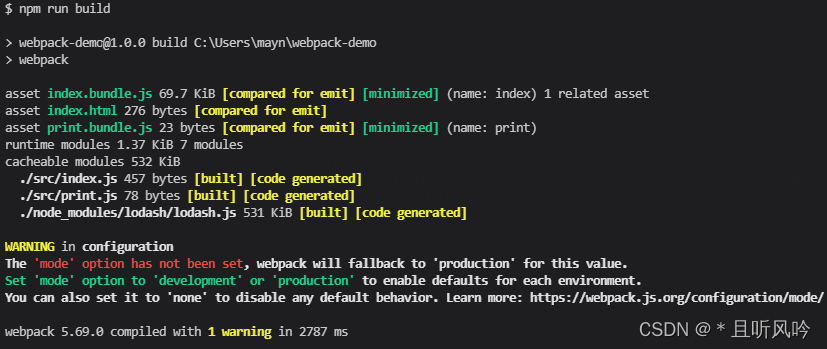
检查 /dist 文件夹,现在,只能看到构建后生成的文件,而没有了旧文件。
manifest
webpack 通过 manifest,可以追踪所有模块到输出 bundle 之间的映射。
|