准备工作
文件夹
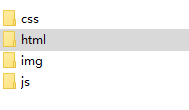
图片
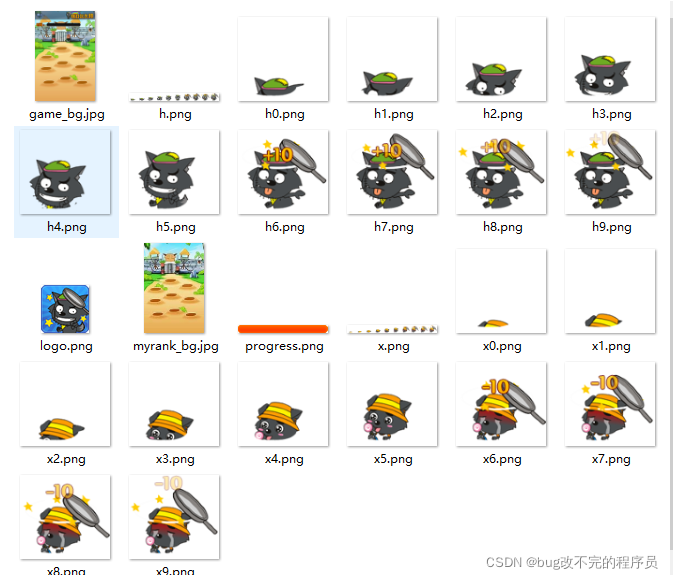
页面搭建
开始页面效果
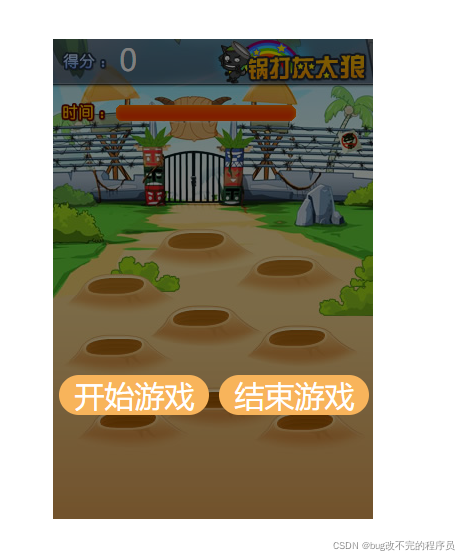
结束效果
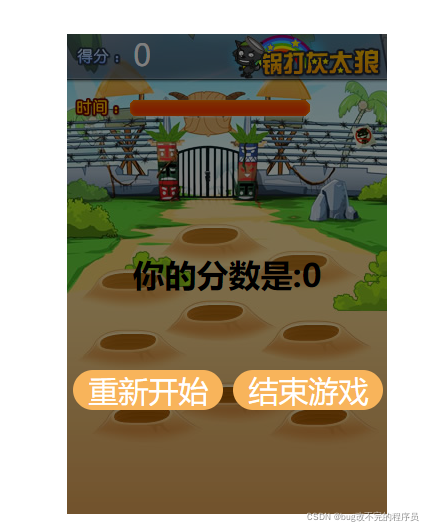
**html部分 **
<div class="war">
<div class="score">0</div>
<div class="showScore">
<h1>你的分数是:<span></span></h1>
</div>
<div class="progress"></div>
<div class="game"></div>
<div class="btns">
<div class="start">开始游戏</div>
<div class="gameOver">结束游戏</div>
<div class="reStart">重新开始</div>
</div>
<div class="difficulty">
<div class="easy">简单</div>
<div class="secondary">中等</div>
<div class="difficult">困难</div>
</div>
<div class="mask"></div>
</div>
美化工作 css
* {
margin: 0;
padding: 0;
}
.war {
width: 320px;
height: 480px;
background-image: url(../img/game_bg.jpg);
margin: 100px auto;
position: relative;
}
.start,
.reStart,
.gameOver {
position: absolute;
background-color: rgb(248, 180, 91);
width: 150px;
line-height: 40px;
left: 2%;
font-size: 30px;
color: #fff;
top: 70%;
text-align: center;
border-radius: 20px;
z-index: 9999;
}
.reStart {
display: none;
}
.gameOver {
margin-left: 160px;
}
.score {
width: 50px;
line-height: 40px;
text-align: center;
color: #fff;
font-size: 30px;
position: absolute;
left: 50px;
}
.game {
height: 100%;
}
.progress {
width: 180px;
height: 16px;
background-image: url(../img/progress.png);
position: absolute;
background-repeat: no-repeat;
top: 66px;
left: 63px;
}
.difficulty {
display: none;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
z-index: 9999;
}
.easy,
.secondary,
.difficult {
background-color: rgb(248, 180, 91);
width: 150px;
line-height: 40px;
font-size: 30px;
color: #fff;
text-align: center;
border-radius: 20px;
margin-bottom: 20px;
}
.showScore {
position: absolute;
top: 50%;
width: 100%;
text-align: center;
line-height: 50px;
transform: translateY(-50%);
display: none;
}
.mask {
width: 100%;
height: 100%;
position: absolute;
top: 0;
z-index: 1;
background-color: rgba(0, 0, 0, 0.5);
}
逻辑部分 js
var mask = document.querySelector('.mask');
var gameOver = document.querySelector('.gameOver');
var easy = document.querySelector('.easy');
var secondary = document.querySelector('.secondary');
var difficult = document.querySelector('.difficult');
var difficulty = document.querySelector('.difficulty');
var dis = document.querySelectorAll('.difficulty>div');
var start = document.querySelector('.start');
var score = document.querySelector('.score');
var reStart = document.querySelector('.reStart');
var progress = document.querySelector('.progress');
var showScore = document.querySelector('.showScore');
var game = document.querySelector('.game');
var w = null;
var flag = true;
var speed = 0;
var time = 0;
start.onclick = function () {
this.style.display = 'none';
difficulty.style.display = 'block';
gameOver.style.display = 'none';
mask.style.display = 'none';
}
reStart.onclick = function () {
this.style.display = 'none';
gameOver.style.display = 'none';
showScore.style.display = 'none'
score.innerText = 0;
difficulty.style.display = 'block';
mask.style.display = 'none';
}
gameOver.onclick = function () {
reStart.style.display = 'none';
start.style.display = 'block';
showScore.style.display = 'none';
score.innerText = 0;
}
dis.forEach(function (val, index) {
val.onclick = function () {
difficulty.style.display = 'none';
if (index == 0) {
speed = myRandom(80, 100);
time = myRandom(100, 400);
} else if (index == 1) {
speed = myRandom(30, 50);
time = myRandom(150, 300);
} else if (index == 2) {
speed = myRandom(10, 30);
time = myRandom(80, 100);
}
progressBar(speed);
startAnimate(time);
}
})
思路:当点击开始游戏按钮后, 提示选择难度 ,难度不同对应的时间和速度也不同,选择其中一个难度,进度条渐渐变短,当进度条长度变为0的时候灰太狼停止运动,并且游戏也结束;当进度条逐渐减少的时候灰太狼或小灰灰冒出来,当点击灰太狼时,进度条长度加5px ,并且得分增加10分 ;当点击小灰灰时,进度条长度不变,分数减少10分 。当点击重新开始游戏时,分数归零,并且进度条恢复原先长度。当点击结束游戏时,和结束游戏效果一致。游戏结束时,进度条恢复。
完整代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
.war {
width: 320px;
height: 480px;
background-image: url(../img/game_bg.jpg);
margin: 100px auto;
position: relative;
}
.start,
.reStart,
.gameOver {
position: absolute;
background-color: rgb(248, 180, 91);
width: 150px;
line-height: 40px;
left: 2%;
font-size: 30px;
color: #fff;
top: 70%;
text-align: center;
border-radius: 20px;
z-index: 9999;
}
.reStart {
display: none;
}
.gameOver {
margin-left: 160px;
}
.score {
width: 50px;
line-height: 40px;
text-align: center;
color: #fff;
font-size: 30px;
position: absolute;
left: 50px;
}
.game {
height: 100%;
}
.progress {
width: 180px;
height: 16px;
background-image: url(../img/progress.png);
position: absolute;
background-repeat: no-repeat;
top: 66px;
left: 63px;
}
.difficulty {
display: none;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
z-index: 9999;
}
.easy,
.secondary,
.difficult {
background-color: rgb(248, 180, 91);
width: 150px;
line-height: 40px;
font-size: 30px;
color: #fff;
text-align: center;
border-radius: 20px;
margin-bottom: 20px;
}
.showScore {
position: absolute;
top: 50%;
width: 100%;
text-align: center;
line-height: 50px;
transform: translateY(-50%);
display: none;
}
.mask {
width: 100%;
height: 100%;
position: absolute;
top: 0;
z-index: 1;
background-color: rgba(0, 0, 0, 0.5);
}
</style>
</head>
<body>
<div class="war">
<div class="score">0</div>
<div class="showScore">
<h1>你的分数是:<span></span></h1>
</div>
<div class="progress"></div>
<div class="game"></div>
<div class="btns">
<div class="start">开始游戏</div>
<div class="gameOver">结束游戏</div>
<div class="reStart">重新开始</div>
</div>
<div class="difficulty">
<div class="easy">简单</div>
<div class="secondary">中等</div>
<div class="difficult">困难</div>
</div>
<div class="mask"></div>
</div>
</body>
<script>
var mask = document.querySelector('.mask');
var gameOver = document.querySelector('.gameOver');
var easy = document.querySelector('.easy');
var secondary = document.querySelector('.secondary');
var difficult = document.querySelector('.difficult');
var difficulty = document.querySelector('.difficulty');
var dis = document.querySelectorAll('.difficulty>div');
var start = document.querySelector('.start');
var score = document.querySelector('.score');
var reStart = document.querySelector('.reStart');
var progress = document.querySelector('.progress');
var showScore = document.querySelector('.showScore');
var game = document.querySelector('.game');
var w = null;
var flag = true;
var speed = 0;
var time = 0;
start.onclick = function () {
this.style.display = 'none';
difficulty.style.display = 'block';
gameOver.style.display = 'none';
mask.style.display = 'none';
}
reStart.onclick = function () {
this.style.display = 'none';
gameOver.style.display = 'none';
showScore.style.display = 'none'
score.innerText = 0;
difficulty.style.display = 'block';
mask.style.display = 'none';
}
gameOver.onclick = function () {
reStart.style.display = 'none';
start.style.display = 'block';
showScore.style.display = 'none';
score.innerText = 0;
}
dis.forEach(function (val, index) {
val.onclick = function () {
difficulty.style.display = 'none';
if (index == 0) {
speed = myRandom(80, 100);
time = myRandom(100, 400);
} else if (index == 1) {
speed = myRandom(30, 50);
time = myRandom(150, 300);
} else if (index == 2) {
speed = myRandom(10, 30);
time = myRandom(80, 100);
}
progressBar(speed);
startAnimate(time);
}
})
function progressBar(speed) {
var speed = speed;
var timer = setInterval(function () {
w = progress.offsetWidth;
if (w <= 0) {
progress.style.width = '180px'
reStart.style.display = 'block';
gameOver.style.display = 'block';
mask.style.display = 'block';
showScore.style.display = 'block'
showScore.querySelector('span').innerText = score.innerText;
clearInterval(timer);
stopAnimate(time)
}
w--
progress.style.width = w + 'px';
}, speed);
}
var timer = null;
function startAnimate(time) {
var time = time;
var imgArr = [
'../img/h0.png', '../img/h1.png', '../img/h2.png', '../img/h3.png', '../img/h4.png', '../img/h5.png',
'../img/h6.png', '../img/h7.png', '../img/h8.png', '../img/h9.png',
]
var imgArr2 = [
'../img/x0.png', '../img/x1.png', '../img/x2.png', '../img/x3.png', '../img/x4.png', '../img/x5.png',
'../img/x6.png', '../img/x7.png', '../img/x8.png', '../img/x9.png',
]
var img = document.createElement('img');
img.style.position = 'absolute';
var arrPos = [{
left: "100px",
top: "115px"
},
{
left: "20px",
top: "160px"
},
{
left: "190px",
top: "142px"
},
{
left: "105px",
top: "193px"
},
{
left: "19px",
top: "221px"
},
{
left: "202px",
top: "212px"
},
{
left: "120px",
top: "275px"
},
{
left: "30px",
top: "295px"
},
{
left: "209px",
top: "297px"
}
]
var pit = Math.round(Math.random() * 8);
img.style.left = arrPos[pit].left;
img.style.top = arrPos[pit].top;
var type = Math.round(Math.random() * 100) >= 30 ? imgArr : imgArr2;
i = 0;
indexEnd = 5;
num = 0;
timer = setInterval(function () {
if (num >= indexEnd) {
i--;
if (i <= 0) {
clearInterval(timer);
img.remove();
startAnimate(time);
}
} else {
i++;
num++;
}
img.setAttribute('src', type[i]);
}, time);
game.appendChild(img);
myScore(img);
}
function myScore(ele) {
flag = true;
ele.onclick = function () {
if (flag) {
num = 5;
i = 5;
indexEnd = 9;
var src = this.getAttribute('src');
var wolf = src.indexOf('h');
if (wolf >= 0) {
w += 5;
progress.style.width = w + 'px';
score.innerText = parseInt(score.innerText) + 10;
} else {
score.innerText = parseInt(score.innerText) - 10;
}
flag = false;
}
}
}
function stopAnimate() {
var img = game.querySelectorAll('img');
img.forEach(function (val) {
val.remove();
})
clearInterval(timer);
}
function myRandom(min, max) {
return Math.round(Math.random() * (max - min) + min);
}
</script>
</html>
|