1.Installing via EMQX Docker Image
docker run -d --name emqx -p 1883:1883 -p 8081:8081 -p 8083:8083 -p 8084:8084 -p 8883:8883 -p 18083:18083 emqx/emqx:4.4.1
2.接下来可以访问 仪表盘(dashboard):
??????http://localhost:18083/
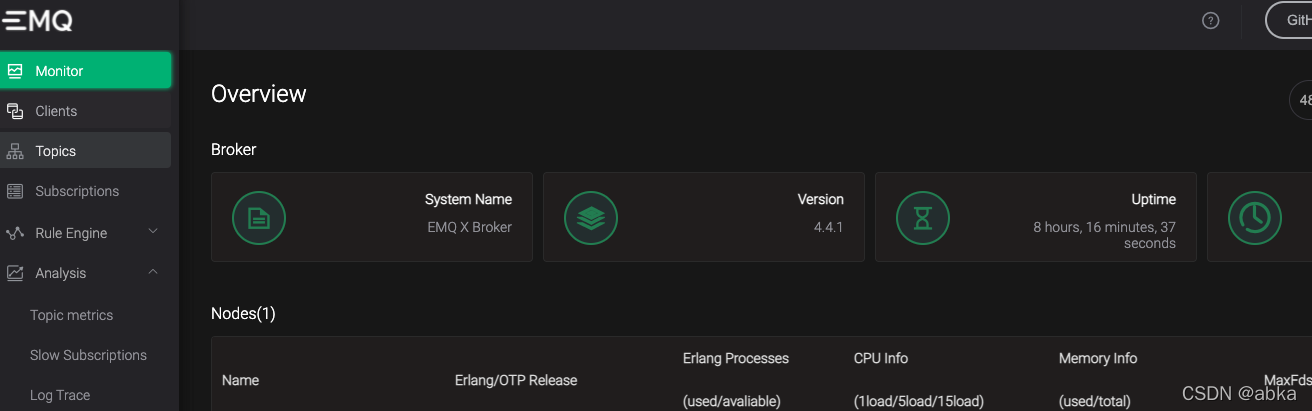
?可以在
etc/plugins/emqx_dashboard.conf
找到用户名密码:admin/public
##--------------------------------------------------------------------
## EMQ X Dashboard
##--------------------------------------------------------------------
## Default user's login name.
##
## Value: String
dashboard.default_user.login = admin
## Default user's password.
##
## Value: String
dashboard.default_user.password = public
Dashboard | EMQX Documentation
3. 安装mqtt库
访问mqtt 可以才有nodejs ,或者其他客户端,这里才有nodejs访问
npm install mqtt
4. 创建send_receiver.js
var mqtt = require("mqtt");
const clientId = 'mqttjs_' + Math.random().toString(16).substr(2, 8)
//const host = 'ws://broker.emqx.io:8083/mqtt'
const host = 'ws://localhost:8083/mqtt'
// const host = 'wss://localhost:8084/mqtt'
const topic = 'WillMsg'
const options = {
keepalive: 60,
clientId: clientId,
protocolId: 'MQTT',
protocolVersion: 4,
clean: true,
reconnectPeriod: 1000,
connectTimeout: 30 * 1000,
will: {
topic: topic,
payload: 'Connection Closed abnormally..!',
qos: 0,
retain: false
},
}
console.log('Connecting mqtt client')
const client = mqtt.connect(host, options)
console.log('Connecting mqtt client end')
client.on('connect',()=>{
console.log('Client connected:' + clientId)
// Subscribe
client.subscribe(topic, { qos: 0 })
})
client.on('error', (err) => {
console.log('Connection error: ', err)
client.end()
})
client.on('reconnect', () => {
console.log('Reconnecting...')
})
// Received
client.on('message', (topic, message, packet) => {
console.log('Received Message: ' + message.toString() + '\nOn topic: ' + topic)
})
5.创建receiver.js
var mqtt = require("mqtt");
const clientId = 'mqttjs_' + Math.random().toString(16).substr(2, 8)
//const host = 'ws://broker.emqx.io:8083/mqtt'
const host = 'ws://localhost:8083/mqtt'
// const host = 'wss://localhost:8084/mqtt'
const topic = 'WillMsg'
const options = {
keepalive: 60,
clientId: clientId,
protocolId: 'MQTT',
protocolVersion: 4,
clean: true,
reconnectPeriod: 1000,
connectTimeout: 30 * 1000,
will: {
topic: topic,
payload: 'Connection Closed abnormally..!',
qos: 0,
retain: false
},
}
console.log('Connecting mqtt client')
const client = mqtt.connect(host, options)
console.log('Connecting mqtt client end')
client.on('connect',()=>{
console.log('Client connected:' + clientId)
// Subscribe
client.subscribe(topic, { qos: 0 })
})
client.on('error', (err) => {
console.log('Connection error: ', err)
client.end()
})
client.on('reconnect', () => {
console.log('Reconnecting...')
})
// Received
client.on('message', (topic, message, packet) => {
console.log('Received Message: ' + message.toString() + '\nOn topic: ' + topic)
})
6.运行测试:
% node receiver.js
Debugger listening on ws://127.0.0.1:60111/099b6f06-dcae-4510-84eb-504d6babf056
For help, see: https://nodejs.org/en/docs/inspector
Debugger attached.
Connecting mqtt client
Connecting mqtt client end
Client connected:mqttjs_077f092a
运行send_receiver.js
% node send_receiver.js
Connecting mqtt client
Connecting mqtt client end
Client connected:mqttjs_077f092a
Received Message: After Client connected:mqttjs_ae0c3a83
On topic: WillMsg
client2 Received Message: After Client connected:mqttjs_ae0c3a83
On topic: WillMsg
% node recever.js
Debugger listening on ws://127.0.0.1:60111/099b6f06-dcae-4510-84eb-504d6babf056
For help, see: https://nodejs.org/en/docs/inspector
Debugger attached.
Connecting mqtt client
Connecting mqtt client end
Client connected:mqttjs_077f092a
Received Message: { "msg": "Hello, World41111!" }
On topic: WillMsg
Received Message: After Client connected:mqttjs_ae0c3a83
On topic: WillMsg
参考:
https://github.com/emqx/emqx
Dashboard | EMQX Documentation
Connect to MQTT broker with Websocket | EMQ
|