## 构造函数
```javascript
function Parent(name, age) {
this.name = name
this.age = age
this.getName = function () {
alert(this.name)
}
}
class Parent {
constructor(name, age) {
this.name = name
this.age = age
}
getName() {
alert(this.name)
}
}
原型继承
把父类的实例作为子类的原型
缺点:子类的实例共享了父类构造函数的引用属性 不能传参
var parent = {
name: "lele",
hobby: ['a', 'b', 'c', 'l', 'w', 'l', 'o'],
age: 9999
}
var children = Object.create(parent)
children.name = 'lllll'
children.age = 055
children.hobby.push("aaa")
console.log(children);
console.log(parent);
组合继承
在子函数中运行父函数,但是要利用call把this改变一下,
再在子函数的prototype里面new Father() ,使Father的原型中的方法也得到继承,最后改变Son的原型中的constructor
缺点:调用了两次父类的构造函数,造成了不必要的消耗,父类方法可以复用
优点可传参,不共享父类引用属性
function Parent(name) {
this.name = name
this.getName = function () {
alert(this.name)
}
}
function Child(name, age) {
Parent.call(this, name)
this.age = age
}
Child.prototype = new Parent()
Child.prototype.constructor = Child
var a = new Child('lelle', 222)
a.getName()
寄生组合继承
function Parent(name) {
this.name = name
this.hobby = ['a', 'adsfs', 1111, 5555555555]
}
Parent.prototype.getName = function () {
alert(`${this.name},${this.hobby}`)
}
function child(name, age) {
Parent.call(this, name)
this.age = age
}
child.prototype = Object.create(Parent.prototype)
child.prototype.constructor = child
var a = new child('name', 99999999)
a.hobby.push('lllllllll')
a.getName()
console.log(Parent);
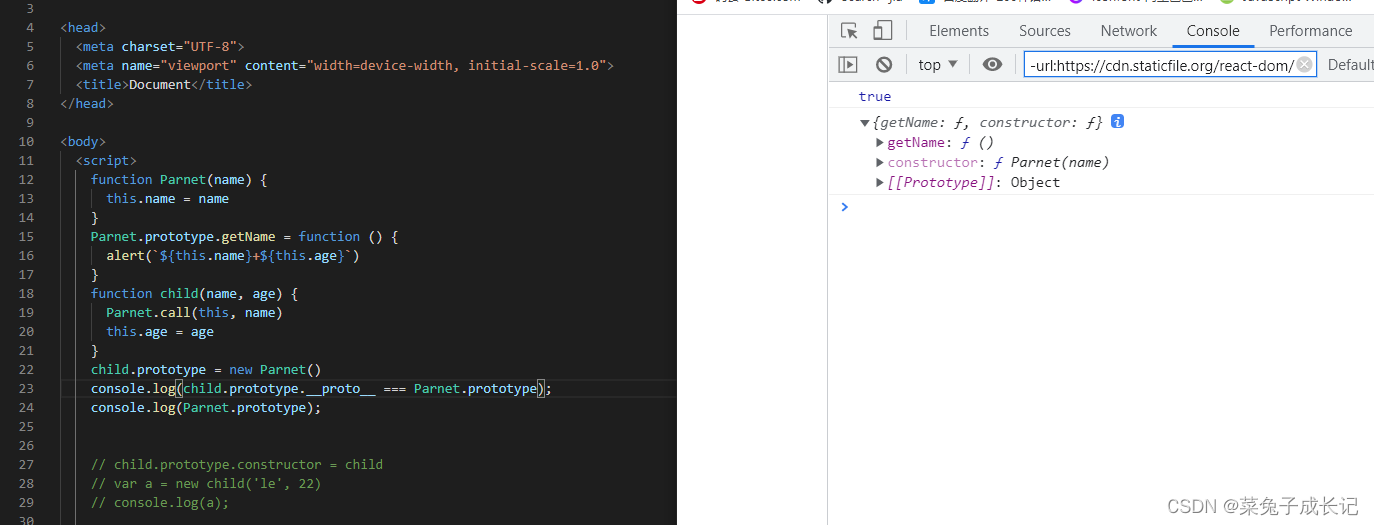
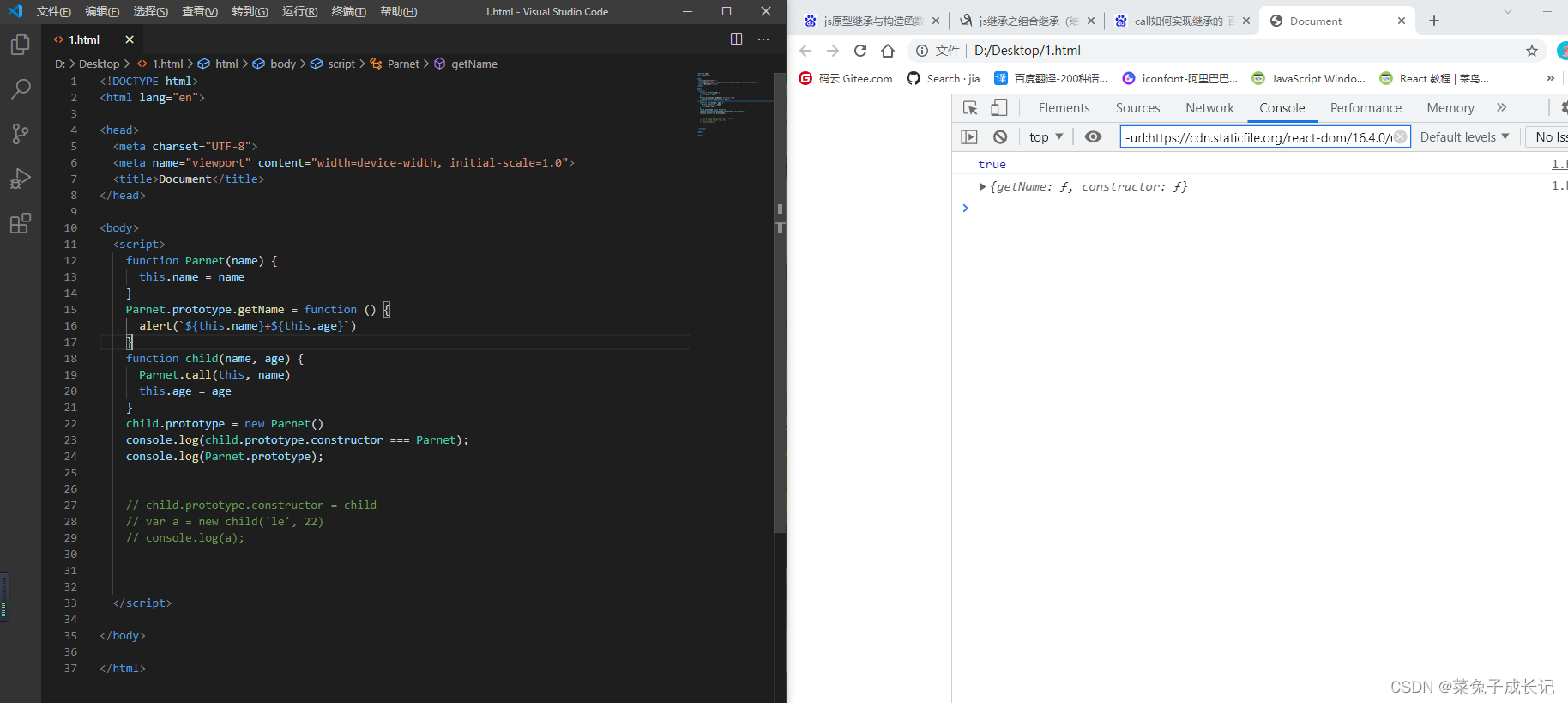
|