实战案例:表白墙
用CSS,HTML和JS中一些比较基础 的知识设计一个简单 的表白墙,其中css样式比较简单,有兴趣的同学可发挥想象自行修改。表白墙发送的内容会以div标签的方式生成在下方。
注意:该表白墙上的数据并不具备持久性,只能保存在当前网页中,一旦页面刷新或者关闭了,这里的数据就没了。如果想要长久的保存数据,可以将这些数据提交到服务器上,然后由服务器将数据储存在文件或者数据库中。但本文介绍的是和JS前端等有关的 知识,数据库或者其他知识这里不展开了
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div class="container">
<!-- 设置标题-->
<h1> 表白墙</h1>
<p>输入后点击提交,会将信息显示在墙上</p>
<div class="row">
<span>谁</span>
<input type="text" class="edit">
</div>
<div class="row">
<span>对谁</span>
<input type="text" class="edit">
</div>
<div class="row">
<span>说什么</span>
<input type="text" class="edit">
</div>
<div>
<input type="button" value="提 交" id="submit">
</div>
<!-- 每次点击提交,都会在下面新增一个row,里面放置用户输入的话-->
</div>
<script>
let submitButton=document.querySelector('#submit');
submitButton.onclick=function () {
let edits=document.querySelectorAll('.edit');
let from=edits[0].value;
let to=edits[1].value;
let message=edits[2].value;
console.log(from+"对"+to+"说"+message);
if(from =='' || to=='' || message==''){
return;
}
let row=document.createElement('div');
row.className = 'row';
row.innerHTML=from+' 对 '+to+' 说 '+message;
let container=document.querySelector('.container');
container.appendChild(row);
for(let i=0;i<edits.length;i++){
edits[i].value='';
}
}
</script>
<style>
* {
margin:0;
padding: 0;
box-sizing: border-box;
}
.container{
width: 400px;
margin: 0 auto;
}
h1{
text-align: center;
padding: 20px 0;
color:red;
}
p{
text-align: center;
color:#666;
padding: 10px 0;
font-size: 14px;
}
.row{
height: 50px;
display: flex;
justify-content: center;
align-items: center;
}
span{
width: 90px ;
font-size: 20px;
color: black;
}
input{
width: 310px;
height: 40px;
}
#submit{
width: 400px;
color: white;
background-color: orange;
border: none;
border-radius: 14px;
font-size: 18px;
}
#submit:active{
background-color: blue;
}
.edit{
font-size: 18px;
padding-left: 5px;
}
</style>
</body>
</html>
运行效果: 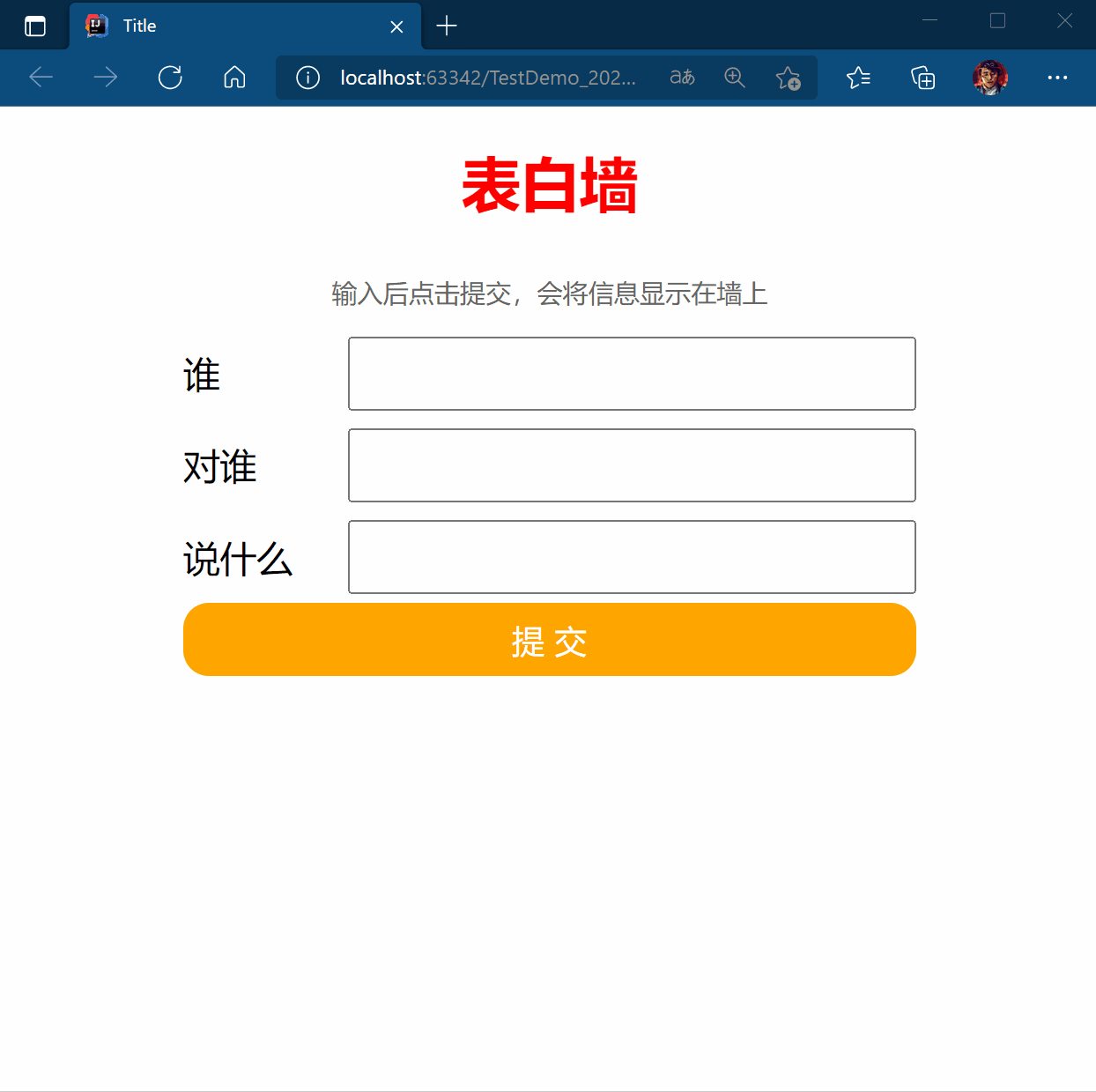
备忘录
效果图:
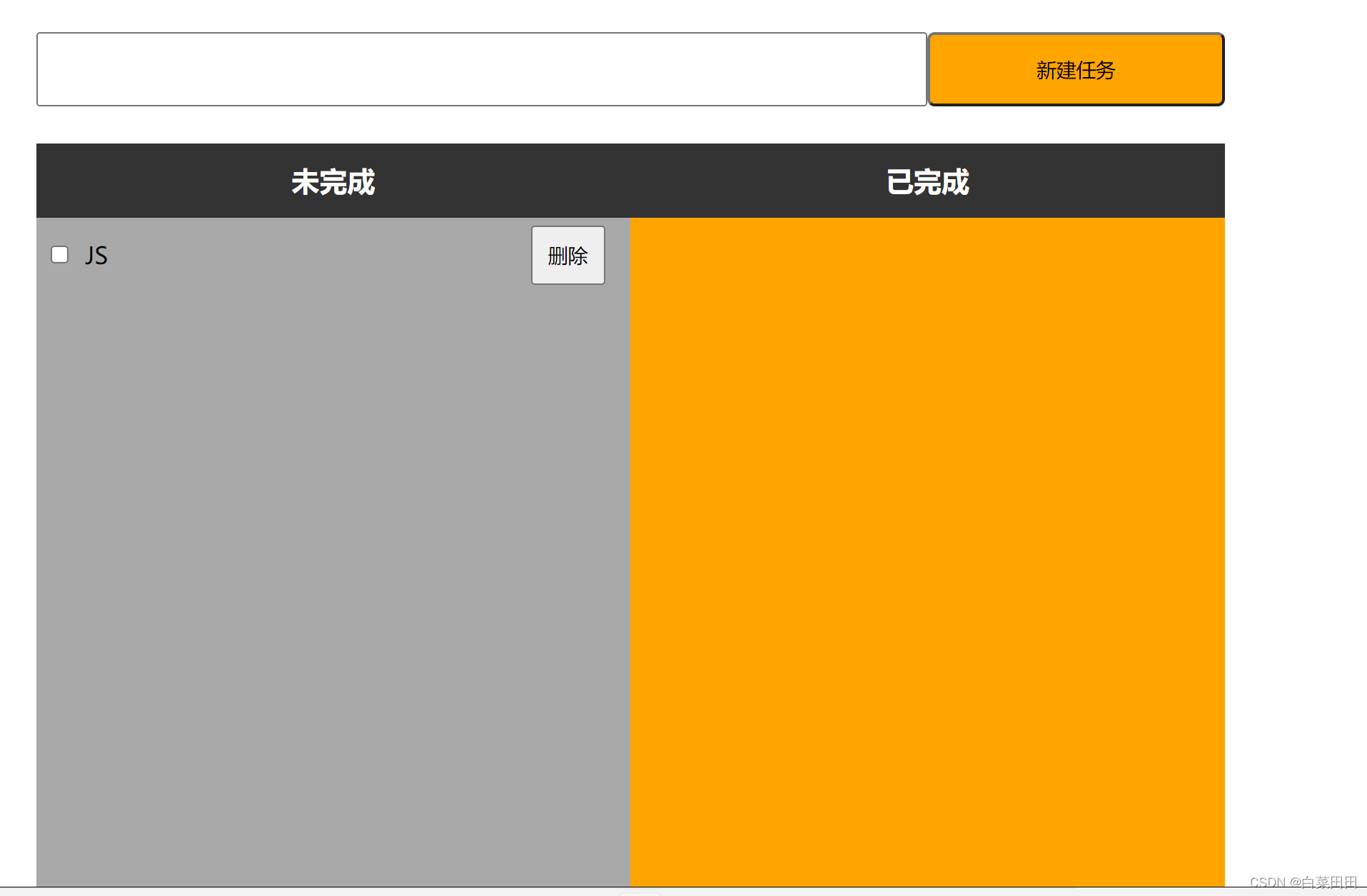
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>备忘录</title>
</head>
<body>
<!--创建输入框和新建任务按钮-->
<div class="nav">
<input type="text">
<button>新建任务</button>
</div>
<!-- 下面包含了左右两个内容区域 -->
<div class="container">
<!-- 左侧结构 -->
<div class="todo">
<h3>未完成</h3>
<!-- 举例子 -->
<!-- <div class="row">-->
<!-- <input type="checkbox">-->
<!-- <span>吃饭</span>-->
<!-- <button>删除</button>-->
<!-- </div>-->
</div>
<!-- 右侧结构 -->
<div class="done">
<h3>已完成</h3>
</div>
</div>
<style>
*{
margin:0;
padding:0;
box-sizing: border-box;
}
.nav{
width:800px;
height:100px;
margin: 0 auto;
display: flex;
align-items: center;
}
.nav input{
height: 50px;
width: 600px;
}
.nav button{
height: 50px;
width: 200px;
background-color: orange;
border-radius: 5px;
color:black;
}
.container{
background-color: orange;
width:800px;
height: 800px;
margin: 0 auto;
display: flex;
}
.container h3{
height: 50px;
text-align: center;
line-height: 50px;
background-color: #333;
color:white;
}
.todo{
background-color: darkgray;
width: 400px;
height: 100%;
}
.done{
width:50%;
height: 100%;
}
.row{
height: 50px;
display: flex;
align-items: center;
}
.row input{
margin:0 10px;
}
.row span{
width:300px;
}
.row button{
width:50px;
height:40px;
}
</style>
<script>
let addTaskButton=document.querySelector('.nav button');
addTaskButton.onclick=function () {
let input=document.querySelector('.nav input');
let taskContent=input.value;
if(taskContent==''){
console.log('当前任务为空,无法新增任务!');
return ;
}
let row=document.createElement('div');
row.className='row';
let checkbox=document.createElement('input');
checkbox.type='checkbox';
let span=document.createElement('span');
span.innerHTML=taskContent;
let button=document.createElement('button');
button.innerHTML='删除';
row.appendChild(checkbox);
row.appendChild(span);
row.appendChild(button);
let todo=document.querySelector('.todo');
todo.appendChild(row);
input.value='';
checkbox.onclick=function () {
if(checkbox.checked){
let target=document.querySelector('.done');
target.appendChild(row);
}else{
let target=document.querySelector('.todo');
target.appendChild(row);
}
}
button.onclick=function () {
let parent=row.parentNode;
parent.removeChild(row);
}
}
</script>
</body>
</html>
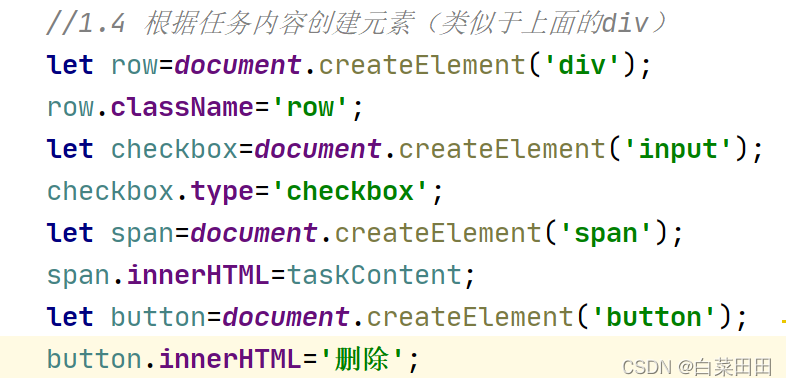
上面这段代码的作用就是由JS直接生成一个HTML片段。
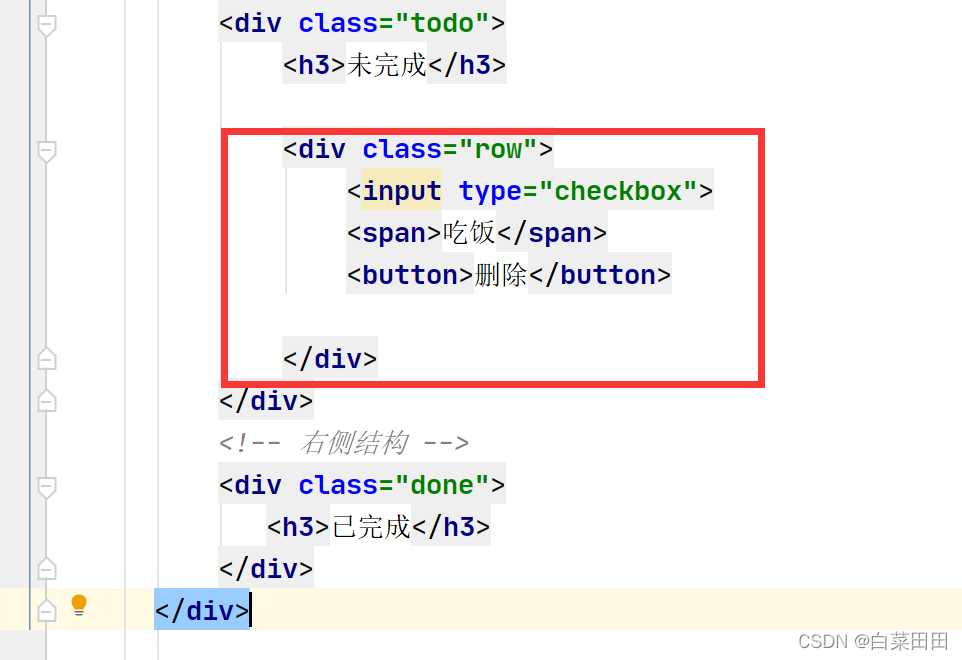 运行效果: 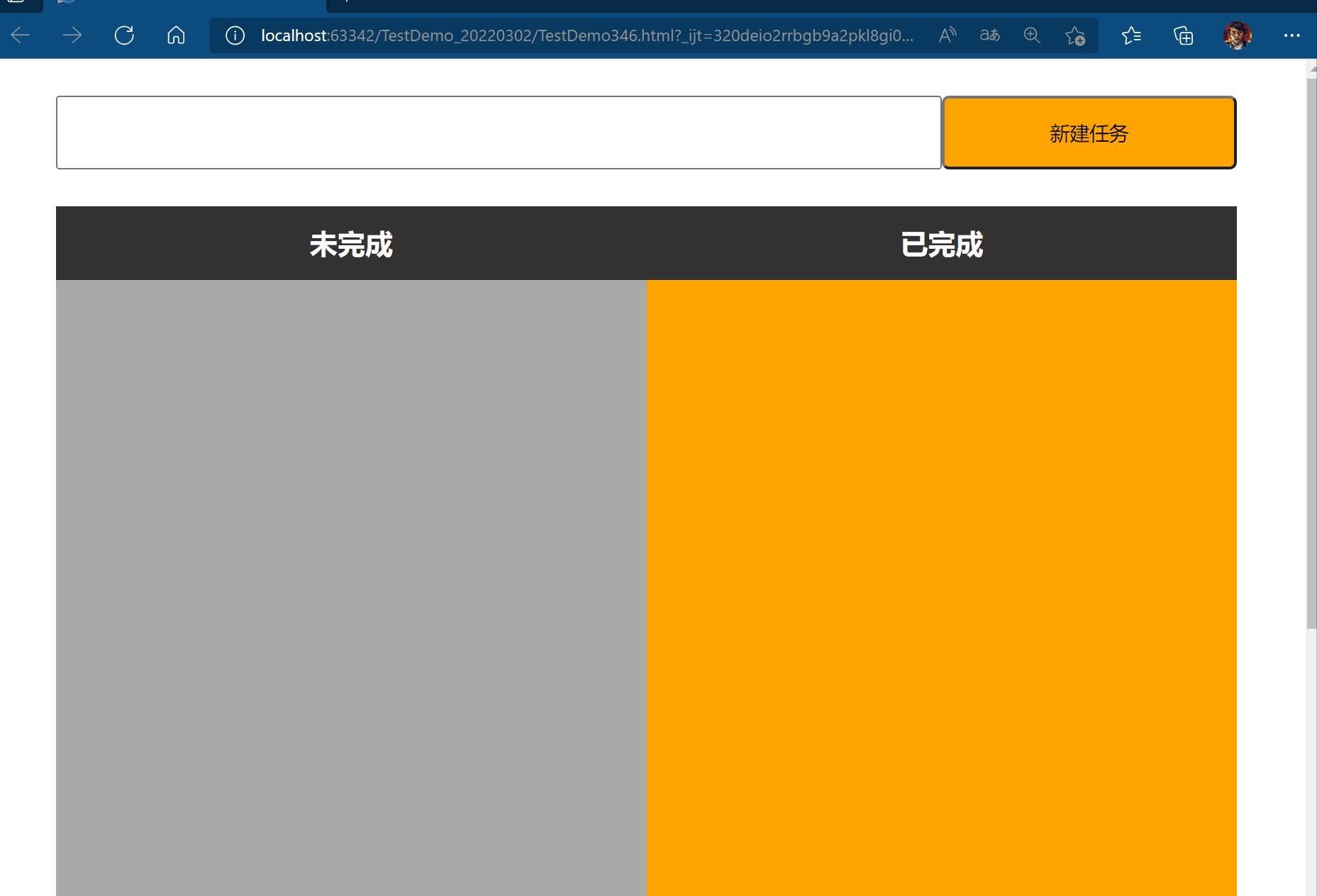
|