一.函数内this的指向
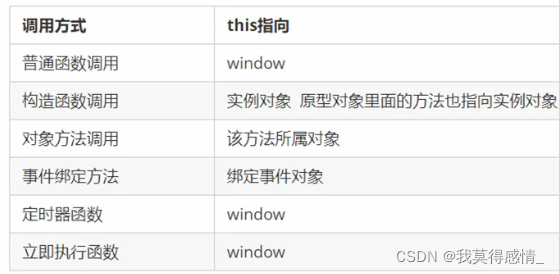
代码验证:
<body>
<button></button>
<script>
// 1.普通函数 this 指向window
function fn() {
console.log('普通函数的this' + this);
}
window.fn();
// 2.对象的方法 this 指向的是对象 o
var o = {
//方法
sayHi: function() {
console.log('对象方法的this:' + this);
}
}
o.sayHi()
// 3.构造函数 this 指向ldh这个实例对象 原型对象里面的this也是指向ldh这个实例对象
function Star() {};
// var ldh = new Star();
Star.prototype.sing = function() {}
// 4.绑定事件函数 this 指向的是函数的调用者 btn这个按钮对象
btn.onclick = function() {
console.log('绑定时间函数的this:' + this);
}; //点击按钮就可以调用
// 5.定时器函数 this 指向的也是 window
setInterval(function() {
console.log('定时器的this' + this);
}, 1000); //这个函数是定时器函数1秒钟调用一次
// 6.立即执行函数 (自动调用) this 指向的也是window
(function() {
console.log('立即执行函数this' + this);
})()
</script>
</body>
结果如下:
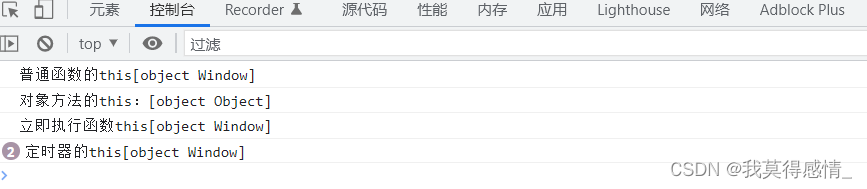
二.改变函数内部this的指向
1.call方法?
call第一个可以调用函数,第二个可以改变函数内的this指向
call的只要作用可以实现继承
<body>
<script>
var o = {
name: 'andy'
}
function fn(a, b) {
console.log(this);
console.log(a + b);
}
fn.call(o, 1, 2);
function Father(uname, age, sex) {
this.uname = uname;
this.age = age;
this.sex = sex;
}
function Son(uname, age, sex) {
Father.call(this, uname, age, sex);
}
var son = new Son('ldh', 18, '男');
console.log(son);
</script>
</body>
结果:
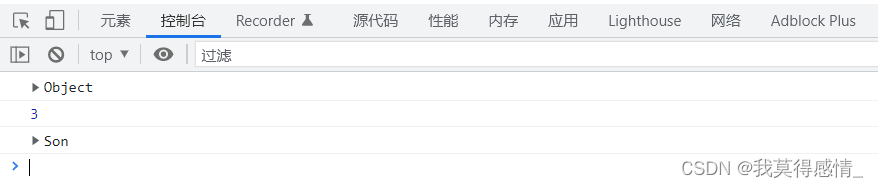
?2.apply方法
调用函数,改变函数内部的this指向
但是它的参数必须是数组(伪数组)
主要运用:利用apply借助数学内置对象求最大值
<body>
<script>
var o = {
name: 'andy'
}
function fn() {
console.log(this);
console.log(arr);
};
fn.apply(o, ['pink']);
var arr = [1, 66, 3, 99, 4];
var max = Math.max.apply(Math, arr);
console.log(max);
</script>
</body>
结果:
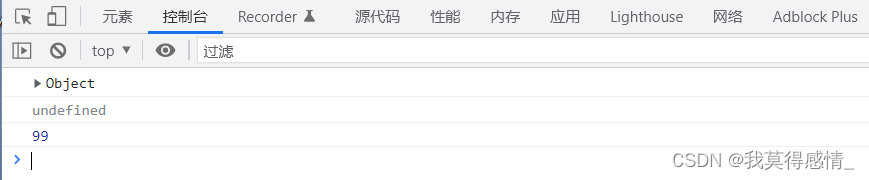
?3.bind方法
不会调用原来的函数 可以改变原来函数内部this指向
返回的是原函数改变this之后产生的新函数
如果有函数不需要立即调用,但又想改变这个函数内部this指向此时用bind
<body>
<button>点击</button>
<button>点击</button>
<button>点击</button>
<script>
var o = {
name: 'andy'
};
function fn(a, b) {
console.log(this);
console.log(a + b);
};
var f = fn.bind(o, 1, 2)
f();
var btns = document.querySelectorAll('button');
for (var i = 0; i < btns.length; i++) {
btns[i].onclick = function() {
this.disabled = true;
setTimeout(function() {
this.disabled = false;
}.bind(this), 2000);
}
}
</script>
</body>
结果:
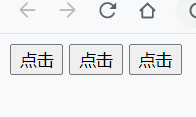
?最后,总结一下:
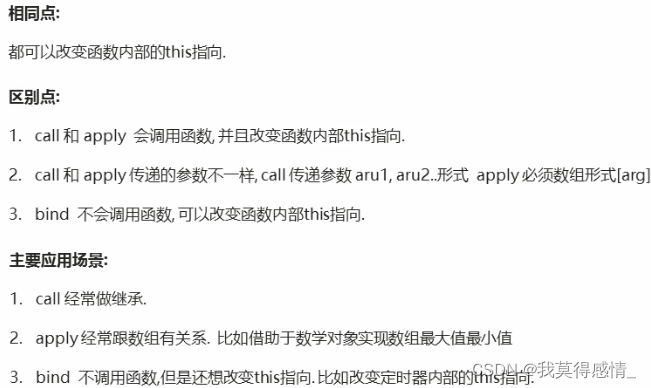
|