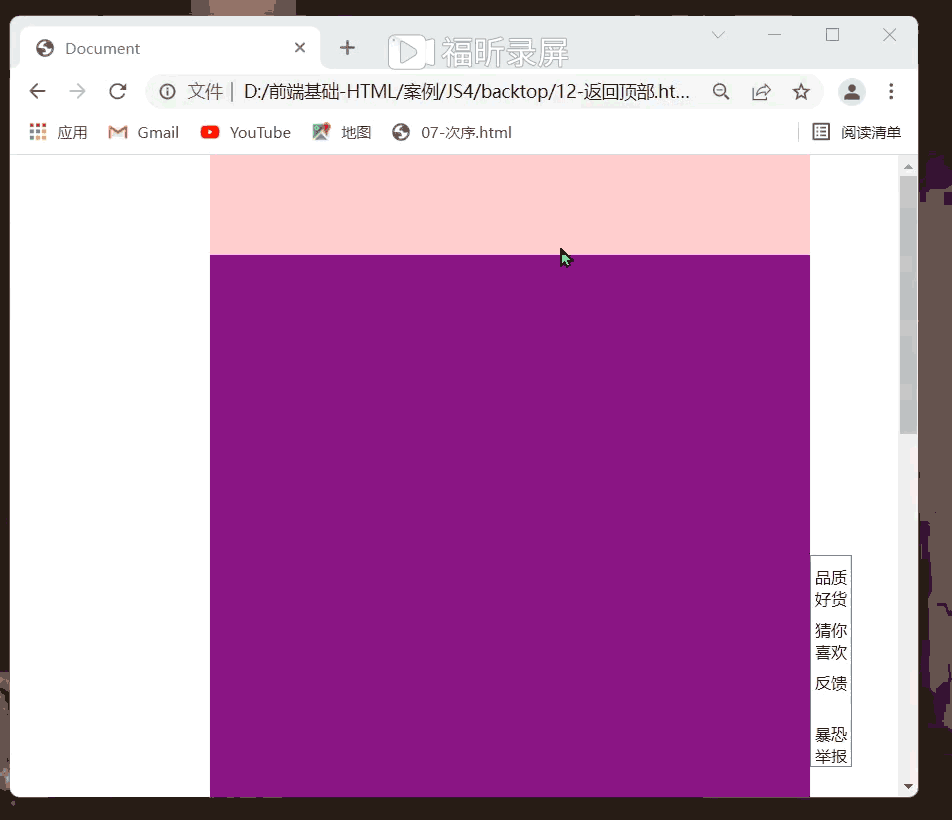
?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0px
}
.box {
width: 600px;
height: auto;
margin-left: 200px;
position: relative;
}
.top {
width: 600px;
height: 100px;
background-color: pink;
}
.body1 {
width: 600px;
height: 900px;
background-color: purple;
}
.nav {
display: none;
width: 600px;
height: 100px;
background-color: red;
position: sticky;
top: 0px
}
.bottom {
width: 600px;
height: 500px;
background-color: blue;
}
ul {
position: absolute;
top: 400px;
left: 600px;
width: 40px;
border: 1px solid grey;
padding-left: 0px;
}
li {
list-style: none;
width: 40px;
height: 41px;
margin: 0px;
text-align: center;
display: inline-block;
line-height: 22px;
margin-top: 10px
}
a {
color: black;
text-decoration: none;
}
.top1 {
display: none
}
</style>
<script src="backtop.js"></script>
</head>
<body>
<div class="box" id="two">
<div class="top"></div>
<div class="nav"></div>
<div class="body1"></div>
<div class="bottom"></div>
<ul>
<li><a href="javascrip:;">品质好货</a>
</li>
<li><a href="javascrip:;">猜你喜欢</a></li>
<li class="top1"><a href="#two">顶部</a></li>
<li><a href="javascrip:;">反馈</a></li>
<li><a href="javascrip:;">暴恐举报</a></li>
</ul>
</div>
</body>
</html>
window.addEventListener('load', function() {
//获得nav
var nav = document.querySelector('.nav')
var ul = document.querySelector('ul')
var top1 = document.querySelector('.top1')
console.log(ul.offsetLeft)
document.addEventListener('scroll', function() {
console.log(window.pageYOffset)
if (window.pageYOffset >= 100) {
nav.style.display = 'block'
} else {
nav.style.display = 'none'
}
if (window.pageYOffset >= 400) {
ul.style.position = 'fixed'
ul.style.top = 0 + 'px'
ul.style.left = 800 + 'px'
} else {
ul.style.position = 'absolute'
ul.style.top = 400 + 'px'
ul.style.left = 600 + 'px'
}
if (window.pageYOffset >= 560) {
top1.style.display = 'block'
} else {
top1.style.display = 'none'
}
})
//给顶部添加点击事件
// top1.addEventListener('click', function() {
// alert(1)
// window.pageYOffset = 0
// })
})
不用锚点链接时
滚动窗口到文档中的特定位置:window.scroll(x,y)
//给顶部添加点击事件
top1.addEventListener('click', function() {
window.scroll(0, 0)
})
可以取得一样的效果
要滚动慢慢的滚动到页面顶部:
1、继续使用封装好的动画函数
2、把所有的left改为与页面垂直相关即可
3、页面滚动了多少,window.pageYOffset()
4、其次就是页面滚动,使用window.scroll(x,y)
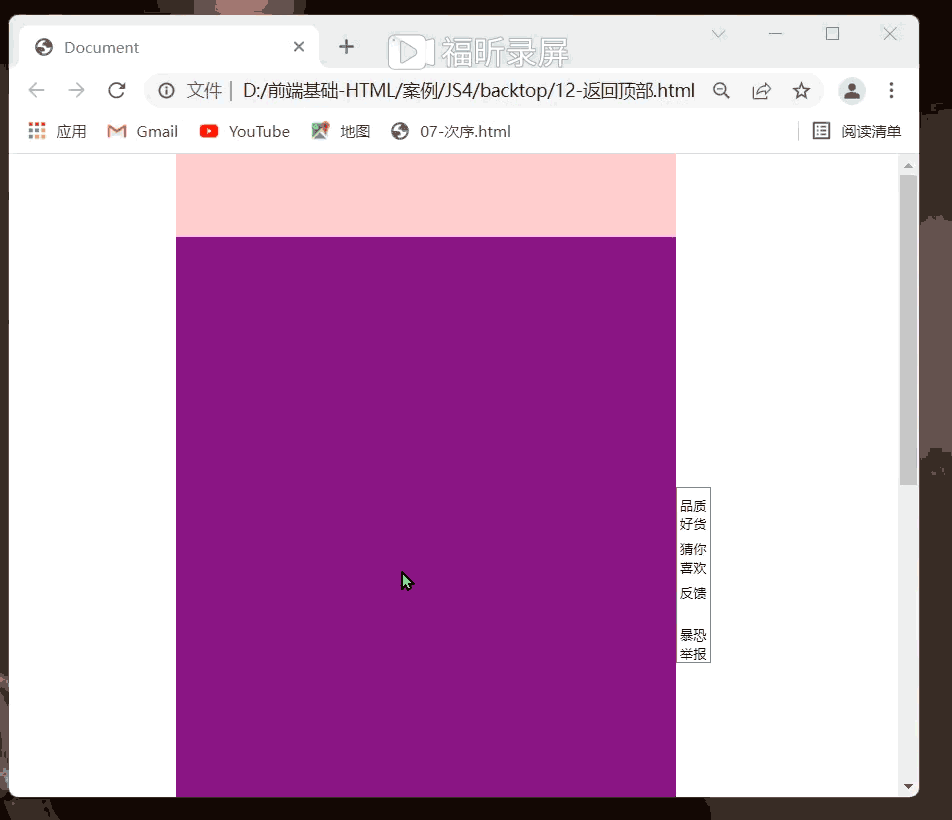
?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0px
}
.box {
width: 600px;
height: auto;
margin-left: 200px;
position: relative;
}
.top {
width: 600px;
height: 100px;
background-color: pink;
}
.body1 {
width: 600px;
height: 900px;
background-color: purple;
}
.nav {
display: none;
width: 600px;
height: 100px;
background-color: red;
position: sticky;
top: 0px
}
.bottom {
width: 600px;
height: 500px;
background-color: blue;
}
ul {
position: absolute;
top: 400px;
left: 600px;
width: 40px;
border: 1px solid grey;
padding-left: 0px;
}
li {
list-style: none;
width: 40px;
height: 41px;
margin: 0px;
text-align: center;
display: inline-block;
line-height: 22px;
margin-top: 10px
}
a {
color: black;
text-decoration: none;
}
.top1 {
display: none
}
</style>
<script src="backtop.js"></script>
<script src="animater.js"></script>
</head>
<body>
<div class="box">
<div class="top"></div>
<div class="nav"></div>
<div class="body1"></div>
<div class="bottom"></div>
<ul>
<li><a href="javascrip:;">品质好货</a>
</li>
<li><a href="javascrip:;">猜你喜欢</a></li>
<li class="top1"><a href="javascrip:;">顶部</a></li>
<li><a href="javascrip:;">反馈</a></li>
<li><a href="javascrip:;">暴恐举报</a></li>
</ul>
</div>
</body>
</html>
//给顶部添加点击事件
top1.addEventListener('click', function() {
// window.scroll(0, 0)
//因为是窗口滚动,所以对象是window。注意不是document
animate(window, 0)
})
function animate(obj, target, callback) {
clearInterval(obj.timer) //先把原先地定时器清除之后,再开启另外一个新地定时器
obj.timer = setInterval(fn, [15])
function fn() {
var a = window.pageYOffset
var step = (target - a) / 10
step = step > 0 ? Math.ceil(step) : Math.floor(step) //将结果赋值回去
//把步长值改为整数,不要出现小数的情况
if (a == target) {
//取消定时器
clearInterval(obj.timer)
//执行回调函数 函数名+()回调函数写到定时器结束里面
//首先判断没有有这个回调函数
if (callback) {
callback()
}
}
// obj.style.left = a + step + 'px'
window.scroll(0, a + step)
}
}
|