浏览器历史记录有两种写入方式:push 和replace 。 push 时将追加历史记录;replace 时将替换历史记录。路由跳转时默认是push 。
默认push追加历史记录
看具体例子吧,项目目录如下。 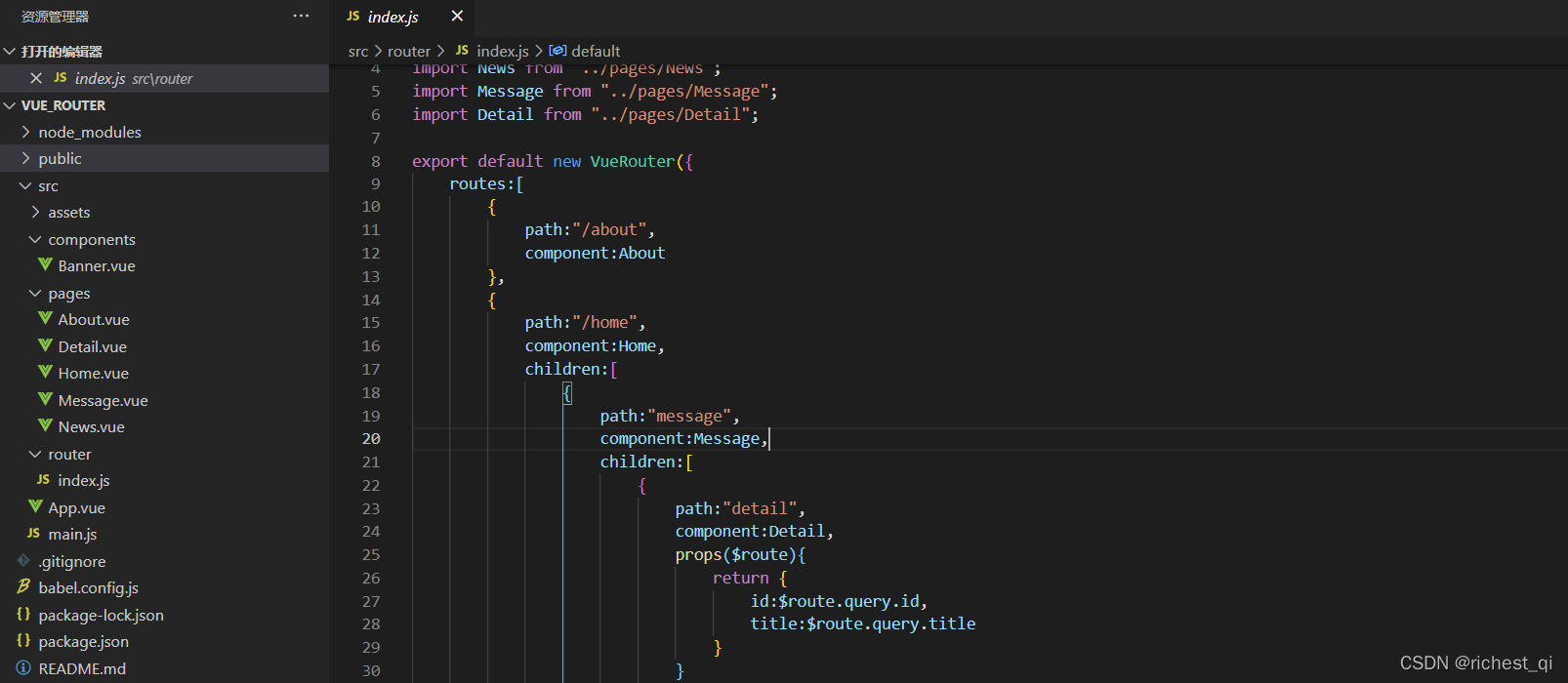
<template>
<div class="col-xs-offset-2 col-xs-8">
<div class="page-header"><h2>Vue Router Demo</h2></div>
</div>
</template>
<script>
export default {
name:"Banner"
}
</script>
<style>
</style>
<template>
<div>
<h2>Home组件内容</h2>
<div>
<ul class="nav nav-tabs">
<li>
<router-link class="list-group-item" active-class="active" to="/home/news">News</router-link>
</li>
<li>
<router-link class="list-group-item" active-class="active" to="/home/message">Message</router-link>
</li>
</ul>
<div>
<router-view></router-view>
</div>
</div>
</div>
</template>
<script>
export default {
name:'Home'
}
</script>
<style>
</style>
<template>
<h2>我是About的内容</h2>
</template>
<script>
export default {
name:'About'
}
</script>
<style>
</style>
<template>
<ul>
<li>news001</li>
<li>news002</li>
<li>news003</li>
</ul>
</template>
<script>
export default {
name:"News"
}
</script>
<style>
</style>
<template>
<div>
<ul>
<li v-for="message in messageList" :key="message.id">
<router-link :to="{
path:'/home/message/detail',
query:{
id:message.id,
title:message.title
}
}">
{{message.title}}
</router-link>
</li>
</ul>
<hr>
<router-view></router-view>
</div>
</template>
<script>
export default {
name:"Message",
data(){
return {
messageList:[
{id:"001",title:"消息01"},
{id:"002",title:"消息02"},
{id:"003",title:"消息03"},
]
}
}
}
</script>
<style>
</style>
<template>
<ul>
<li>消息编号:{{id}}</li>
<li>消息标题:{{title}}</li>
</ul>
</template>
<script>
export default {
name:"Detail",
props:['id','title']
}
</script>
<style>
</style>
import VueRouter from "vue-router";
import Home from "../pages/Home";
import About from "../pages/About";
import News from "../pages/News";
import Message from "../pages/Message";
import Detail from "../pages/Detail";
export default new VueRouter({
routes:[
{
path:"/about",
component:About
},
{
path:"/home",
component:Home,
children:[
{
path:"message",
component:Message,
children:[
{
path:"detail",
component:Detail,
props($route){
return {
id:$route.query.id,
title:$route.query.title
}
}
}
]
},
{
path:"news",
component:News
}
]
}
]
})
<template>
<div>
<div class="row">
<Banner/>
</div>
<div class="row">
<div class="col-xs-2 col-xs-offset-2">
<div class="list-group">
<router-link to="/about" active-class="active" class="list-group-item">About</router-link>
<router-link to="/home" active-class="active" class="list-group-item">Home</router-link>
</div>
</div>
<div class="col-xs-6">
<div class="panel">
<div class="panel-body">
<router-view></router-view>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
import Banner from "./components/Banner.vue";
export default {
name: 'App',
components:{
Banner
}
}
</script>
import Vue from 'vue'
import App from './App.vue'
import VueRouter from "vue-router";
import router from "./router/index";
Vue.config.productionTip = false;
Vue.use(VueRouter);
new Vue({
render: h => h(App),
router:router
}).$mount('#app')
- 启动应用,测试效果。
- http://localhost:8080/#/
浏览器历史记录有: http://localhost:8080/#/
- http://localhost:8080/#/about
浏览器历史记录有: http://localhost:8080/#/ http://localhost:8080/#/about
- http://localhost:8080/#/home
浏览器历史记录有: http://localhost:8080/#/ http://localhost:8080/#/about http://localhost:8080/#/home
- http://localhost:8080/#/home/news
浏览器历史记录有: http://localhost:8080/#/ http://localhost:8080/#/about http://localhost:8080/#/home http://localhost:8080/#/home/news
- 点
← 回退,回到 http://localhost:8080/#/home
浏览器历史记录有: http://localhost:8080/#/ http://localhost:8080/#/about http://localhost:8080/#/home
- 继续点
← 回退,回到 http://localhost:8080/#/about
浏览器历史记录有: http://localhost:8080/#/ http://localhost:8080/#/about
- 继续点
← 回退,回到 http://localhost:8080/#/,此时← 置灰,不可回退
routerLink的replace属性将替换历史记录
- 修改App.vue,给routerLink添加replace属性:
replace 或者:replace="true" 均可。
<template>
<div>
<div class="row">
<Banner/>
</div>
<div class="row">
<div class="col-xs-2 col-xs-offset-2">
<div class="list-group">
<router-link replace to="/about" active-class="active" class="list-group-item">About</router-link>
<router-link replace to="/home" active-class="active" class="list-group-item">Home</router-link>
</div>
</div>
<div class="col-xs-6">
<div class="panel">
<div class="panel-body">
<router-view></router-view>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
import Banner from "./components/Banner.vue";
export default {
name: 'App',
components:{
Banner
}
}
</script>
- 启动应用,测试效果。
- http://localhost:8080/#/
浏览器历史记录有: http://localhost:8080/#/
- http://localhost:8080/#/about
浏览器历史记录有: http://localhost:8080/#/about
- http://localhost:8080/#/home
浏览器历史记录有: http://localhost:8080/#/home
- http://localhost:8080/#/home/news
浏览器历史记录有: http://localhost:8080/#/home http://localhost:8080/#/home/news
- 点
← 回退,回到 http://localhost:8080/#/home ,此时← 置灰,不可回退。
|